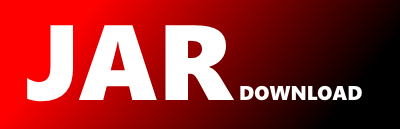
com.pulumi.aws.cfg.kotlin.RemediationConfiguration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cfg.kotlin
import com.pulumi.aws.cfg.kotlin.outputs.RemediationConfigurationExecutionControls
import com.pulumi.aws.cfg.kotlin.outputs.RemediationConfigurationParameter
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.aws.cfg.kotlin.outputs.RemediationConfigurationExecutionControls.Companion.toKotlin as remediationConfigurationExecutionControlsToKotlin
import com.pulumi.aws.cfg.kotlin.outputs.RemediationConfigurationParameter.Companion.toKotlin as remediationConfigurationParameterToKotlin
/**
* Builder for [RemediationConfiguration].
*/
@PulumiTagMarker
public class RemediationConfigurationResourceBuilder internal constructor() {
public var name: String? = null
public var args: RemediationConfigurationArgs = RemediationConfigurationArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend RemediationConfigurationArgsBuilder.() -> Unit) {
val builder = RemediationConfigurationArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): RemediationConfiguration {
val builtJavaResource = com.pulumi.aws.cfg.RemediationConfiguration(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return RemediationConfiguration(builtJavaResource)
}
}
/**
* Provides an AWS Config Remediation Configuration.
* > **Note:** Config Remediation Configuration requires an existing Config Rule to be present.
* ## Example Usage
* AWS managed rules can be used by setting the source owner to `AWS` and the source identifier to the name of the managed rule. More information about AWS managed rules can be found in the [AWS Config Developer Guide](https://docs.aws.amazon.com/config/latest/developerguide/evaluate-config_use-managed-rules.html).
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const _this = new aws.cfg.Rule("this", {
* name: "example",
* source: {
* owner: "AWS",
* sourceIdentifier: "S3_BUCKET_VERSIONING_ENABLED",
* },
* });
* const thisRemediationConfiguration = new aws.cfg.RemediationConfiguration("this", {
* configRuleName: _this.name,
* resourceType: "AWS::S3::Bucket",
* targetType: "SSM_DOCUMENT",
* targetId: "AWS-EnableS3BucketEncryption",
* targetVersion: "1",
* parameters: [
* {
* name: "AutomationAssumeRole",
* staticValue: "arn:aws:iam::875924563244:role/security_config",
* },
* {
* name: "BucketName",
* resourceValue: "RESOURCE_ID",
* },
* {
* name: "SSEAlgorithm",
* staticValue: "AES256",
* },
* ],
* automatic: true,
* maximumAutomaticAttempts: 10,
* retryAttemptSeconds: 600,
* executionControls: {
* ssmControls: {
* concurrentExecutionRatePercentage: 25,
* errorPercentage: 20,
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* this = aws.cfg.Rule("this",
* name="example",
* source={
* "owner": "AWS",
* "source_identifier": "S3_BUCKET_VERSIONING_ENABLED",
* })
* this_remediation_configuration = aws.cfg.RemediationConfiguration("this",
* config_rule_name=this.name,
* resource_type="AWS::S3::Bucket",
* target_type="SSM_DOCUMENT",
* target_id="AWS-EnableS3BucketEncryption",
* target_version="1",
* parameters=[
* {
* "name": "AutomationAssumeRole",
* "static_value": "arn:aws:iam::875924563244:role/security_config",
* },
* {
* "name": "BucketName",
* "resource_value": "RESOURCE_ID",
* },
* {
* "name": "SSEAlgorithm",
* "static_value": "AES256",
* },
* ],
* automatic=True,
* maximum_automatic_attempts=10,
* retry_attempt_seconds=600,
* execution_controls={
* "ssm_controls": {
* "concurrent_execution_rate_percentage": 25,
* "error_percentage": 20,
* },
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var @this = new Aws.Cfg.Rule("this", new()
* {
* Name = "example",
* Source = new Aws.Cfg.Inputs.RuleSourceArgs
* {
* Owner = "AWS",
* SourceIdentifier = "S3_BUCKET_VERSIONING_ENABLED",
* },
* });
* var thisRemediationConfiguration = new Aws.Cfg.RemediationConfiguration("this", new()
* {
* ConfigRuleName = @this.Name,
* ResourceType = "AWS::S3::Bucket",
* TargetType = "SSM_DOCUMENT",
* TargetId = "AWS-EnableS3BucketEncryption",
* TargetVersion = "1",
* Parameters = new[]
* {
* new Aws.Cfg.Inputs.RemediationConfigurationParameterArgs
* {
* Name = "AutomationAssumeRole",
* StaticValue = "arn:aws:iam::875924563244:role/security_config",
* },
* new Aws.Cfg.Inputs.RemediationConfigurationParameterArgs
* {
* Name = "BucketName",
* ResourceValue = "RESOURCE_ID",
* },
* new Aws.Cfg.Inputs.RemediationConfigurationParameterArgs
* {
* Name = "SSEAlgorithm",
* StaticValue = "AES256",
* },
* },
* Automatic = true,
* MaximumAutomaticAttempts = 10,
* RetryAttemptSeconds = 600,
* ExecutionControls = new Aws.Cfg.Inputs.RemediationConfigurationExecutionControlsArgs
* {
* SsmControls = new Aws.Cfg.Inputs.RemediationConfigurationExecutionControlsSsmControlsArgs
* {
* ConcurrentExecutionRatePercentage = 25,
* ErrorPercentage = 20,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cfg"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* this, err := cfg.NewRule(ctx, "this", &cfg.RuleArgs{
* Name: pulumi.String("example"),
* Source: &cfg.RuleSourceArgs{
* Owner: pulumi.String("AWS"),
* SourceIdentifier: pulumi.String("S3_BUCKET_VERSIONING_ENABLED"),
* },
* })
* if err != nil {
* return err
* }
* _, err = cfg.NewRemediationConfiguration(ctx, "this", &cfg.RemediationConfigurationArgs{
* ConfigRuleName: this.Name,
* ResourceType: pulumi.String("AWS::S3::Bucket"),
* TargetType: pulumi.String("SSM_DOCUMENT"),
* TargetId: pulumi.String("AWS-EnableS3BucketEncryption"),
* TargetVersion: pulumi.String("1"),
* Parameters: cfg.RemediationConfigurationParameterArray{
* &cfg.RemediationConfigurationParameterArgs{
* Name: pulumi.String("AutomationAssumeRole"),
* StaticValue: pulumi.String("arn:aws:iam::875924563244:role/security_config"),
* },
* &cfg.RemediationConfigurationParameterArgs{
* Name: pulumi.String("BucketName"),
* ResourceValue: pulumi.String("RESOURCE_ID"),
* },
* &cfg.RemediationConfigurationParameterArgs{
* Name: pulumi.String("SSEAlgorithm"),
* StaticValue: pulumi.String("AES256"),
* },
* },
* Automatic: pulumi.Bool(true),
* MaximumAutomaticAttempts: pulumi.Int(10),
* RetryAttemptSeconds: pulumi.Int(600),
* ExecutionControls: &cfg.RemediationConfigurationExecutionControlsArgs{
* SsmControls: &cfg.RemediationConfigurationExecutionControlsSsmControlsArgs{
* ConcurrentExecutionRatePercentage: pulumi.Int(25),
* ErrorPercentage: pulumi.Int(20),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cfg.Rule;
* import com.pulumi.aws.cfg.RuleArgs;
* import com.pulumi.aws.cfg.inputs.RuleSourceArgs;
* import com.pulumi.aws.cfg.RemediationConfiguration;
* import com.pulumi.aws.cfg.RemediationConfigurationArgs;
* import com.pulumi.aws.cfg.inputs.RemediationConfigurationParameterArgs;
* import com.pulumi.aws.cfg.inputs.RemediationConfigurationExecutionControlsArgs;
* import com.pulumi.aws.cfg.inputs.RemediationConfigurationExecutionControlsSsmControlsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var this_ = new Rule("this", RuleArgs.builder()
* .name("example")
* .source(RuleSourceArgs.builder()
* .owner("AWS")
* .sourceIdentifier("S3_BUCKET_VERSIONING_ENABLED")
* .build())
* .build());
* var thisRemediationConfiguration = new RemediationConfiguration("thisRemediationConfiguration", RemediationConfigurationArgs.builder()
* .configRuleName(this_.name())
* .resourceType("AWS::S3::Bucket")
* .targetType("SSM_DOCUMENT")
* .targetId("AWS-EnableS3BucketEncryption")
* .targetVersion("1")
* .parameters(
* RemediationConfigurationParameterArgs.builder()
* .name("AutomationAssumeRole")
* .staticValue("arn:aws:iam::875924563244:role/security_config")
* .build(),
* RemediationConfigurationParameterArgs.builder()
* .name("BucketName")
* .resourceValue("RESOURCE_ID")
* .build(),
* RemediationConfigurationParameterArgs.builder()
* .name("SSEAlgorithm")
* .staticValue("AES256")
* .build())
* .automatic(true)
* .maximumAutomaticAttempts(10)
* .retryAttemptSeconds(600)
* .executionControls(RemediationConfigurationExecutionControlsArgs.builder()
* .ssmControls(RemediationConfigurationExecutionControlsSsmControlsArgs.builder()
* .concurrentExecutionRatePercentage(25)
* .errorPercentage(20)
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* this:
* type: aws:cfg:Rule
* properties:
* name: example
* source:
* owner: AWS
* sourceIdentifier: S3_BUCKET_VERSIONING_ENABLED
* thisRemediationConfiguration:
* type: aws:cfg:RemediationConfiguration
* name: this
* properties:
* configRuleName: ${this.name}
* resourceType: AWS::S3::Bucket
* targetType: SSM_DOCUMENT
* targetId: AWS-EnableS3BucketEncryption
* targetVersion: '1'
* parameters:
* - name: AutomationAssumeRole
* staticValue: arn:aws:iam::875924563244:role/security_config
* - name: BucketName
* resourceValue: RESOURCE_ID
* - name: SSEAlgorithm
* staticValue: AES256
* automatic: true
* maximumAutomaticAttempts: 10
* retryAttemptSeconds: 600
* executionControls:
* ssmControls:
* concurrentExecutionRatePercentage: 25
* errorPercentage: 20
* ```
*
* ## Import
* Using `pulumi import`, import Remediation Configurations using the name config_rule_name. For example:
* ```sh
* $ pulumi import aws:cfg/remediationConfiguration:RemediationConfiguration this example
* ```
*/
public class RemediationConfiguration internal constructor(
override val javaResource: com.pulumi.aws.cfg.RemediationConfiguration,
) : KotlinCustomResource(javaResource, RemediationConfigurationMapper) {
/**
* ARN of the Config Remediation Configuration.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Remediation is triggered automatically if `true`.
*/
public val automatic: Output?
get() = javaResource.automatic().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Name of the AWS Config rule.
*/
public val configRuleName: Output
get() = javaResource.configRuleName().applyValue({ args0 -> args0 })
/**
* Configuration block for execution controls. See below.
*/
public val executionControls: Output?
get() = javaResource.executionControls().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
remediationConfigurationExecutionControlsToKotlin(args0)
})
}).orElse(null)
})
/**
* Maximum number of failed attempts for auto-remediation. If you do not select a number, the default is 5.
*/
public val maximumAutomaticAttempts: Output?
get() = javaResource.maximumAutomaticAttempts().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Can be specified multiple times for each parameter. Each parameter block supports arguments below.
*/
public val parameters: Output>?
get() = javaResource.parameters().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> remediationConfigurationParameterToKotlin(args0) })
})
}).orElse(null)
})
/**
* Type of resource.
*/
public val resourceType: Output?
get() = javaResource.resourceType().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Maximum time in seconds that AWS Config runs auto-remediation. If you do not select a number, the default is 60 seconds.
*/
public val retryAttemptSeconds: Output?
get() = javaResource.retryAttemptSeconds().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Target ID is the name of the public document.
*/
public val targetId: Output
get() = javaResource.targetId().applyValue({ args0 -> args0 })
/**
* Type of the target. Target executes remediation. For example, SSM document.
* The following arguments are optional:
*/
public val targetType: Output
get() = javaResource.targetType().applyValue({ args0 -> args0 })
/**
* Version of the target. For example, version of the SSM document
*/
public val targetVersion: Output?
get() = javaResource.targetVersion().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
}
public object RemediationConfigurationMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.aws.cfg.RemediationConfiguration::class == javaResource::class
override fun map(javaResource: Resource): RemediationConfiguration =
RemediationConfiguration(javaResource as com.pulumi.aws.cfg.RemediationConfiguration)
}
/**
* @see [RemediationConfiguration].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [RemediationConfiguration].
*/
public suspend fun remediationConfiguration(
name: String,
block: suspend RemediationConfigurationResourceBuilder.() -> Unit,
): RemediationConfiguration {
val builder = RemediationConfigurationResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [RemediationConfiguration].
* @param name The _unique_ name of the resulting resource.
*/
public fun remediationConfiguration(name: String): RemediationConfiguration {
val builder = RemediationConfigurationResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy