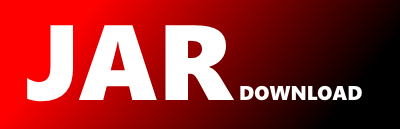
com.pulumi.aws.bedrock.kotlin.inputs.AgentKnowledgeBaseStorageConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.bedrock.kotlin.inputs
import com.pulumi.aws.bedrock.inputs.AgentKnowledgeBaseStorageConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property opensearchServerlessConfiguration The storage configuration of the knowledge base in Amazon OpenSearch Service. See `opensearch_serverless_configuration` block for details.
* @property pineconeConfiguration The storage configuration of the knowledge base in Pinecone. See `pinecone_configuration` block for details.
* @property rdsConfiguration Details about the storage configuration of the knowledge base in Amazon RDS. For more information, see [Create a vector index in Amazon RDS](https://docs.aws.amazon.com/bedrock/latest/userguide/knowledge-base-setup.html). See `rds_configuration` block for details.
* @property redisEnterpriseCloudConfiguration The storage configuration of the knowledge base in Redis Enterprise Cloud. See `redis_enterprise_cloud_configuration` block for details.
* @property type Vector store service in which the knowledge base is stored. Valid Values: `OPENSEARCH_SERVERLESS`, `PINECONE`, `REDIS_ENTERPRISE_CLOUD`, `RDS`.
*/
public data class AgentKnowledgeBaseStorageConfigurationArgs(
public val opensearchServerlessConfiguration: Output? = null,
public val pineconeConfiguration: Output? = null,
public val rdsConfiguration: Output? =
null,
public val redisEnterpriseCloudConfiguration: Output? = null,
public val type: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.bedrock.inputs.AgentKnowledgeBaseStorageConfigurationArgs =
com.pulumi.aws.bedrock.inputs.AgentKnowledgeBaseStorageConfigurationArgs.builder()
.opensearchServerlessConfiguration(
opensearchServerlessConfiguration?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.pineconeConfiguration(
pineconeConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.rdsConfiguration(rdsConfiguration?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.redisEnterpriseCloudConfiguration(
redisEnterpriseCloudConfiguration?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.type(type.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AgentKnowledgeBaseStorageConfigurationArgs].
*/
@PulumiTagMarker
public class AgentKnowledgeBaseStorageConfigurationArgsBuilder internal constructor() {
private var opensearchServerlessConfiguration:
Output? = null
private var pineconeConfiguration:
Output? = null
private var rdsConfiguration: Output? =
null
private var redisEnterpriseCloudConfiguration:
Output? = null
private var type: Output? = null
/**
* @param value The storage configuration of the knowledge base in Amazon OpenSearch Service. See `opensearch_serverless_configuration` block for details.
*/
@JvmName("ckncerpmpjtcctge")
public suspend fun opensearchServerlessConfiguration(`value`: Output) {
this.opensearchServerlessConfiguration = value
}
/**
* @param value The storage configuration of the knowledge base in Pinecone. See `pinecone_configuration` block for details.
*/
@JvmName("fueqkgelykwqapge")
public suspend fun pineconeConfiguration(`value`: Output) {
this.pineconeConfiguration = value
}
/**
* @param value Details about the storage configuration of the knowledge base in Amazon RDS. For more information, see [Create a vector index in Amazon RDS](https://docs.aws.amazon.com/bedrock/latest/userguide/knowledge-base-setup.html). See `rds_configuration` block for details.
*/
@JvmName("dqiviofhjwlakvqd")
public suspend fun rdsConfiguration(`value`: Output) {
this.rdsConfiguration = value
}
/**
* @param value The storage configuration of the knowledge base in Redis Enterprise Cloud. See `redis_enterprise_cloud_configuration` block for details.
*/
@JvmName("erqvrrfyxygfkjlk")
public suspend fun redisEnterpriseCloudConfiguration(`value`: Output) {
this.redisEnterpriseCloudConfiguration = value
}
/**
* @param value Vector store service in which the knowledge base is stored. Valid Values: `OPENSEARCH_SERVERLESS`, `PINECONE`, `REDIS_ENTERPRISE_CLOUD`, `RDS`.
*/
@JvmName("ymkcddhbwpbcmlmn")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value The storage configuration of the knowledge base in Amazon OpenSearch Service. See `opensearch_serverless_configuration` block for details.
*/
@JvmName("cmmupwcshcgladxf")
public suspend fun opensearchServerlessConfiguration(`value`: AgentKnowledgeBaseStorageConfigurationOpensearchServerlessConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.opensearchServerlessConfiguration = mapped
}
/**
* @param argument The storage configuration of the knowledge base in Amazon OpenSearch Service. See `opensearch_serverless_configuration` block for details.
*/
@JvmName("saxcpqceuddsfijt")
public suspend fun opensearchServerlessConfiguration(argument: suspend AgentKnowledgeBaseStorageConfigurationOpensearchServerlessConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
AgentKnowledgeBaseStorageConfigurationOpensearchServerlessConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.opensearchServerlessConfiguration = mapped
}
/**
* @param value The storage configuration of the knowledge base in Pinecone. See `pinecone_configuration` block for details.
*/
@JvmName("keteiqqxwgggtsqj")
public suspend fun pineconeConfiguration(`value`: AgentKnowledgeBaseStorageConfigurationPineconeConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pineconeConfiguration = mapped
}
/**
* @param argument The storage configuration of the knowledge base in Pinecone. See `pinecone_configuration` block for details.
*/
@JvmName("moiiikasidefvwka")
public suspend fun pineconeConfiguration(argument: suspend AgentKnowledgeBaseStorageConfigurationPineconeConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
AgentKnowledgeBaseStorageConfigurationPineconeConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.pineconeConfiguration = mapped
}
/**
* @param value Details about the storage configuration of the knowledge base in Amazon RDS. For more information, see [Create a vector index in Amazon RDS](https://docs.aws.amazon.com/bedrock/latest/userguide/knowledge-base-setup.html). See `rds_configuration` block for details.
*/
@JvmName("enwvyikwoveumsat")
public suspend fun rdsConfiguration(`value`: AgentKnowledgeBaseStorageConfigurationRdsConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rdsConfiguration = mapped
}
/**
* @param argument Details about the storage configuration of the knowledge base in Amazon RDS. For more information, see [Create a vector index in Amazon RDS](https://docs.aws.amazon.com/bedrock/latest/userguide/knowledge-base-setup.html). See `rds_configuration` block for details.
*/
@JvmName("wjcpbycjedrevjcf")
public suspend fun rdsConfiguration(argument: suspend AgentKnowledgeBaseStorageConfigurationRdsConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
AgentKnowledgeBaseStorageConfigurationRdsConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.rdsConfiguration = mapped
}
/**
* @param value The storage configuration of the knowledge base in Redis Enterprise Cloud. See `redis_enterprise_cloud_configuration` block for details.
*/
@JvmName("fstiolarovuiiyyg")
public suspend fun redisEnterpriseCloudConfiguration(`value`: AgentKnowledgeBaseStorageConfigurationRedisEnterpriseCloudConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.redisEnterpriseCloudConfiguration = mapped
}
/**
* @param argument The storage configuration of the knowledge base in Redis Enterprise Cloud. See `redis_enterprise_cloud_configuration` block for details.
*/
@JvmName("ahsjhxlukqgcmwev")
public suspend fun redisEnterpriseCloudConfiguration(argument: suspend AgentKnowledgeBaseStorageConfigurationRedisEnterpriseCloudConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
AgentKnowledgeBaseStorageConfigurationRedisEnterpriseCloudConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.redisEnterpriseCloudConfiguration = mapped
}
/**
* @param value Vector store service in which the knowledge base is stored. Valid Values: `OPENSEARCH_SERVERLESS`, `PINECONE`, `REDIS_ENTERPRISE_CLOUD`, `RDS`.
*/
@JvmName("loaivoysbldserux")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): AgentKnowledgeBaseStorageConfigurationArgs =
AgentKnowledgeBaseStorageConfigurationArgs(
opensearchServerlessConfiguration = opensearchServerlessConfiguration,
pineconeConfiguration = pineconeConfiguration,
rdsConfiguration = rdsConfiguration,
redisEnterpriseCloudConfiguration = redisEnterpriseCloudConfiguration,
type = type ?: throw PulumiNullFieldException("type"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy