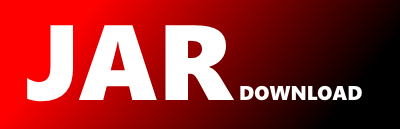
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupMixedInstancesPolicyLaunchTemplateOverrideInstanceRequirement.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.autoscaling.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property acceleratorCounts
* @property acceleratorManufacturers List of accelerator manufacturer names.
* @property acceleratorNames List of accelerator names.
* @property acceleratorTotalMemoryMibs List of objects describing the minimum and maximum total memory of the accelerators.
* @property acceleratorTypes List of accelerator types.
* @property allowedInstanceTypes List of instance types to apply the specified attributes against.
* @property bareMetal Indicates whether bare metal instances are included, excluded, or required.
* @property baselineEbsBandwidthMbps List of objects describing the minimum and maximum baseline EBS bandwidth (Mbps).
* @property burstablePerformance Indicates whether burstable performance instance types are included, excluded, or required.
* @property cpuManufacturers List of CPU manufacturer names.
* @property excludedInstanceTypes List of excluded instance types.
* @property instanceGenerations List of instance generation names.
* @property localStorage Indicates whether instance types with instance store volumes are included, excluded, or required.
* @property localStorageTypes List of local storage type names.
* @property maxSpotPriceAsPercentageOfOptimalOnDemandPrice Price protection threshold for Spot Instances.
* @property memoryGibPerVcpus List of objects describing the minimum and maximum amount of memory (GiB) per vCPU.
* @property memoryMibs List of objects describing the minimum and maximum amount of memory (MiB).
* @property networkBandwidthGbps List of objects describing the minimum and maximum amount of network bandwidth (Gbps).
* @property networkInterfaceCounts List of objects describing the minimum and maximum amount of network interfaces.
* @property onDemandMaxPricePercentageOverLowestPrice Price protection threshold for On-Demand Instances.
* @property requireHibernateSupport Indicates whether instance types must support On-Demand Instance Hibernation.
* @property spotMaxPricePercentageOverLowestPrice Price protection threshold for Spot Instances.
* @property totalLocalStorageGbs List of objects describing the minimum and maximum total storage (GB).
* @property vcpuCounts List of objects describing the minimum and maximum number of vCPUs.
*/
public data class GetGroupMixedInstancesPolicyLaunchTemplateOverrideInstanceRequirement(
public val acceleratorCounts: List,
public val acceleratorManufacturers: List,
public val acceleratorNames: List,
public val acceleratorTotalMemoryMibs: List,
public val acceleratorTypes: List,
public val allowedInstanceTypes: List,
public val bareMetal: String,
public val baselineEbsBandwidthMbps: List,
public val burstablePerformance: String,
public val cpuManufacturers: List,
public val excludedInstanceTypes: List,
public val instanceGenerations: List,
public val localStorage: String,
public val localStorageTypes: List,
public val maxSpotPriceAsPercentageOfOptimalOnDemandPrice: Int,
public val memoryGibPerVcpus: List,
public val memoryMibs: List,
public val networkBandwidthGbps: List,
public val networkInterfaceCounts: List,
public val onDemandMaxPricePercentageOverLowestPrice: Int,
public val requireHibernateSupport: Boolean,
public val spotMaxPricePercentageOverLowestPrice: Int,
public val totalLocalStorageGbs: List,
public val vcpuCounts: List,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.autoscaling.outputs.GetGroupMixedInstancesPolicyLaunchTemplateOverrideInstanceRequirement): GetGroupMixedInstancesPolicyLaunchTemplateOverrideInstanceRequirement =
GetGroupMixedInstancesPolicyLaunchTemplateOverrideInstanceRequirement(
acceleratorCounts = javaType.acceleratorCounts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupMixedInstancesPolicyLaunchTemplateOverrideInstanceRequirementAcceleratorCount.Companion.toKotlin(args0)
})
}),
acceleratorManufacturers = javaType.acceleratorManufacturers().map({ args0 -> args0 }),
acceleratorNames = javaType.acceleratorNames().map({ args0 -> args0 }),
acceleratorTotalMemoryMibs = javaType.acceleratorTotalMemoryMibs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupMixedInstancesPolicyLaunchTemplateOverrideInstanceRequirementAcceleratorTotalMemoryMib.Companion.toKotlin(args0)
})
}),
acceleratorTypes = javaType.acceleratorTypes().map({ args0 -> args0 }),
allowedInstanceTypes = javaType.allowedInstanceTypes().map({ args0 -> args0 }),
bareMetal = javaType.bareMetal(),
baselineEbsBandwidthMbps = javaType.baselineEbsBandwidthMbps().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupMixedInstancesPolicyLaunchTemplateOverrideInstanceRequirementBaselineEbsBandwidthMbp.Companion.toKotlin(args0)
})
}),
burstablePerformance = javaType.burstablePerformance(),
cpuManufacturers = javaType.cpuManufacturers().map({ args0 -> args0 }),
excludedInstanceTypes = javaType.excludedInstanceTypes().map({ args0 -> args0 }),
instanceGenerations = javaType.instanceGenerations().map({ args0 -> args0 }),
localStorage = javaType.localStorage(),
localStorageTypes = javaType.localStorageTypes().map({ args0 -> args0 }),
maxSpotPriceAsPercentageOfOptimalOnDemandPrice = javaType.maxSpotPriceAsPercentageOfOptimalOnDemandPrice(),
memoryGibPerVcpus = javaType.memoryGibPerVcpus().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupMixedInstancesPolicyLaunchTemplateOverrideInstanceRequirementMemoryGibPerVcpus.Companion.toKotlin(args0)
})
}),
memoryMibs = javaType.memoryMibs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupMixedInstancesPolicyLaunchTemplateOverrideInstanceRequirementMemoryMib.Companion.toKotlin(args0)
})
}),
networkBandwidthGbps = javaType.networkBandwidthGbps().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupMixedInstancesPolicyLaunchTemplateOverrideInstanceRequirementNetworkBandwidthGbp.Companion.toKotlin(args0)
})
}),
networkInterfaceCounts = javaType.networkInterfaceCounts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupMixedInstancesPolicyLaunchTemplateOverrideInstanceRequirementNetworkInterfaceCount.Companion.toKotlin(args0)
})
}),
onDemandMaxPricePercentageOverLowestPrice = javaType.onDemandMaxPricePercentageOverLowestPrice(),
requireHibernateSupport = javaType.requireHibernateSupport(),
spotMaxPricePercentageOverLowestPrice = javaType.spotMaxPricePercentageOverLowestPrice(),
totalLocalStorageGbs = javaType.totalLocalStorageGbs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupMixedInstancesPolicyLaunchTemplateOverrideInstanceRequirementTotalLocalStorageGb.Companion.toKotlin(args0)
})
}),
vcpuCounts = javaType.vcpuCounts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupMixedInstancesPolicyLaunchTemplateOverrideInstanceRequirementVcpuCount.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy