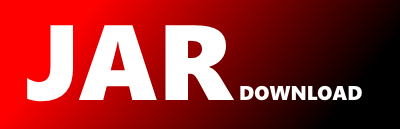
com.pulumi.aws.cloudwatch.kotlin.inputs.EventConnectionAuthParametersOauthArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cloudwatch.kotlin.inputs
import com.pulumi.aws.cloudwatch.inputs.EventConnectionAuthParametersOauthArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property authorizationEndpoint The URL to the authorization endpoint.
* @property clientParameters Contains the client parameters for OAuth authorization. Contains the following two parameters.
* @property httpMethod A password for the authorization. Created and stored in AWS Secrets Manager.
* @property oauthHttpParameters OAuth Http Parameters are additional credentials used to sign the request to the authorization endpoint to exchange the OAuth Client information for an access token. Secret values are stored and managed by AWS Secrets Manager. A maximum of 1 are allowed. Documented below.
*/
public data class EventConnectionAuthParametersOauthArgs(
public val authorizationEndpoint: Output,
public val clientParameters: Output? =
null,
public val httpMethod: Output,
public val oauthHttpParameters: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.cloudwatch.inputs.EventConnectionAuthParametersOauthArgs =
com.pulumi.aws.cloudwatch.inputs.EventConnectionAuthParametersOauthArgs.builder()
.authorizationEndpoint(authorizationEndpoint.applyValue({ args0 -> args0 }))
.clientParameters(clientParameters?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.httpMethod(httpMethod.applyValue({ args0 -> args0 }))
.oauthHttpParameters(
oauthHttpParameters.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [EventConnectionAuthParametersOauthArgs].
*/
@PulumiTagMarker
public class EventConnectionAuthParametersOauthArgsBuilder internal constructor() {
private var authorizationEndpoint: Output? = null
private var clientParameters: Output? =
null
private var httpMethod: Output? = null
private var oauthHttpParameters:
Output? = null
/**
* @param value The URL to the authorization endpoint.
*/
@JvmName("khbafoqtsbcwrxsf")
public suspend fun authorizationEndpoint(`value`: Output) {
this.authorizationEndpoint = value
}
/**
* @param value Contains the client parameters for OAuth authorization. Contains the following two parameters.
*/
@JvmName("bpjotgtprtuqnefn")
public suspend fun clientParameters(`value`: Output) {
this.clientParameters = value
}
/**
* @param value A password for the authorization. Created and stored in AWS Secrets Manager.
*/
@JvmName("mmbcgkbqgrcvdsvy")
public suspend fun httpMethod(`value`: Output) {
this.httpMethod = value
}
/**
* @param value OAuth Http Parameters are additional credentials used to sign the request to the authorization endpoint to exchange the OAuth Client information for an access token. Secret values are stored and managed by AWS Secrets Manager. A maximum of 1 are allowed. Documented below.
*/
@JvmName("fscuycsxcgvwghtr")
public suspend fun oauthHttpParameters(`value`: Output) {
this.oauthHttpParameters = value
}
/**
* @param value The URL to the authorization endpoint.
*/
@JvmName("qyhwhwcjygrupscq")
public suspend fun authorizationEndpoint(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.authorizationEndpoint = mapped
}
/**
* @param value Contains the client parameters for OAuth authorization. Contains the following two parameters.
*/
@JvmName("cvcejexrunkprgjo")
public suspend fun clientParameters(`value`: EventConnectionAuthParametersOauthClientParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clientParameters = mapped
}
/**
* @param argument Contains the client parameters for OAuth authorization. Contains the following two parameters.
*/
@JvmName("gggqmavbebmaeuwg")
public suspend fun clientParameters(argument: suspend EventConnectionAuthParametersOauthClientParametersArgsBuilder.() -> Unit) {
val toBeMapped = EventConnectionAuthParametersOauthClientParametersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.clientParameters = mapped
}
/**
* @param value A password for the authorization. Created and stored in AWS Secrets Manager.
*/
@JvmName("wydnkqxooepgcrob")
public suspend fun httpMethod(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.httpMethod = mapped
}
/**
* @param value OAuth Http Parameters are additional credentials used to sign the request to the authorization endpoint to exchange the OAuth Client information for an access token. Secret values are stored and managed by AWS Secrets Manager. A maximum of 1 are allowed. Documented below.
*/
@JvmName("iwwrxncnbjuxrydx")
public suspend fun oauthHttpParameters(`value`: EventConnectionAuthParametersOauthOauthHttpParametersArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.oauthHttpParameters = mapped
}
/**
* @param argument OAuth Http Parameters are additional credentials used to sign the request to the authorization endpoint to exchange the OAuth Client information for an access token. Secret values are stored and managed by AWS Secrets Manager. A maximum of 1 are allowed. Documented below.
*/
@JvmName("wxflsplomtcjewds")
public suspend fun oauthHttpParameters(argument: suspend EventConnectionAuthParametersOauthOauthHttpParametersArgsBuilder.() -> Unit) {
val toBeMapped =
EventConnectionAuthParametersOauthOauthHttpParametersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.oauthHttpParameters = mapped
}
internal fun build(): EventConnectionAuthParametersOauthArgs =
EventConnectionAuthParametersOauthArgs(
authorizationEndpoint = authorizationEndpoint ?: throw
PulumiNullFieldException("authorizationEndpoint"),
clientParameters = clientParameters,
httpMethod = httpMethod ?: throw PulumiNullFieldException("httpMethod"),
oauthHttpParameters = oauthHttpParameters ?: throw PulumiNullFieldException("oauthHttpParameters"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy