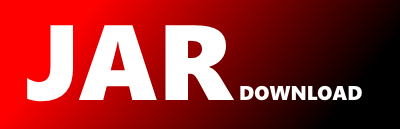
com.pulumi.aws.cloudfront.kotlin.inputs.DistributionViewerCertificateArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cloudfront.kotlin.inputs
import com.pulumi.aws.cloudfront.inputs.DistributionViewerCertificateArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property acmCertificateArn ARN of the [AWS Certificate Manager](https://aws.amazon.com/certificate-manager/) certificate that you wish to use with this distribution. Specify this, `cloudfront_default_certificate`, or `iam_certificate_id`. The ACM certificate must be in US-EAST-1.
* @property cloudfrontDefaultCertificate `true` if you want viewers to use HTTPS to request your objects and you're using the CloudFront domain name for your distribution. Specify this, `acm_certificate_arn`, or `iam_certificate_id`.
* @property iamCertificateId IAM certificate identifier of the custom viewer certificate for this distribution if you are using a custom domain. Specify this, `acm_certificate_arn`, or `cloudfront_default_certificate`.
* @property minimumProtocolVersion Minimum version of the SSL protocol that you want CloudFront to use for HTTPS connections. Can only be set if `cloudfront_default_certificate = false`. See all possible values in [this](https://docs.aws.amazon.com/AmazonCloudFront/latest/DeveloperGuide/secure-connections-supported-viewer-protocols-ciphers.html) table under "Security policy." Some examples include: `TLSv1.2_2019` and `TLSv1.2_2021`. Default: `TLSv1`. **NOTE**: If you are using a custom certificate (specified with `acm_certificate_arn` or `iam_certificate_id`), and have specified `sni-only` in `ssl_support_method`, `TLSv1` or later must be specified. If you have specified `vip` in `ssl_support_method`, only `SSLv3` or `TLSv1` can be specified. If you have specified `cloudfront_default_certificate`, `TLSv1` must be specified.
* @property sslSupportMethod How you want CloudFront to serve HTTPS requests. One of `vip`, `sni-only`, or `static-ip`. Required if you specify `acm_certificate_arn` or `iam_certificate_id`. **NOTE:** `vip` causes CloudFront to use a dedicated IP address and may incur extra charges.
*/
public data class DistributionViewerCertificateArgs(
public val acmCertificateArn: Output? = null,
public val cloudfrontDefaultCertificate: Output? = null,
public val iamCertificateId: Output? = null,
public val minimumProtocolVersion: Output? = null,
public val sslSupportMethod: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.cloudfront.inputs.DistributionViewerCertificateArgs =
com.pulumi.aws.cloudfront.inputs.DistributionViewerCertificateArgs.builder()
.acmCertificateArn(acmCertificateArn?.applyValue({ args0 -> args0 }))
.cloudfrontDefaultCertificate(cloudfrontDefaultCertificate?.applyValue({ args0 -> args0 }))
.iamCertificateId(iamCertificateId?.applyValue({ args0 -> args0 }))
.minimumProtocolVersion(minimumProtocolVersion?.applyValue({ args0 -> args0 }))
.sslSupportMethod(sslSupportMethod?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DistributionViewerCertificateArgs].
*/
@PulumiTagMarker
public class DistributionViewerCertificateArgsBuilder internal constructor() {
private var acmCertificateArn: Output? = null
private var cloudfrontDefaultCertificate: Output? = null
private var iamCertificateId: Output? = null
private var minimumProtocolVersion: Output? = null
private var sslSupportMethod: Output? = null
/**
* @param value ARN of the [AWS Certificate Manager](https://aws.amazon.com/certificate-manager/) certificate that you wish to use with this distribution. Specify this, `cloudfront_default_certificate`, or `iam_certificate_id`. The ACM certificate must be in US-EAST-1.
*/
@JvmName("wpwcmxdmwyosttqw")
public suspend fun acmCertificateArn(`value`: Output) {
this.acmCertificateArn = value
}
/**
* @param value `true` if you want viewers to use HTTPS to request your objects and you're using the CloudFront domain name for your distribution. Specify this, `acm_certificate_arn`, or `iam_certificate_id`.
*/
@JvmName("fifvpvkvervaxskd")
public suspend fun cloudfrontDefaultCertificate(`value`: Output) {
this.cloudfrontDefaultCertificate = value
}
/**
* @param value IAM certificate identifier of the custom viewer certificate for this distribution if you are using a custom domain. Specify this, `acm_certificate_arn`, or `cloudfront_default_certificate`.
*/
@JvmName("hbcnircovlavcbba")
public suspend fun iamCertificateId(`value`: Output) {
this.iamCertificateId = value
}
/**
* @param value Minimum version of the SSL protocol that you want CloudFront to use for HTTPS connections. Can only be set if `cloudfront_default_certificate = false`. See all possible values in [this](https://docs.aws.amazon.com/AmazonCloudFront/latest/DeveloperGuide/secure-connections-supported-viewer-protocols-ciphers.html) table under "Security policy." Some examples include: `TLSv1.2_2019` and `TLSv1.2_2021`. Default: `TLSv1`. **NOTE**: If you are using a custom certificate (specified with `acm_certificate_arn` or `iam_certificate_id`), and have specified `sni-only` in `ssl_support_method`, `TLSv1` or later must be specified. If you have specified `vip` in `ssl_support_method`, only `SSLv3` or `TLSv1` can be specified. If you have specified `cloudfront_default_certificate`, `TLSv1` must be specified.
*/
@JvmName("wkgctogvwkhcabuu")
public suspend fun minimumProtocolVersion(`value`: Output) {
this.minimumProtocolVersion = value
}
/**
* @param value How you want CloudFront to serve HTTPS requests. One of `vip`, `sni-only`, or `static-ip`. Required if you specify `acm_certificate_arn` or `iam_certificate_id`. **NOTE:** `vip` causes CloudFront to use a dedicated IP address and may incur extra charges.
*/
@JvmName("njnuhkeiscwgxxnx")
public suspend fun sslSupportMethod(`value`: Output) {
this.sslSupportMethod = value
}
/**
* @param value ARN of the [AWS Certificate Manager](https://aws.amazon.com/certificate-manager/) certificate that you wish to use with this distribution. Specify this, `cloudfront_default_certificate`, or `iam_certificate_id`. The ACM certificate must be in US-EAST-1.
*/
@JvmName("llqgtsyhleakdrbb")
public suspend fun acmCertificateArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.acmCertificateArn = mapped
}
/**
* @param value `true` if you want viewers to use HTTPS to request your objects and you're using the CloudFront domain name for your distribution. Specify this, `acm_certificate_arn`, or `iam_certificate_id`.
*/
@JvmName("aovlvywsaekeemcy")
public suspend fun cloudfrontDefaultCertificate(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cloudfrontDefaultCertificate = mapped
}
/**
* @param value IAM certificate identifier of the custom viewer certificate for this distribution if you are using a custom domain. Specify this, `acm_certificate_arn`, or `cloudfront_default_certificate`.
*/
@JvmName("dnrcgfrgloifcrpo")
public suspend fun iamCertificateId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.iamCertificateId = mapped
}
/**
* @param value Minimum version of the SSL protocol that you want CloudFront to use for HTTPS connections. Can only be set if `cloudfront_default_certificate = false`. See all possible values in [this](https://docs.aws.amazon.com/AmazonCloudFront/latest/DeveloperGuide/secure-connections-supported-viewer-protocols-ciphers.html) table under "Security policy." Some examples include: `TLSv1.2_2019` and `TLSv1.2_2021`. Default: `TLSv1`. **NOTE**: If you are using a custom certificate (specified with `acm_certificate_arn` or `iam_certificate_id`), and have specified `sni-only` in `ssl_support_method`, `TLSv1` or later must be specified. If you have specified `vip` in `ssl_support_method`, only `SSLv3` or `TLSv1` can be specified. If you have specified `cloudfront_default_certificate`, `TLSv1` must be specified.
*/
@JvmName("uvudliomcucbkvaj")
public suspend fun minimumProtocolVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minimumProtocolVersion = mapped
}
/**
* @param value How you want CloudFront to serve HTTPS requests. One of `vip`, `sni-only`, or `static-ip`. Required if you specify `acm_certificate_arn` or `iam_certificate_id`. **NOTE:** `vip` causes CloudFront to use a dedicated IP address and may incur extra charges.
*/
@JvmName("rrmastashhxhrarp")
public suspend fun sslSupportMethod(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sslSupportMethod = mapped
}
internal fun build(): DistributionViewerCertificateArgs = DistributionViewerCertificateArgs(
acmCertificateArn = acmCertificateArn,
cloudfrontDefaultCertificate = cloudfrontDefaultCertificate,
iamCertificateId = iamCertificateId,
minimumProtocolVersion = minimumProtocolVersion,
sslSupportMethod = sslSupportMethod,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy