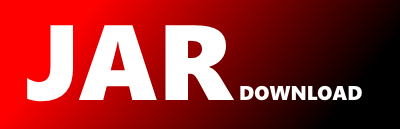
com.pulumi.aws.cloudsearch.kotlin.inputs.DomainIndexFieldArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cloudsearch.kotlin.inputs
import com.pulumi.aws.cloudsearch.inputs.DomainIndexFieldArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property analysisScheme The analysis scheme you want to use for a `text` field. The analysis scheme specifies the language-specific text processing options that are used during indexing.
* @property defaultValue The default value for the field. This value is used when no value is specified for the field in the document data.
* @property facet You can get facet information by enabling this.
* @property highlight You can highlight information.
* @property name A unique name for the field. Field names must begin with a letter and be at least 1 and no more than 64 characters long. The allowed characters are: `a`-`z` (lower-case letters), `0`-`9`, and `_` (underscore). The name `score` is reserved and cannot be used as a field name.
* @property return You can enable returning the value of all searchable fields.
* @property search You can set whether this index should be searchable or not.
* @property sort You can enable the property to be sortable.
* @property sourceFields A comma-separated list of source fields to map to the field. Specifying a source field copies data from one field to another, enabling you to use the same source data in different ways by configuring different options for the fields.
* @property type The field type. Valid values: `date`, `date-array`, `double`, `double-array`, `int`, `int-array`, `literal`, `literal-array`, `text`, `text-array`.
*/
public data class DomainIndexFieldArgs(
public val analysisScheme: Output? = null,
public val defaultValue: Output? = null,
public val facet: Output? = null,
public val highlight: Output? = null,
public val name: Output,
public val `return`: Output? = null,
public val search: Output? = null,
public val sort: Output? = null,
public val sourceFields: Output? = null,
public val type: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.cloudsearch.inputs.DomainIndexFieldArgs =
com.pulumi.aws.cloudsearch.inputs.DomainIndexFieldArgs.builder()
.analysisScheme(analysisScheme?.applyValue({ args0 -> args0 }))
.defaultValue(defaultValue?.applyValue({ args0 -> args0 }))
.facet(facet?.applyValue({ args0 -> args0 }))
.highlight(highlight?.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.return_(`return`?.applyValue({ args0 -> args0 }))
.search(search?.applyValue({ args0 -> args0 }))
.sort(sort?.applyValue({ args0 -> args0 }))
.sourceFields(sourceFields?.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DomainIndexFieldArgs].
*/
@PulumiTagMarker
public class DomainIndexFieldArgsBuilder internal constructor() {
private var analysisScheme: Output? = null
private var defaultValue: Output? = null
private var facet: Output? = null
private var highlight: Output? = null
private var name: Output? = null
private var `return`: Output? = null
private var search: Output? = null
private var sort: Output? = null
private var sourceFields: Output? = null
private var type: Output? = null
/**
* @param value The analysis scheme you want to use for a `text` field. The analysis scheme specifies the language-specific text processing options that are used during indexing.
*/
@JvmName("whftyyewotxinsws")
public suspend fun analysisScheme(`value`: Output) {
this.analysisScheme = value
}
/**
* @param value The default value for the field. This value is used when no value is specified for the field in the document data.
*/
@JvmName("pvjromhwaanlhsta")
public suspend fun defaultValue(`value`: Output) {
this.defaultValue = value
}
/**
* @param value You can get facet information by enabling this.
*/
@JvmName("madeorxxomaiufiu")
public suspend fun facet(`value`: Output) {
this.facet = value
}
/**
* @param value You can highlight information.
*/
@JvmName("fabbvbuuevsjcwxa")
public suspend fun highlight(`value`: Output) {
this.highlight = value
}
/**
* @param value A unique name for the field. Field names must begin with a letter and be at least 1 and no more than 64 characters long. The allowed characters are: `a`-`z` (lower-case letters), `0`-`9`, and `_` (underscore). The name `score` is reserved and cannot be used as a field name.
*/
@JvmName("anyxolbsweicaxjd")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value You can enable returning the value of all searchable fields.
*/
@JvmName("xykclaxdbcpxhhbt")
public suspend fun `return`(`value`: Output) {
this.`return` = value
}
/**
* @param value You can set whether this index should be searchable or not.
*/
@JvmName("decnuulmbraaxhrr")
public suspend fun search(`value`: Output) {
this.search = value
}
/**
* @param value You can enable the property to be sortable.
*/
@JvmName("yvfuypuacphkawhh")
public suspend fun sort(`value`: Output) {
this.sort = value
}
/**
* @param value A comma-separated list of source fields to map to the field. Specifying a source field copies data from one field to another, enabling you to use the same source data in different ways by configuring different options for the fields.
*/
@JvmName("sgajvlaptgsggkmq")
public suspend fun sourceFields(`value`: Output) {
this.sourceFields = value
}
/**
* @param value The field type. Valid values: `date`, `date-array`, `double`, `double-array`, `int`, `int-array`, `literal`, `literal-array`, `text`, `text-array`.
*/
@JvmName("ggilryvfnvngxnwg")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value The analysis scheme you want to use for a `text` field. The analysis scheme specifies the language-specific text processing options that are used during indexing.
*/
@JvmName("qnocttymdhfwgghh")
public suspend fun analysisScheme(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.analysisScheme = mapped
}
/**
* @param value The default value for the field. This value is used when no value is specified for the field in the document data.
*/
@JvmName("kslclvctqtryrnhg")
public suspend fun defaultValue(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultValue = mapped
}
/**
* @param value You can get facet information by enabling this.
*/
@JvmName("lminoifewxjmndej")
public suspend fun facet(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.facet = mapped
}
/**
* @param value You can highlight information.
*/
@JvmName("kmubopqmcqqshkea")
public suspend fun highlight(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.highlight = mapped
}
/**
* @param value A unique name for the field. Field names must begin with a letter and be at least 1 and no more than 64 characters long. The allowed characters are: `a`-`z` (lower-case letters), `0`-`9`, and `_` (underscore). The name `score` is reserved and cannot be used as a field name.
*/
@JvmName("redydwlqlvaituiv")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value You can enable returning the value of all searchable fields.
*/
@JvmName("qjtofrpdifioxrlg")
public suspend fun `return`(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.`return` = mapped
}
/**
* @param value You can set whether this index should be searchable or not.
*/
@JvmName("vkywedqvtfgnuchs")
public suspend fun search(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.search = mapped
}
/**
* @param value You can enable the property to be sortable.
*/
@JvmName("aojxadukcwyfgcph")
public suspend fun sort(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sort = mapped
}
/**
* @param value A comma-separated list of source fields to map to the field. Specifying a source field copies data from one field to another, enabling you to use the same source data in different ways by configuring different options for the fields.
*/
@JvmName("juejvmuuaragkrqh")
public suspend fun sourceFields(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceFields = mapped
}
/**
* @param value The field type. Valid values: `date`, `date-array`, `double`, `double-array`, `int`, `int-array`, `literal`, `literal-array`, `text`, `text-array`.
*/
@JvmName("sexqqqrvxdijexrr")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): DomainIndexFieldArgs = DomainIndexFieldArgs(
analysisScheme = analysisScheme,
defaultValue = defaultValue,
facet = facet,
highlight = highlight,
name = name ?: throw PulumiNullFieldException("name"),
`return` = `return`,
search = search,
sort = sort,
sourceFields = sourceFields,
type = type ?: throw PulumiNullFieldException("type"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy