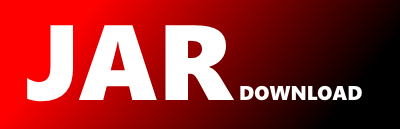
com.pulumi.aws.codeartifact.kotlin.RepositoryArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.codeartifact.kotlin
import com.pulumi.aws.codeartifact.RepositoryArgs.builder
import com.pulumi.aws.codeartifact.kotlin.inputs.RepositoryExternalConnectionsArgs
import com.pulumi.aws.codeartifact.kotlin.inputs.RepositoryExternalConnectionsArgsBuilder
import com.pulumi.aws.codeartifact.kotlin.inputs.RepositoryUpstreamArgs
import com.pulumi.aws.codeartifact.kotlin.inputs.RepositoryUpstreamArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Provides a CodeArtifact Repository Resource.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.kms.Key("example", {description: "domain key"});
* const exampleDomain = new aws.codeartifact.Domain("example", {
* domain: "example",
* encryptionKey: example.arn,
* });
* const test = new aws.codeartifact.Repository("test", {
* repository: "example",
* domain: exampleDomain.domain,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.kms.Key("example", description="domain key")
* example_domain = aws.codeartifact.Domain("example",
* domain="example",
* encryption_key=example.arn)
* test = aws.codeartifact.Repository("test",
* repository="example",
* domain=example_domain.domain)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Kms.Key("example", new()
* {
* Description = "domain key",
* });
* var exampleDomain = new Aws.CodeArtifact.Domain("example", new()
* {
* DomainName = "example",
* EncryptionKey = example.Arn,
* });
* var test = new Aws.CodeArtifact.Repository("test", new()
* {
* RepositoryName = "example",
* Domain = exampleDomain.DomainName,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/codeartifact"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/kms"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := kms.NewKey(ctx, "example", &kms.KeyArgs{
* Description: pulumi.String("domain key"),
* })
* if err != nil {
* return err
* }
* exampleDomain, err := codeartifact.NewDomain(ctx, "example", &codeartifact.DomainArgs{
* Domain: pulumi.String("example"),
* EncryptionKey: example.Arn,
* })
* if err != nil {
* return err
* }
* _, err = codeartifact.NewRepository(ctx, "test", &codeartifact.RepositoryArgs{
* Repository: pulumi.String("example"),
* Domain: exampleDomain.Domain,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kms.Key;
* import com.pulumi.aws.kms.KeyArgs;
* import com.pulumi.aws.codeartifact.Domain;
* import com.pulumi.aws.codeartifact.DomainArgs;
* import com.pulumi.aws.codeartifact.Repository;
* import com.pulumi.aws.codeartifact.RepositoryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Key("example", KeyArgs.builder()
* .description("domain key")
* .build());
* var exampleDomain = new Domain("exampleDomain", DomainArgs.builder()
* .domain("example")
* .encryptionKey(example.arn())
* .build());
* var test = new Repository("test", RepositoryArgs.builder()
* .repository("example")
* .domain(exampleDomain.domain())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:kms:Key
* properties:
* description: domain key
* exampleDomain:
* type: aws:codeartifact:Domain
* name: example
* properties:
* domain: example
* encryptionKey: ${example.arn}
* test:
* type: aws:codeartifact:Repository
* properties:
* repository: example
* domain: ${exampleDomain.domain}
* ```
*
* ### With Upstream Repository
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const upstream = new aws.codeartifact.Repository("upstream", {
* repository: "upstream",
* domain: testAwsCodeartifactDomain.domain,
* });
* const test = new aws.codeartifact.Repository("test", {
* repository: "example",
* domain: example.domain,
* upstreams: [{
* repositoryName: upstream.repository,
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* upstream = aws.codeartifact.Repository("upstream",
* repository="upstream",
* domain=test_aws_codeartifact_domain["domain"])
* test = aws.codeartifact.Repository("test",
* repository="example",
* domain=example["domain"],
* upstreams=[{
* "repository_name": upstream.repository,
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var upstream = new Aws.CodeArtifact.Repository("upstream", new()
* {
* RepositoryName = "upstream",
* Domain = testAwsCodeartifactDomain.Domain,
* });
* var test = new Aws.CodeArtifact.Repository("test", new()
* {
* RepositoryName = "example",
* Domain = example.Domain,
* Upstreams = new[]
* {
* new Aws.CodeArtifact.Inputs.RepositoryUpstreamArgs
* {
* RepositoryName = upstream.RepositoryName,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/codeartifact"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* upstream, err := codeartifact.NewRepository(ctx, "upstream", &codeartifact.RepositoryArgs{
* Repository: pulumi.String("upstream"),
* Domain: pulumi.Any(testAwsCodeartifactDomain.Domain),
* })
* if err != nil {
* return err
* }
* _, err = codeartifact.NewRepository(ctx, "test", &codeartifact.RepositoryArgs{
* Repository: pulumi.String("example"),
* Domain: pulumi.Any(example.Domain),
* Upstreams: codeartifact.RepositoryUpstreamArray{
* &codeartifact.RepositoryUpstreamArgs{
* RepositoryName: upstream.Repository,
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.codeartifact.Repository;
* import com.pulumi.aws.codeartifact.RepositoryArgs;
* import com.pulumi.aws.codeartifact.inputs.RepositoryUpstreamArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var upstream = new Repository("upstream", RepositoryArgs.builder()
* .repository("upstream")
* .domain(testAwsCodeartifactDomain.domain())
* .build());
* var test = new Repository("test", RepositoryArgs.builder()
* .repository("example")
* .domain(example.domain())
* .upstreams(RepositoryUpstreamArgs.builder()
* .repositoryName(upstream.repository())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* upstream:
* type: aws:codeartifact:Repository
* properties:
* repository: upstream
* domain: ${testAwsCodeartifactDomain.domain}
* test:
* type: aws:codeartifact:Repository
* properties:
* repository: example
* domain: ${example.domain}
* upstreams:
* - repositoryName: ${upstream.repository}
* ```
*
* ### With External Connection
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const upstream = new aws.codeartifact.Repository("upstream", {
* repository: "upstream",
* domain: testAwsCodeartifactDomain.domain,
* });
* const test = new aws.codeartifact.Repository("test", {
* repository: "example",
* domain: example.domain,
* externalConnections: {
* externalConnectionName: "public:npmjs",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* upstream = aws.codeartifact.Repository("upstream",
* repository="upstream",
* domain=test_aws_codeartifact_domain["domain"])
* test = aws.codeartifact.Repository("test",
* repository="example",
* domain=example["domain"],
* external_connections={
* "external_connection_name": "public:npmjs",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var upstream = new Aws.CodeArtifact.Repository("upstream", new()
* {
* RepositoryName = "upstream",
* Domain = testAwsCodeartifactDomain.Domain,
* });
* var test = new Aws.CodeArtifact.Repository("test", new()
* {
* RepositoryName = "example",
* Domain = example.Domain,
* ExternalConnections = new Aws.CodeArtifact.Inputs.RepositoryExternalConnectionsArgs
* {
* ExternalConnectionName = "public:npmjs",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/codeartifact"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := codeartifact.NewRepository(ctx, "upstream", &codeartifact.RepositoryArgs{
* Repository: pulumi.String("upstream"),
* Domain: pulumi.Any(testAwsCodeartifactDomain.Domain),
* })
* if err != nil {
* return err
* }
* _, err = codeartifact.NewRepository(ctx, "test", &codeartifact.RepositoryArgs{
* Repository: pulumi.String("example"),
* Domain: pulumi.Any(example.Domain),
* ExternalConnections: &codeartifact.RepositoryExternalConnectionsArgs{
* ExternalConnectionName: pulumi.String("public:npmjs"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.codeartifact.Repository;
* import com.pulumi.aws.codeartifact.RepositoryArgs;
* import com.pulumi.aws.codeartifact.inputs.RepositoryExternalConnectionsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var upstream = new Repository("upstream", RepositoryArgs.builder()
* .repository("upstream")
* .domain(testAwsCodeartifactDomain.domain())
* .build());
* var test = new Repository("test", RepositoryArgs.builder()
* .repository("example")
* .domain(example.domain())
* .externalConnections(RepositoryExternalConnectionsArgs.builder()
* .externalConnectionName("public:npmjs")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* upstream:
* type: aws:codeartifact:Repository
* properties:
* repository: upstream
* domain: ${testAwsCodeartifactDomain.domain}
* test:
* type: aws:codeartifact:Repository
* properties:
* repository: example
* domain: ${example.domain}
* externalConnections:
* externalConnectionName: public:npmjs
* ```
*
* ## Import
* Using `pulumi import`, import CodeArtifact Repository using the CodeArtifact Repository ARN. For example:
* ```sh
* $ pulumi import aws:codeartifact/repository:Repository example arn:aws:codeartifact:us-west-2:012345678912:repository/tf-acc-test-6968272603913957763/tf-acc-test-6968272603913957763
* ```
* @property description The description of the repository.
* @property domain The domain that contains the created repository.
* @property domainOwner The account number of the AWS account that owns the domain.
* @property externalConnections An array of external connections associated with the repository. Only one external connection can be set per repository. see External Connections.
* @property repository The name of the repository to create.
* @property tags Key-value map of resource tags. .If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
* @property upstreams A list of upstream repositories to associate with the repository. The order of the upstream repositories in the list determines their priority order when AWS CodeArtifact looks for a requested package version. see Upstream
*/
public data class RepositoryArgs(
public val description: Output? = null,
public val domain: Output? = null,
public val domainOwner: Output? = null,
public val externalConnections: Output? = null,
public val repository: Output? = null,
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy