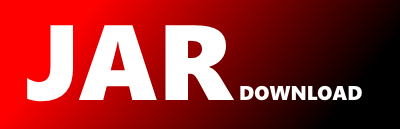
com.pulumi.aws.codeartifact.kotlin.RepositoryPermissionsPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.codeartifact.kotlin
import com.pulumi.aws.codeartifact.RepositoryPermissionsPolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Provides a CodeArtifact Repostory Permissions Policy Resource.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const exampleKey = new aws.kms.Key("example", {description: "domain key"});
* const exampleDomain = new aws.codeartifact.Domain("example", {
* domain: "example",
* encryptionKey: exampleKey.arn,
* });
* const exampleRepository = new aws.codeartifact.Repository("example", {
* repository: "example",
* domain: exampleDomain.domain,
* });
* const example = aws.iam.getPolicyDocumentOutput({
* statements: [{
* effect: "Allow",
* principals: [{
* type: "*",
* identifiers: ["*"],
* }],
* actions: ["codeartifact:ReadFromRepository"],
* resources: [exampleRepository.arn],
* }],
* });
* const exampleRepositoryPermissionsPolicy = new aws.codeartifact.RepositoryPermissionsPolicy("example", {
* repository: exampleRepository.repository,
* domain: exampleDomain.domain,
* policyDocument: example.apply(example => example.json),
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example_key = aws.kms.Key("example", description="domain key")
* example_domain = aws.codeartifact.Domain("example",
* domain="example",
* encryption_key=example_key.arn)
* example_repository = aws.codeartifact.Repository("example",
* repository="example",
* domain=example_domain.domain)
* example = aws.iam.get_policy_document_output(statements=[{
* "effect": "Allow",
* "principals": [{
* "type": "*",
* "identifiers": ["*"],
* }],
* "actions": ["codeartifact:ReadFromRepository"],
* "resources": [example_repository.arn],
* }])
* example_repository_permissions_policy = aws.codeartifact.RepositoryPermissionsPolicy("example",
* repository=example_repository.repository,
* domain=example_domain.domain,
* policy_document=example.json)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var exampleKey = new Aws.Kms.Key("example", new()
* {
* Description = "domain key",
* });
* var exampleDomain = new Aws.CodeArtifact.Domain("example", new()
* {
* DomainName = "example",
* EncryptionKey = exampleKey.Arn,
* });
* var exampleRepository = new Aws.CodeArtifact.Repository("example", new()
* {
* RepositoryName = "example",
* Domain = exampleDomain.DomainName,
* });
* var example = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Effect = "Allow",
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "*",
* Identifiers = new[]
* {
* "*",
* },
* },
* },
* Actions = new[]
* {
* "codeartifact:ReadFromRepository",
* },
* Resources = new[]
* {
* exampleRepository.Arn,
* },
* },
* },
* });
* var exampleRepositoryPermissionsPolicy = new Aws.CodeArtifact.RepositoryPermissionsPolicy("example", new()
* {
* Repository = exampleRepository.RepositoryName,
* Domain = exampleDomain.DomainName,
* PolicyDocument = example.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/codeartifact"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/kms"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* exampleKey, err := kms.NewKey(ctx, "example", &kms.KeyArgs{
* Description: pulumi.String("domain key"),
* })
* if err != nil {
* return err
* }
* exampleDomain, err := codeartifact.NewDomain(ctx, "example", &codeartifact.DomainArgs{
* Domain: pulumi.String("example"),
* EncryptionKey: exampleKey.Arn,
* })
* if err != nil {
* return err
* }
* exampleRepository, err := codeartifact.NewRepository(ctx, "example", &codeartifact.RepositoryArgs{
* Repository: pulumi.String("example"),
* Domain: exampleDomain.Domain,
* })
* if err != nil {
* return err
* }
* example := iam.GetPolicyDocumentOutput(ctx, iam.GetPolicyDocumentOutputArgs{
* Statements: iam.GetPolicyDocumentStatementArray{
* &iam.GetPolicyDocumentStatementArgs{
* Effect: pulumi.String("Allow"),
* Principals: iam.GetPolicyDocumentStatementPrincipalArray{
* &iam.GetPolicyDocumentStatementPrincipalArgs{
* Type: pulumi.String("*"),
* Identifiers: pulumi.StringArray{
* pulumi.String("*"),
* },
* },
* },
* Actions: pulumi.StringArray{
* pulumi.String("codeartifact:ReadFromRepository"),
* },
* Resources: pulumi.StringArray{
* exampleRepository.Arn,
* },
* },
* },
* }, nil)
* _, err = codeartifact.NewRepositoryPermissionsPolicy(ctx, "example", &codeartifact.RepositoryPermissionsPolicyArgs{
* Repository: exampleRepository.Repository,
* Domain: exampleDomain.Domain,
* PolicyDocument: pulumi.String(example.ApplyT(func(example iam.GetPolicyDocumentResult) (*string, error) {
* return &example.Json, nil
* }).(pulumi.StringPtrOutput)),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kms.Key;
* import com.pulumi.aws.kms.KeyArgs;
* import com.pulumi.aws.codeartifact.Domain;
* import com.pulumi.aws.codeartifact.DomainArgs;
* import com.pulumi.aws.codeartifact.Repository;
* import com.pulumi.aws.codeartifact.RepositoryArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.codeartifact.RepositoryPermissionsPolicy;
* import com.pulumi.aws.codeartifact.RepositoryPermissionsPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var exampleKey = new Key("exampleKey", KeyArgs.builder()
* .description("domain key")
* .build());
* var exampleDomain = new Domain("exampleDomain", DomainArgs.builder()
* .domain("example")
* .encryptionKey(exampleKey.arn())
* .build());
* var exampleRepository = new Repository("exampleRepository", RepositoryArgs.builder()
* .repository("example")
* .domain(exampleDomain.domain())
* .build());
* final var example = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("*")
* .identifiers("*")
* .build())
* .actions("codeartifact:ReadFromRepository")
* .resources(exampleRepository.arn())
* .build())
* .build());
* var exampleRepositoryPermissionsPolicy = new RepositoryPermissionsPolicy("exampleRepositoryPermissionsPolicy", RepositoryPermissionsPolicyArgs.builder()
* .repository(exampleRepository.repository())
* .domain(exampleDomain.domain())
* .policyDocument(example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult).applyValue(example -> example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json())))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleKey:
* type: aws:kms:Key
* name: example
* properties:
* description: domain key
* exampleDomain:
* type: aws:codeartifact:Domain
* name: example
* properties:
* domain: example
* encryptionKey: ${exampleKey.arn}
* exampleRepository:
* type: aws:codeartifact:Repository
* name: example
* properties:
* repository: example
* domain: ${exampleDomain.domain}
* exampleRepositoryPermissionsPolicy:
* type: aws:codeartifact:RepositoryPermissionsPolicy
* name: example
* properties:
* repository: ${exampleRepository.repository}
* domain: ${exampleDomain.domain}
* policyDocument: ${example.json}
* variables:
* example:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - effect: Allow
* principals:
* - type: '*'
* identifiers:
* - '*'
* actions:
* - codeartifact:ReadFromRepository
* resources:
* - ${exampleRepository.arn}
* ```
*
* ## Import
* Using `pulumi import`, import CodeArtifact Repository Permissions Policies using the CodeArtifact Repository ARN. For example:
* ```sh
* $ pulumi import aws:codeartifact/repositoryPermissionsPolicy:RepositoryPermissionsPolicy example arn:aws:codeartifact:us-west-2:012345678912:repository/tf-acc-test-6968272603913957763/tf-acc-test-6968272603913957763
* ```
* @property domain The name of the domain on which to set the resource policy.
* @property domainOwner The account number of the AWS account that owns the domain.
* @property policyDocument A JSON policy string to be set as the access control resource policy on the provided domain.
* @property policyRevision The current revision of the resource policy to be set. This revision is used for optimistic locking, which prevents others from overwriting your changes to the domain's resource policy.
* @property repository The name of the repository to set the resource policy on.
*/
public data class RepositoryPermissionsPolicyArgs(
public val domain: Output? = null,
public val domainOwner: Output? = null,
public val policyDocument: Output? = null,
public val policyRevision: Output? = null,
public val repository: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.codeartifact.RepositoryPermissionsPolicyArgs =
com.pulumi.aws.codeartifact.RepositoryPermissionsPolicyArgs.builder()
.domain(domain?.applyValue({ args0 -> args0 }))
.domainOwner(domainOwner?.applyValue({ args0 -> args0 }))
.policyDocument(policyDocument?.applyValue({ args0 -> args0 }))
.policyRevision(policyRevision?.applyValue({ args0 -> args0 }))
.repository(repository?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RepositoryPermissionsPolicyArgs].
*/
@PulumiTagMarker
public class RepositoryPermissionsPolicyArgsBuilder internal constructor() {
private var domain: Output? = null
private var domainOwner: Output? = null
private var policyDocument: Output? = null
private var policyRevision: Output? = null
private var repository: Output? = null
/**
* @param value The name of the domain on which to set the resource policy.
*/
@JvmName("fxacpcnaqxubexqk")
public suspend fun domain(`value`: Output) {
this.domain = value
}
/**
* @param value The account number of the AWS account that owns the domain.
*/
@JvmName("xpdfvojirjxigkye")
public suspend fun domainOwner(`value`: Output) {
this.domainOwner = value
}
/**
* @param value A JSON policy string to be set as the access control resource policy on the provided domain.
*/
@JvmName("xqupdtjyptbgywgf")
public suspend fun policyDocument(`value`: Output) {
this.policyDocument = value
}
/**
* @param value The current revision of the resource policy to be set. This revision is used for optimistic locking, which prevents others from overwriting your changes to the domain's resource policy.
*/
@JvmName("eqiwwgbhfwkdkedg")
public suspend fun policyRevision(`value`: Output) {
this.policyRevision = value
}
/**
* @param value The name of the repository to set the resource policy on.
*/
@JvmName("dkpfpetssgxtpjmd")
public suspend fun repository(`value`: Output) {
this.repository = value
}
/**
* @param value The name of the domain on which to set the resource policy.
*/
@JvmName("reeajjbjadqmvoot")
public suspend fun domain(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.domain = mapped
}
/**
* @param value The account number of the AWS account that owns the domain.
*/
@JvmName("ortitdcnstiogbsa")
public suspend fun domainOwner(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.domainOwner = mapped
}
/**
* @param value A JSON policy string to be set as the access control resource policy on the provided domain.
*/
@JvmName("drlnnurjqibajcet")
public suspend fun policyDocument(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.policyDocument = mapped
}
/**
* @param value The current revision of the resource policy to be set. This revision is used for optimistic locking, which prevents others from overwriting your changes to the domain's resource policy.
*/
@JvmName("wsaclxhdkfuckcvv")
public suspend fun policyRevision(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.policyRevision = mapped
}
/**
* @param value The name of the repository to set the resource policy on.
*/
@JvmName("dkfgdhibolxvegpy")
public suspend fun repository(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.repository = mapped
}
internal fun build(): RepositoryPermissionsPolicyArgs = RepositoryPermissionsPolicyArgs(
domain = domain,
domainOwner = domainOwner,
policyDocument = policyDocument,
policyRevision = policyRevision,
repository = repository,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy