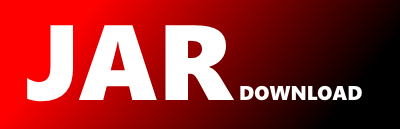
com.pulumi.aws.cloudcontrol.kotlin.Resource.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cloudcontrol.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [Resource].
*/
@PulumiTagMarker
public class ResourceResourceBuilder internal constructor() {
public var name: String? = null
public var args: ResourceArgs = ResourceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ResourceArgsBuilder.() -> Unit) {
val builder = ResourceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Resource {
val builtJavaResource = com.pulumi.aws.cloudcontrol.Resource(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Resource(builtJavaResource)
}
}
/**
* Manages a Cloud Control API Resource. The configuration and lifecycle handling of these resources is proxied through Cloud Control API handlers to the backend service.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.cloudcontrol.Resource("example", {
* typeName: "AWS::ECS::Cluster",
* desiredState: JSON.stringify({
* ClusterName: "example",
* Tags: [{
* Key: "CostCenter",
* Value: "IT",
* }],
* }),
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_aws as aws
* example = aws.cloudcontrol.Resource("example",
* type_name="AWS::ECS::Cluster",
* desired_state=json.dumps({
* "ClusterName": "example",
* "Tags": [{
* "Key": "CostCenter",
* "Value": "IT",
* }],
* }))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.CloudControl.Resource("example", new()
* {
* TypeName = "AWS::ECS::Cluster",
* DesiredState = JsonSerializer.Serialize(new Dictionary
* {
* ["ClusterName"] = "example",
* ["Tags"] = new[]
* {
* new Dictionary
* {
* ["Key"] = "CostCenter",
* ["Value"] = "IT",
* },
* },
* }),
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cloudcontrol"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "ClusterName": "example",
* "Tags": []map[string]interface{}{
* map[string]interface{}{
* "Key": "CostCenter",
* "Value": "IT",
* },
* },
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* _, err = cloudcontrol.NewResource(ctx, "example", &cloudcontrol.ResourceArgs{
* TypeName: pulumi.String("AWS::ECS::Cluster"),
* DesiredState: pulumi.String(json0),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudcontrol.Resource;
* import com.pulumi.aws.cloudcontrol.ResourceArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Resource("example", ResourceArgs.builder()
* .typeName("AWS::ECS::Cluster")
* .desiredState(serializeJson(
* jsonObject(
* jsonProperty("ClusterName", "example"),
* jsonProperty("Tags", jsonArray(jsonObject(
* jsonProperty("Key", "CostCenter"),
* jsonProperty("Value", "IT")
* )))
* )))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:cloudcontrol:Resource
* properties:
* typeName: AWS::ECS::Cluster
* desiredState:
* fn::toJSON:
* ClusterName: example
* Tags:
* - Key: CostCenter
* Value: IT
* ```
*
*/
public class Resource internal constructor(
override val javaResource: com.pulumi.aws.cloudcontrol.Resource,
) : KotlinCustomResource(javaResource, ResourceMapper) {
/**
* JSON string matching the CloudFormation resource type schema with desired configuration.
*/
public val desiredState: Output
get() = javaResource.desiredState().applyValue({ args0 -> args0 })
/**
* JSON string matching the CloudFormation resource type schema with current configuration. Underlying attributes can be referenced via the `jsondecode()` function, for example, `jsondecode(data.aws_cloudcontrolapi_resource.example.properties)["example"]`.
*/
public val properties: Output
get() = javaResource.properties().applyValue({ args0 -> args0 })
/**
* Amazon Resource Name (ARN) of the IAM Role to assume for operations.
*/
public val roleArn: Output?
get() = javaResource.roleArn().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* JSON string of the CloudFormation resource type schema which is used for plan time validation where possible. Automatically fetched if not provided. In large scale environments with multiple resources using the same `type_name`, it is recommended to fetch the schema once via the `aws.cloudformation.CloudFormationType` data source and use this argument to reduce `DescribeType` API operation throttling. This value is marked sensitive only to prevent large plan differences from showing.
*/
public val schema: Output
get() = javaResource.schema().applyValue({ args0 -> args0 })
/**
* CloudFormation resource type name. For example, `AWS::EC2::VPC`.
* The following arguments are optional:
*/
public val typeName: Output
get() = javaResource.typeName().applyValue({ args0 -> args0 })
/**
* Identifier of the CloudFormation resource type version.
*/
public val typeVersionId: Output?
get() = javaResource.typeVersionId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
}
public object ResourceMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: com.pulumi.resources.Resource): Boolean =
com.pulumi.aws.cloudcontrol.Resource::class == javaResource::class
override fun map(javaResource: com.pulumi.resources.Resource): Resource = Resource(
javaResource as
com.pulumi.aws.cloudcontrol.Resource,
)
}
/**
* @see [Resource].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Resource].
*/
public suspend fun resource(name: String, block: suspend ResourceResourceBuilder.() -> Unit): Resource {
val builder = ResourceResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Resource].
* @param name The _unique_ name of the resulting resource.
*/
public fun resource(name: String): Resource {
val builder = ResourceResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy