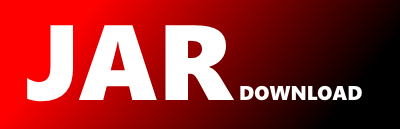
com.pulumi.aws.autoscalingplans.kotlin.inputs.ScalingPlanScalingInstructionArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.autoscalingplans.kotlin.inputs
import com.pulumi.aws.autoscalingplans.inputs.ScalingPlanScalingInstructionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property customizedLoadMetricSpecification Customized load metric to use for predictive scaling. You must specify either `customized_load_metric_specification` or `predefined_load_metric_specification` when configuring predictive scaling.
* More details can be found in the [AWS Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/plans/APIReference/API_CustomizedLoadMetricSpecification.html).
* @property disableDynamicScaling Boolean controlling whether dynamic scaling by AWS Auto Scaling is disabled. Defaults to `false`.
* @property maxCapacity Maximum capacity of the resource. The exception to this upper limit is if you specify a non-default setting for `predictive_scaling_max_capacity_behavior`.
* @property minCapacity Minimum capacity of the resource.
* @property predefinedLoadMetricSpecification Predefined load metric to use for predictive scaling. You must specify either `predefined_load_metric_specification` or `customized_load_metric_specification` when configuring predictive scaling.
* More details can be found in the [AWS Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/plans/APIReference/API_PredefinedLoadMetricSpecification.html).
* @property predictiveScalingMaxCapacityBehavior Defines the behavior that should be applied if the forecast capacity approaches or exceeds the maximum capacity specified for the resource.
* Valid values: `SetForecastCapacityToMaxCapacity`, `SetMaxCapacityAboveForecastCapacity`, `SetMaxCapacityToForecastCapacity`.
* @property predictiveScalingMaxCapacityBuffer Size of the capacity buffer to use when the forecast capacity is close to or exceeds the maximum capacity.
* @property predictiveScalingMode Predictive scaling mode. Valid values: `ForecastAndScale`, `ForecastOnly`.
* @property resourceId ID of the resource. This string consists of the resource type and unique identifier.
* @property scalableDimension Scalable dimension associated with the resource. Valid values: `autoscaling:autoScalingGroup:DesiredCapacity`, `dynamodb:index:ReadCapacityUnits`, `dynamodb:index:WriteCapacityUnits`, `dynamodb:table:ReadCapacityUnits`, `dynamodb:table:WriteCapacityUnits`, `ecs:service:DesiredCount`, `ec2:spot-fleet-request:TargetCapacity`, `rds:cluster:ReadReplicaCount`.
* @property scalingPolicyUpdateBehavior Controls whether a resource's externally created scaling policies are kept or replaced. Valid values: `KeepExternalPolicies`, `ReplaceExternalPolicies`. Defaults to `KeepExternalPolicies`.
* @property scheduledActionBufferTime Amount of time, in seconds, to buffer the run time of scheduled scaling actions when scaling out.
* @property serviceNamespace Namespace of the AWS service. Valid values: `autoscaling`, `dynamodb`, `ecs`, `ec2`, `rds`.
* @property targetTrackingConfigurations Structure that defines new target tracking configurations. Each of these structures includes a specific scaling metric and a target value for the metric, along with various parameters to use with dynamic scaling.
* More details can be found in the [AWS Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/plans/APIReference/API_TargetTrackingConfiguration.html).
*/
public data class ScalingPlanScalingInstructionArgs(
public val customizedLoadMetricSpecification: Output? = null,
public val disableDynamicScaling: Output? = null,
public val maxCapacity: Output,
public val minCapacity: Output,
public val predefinedLoadMetricSpecification: Output? = null,
public val predictiveScalingMaxCapacityBehavior: Output? = null,
public val predictiveScalingMaxCapacityBuffer: Output? = null,
public val predictiveScalingMode: Output? = null,
public val resourceId: Output,
public val scalableDimension: Output,
public val scalingPolicyUpdateBehavior: Output? = null,
public val scheduledActionBufferTime: Output? = null,
public val serviceNamespace: Output,
public val targetTrackingConfigurations: Output>,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.autoscalingplans.inputs.ScalingPlanScalingInstructionArgs =
com.pulumi.aws.autoscalingplans.inputs.ScalingPlanScalingInstructionArgs.builder()
.customizedLoadMetricSpecification(
customizedLoadMetricSpecification?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.disableDynamicScaling(disableDynamicScaling?.applyValue({ args0 -> args0 }))
.maxCapacity(maxCapacity.applyValue({ args0 -> args0 }))
.minCapacity(minCapacity.applyValue({ args0 -> args0 }))
.predefinedLoadMetricSpecification(
predefinedLoadMetricSpecification?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.predictiveScalingMaxCapacityBehavior(
predictiveScalingMaxCapacityBehavior?.applyValue({ args0 ->
args0
}),
)
.predictiveScalingMaxCapacityBuffer(
predictiveScalingMaxCapacityBuffer?.applyValue({ args0 ->
args0
}),
)
.predictiveScalingMode(predictiveScalingMode?.applyValue({ args0 -> args0 }))
.resourceId(resourceId.applyValue({ args0 -> args0 }))
.scalableDimension(scalableDimension.applyValue({ args0 -> args0 }))
.scalingPolicyUpdateBehavior(scalingPolicyUpdateBehavior?.applyValue({ args0 -> args0 }))
.scheduledActionBufferTime(scheduledActionBufferTime?.applyValue({ args0 -> args0 }))
.serviceNamespace(serviceNamespace.applyValue({ args0 -> args0 }))
.targetTrackingConfigurations(
targetTrackingConfigurations.applyValue({ args0 ->
args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
).build()
}
/**
* Builder for [ScalingPlanScalingInstructionArgs].
*/
@PulumiTagMarker
public class ScalingPlanScalingInstructionArgsBuilder internal constructor() {
private var customizedLoadMetricSpecification:
Output? = null
private var disableDynamicScaling: Output? = null
private var maxCapacity: Output? = null
private var minCapacity: Output? = null
private var predefinedLoadMetricSpecification:
Output? = null
private var predictiveScalingMaxCapacityBehavior: Output? = null
private var predictiveScalingMaxCapacityBuffer: Output? = null
private var predictiveScalingMode: Output? = null
private var resourceId: Output? = null
private var scalableDimension: Output? = null
private var scalingPolicyUpdateBehavior: Output? = null
private var scheduledActionBufferTime: Output? = null
private var serviceNamespace: Output? = null
private var targetTrackingConfigurations:
Output>? = null
/**
* @param value Customized load metric to use for predictive scaling. You must specify either `customized_load_metric_specification` or `predefined_load_metric_specification` when configuring predictive scaling.
* More details can be found in the [AWS Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/plans/APIReference/API_CustomizedLoadMetricSpecification.html).
*/
@JvmName("jddmehacipfvarte")
public suspend fun customizedLoadMetricSpecification(`value`: Output) {
this.customizedLoadMetricSpecification = value
}
/**
* @param value Boolean controlling whether dynamic scaling by AWS Auto Scaling is disabled. Defaults to `false`.
*/
@JvmName("hkelrqxmyhxbfwpe")
public suspend fun disableDynamicScaling(`value`: Output) {
this.disableDynamicScaling = value
}
/**
* @param value Maximum capacity of the resource. The exception to this upper limit is if you specify a non-default setting for `predictive_scaling_max_capacity_behavior`.
*/
@JvmName("sbtmifgrepkvnreb")
public suspend fun maxCapacity(`value`: Output) {
this.maxCapacity = value
}
/**
* @param value Minimum capacity of the resource.
*/
@JvmName("xybburqbywhcbjmq")
public suspend fun minCapacity(`value`: Output) {
this.minCapacity = value
}
/**
* @param value Predefined load metric to use for predictive scaling. You must specify either `predefined_load_metric_specification` or `customized_load_metric_specification` when configuring predictive scaling.
* More details can be found in the [AWS Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/plans/APIReference/API_PredefinedLoadMetricSpecification.html).
*/
@JvmName("eqcebhcupmkknvmx")
public suspend fun predefinedLoadMetricSpecification(`value`: Output) {
this.predefinedLoadMetricSpecification = value
}
/**
* @param value Defines the behavior that should be applied if the forecast capacity approaches or exceeds the maximum capacity specified for the resource.
* Valid values: `SetForecastCapacityToMaxCapacity`, `SetMaxCapacityAboveForecastCapacity`, `SetMaxCapacityToForecastCapacity`.
*/
@JvmName("tnaaemcgowwtqbsq")
public suspend fun predictiveScalingMaxCapacityBehavior(`value`: Output) {
this.predictiveScalingMaxCapacityBehavior = value
}
/**
* @param value Size of the capacity buffer to use when the forecast capacity is close to or exceeds the maximum capacity.
*/
@JvmName("vnmrnhwwhkgxneqk")
public suspend fun predictiveScalingMaxCapacityBuffer(`value`: Output) {
this.predictiveScalingMaxCapacityBuffer = value
}
/**
* @param value Predictive scaling mode. Valid values: `ForecastAndScale`, `ForecastOnly`.
*/
@JvmName("yjmdxumhwnfeolho")
public suspend fun predictiveScalingMode(`value`: Output) {
this.predictiveScalingMode = value
}
/**
* @param value ID of the resource. This string consists of the resource type and unique identifier.
*/
@JvmName("jpoxxypydtdaippu")
public suspend fun resourceId(`value`: Output) {
this.resourceId = value
}
/**
* @param value Scalable dimension associated with the resource. Valid values: `autoscaling:autoScalingGroup:DesiredCapacity`, `dynamodb:index:ReadCapacityUnits`, `dynamodb:index:WriteCapacityUnits`, `dynamodb:table:ReadCapacityUnits`, `dynamodb:table:WriteCapacityUnits`, `ecs:service:DesiredCount`, `ec2:spot-fleet-request:TargetCapacity`, `rds:cluster:ReadReplicaCount`.
*/
@JvmName("sfsrchqruubifstc")
public suspend fun scalableDimension(`value`: Output) {
this.scalableDimension = value
}
/**
* @param value Controls whether a resource's externally created scaling policies are kept or replaced. Valid values: `KeepExternalPolicies`, `ReplaceExternalPolicies`. Defaults to `KeepExternalPolicies`.
*/
@JvmName("bothkaahbljwumub")
public suspend fun scalingPolicyUpdateBehavior(`value`: Output) {
this.scalingPolicyUpdateBehavior = value
}
/**
* @param value Amount of time, in seconds, to buffer the run time of scheduled scaling actions when scaling out.
*/
@JvmName("irhmgcdgompvbsjr")
public suspend fun scheduledActionBufferTime(`value`: Output) {
this.scheduledActionBufferTime = value
}
/**
* @param value Namespace of the AWS service. Valid values: `autoscaling`, `dynamodb`, `ecs`, `ec2`, `rds`.
*/
@JvmName("nnxconejrhfoqitx")
public suspend fun serviceNamespace(`value`: Output) {
this.serviceNamespace = value
}
/**
* @param value Structure that defines new target tracking configurations. Each of these structures includes a specific scaling metric and a target value for the metric, along with various parameters to use with dynamic scaling.
* More details can be found in the [AWS Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/plans/APIReference/API_TargetTrackingConfiguration.html).
*/
@JvmName("kgekyuubbitihubt")
public suspend fun targetTrackingConfigurations(`value`: Output>) {
this.targetTrackingConfigurations = value
}
@JvmName("balaogakjvkosvvi")
public suspend fun targetTrackingConfigurations(vararg values: Output) {
this.targetTrackingConfigurations = Output.all(values.asList())
}
/**
* @param values Structure that defines new target tracking configurations. Each of these structures includes a specific scaling metric and a target value for the metric, along with various parameters to use with dynamic scaling.
* More details can be found in the [AWS Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/plans/APIReference/API_TargetTrackingConfiguration.html).
*/
@JvmName("xvjobigvjntcxiaq")
public suspend fun targetTrackingConfigurations(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy