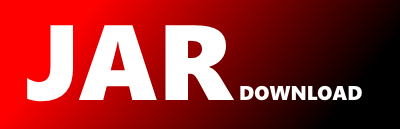
com.pulumi.aws.autoscalingplans.kotlin.outputs.ScalingPlanScalingInstruction.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.autoscalingplans.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property customizedLoadMetricSpecification Customized load metric to use for predictive scaling. You must specify either `customized_load_metric_specification` or `predefined_load_metric_specification` when configuring predictive scaling.
* More details can be found in the [AWS Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/plans/APIReference/API_CustomizedLoadMetricSpecification.html).
* @property disableDynamicScaling Boolean controlling whether dynamic scaling by AWS Auto Scaling is disabled. Defaults to `false`.
* @property maxCapacity Maximum capacity of the resource. The exception to this upper limit is if you specify a non-default setting for `predictive_scaling_max_capacity_behavior`.
* @property minCapacity Minimum capacity of the resource.
* @property predefinedLoadMetricSpecification Predefined load metric to use for predictive scaling. You must specify either `predefined_load_metric_specification` or `customized_load_metric_specification` when configuring predictive scaling.
* More details can be found in the [AWS Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/plans/APIReference/API_PredefinedLoadMetricSpecification.html).
* @property predictiveScalingMaxCapacityBehavior Defines the behavior that should be applied if the forecast capacity approaches or exceeds the maximum capacity specified for the resource.
* Valid values: `SetForecastCapacityToMaxCapacity`, `SetMaxCapacityAboveForecastCapacity`, `SetMaxCapacityToForecastCapacity`.
* @property predictiveScalingMaxCapacityBuffer Size of the capacity buffer to use when the forecast capacity is close to or exceeds the maximum capacity.
* @property predictiveScalingMode Predictive scaling mode. Valid values: `ForecastAndScale`, `ForecastOnly`.
* @property resourceId ID of the resource. This string consists of the resource type and unique identifier.
* @property scalableDimension Scalable dimension associated with the resource. Valid values: `autoscaling:autoScalingGroup:DesiredCapacity`, `dynamodb:index:ReadCapacityUnits`, `dynamodb:index:WriteCapacityUnits`, `dynamodb:table:ReadCapacityUnits`, `dynamodb:table:WriteCapacityUnits`, `ecs:service:DesiredCount`, `ec2:spot-fleet-request:TargetCapacity`, `rds:cluster:ReadReplicaCount`.
* @property scalingPolicyUpdateBehavior Controls whether a resource's externally created scaling policies are kept or replaced. Valid values: `KeepExternalPolicies`, `ReplaceExternalPolicies`. Defaults to `KeepExternalPolicies`.
* @property scheduledActionBufferTime Amount of time, in seconds, to buffer the run time of scheduled scaling actions when scaling out.
* @property serviceNamespace Namespace of the AWS service. Valid values: `autoscaling`, `dynamodb`, `ecs`, `ec2`, `rds`.
* @property targetTrackingConfigurations Structure that defines new target tracking configurations. Each of these structures includes a specific scaling metric and a target value for the metric, along with various parameters to use with dynamic scaling.
* More details can be found in the [AWS Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/plans/APIReference/API_TargetTrackingConfiguration.html).
*/
public data class ScalingPlanScalingInstruction(
public val customizedLoadMetricSpecification: ScalingPlanScalingInstructionCustomizedLoadMetricSpecification? = null,
public val disableDynamicScaling: Boolean? = null,
public val maxCapacity: Int,
public val minCapacity: Int,
public val predefinedLoadMetricSpecification: ScalingPlanScalingInstructionPredefinedLoadMetricSpecification? = null,
public val predictiveScalingMaxCapacityBehavior: String? = null,
public val predictiveScalingMaxCapacityBuffer: Int? = null,
public val predictiveScalingMode: String? = null,
public val resourceId: String,
public val scalableDimension: String,
public val scalingPolicyUpdateBehavior: String? = null,
public val scheduledActionBufferTime: Int? = null,
public val serviceNamespace: String,
public val targetTrackingConfigurations: List,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.autoscalingplans.outputs.ScalingPlanScalingInstruction): ScalingPlanScalingInstruction = ScalingPlanScalingInstruction(
customizedLoadMetricSpecification = javaType.customizedLoadMetricSpecification().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscalingplans.kotlin.outputs.ScalingPlanScalingInstructionCustomizedLoadMetricSpecification.Companion.toKotlin(args0)
})
}).orElse(null),
disableDynamicScaling = javaType.disableDynamicScaling().map({ args0 -> args0 }).orElse(null),
maxCapacity = javaType.maxCapacity(),
minCapacity = javaType.minCapacity(),
predefinedLoadMetricSpecification = javaType.predefinedLoadMetricSpecification().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscalingplans.kotlin.outputs.ScalingPlanScalingInstructionPredefinedLoadMetricSpecification.Companion.toKotlin(args0)
})
}).orElse(null),
predictiveScalingMaxCapacityBehavior = javaType.predictiveScalingMaxCapacityBehavior().map({ args0 ->
args0
}).orElse(null),
predictiveScalingMaxCapacityBuffer = javaType.predictiveScalingMaxCapacityBuffer().map({ args0 ->
args0
}).orElse(null),
predictiveScalingMode = javaType.predictiveScalingMode().map({ args0 -> args0 }).orElse(null),
resourceId = javaType.resourceId(),
scalableDimension = javaType.scalableDimension(),
scalingPolicyUpdateBehavior = javaType.scalingPolicyUpdateBehavior().map({ args0 ->
args0
}).orElse(null),
scheduledActionBufferTime = javaType.scheduledActionBufferTime().map({ args0 ->
args0
}).orElse(null),
serviceNamespace = javaType.serviceNamespace(),
targetTrackingConfigurations = javaType.targetTrackingConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.autoscalingplans.kotlin.outputs.ScalingPlanScalingInstructionTargetTrackingConfiguration.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy