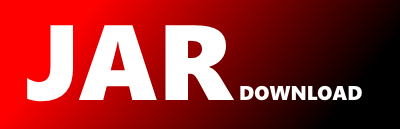
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.batch.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getJobDefinition.
* @property arn
* @property arnPrefix
* @property containerOrchestrationType The orchestration type of the compute environment.
* @property eksProperties An object with various properties that are specific to Amazon EKS based jobs. This must not be specified for Amazon ECS based job definitions.
* @property id The ARN
* @property name The name of the volume.
* @property nodeProperties An object with various properties specific to multi-node parallel jobs. If you specify node properties for a job, it becomes a multi-node parallel job. For more information, see Multi-node Parallel Jobs in the AWS Batch User Guide. If the job definition's type parameter is container, then you must specify either containerProperties or nodeProperties.
* @property retryStrategies The retry strategy to use for failed jobs that are submitted with this job definition. Any retry strategy that's specified during a SubmitJob operation overrides the retry strategy defined here. If a job is terminated due to a timeout, it isn't retried.
* @property revision
* @property schedulingPriority The scheduling priority for jobs that are submitted with this job definition. This only affects jobs in job queues with a fair share policy. Jobs with a higher scheduling priority are scheduled before jobs with a lower scheduling priority.
* @property status
* @property tags
* @property timeouts The timeout configuration for jobs that are submitted with this job definition, after which AWS Batch terminates your jobs if they have not finished. If a job is terminated due to a timeout, it isn't retried. The minimum value for the timeout is 60 seconds.
* @property type The type of resource to assign to a container. The supported resources include `GPU`, `MEMORY`, and `VCPU`.
*/
public data class GetJobDefinitionResult(
public val arn: String? = null,
public val arnPrefix: String,
public val containerOrchestrationType: String,
public val eksProperties: List,
public val id: String,
public val name: String? = null,
public val nodeProperties: List,
public val retryStrategies: List,
public val revision: Int? = null,
public val schedulingPriority: Int,
public val status: String? = null,
public val tags: Map,
public val timeouts: List,
public val type: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.batch.outputs.GetJobDefinitionResult): GetJobDefinitionResult = GetJobDefinitionResult(
arn = javaType.arn().map({ args0 -> args0 }).orElse(null),
arnPrefix = javaType.arnPrefix(),
containerOrchestrationType = javaType.containerOrchestrationType(),
eksProperties = javaType.eksProperties().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionEksProperty.Companion.toKotlin(args0)
})
}),
id = javaType.id(),
name = javaType.name().map({ args0 -> args0 }).orElse(null),
nodeProperties = javaType.nodeProperties().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionNodeProperty.Companion.toKotlin(args0)
})
}),
retryStrategies = javaType.retryStrategies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionRetryStrategy.Companion.toKotlin(args0)
})
}),
revision = javaType.revision().map({ args0 -> args0 }).orElse(null),
schedulingPriority = javaType.schedulingPriority(),
status = javaType.status().map({ args0 -> args0 }).orElse(null),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
timeouts = javaType.timeouts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.batch.kotlin.outputs.GetJobDefinitionTimeout.Companion.toKotlin(args0)
})
}),
type = javaType.type(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy