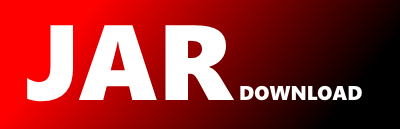
com.pulumi.aws.cleanrooms.kotlin.ConfiguredTableArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cleanrooms.kotlin
import com.pulumi.aws.cleanrooms.ConfiguredTableArgs.builder
import com.pulumi.aws.cleanrooms.kotlin.inputs.ConfiguredTableTableReferenceArgs
import com.pulumi.aws.cleanrooms.kotlin.inputs.ConfiguredTableTableReferenceArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Provides a AWS Clean Rooms configured table. Configured tables are used to represent references to existing tables in the AWS Glue Data Catalog.
* ## Example Usage
* ### Configured table with tags
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const testConfiguredTable = new aws.cleanrooms.ConfiguredTable("test_configured_table", {
* name: "pulumi-example-table",
* description: "I made this table with Pulumi!",
* analysisMethod: "DIRECT_QUERY",
* allowedColumns: [
* "column1",
* "column2",
* "column3",
* ],
* tableReference: {
* databaseName: "example_database",
* tableName: "example_table",
* },
* tags: {
* Project: "Pulumi",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* test_configured_table = aws.cleanrooms.ConfiguredTable("test_configured_table",
* name="pulumi-example-table",
* description="I made this table with Pulumi!",
* analysis_method="DIRECT_QUERY",
* allowed_columns=[
* "column1",
* "column2",
* "column3",
* ],
* table_reference={
* "database_name": "example_database",
* "table_name": "example_table",
* },
* tags={
* "Project": "Pulumi",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var testConfiguredTable = new Aws.CleanRooms.ConfiguredTable("test_configured_table", new()
* {
* Name = "pulumi-example-table",
* Description = "I made this table with Pulumi!",
* AnalysisMethod = "DIRECT_QUERY",
* AllowedColumns = new[]
* {
* "column1",
* "column2",
* "column3",
* },
* TableReference = new Aws.CleanRooms.Inputs.ConfiguredTableTableReferenceArgs
* {
* DatabaseName = "example_database",
* TableName = "example_table",
* },
* Tags =
* {
* { "Project", "Pulumi" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cleanrooms"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cleanrooms.NewConfiguredTable(ctx, "test_configured_table", &cleanrooms.ConfiguredTableArgs{
* Name: pulumi.String("pulumi-example-table"),
* Description: pulumi.String("I made this table with Pulumi!"),
* AnalysisMethod: pulumi.String("DIRECT_QUERY"),
* AllowedColumns: pulumi.StringArray{
* pulumi.String("column1"),
* pulumi.String("column2"),
* pulumi.String("column3"),
* },
* TableReference: &cleanrooms.ConfiguredTableTableReferenceArgs{
* DatabaseName: pulumi.String("example_database"),
* TableName: pulumi.String("example_table"),
* },
* Tags: pulumi.StringMap{
* "Project": pulumi.String("Pulumi"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cleanrooms.ConfiguredTable;
* import com.pulumi.aws.cleanrooms.ConfiguredTableArgs;
* import com.pulumi.aws.cleanrooms.inputs.ConfiguredTableTableReferenceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var testConfiguredTable = new ConfiguredTable("testConfiguredTable", ConfiguredTableArgs.builder()
* .name("pulumi-example-table")
* .description("I made this table with Pulumi!")
* .analysisMethod("DIRECT_QUERY")
* .allowedColumns(
* "column1",
* "column2",
* "column3")
* .tableReference(ConfiguredTableTableReferenceArgs.builder()
* .databaseName("example_database")
* .tableName("example_table")
* .build())
* .tags(Map.of("Project", "Pulumi"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* testConfiguredTable:
* type: aws:cleanrooms:ConfiguredTable
* name: test_configured_table
* properties:
* name: pulumi-example-table
* description: I made this table with Pulumi!
* analysisMethod: DIRECT_QUERY
* allowedColumns:
* - column1
* - column2
* - column3
* tableReference:
* databaseName: example_database
* tableName: example_table
* tags:
* Project: Pulumi
* ```
*
* ## Import
* Using `pulumi import`, import `aws_cleanrooms_configured_table` using the `id`. For example:
* ```sh
* $ pulumi import aws:cleanrooms/configuredTable:ConfiguredTable table 1234abcd-12ab-34cd-56ef-1234567890ab
* ```
* @property allowedColumns The columns of the references table which will be included in the configured table.
* @property analysisMethod The analysis method for the configured table. The only valid value is currently `DIRECT_QUERY`.
* @property description A description for the configured table.
* @property name The name of the configured table.
* @property tableReference A reference to the AWS Glue table which will be used to create the configured table.
* * `table_reference.database_name` - (Required - Forces new resource) - The name of the AWS Glue database which contains the table.
* * `table_reference.table_name` - (Required - Forces new resource) - The name of the AWS Glue table which will be used to create the configured table.
* @property tags Key value pairs which tag the configured table.
*/
public data class ConfiguredTableArgs(
public val allowedColumns: Output>? = null,
public val analysisMethod: Output? = null,
public val description: Output? = null,
public val name: Output? = null,
public val tableReference: Output? = null,
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy