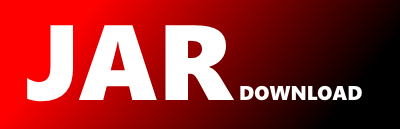
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionNodePropertyNodeRangePropertyContainer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.batch.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property commands The command that's passed to the container.
* @property environments The environment variables to pass to a container.
* @property ephemeralStorages The amount of ephemeral storage to allocate for the task. This parameter is used to expand the total amount of ephemeral storage available, beyond the default amount, for tasks hosted on AWS Fargate.
* @property executionRoleArn The Amazon Resource Name (ARN) of the execution role that AWS Batch can assume. For jobs that run on Fargate resources, you must provide an execution role.
* @property fargatePlatformConfigurations The platform configuration for jobs that are running on Fargate resources. Jobs that are running on EC2 resources must not specify this parameter.
* @property image The image used to start a container.
* @property instanceType The instance type to use for a multi-node parallel job.
* @property jobRoleArn The Amazon Resource Name (ARN) of the IAM role that the container can assume for AWS permissions.
* @property linuxParameters Linux-specific modifications that are applied to the container.
* @property logConfigurations The log configuration specification for the container.
* @property mountPoints The mount points for data volumes in your container.
* @property networkConfigurations The network configuration for jobs that are running on Fargate resources.
* @property privileged When this parameter is true, the container is given elevated permissions on the host container instance (similar to the root user).
* @property readonlyRootFilesystem When this parameter is true, the container is given read-only access to its root file system.
* @property resourceRequirements The type and amount of resources to assign to a container.
* @property runtimePlatforms An object that represents the compute environment architecture for AWS Batch jobs on Fargate.
* @property secrets The secrets for the container.
* @property ulimits A list of ulimits to set in the container.
* @property user The user name to use inside the container.
* @property volumes A list of data volumes used in a job.
*/
public data class GetJobDefinitionNodePropertyNodeRangePropertyContainer(
public val commands: List,
public val environments: List,
public val ephemeralStorages: List,
public val executionRoleArn: String,
public val fargatePlatformConfigurations: List,
public val image: String,
public val instanceType: String,
public val jobRoleArn: String,
public val linuxParameters: List,
public val logConfigurations: List,
public val mountPoints: List,
public val networkConfigurations: List,
public val privileged: Boolean,
public val readonlyRootFilesystem: Boolean,
public val resourceRequirements: List,
public val runtimePlatforms: List,
public val secrets: List,
public val ulimits: List,
public val user: String,
public val volumes: List,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.batch.outputs.GetJobDefinitionNodePropertyNodeRangePropertyContainer): GetJobDefinitionNodePropertyNodeRangePropertyContainer =
GetJobDefinitionNodePropertyNodeRangePropertyContainer(
commands = javaType.commands().map({ args0 -> args0 }),
environments = javaType.environments().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionNodePropertyNodeRangePropertyContainerEnvironment.Companion.toKotlin(args0)
})
}),
ephemeralStorages = javaType.ephemeralStorages().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionNodePropertyNodeRangePropertyContainerEphemeralStorage.Companion.toKotlin(args0)
})
}),
executionRoleArn = javaType.executionRoleArn(),
fargatePlatformConfigurations = javaType.fargatePlatformConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionNodePropertyNodeRangePropertyContainerFargatePlatformConfiguration.Companion.toKotlin(args0)
})
}),
image = javaType.image(),
instanceType = javaType.instanceType(),
jobRoleArn = javaType.jobRoleArn(),
linuxParameters = javaType.linuxParameters().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionNodePropertyNodeRangePropertyContainerLinuxParameter.Companion.toKotlin(args0)
})
}),
logConfigurations = javaType.logConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionNodePropertyNodeRangePropertyContainerLogConfiguration.Companion.toKotlin(args0)
})
}),
mountPoints = javaType.mountPoints().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionNodePropertyNodeRangePropertyContainerMountPoint.Companion.toKotlin(args0)
})
}),
networkConfigurations = javaType.networkConfigurations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionNodePropertyNodeRangePropertyContainerNetworkConfiguration.Companion.toKotlin(args0)
})
}),
privileged = javaType.privileged(),
readonlyRootFilesystem = javaType.readonlyRootFilesystem(),
resourceRequirements = javaType.resourceRequirements().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionNodePropertyNodeRangePropertyContainerResourceRequirement.Companion.toKotlin(args0)
})
}),
runtimePlatforms = javaType.runtimePlatforms().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionNodePropertyNodeRangePropertyContainerRuntimePlatform.Companion.toKotlin(args0)
})
}),
secrets = javaType.secrets().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionNodePropertyNodeRangePropertyContainerSecret.Companion.toKotlin(args0)
})
}),
ulimits = javaType.ulimits().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionNodePropertyNodeRangePropertyContainerUlimit.Companion.toKotlin(args0)
})
}),
user = javaType.user(),
volumes = javaType.volumes().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionNodePropertyNodeRangePropertyContainerVolume.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy