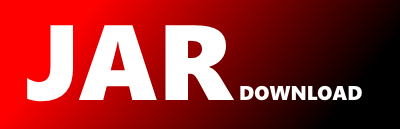
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.autoscaling.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* A collection of values returned by getGroup.
* @property arn ARN of the Auto Scaling group.
* @property availabilityZones One or more Availability Zones for the group.
* @property defaultCooldown
* @property desiredCapacity Desired size of the group.
* @property desiredCapacityType The unit of measurement for the value returned for `desired_capacity`.
* @property enabledMetrics List of metrics enabled for collection.
* @property healthCheckGracePeriod The amount of time, in seconds, that Amazon EC2 Auto Scaling waits before checking the health status of an EC2 instance that has come into service.
* @property healthCheckType Service to use for the health checks. The valid values are EC2 and ELB.
* @property id The provider-assigned unique ID for this managed resource.
* @property instanceMaintenancePolicies Instance maintenance policy for the group.
* @property launchConfiguration The name of the associated launch configuration.
* @property launchTemplates List of launch templates along with the overrides.
* @property loadBalancers One or more load balancers associated with the group.
* @property maxInstanceLifetime Maximum amount of time, in seconds, that an instance can be in service.
* @property maxSize Maximum size of the group.
* @property minSize Minimum number of instances to maintain in the warm pool.
* @property mixedInstancesPolicies List of mixed instances policy objects for the group.
* @property name Name of the Auto Scaling Group.
* @property newInstancesProtectedFromScaleIn
* @property placementGroup Name of the placement group into which to launch your instances, if any. For more information, see Placement Groups (http://docs.aws.amazon.com/AWSEC2/latest/UserGuide/placement-groups.html) in the Amazon Elastic Compute Cloud User Guide.
* @property predictedCapacity Predicted capacity of the group.
* @property serviceLinkedRoleArn ARN of the service-linked role that the Auto Scaling group uses to call other AWS services on your behalf.
* @property status Current state of the group when DeleteAutoScalingGroup is in progress.
* @property suspendedProcesses List of processes suspended processes for the Auto Scaling Group.
* @property tags List of tags for the group.
* @property targetGroupArns ARNs of the target groups for your load balancer.
* @property terminationPolicies The termination policies for the group.
* @property trafficSources Traffic sources.
* @property vpcZoneIdentifier VPC ID for the group.
* @property warmPoolSize Current size of the warm pool.
* @property warmPools List of warm pool configuration objects.
*/
public data class GetGroupResult(
public val arn: String,
public val availabilityZones: List,
public val defaultCooldown: Int,
public val desiredCapacity: Int,
public val desiredCapacityType: String,
public val enabledMetrics: List,
public val healthCheckGracePeriod: Int,
public val healthCheckType: String,
public val id: String,
public val instanceMaintenancePolicies: List,
public val launchConfiguration: String,
public val launchTemplates: List,
public val loadBalancers: List,
public val maxInstanceLifetime: Int,
public val maxSize: Int,
public val minSize: Int,
public val mixedInstancesPolicies: List,
public val name: String,
public val newInstancesProtectedFromScaleIn: Boolean,
public val placementGroup: String,
public val predictedCapacity: Int,
public val serviceLinkedRoleArn: String,
public val status: String,
public val suspendedProcesses: List,
public val tags: List,
public val targetGroupArns: List,
public val terminationPolicies: List,
public val trafficSources: List,
public val vpcZoneIdentifier: String,
public val warmPoolSize: Int,
public val warmPools: List,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.autoscaling.outputs.GetGroupResult): GetGroupResult = GetGroupResult(
arn = javaType.arn(),
availabilityZones = javaType.availabilityZones().map({ args0 -> args0 }),
defaultCooldown = javaType.defaultCooldown(),
desiredCapacity = javaType.desiredCapacity(),
desiredCapacityType = javaType.desiredCapacityType(),
enabledMetrics = javaType.enabledMetrics().map({ args0 -> args0 }),
healthCheckGracePeriod = javaType.healthCheckGracePeriod(),
healthCheckType = javaType.healthCheckType(),
id = javaType.id(),
instanceMaintenancePolicies = javaType.instanceMaintenancePolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupInstanceMaintenancePolicy.Companion.toKotlin(args0)
})
}),
launchConfiguration = javaType.launchConfiguration(),
launchTemplates = javaType.launchTemplates().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupLaunchTemplate.Companion.toKotlin(args0)
})
}),
loadBalancers = javaType.loadBalancers().map({ args0 -> args0 }),
maxInstanceLifetime = javaType.maxInstanceLifetime(),
maxSize = javaType.maxSize(),
minSize = javaType.minSize(),
mixedInstancesPolicies = javaType.mixedInstancesPolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupMixedInstancesPolicy.Companion.toKotlin(args0)
})
}),
name = javaType.name(),
newInstancesProtectedFromScaleIn = javaType.newInstancesProtectedFromScaleIn(),
placementGroup = javaType.placementGroup(),
predictedCapacity = javaType.predictedCapacity(),
serviceLinkedRoleArn = javaType.serviceLinkedRoleArn(),
status = javaType.status(),
suspendedProcesses = javaType.suspendedProcesses().map({ args0 -> args0 }),
tags = javaType.tags().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupTag.Companion.toKotlin(args0)
})
}),
targetGroupArns = javaType.targetGroupArns().map({ args0 -> args0 }),
terminationPolicies = javaType.terminationPolicies().map({ args0 -> args0 }),
trafficSources = javaType.trafficSources().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupTrafficSource.Companion.toKotlin(args0)
})
}),
vpcZoneIdentifier = javaType.vpcZoneIdentifier(),
warmPoolSize = javaType.warmPoolSize(),
warmPools = javaType.warmPools().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscaling.kotlin.outputs.GetGroupWarmPool.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy