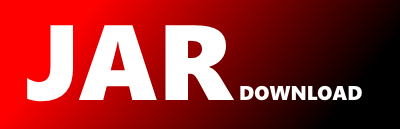
com.pulumi.aws.cloudformation.kotlin.inputs.StackInstancesOperationPreferencesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cloudformation.kotlin.inputs
import com.pulumi.aws.cloudformation.inputs.StackInstancesOperationPreferencesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property concurrencyMode How the concurrency level behaves during the operation execution. Valid values are `STRICT_FAILURE_TOLERANCE` and `SOFT_FAILURE_TOLERANCE`.
* @property failureToleranceCount Number of accounts, per region, for which this operation can fail before CloudFormation stops the operation in that region.
* @property failureTolerancePercentage Percentage of accounts, per region, for which this stack operation can fail before CloudFormation stops the operation in that region.
* @property maxConcurrentCount Maximum number of accounts in which to perform this operation at one time.
* @property maxConcurrentPercentage Maximum percentage of accounts in which to perform this operation at one time.
* @property regionConcurrencyType Concurrency type of deploying stack sets operations in regions, could be in parallel or one region at a time. Valid values are `SEQUENTIAL` and `PARALLEL`.
* @property regionOrders Order of the regions where you want to perform the stack operation.
*/
public data class StackInstancesOperationPreferencesArgs(
public val concurrencyMode: Output? = null,
public val failureToleranceCount: Output? = null,
public val failureTolerancePercentage: Output? = null,
public val maxConcurrentCount: Output? = null,
public val maxConcurrentPercentage: Output? = null,
public val regionConcurrencyType: Output? = null,
public val regionOrders: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.cloudformation.inputs.StackInstancesOperationPreferencesArgs = com.pulumi.aws.cloudformation.inputs.StackInstancesOperationPreferencesArgs.builder()
.concurrencyMode(concurrencyMode?.applyValue({ args0 -> args0 }))
.failureToleranceCount(failureToleranceCount?.applyValue({ args0 -> args0 }))
.failureTolerancePercentage(failureTolerancePercentage?.applyValue({ args0 -> args0 }))
.maxConcurrentCount(maxConcurrentCount?.applyValue({ args0 -> args0 }))
.maxConcurrentPercentage(maxConcurrentPercentage?.applyValue({ args0 -> args0 }))
.regionConcurrencyType(regionConcurrencyType?.applyValue({ args0 -> args0 }))
.regionOrders(regionOrders?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [StackInstancesOperationPreferencesArgs].
*/
@PulumiTagMarker
public class StackInstancesOperationPreferencesArgsBuilder internal constructor() {
private var concurrencyMode: Output? = null
private var failureToleranceCount: Output? = null
private var failureTolerancePercentage: Output? = null
private var maxConcurrentCount: Output? = null
private var maxConcurrentPercentage: Output? = null
private var regionConcurrencyType: Output? = null
private var regionOrders: Output>? = null
/**
* @param value How the concurrency level behaves during the operation execution. Valid values are `STRICT_FAILURE_TOLERANCE` and `SOFT_FAILURE_TOLERANCE`.
*/
@JvmName("swbjqnhymucuhosa")
public suspend fun concurrencyMode(`value`: Output) {
this.concurrencyMode = value
}
/**
* @param value Number of accounts, per region, for which this operation can fail before CloudFormation stops the operation in that region.
*/
@JvmName("etoideqjfbvisfgq")
public suspend fun failureToleranceCount(`value`: Output) {
this.failureToleranceCount = value
}
/**
* @param value Percentage of accounts, per region, for which this stack operation can fail before CloudFormation stops the operation in that region.
*/
@JvmName("cxyuwlxpcufgpbme")
public suspend fun failureTolerancePercentage(`value`: Output) {
this.failureTolerancePercentage = value
}
/**
* @param value Maximum number of accounts in which to perform this operation at one time.
*/
@JvmName("kdpdexbwyetbbxay")
public suspend fun maxConcurrentCount(`value`: Output) {
this.maxConcurrentCount = value
}
/**
* @param value Maximum percentage of accounts in which to perform this operation at one time.
*/
@JvmName("vmuquuwmlrtivcdq")
public suspend fun maxConcurrentPercentage(`value`: Output) {
this.maxConcurrentPercentage = value
}
/**
* @param value Concurrency type of deploying stack sets operations in regions, could be in parallel or one region at a time. Valid values are `SEQUENTIAL` and `PARALLEL`.
*/
@JvmName("nurjnkrqhavaswjp")
public suspend fun regionConcurrencyType(`value`: Output) {
this.regionConcurrencyType = value
}
/**
* @param value Order of the regions where you want to perform the stack operation.
*/
@JvmName("wmldjcpdyegnrimi")
public suspend fun regionOrders(`value`: Output>) {
this.regionOrders = value
}
@JvmName("pyaxaexhdcjbixld")
public suspend fun regionOrders(vararg values: Output) {
this.regionOrders = Output.all(values.asList())
}
/**
* @param values Order of the regions where you want to perform the stack operation.
*/
@JvmName("yhowmjwwcsjbniym")
public suspend fun regionOrders(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy