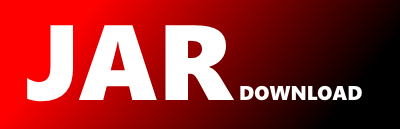
com.pulumi.aws.cloudwatch.kotlin.inputs.EventTargetRedshiftTargetArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cloudwatch.kotlin.inputs
import com.pulumi.aws.cloudwatch.inputs.EventTargetRedshiftTargetArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property database The name of the database.
* @property dbUser The database user name.
* @property secretsManagerArn The name or ARN of the secret that enables access to the database.
* @property sql The SQL statement text to run.
* @property statementName The name of the SQL statement.
* @property withEvent Indicates whether to send an event back to EventBridge after the SQL statement runs.
*/
public data class EventTargetRedshiftTargetArgs(
public val database: Output,
public val dbUser: Output? = null,
public val secretsManagerArn: Output? = null,
public val sql: Output? = null,
public val statementName: Output? = null,
public val withEvent: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.cloudwatch.inputs.EventTargetRedshiftTargetArgs =
com.pulumi.aws.cloudwatch.inputs.EventTargetRedshiftTargetArgs.builder()
.database(database.applyValue({ args0 -> args0 }))
.dbUser(dbUser?.applyValue({ args0 -> args0 }))
.secretsManagerArn(secretsManagerArn?.applyValue({ args0 -> args0 }))
.sql(sql?.applyValue({ args0 -> args0 }))
.statementName(statementName?.applyValue({ args0 -> args0 }))
.withEvent(withEvent?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EventTargetRedshiftTargetArgs].
*/
@PulumiTagMarker
public class EventTargetRedshiftTargetArgsBuilder internal constructor() {
private var database: Output? = null
private var dbUser: Output? = null
private var secretsManagerArn: Output? = null
private var sql: Output? = null
private var statementName: Output? = null
private var withEvent: Output? = null
/**
* @param value The name of the database.
*/
@JvmName("bgbleiehhsjkmxsn")
public suspend fun database(`value`: Output) {
this.database = value
}
/**
* @param value The database user name.
*/
@JvmName("vasgmbxwdaysypjp")
public suspend fun dbUser(`value`: Output) {
this.dbUser = value
}
/**
* @param value The name or ARN of the secret that enables access to the database.
*/
@JvmName("arakwcmjvadlcfgk")
public suspend fun secretsManagerArn(`value`: Output) {
this.secretsManagerArn = value
}
/**
* @param value The SQL statement text to run.
*/
@JvmName("hardoqkprectigfh")
public suspend fun sql(`value`: Output) {
this.sql = value
}
/**
* @param value The name of the SQL statement.
*/
@JvmName("qhpfbdfeaoscmbmq")
public suspend fun statementName(`value`: Output) {
this.statementName = value
}
/**
* @param value Indicates whether to send an event back to EventBridge after the SQL statement runs.
*/
@JvmName("oosmcsghcscmikdb")
public suspend fun withEvent(`value`: Output) {
this.withEvent = value
}
/**
* @param value The name of the database.
*/
@JvmName("wksvqswfcwlcyxgf")
public suspend fun database(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.database = mapped
}
/**
* @param value The database user name.
*/
@JvmName("tniuudnnqyjyawpn")
public suspend fun dbUser(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dbUser = mapped
}
/**
* @param value The name or ARN of the secret that enables access to the database.
*/
@JvmName("hhbkyxmjotdoghmm")
public suspend fun secretsManagerArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secretsManagerArn = mapped
}
/**
* @param value The SQL statement text to run.
*/
@JvmName("atkofbsvosgmblgw")
public suspend fun sql(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sql = mapped
}
/**
* @param value The name of the SQL statement.
*/
@JvmName("qctsyfmpnivcsoiv")
public suspend fun statementName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.statementName = mapped
}
/**
* @param value Indicates whether to send an event back to EventBridge after the SQL statement runs.
*/
@JvmName("oiynthfhfetegqam")
public suspend fun withEvent(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.withEvent = mapped
}
internal fun build(): EventTargetRedshiftTargetArgs = EventTargetRedshiftTargetArgs(
database = database ?: throw PulumiNullFieldException("database"),
dbUser = dbUser,
secretsManagerArn = secretsManagerArn,
sql = sql,
statementName = statementName,
withEvent = withEvent,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy