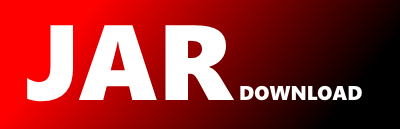
com.pulumi.aws.cloudfront.kotlin.outputs.DistributionDefaultCacheBehavior.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cloudfront.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property allowedMethods Controls which HTTP methods CloudFront processes and forwards to your Amazon S3 bucket or your custom origin.
* @property cachePolicyId Unique identifier of the cache policy that is attached to the cache behavior. If configuring the `default_cache_behavior` either `cache_policy_id` or `forwarded_values` must be set.
* @property cachedMethods Controls whether CloudFront caches the response to requests using the specified HTTP methods.
* @property compress Whether you want CloudFront to automatically compress content for web requests that include `Accept-Encoding: gzip` in the request header (default: `false`).
* @property defaultTtl Default amount of time (in seconds) that an object is in a CloudFront cache before CloudFront forwards another request in the absence of an `Cache-Control max-age` or `Expires` header.
* @property fieldLevelEncryptionId Field level encryption configuration ID.
* @property forwardedValues The forwarded values configuration that specifies how CloudFront handles query strings, cookies and headers (maximum one).
* @property functionAssociations A config block that triggers a cloudfront function with specific actions (maximum 2).
* @property lambdaFunctionAssociations A config block that triggers a lambda function with specific actions (maximum 4).
* @property maxTtl Maximum amount of time (in seconds) that an object is in a CloudFront cache before CloudFront forwards another request to your origin to determine whether the object has been updated. Only effective in the presence of `Cache-Control max-age`, `Cache-Control s-maxage`, and `Expires` headers.
* @property minTtl Minimum amount of time that you want objects to stay in CloudFront caches before CloudFront queries your origin to see whether the object has been updated. Defaults to 0 seconds.
* @property originRequestPolicyId Unique identifier of the origin request policy that is attached to the behavior.
* @property realtimeLogConfigArn ARN of the real-time log configuration that is attached to this cache behavior.
* @property responseHeadersPolicyId Identifier for a response headers policy.
* @property smoothStreaming Indicates whether you want to distribute media files in Microsoft Smooth Streaming format using the origin that is associated with this cache behavior.
* @property targetOriginId Value of ID for the origin that you want CloudFront to route requests to when a request matches the path pattern either for a cache behavior or for the default cache behavior.
* @property trustedKeyGroups List of nested attributes for active trusted key groups, if the distribution is set up to serve private content with signed URLs.
* @property trustedSigners List of nested attributes for active trusted signers, if the distribution is set up to serve private content with signed URLs.
* @property viewerProtocolPolicy Use this element to specify the protocol that users can use to access the files in the origin specified by TargetOriginId when a request matches the path pattern in PathPattern. One of `allow-all`, `https-only`, or `redirect-to-https`.
*/
public data class DistributionDefaultCacheBehavior(
public val allowedMethods: List,
public val cachePolicyId: String? = null,
public val cachedMethods: List,
public val compress: Boolean? = null,
public val defaultTtl: Int? = null,
public val fieldLevelEncryptionId: String? = null,
public val forwardedValues: DistributionDefaultCacheBehaviorForwardedValues? = null,
public val functionAssociations: List? =
null,
public val lambdaFunctionAssociations: List? = null,
public val maxTtl: Int? = null,
public val minTtl: Int? = null,
public val originRequestPolicyId: String? = null,
public val realtimeLogConfigArn: String? = null,
public val responseHeadersPolicyId: String? = null,
public val smoothStreaming: Boolean? = null,
public val targetOriginId: String,
public val trustedKeyGroups: List? = null,
public val trustedSigners: List? = null,
public val viewerProtocolPolicy: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.cloudfront.outputs.DistributionDefaultCacheBehavior): DistributionDefaultCacheBehavior = DistributionDefaultCacheBehavior(
allowedMethods = javaType.allowedMethods().map({ args0 -> args0 }),
cachePolicyId = javaType.cachePolicyId().map({ args0 -> args0 }).orElse(null),
cachedMethods = javaType.cachedMethods().map({ args0 -> args0 }),
compress = javaType.compress().map({ args0 -> args0 }).orElse(null),
defaultTtl = javaType.defaultTtl().map({ args0 -> args0 }).orElse(null),
fieldLevelEncryptionId = javaType.fieldLevelEncryptionId().map({ args0 -> args0 }).orElse(null),
forwardedValues = javaType.forwardedValues().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.cloudfront.kotlin.outputs.DistributionDefaultCacheBehaviorForwardedValues.Companion.toKotlin(args0)
})
}).orElse(null),
functionAssociations = javaType.functionAssociations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.cloudfront.kotlin.outputs.DistributionDefaultCacheBehaviorFunctionAssociation.Companion.toKotlin(args0)
})
}),
lambdaFunctionAssociations = javaType.lambdaFunctionAssociations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.cloudfront.kotlin.outputs.DistributionDefaultCacheBehaviorLambdaFunctionAssociation.Companion.toKotlin(args0)
})
}),
maxTtl = javaType.maxTtl().map({ args0 -> args0 }).orElse(null),
minTtl = javaType.minTtl().map({ args0 -> args0 }).orElse(null),
originRequestPolicyId = javaType.originRequestPolicyId().map({ args0 -> args0 }).orElse(null),
realtimeLogConfigArn = javaType.realtimeLogConfigArn().map({ args0 -> args0 }).orElse(null),
responseHeadersPolicyId = javaType.responseHeadersPolicyId().map({ args0 -> args0 }).orElse(null),
smoothStreaming = javaType.smoothStreaming().map({ args0 -> args0 }).orElse(null),
targetOriginId = javaType.targetOriginId(),
trustedKeyGroups = javaType.trustedKeyGroups().map({ args0 -> args0 }),
trustedSigners = javaType.trustedSigners().map({ args0 -> args0 }),
viewerProtocolPolicy = javaType.viewerProtocolPolicy(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy