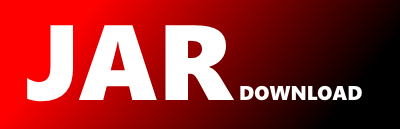
com.pulumi.aws.backup.kotlin.VaultNotificationsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.backup.kotlin
import com.pulumi.aws.backup.VaultNotificationsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Provides an AWS Backup vault notifications resource.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const testTopic = new aws.sns.Topic("test", {name: "backup-vault-events"});
* const test = testTopic.arn.apply(arn => aws.iam.getPolicyDocumentOutput({
* policyId: "__default_policy_ID",
* statements: [{
* actions: ["SNS:Publish"],
* effect: "Allow",
* principals: [{
* type: "Service",
* identifiers: ["backup.amazonaws.com"],
* }],
* resources: [arn],
* sid: "__default_statement_ID",
* }],
* }));
* const testTopicPolicy = new aws.sns.TopicPolicy("test", {
* arn: testTopic.arn,
* policy: test.apply(test => test.json),
* });
* const testVaultNotifications = new aws.backup.VaultNotifications("test", {
* backupVaultName: "example_backup_vault",
* snsTopicArn: testTopic.arn,
* backupVaultEvents: [
* "BACKUP_JOB_STARTED",
* "RESTORE_JOB_COMPLETED",
* ],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* test_topic = aws.sns.Topic("test", name="backup-vault-events")
* test = test_topic.arn.apply(lambda arn: aws.iam.get_policy_document_output(policy_id="__default_policy_ID",
* statements=[{
* "actions": ["SNS:Publish"],
* "effect": "Allow",
* "principals": [{
* "type": "Service",
* "identifiers": ["backup.amazonaws.com"],
* }],
* "resources": [arn],
* "sid": "__default_statement_ID",
* }]))
* test_topic_policy = aws.sns.TopicPolicy("test",
* arn=test_topic.arn,
* policy=test.json)
* test_vault_notifications = aws.backup.VaultNotifications("test",
* backup_vault_name="example_backup_vault",
* sns_topic_arn=test_topic.arn,
* backup_vault_events=[
* "BACKUP_JOB_STARTED",
* "RESTORE_JOB_COMPLETED",
* ])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var testTopic = new Aws.Sns.Topic("test", new()
* {
* Name = "backup-vault-events",
* });
* var test = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* PolicyId = "__default_policy_ID",
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Actions = new[]
* {
* "SNS:Publish",
* },
* Effect = "Allow",
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "Service",
* Identifiers = new[]
* {
* "backup.amazonaws.com",
* },
* },
* },
* Resources = new[]
* {
* testTopic.Arn,
* },
* Sid = "__default_statement_ID",
* },
* },
* });
* var testTopicPolicy = new Aws.Sns.TopicPolicy("test", new()
* {
* Arn = testTopic.Arn,
* Policy = test.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* var testVaultNotifications = new Aws.Backup.VaultNotifications("test", new()
* {
* BackupVaultName = "example_backup_vault",
* SnsTopicArn = testTopic.Arn,
* BackupVaultEvents = new[]
* {
* "BACKUP_JOB_STARTED",
* "RESTORE_JOB_COMPLETED",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/backup"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/sns"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* testTopic, err := sns.NewTopic(ctx, "test", &sns.TopicArgs{
* Name: pulumi.String("backup-vault-events"),
* })
* if err != nil {
* return err
* }
* test := testTopic.Arn.ApplyT(func(arn string) (iam.GetPolicyDocumentResult, error) {
* return iam.GetPolicyDocumentResult(interface{}(iam.GetPolicyDocumentOutput(ctx, iam.GetPolicyDocumentOutputArgs{
* PolicyId: "__default_policy_ID",
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Actions: []string{
* "SNS:Publish",
* },
* Effect: "Allow",
* Principals: []iam.GetPolicyDocumentStatementPrincipal{
* {
* Type: "Service",
* Identifiers: []string{
* "backup.amazonaws.com",
* },
* },
* },
* Resources: interface{}{
* arn,
* },
* Sid: "__default_statement_ID",
* },
* },
* }, nil))), nil
* }).(iam.GetPolicyDocumentResultOutput)
* _, err = sns.NewTopicPolicy(ctx, "test", &sns.TopicPolicyArgs{
* Arn: testTopic.Arn,
* Policy: pulumi.String(test.ApplyT(func(test iam.GetPolicyDocumentResult) (*string, error) {
* return &test.Json, nil
* }).(pulumi.StringPtrOutput)),
* })
* if err != nil {
* return err
* }
* _, err = backup.NewVaultNotifications(ctx, "test", &backup.VaultNotificationsArgs{
* BackupVaultName: pulumi.String("example_backup_vault"),
* SnsTopicArn: testTopic.Arn,
* BackupVaultEvents: pulumi.StringArray{
* pulumi.String("BACKUP_JOB_STARTED"),
* pulumi.String("RESTORE_JOB_COMPLETED"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sns.Topic;
* import com.pulumi.aws.sns.TopicArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.sns.TopicPolicy;
* import com.pulumi.aws.sns.TopicPolicyArgs;
* import com.pulumi.aws.backup.VaultNotifications;
* import com.pulumi.aws.backup.VaultNotificationsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var testTopic = new Topic("testTopic", TopicArgs.builder()
* .name("backup-vault-events")
* .build());
* final var test = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .policyId("__default_policy_ID")
* .statements(GetPolicyDocumentStatementArgs.builder()
* .actions("SNS:Publish")
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("backup.amazonaws.com")
* .build())
* .resources(testTopic.arn())
* .sid("__default_statement_ID")
* .build())
* .build());
* var testTopicPolicy = new TopicPolicy("testTopicPolicy", TopicPolicyArgs.builder()
* .arn(testTopic.arn())
* .policy(test.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult).applyValue(test -> test.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json())))
* .build());
* var testVaultNotifications = new VaultNotifications("testVaultNotifications", VaultNotificationsArgs.builder()
* .backupVaultName("example_backup_vault")
* .snsTopicArn(testTopic.arn())
* .backupVaultEvents(
* "BACKUP_JOB_STARTED",
* "RESTORE_JOB_COMPLETED")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* testTopic:
* type: aws:sns:Topic
* name: test
* properties:
* name: backup-vault-events
* testTopicPolicy:
* type: aws:sns:TopicPolicy
* name: test
* properties:
* arn: ${testTopic.arn}
* policy: ${test.json}
* testVaultNotifications:
* type: aws:backup:VaultNotifications
* name: test
* properties:
* backupVaultName: example_backup_vault
* snsTopicArn: ${testTopic.arn}
* backupVaultEvents:
* - BACKUP_JOB_STARTED
* - RESTORE_JOB_COMPLETED
* variables:
* test:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* policyId: __default_policy_ID
* statements:
* - actions:
* - SNS:Publish
* effect: Allow
* principals:
* - type: Service
* identifiers:
* - backup.amazonaws.com
* resources:
* - ${testTopic.arn}
* sid: __default_statement_ID
* ```
*
* ## Import
* Using `pulumi import`, import Backup vault notifications using the `name`. For example:
* ```sh
* $ pulumi import aws:backup/vaultNotifications:VaultNotifications test TestVault
* ```
* @property backupVaultEvents An array of events that indicate the status of jobs to back up resources to the backup vault.
* @property backupVaultName Name of the backup vault to add notifications for.
* @property snsTopicArn The Amazon Resource Name (ARN) that specifies the topic for a backup vault’s events
*/
public data class VaultNotificationsArgs(
public val backupVaultEvents: Output>? = null,
public val backupVaultName: Output? = null,
public val snsTopicArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.backup.VaultNotificationsArgs =
com.pulumi.aws.backup.VaultNotificationsArgs.builder()
.backupVaultEvents(backupVaultEvents?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.backupVaultName(backupVaultName?.applyValue({ args0 -> args0 }))
.snsTopicArn(snsTopicArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [VaultNotificationsArgs].
*/
@PulumiTagMarker
public class VaultNotificationsArgsBuilder internal constructor() {
private var backupVaultEvents: Output>? = null
private var backupVaultName: Output? = null
private var snsTopicArn: Output? = null
/**
* @param value An array of events that indicate the status of jobs to back up resources to the backup vault.
*/
@JvmName("cwibijlhetqswrmn")
public suspend fun backupVaultEvents(`value`: Output>) {
this.backupVaultEvents = value
}
@JvmName("nalxiopumuutpsri")
public suspend fun backupVaultEvents(vararg values: Output) {
this.backupVaultEvents = Output.all(values.asList())
}
/**
* @param values An array of events that indicate the status of jobs to back up resources to the backup vault.
*/
@JvmName("wwoviwnwkoxsvilg")
public suspend fun backupVaultEvents(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy