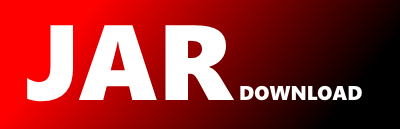
com.pulumi.aws.codebuild.kotlin.Fleet.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.codebuild.kotlin
import com.pulumi.aws.codebuild.kotlin.outputs.FleetScalingConfiguration
import com.pulumi.aws.codebuild.kotlin.outputs.FleetStatus
import com.pulumi.aws.codebuild.kotlin.outputs.FleetVpcConfig
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.aws.codebuild.kotlin.outputs.FleetScalingConfiguration.Companion.toKotlin as fleetScalingConfigurationToKotlin
import com.pulumi.aws.codebuild.kotlin.outputs.FleetStatus.Companion.toKotlin as fleetStatusToKotlin
import com.pulumi.aws.codebuild.kotlin.outputs.FleetVpcConfig.Companion.toKotlin as fleetVpcConfigToKotlin
/**
* Builder for [Fleet].
*/
@PulumiTagMarker
public class FleetResourceBuilder internal constructor() {
public var name: String? = null
public var args: FleetArgs = FleetArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend FleetArgsBuilder.() -> Unit) {
val builder = FleetArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Fleet {
val builtJavaResource = com.pulumi.aws.codebuild.Fleet(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Fleet(builtJavaResource)
}
}
/**
* Provides a CodeBuild Fleet Resource.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const test = new aws.codebuild.Fleet("test", {
* baseCapacity: 2,
* computeType: "BUILD_GENERAL1_SMALL",
* environmentType: "LINUX_CONTAINER",
* name: "full-example-codebuild-fleet",
* overflowBehavior: "QUEUE",
* scalingConfiguration: {
* maxCapacity: 5,
* scalingType: "TARGET_TRACKING_SCALING",
* targetTrackingScalingConfigs: [{
* metricType: "FLEET_UTILIZATION_RATE",
* targetValue: 97.5,
* }],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* test = aws.codebuild.Fleet("test",
* base_capacity=2,
* compute_type="BUILD_GENERAL1_SMALL",
* environment_type="LINUX_CONTAINER",
* name="full-example-codebuild-fleet",
* overflow_behavior="QUEUE",
* scaling_configuration={
* "max_capacity": 5,
* "scaling_type": "TARGET_TRACKING_SCALING",
* "target_tracking_scaling_configs": [{
* "metric_type": "FLEET_UTILIZATION_RATE",
* "target_value": 97.5,
* }],
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var test = new Aws.CodeBuild.Fleet("test", new()
* {
* BaseCapacity = 2,
* ComputeType = "BUILD_GENERAL1_SMALL",
* EnvironmentType = "LINUX_CONTAINER",
* Name = "full-example-codebuild-fleet",
* OverflowBehavior = "QUEUE",
* ScalingConfiguration = new Aws.CodeBuild.Inputs.FleetScalingConfigurationArgs
* {
* MaxCapacity = 5,
* ScalingType = "TARGET_TRACKING_SCALING",
* TargetTrackingScalingConfigs = new[]
* {
* new Aws.CodeBuild.Inputs.FleetScalingConfigurationTargetTrackingScalingConfigArgs
* {
* MetricType = "FLEET_UTILIZATION_RATE",
* TargetValue = 97.5,
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/codebuild"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := codebuild.NewFleet(ctx, "test", &codebuild.FleetArgs{
* BaseCapacity: pulumi.Int(2),
* ComputeType: pulumi.String("BUILD_GENERAL1_SMALL"),
* EnvironmentType: pulumi.String("LINUX_CONTAINER"),
* Name: pulumi.String("full-example-codebuild-fleet"),
* OverflowBehavior: pulumi.String("QUEUE"),
* ScalingConfiguration: &codebuild.FleetScalingConfigurationArgs{
* MaxCapacity: pulumi.Int(5),
* ScalingType: pulumi.String("TARGET_TRACKING_SCALING"),
* TargetTrackingScalingConfigs: codebuild.FleetScalingConfigurationTargetTrackingScalingConfigArray{
* &codebuild.FleetScalingConfigurationTargetTrackingScalingConfigArgs{
* MetricType: pulumi.String("FLEET_UTILIZATION_RATE"),
* TargetValue: pulumi.Float64(97.5),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.codebuild.Fleet;
* import com.pulumi.aws.codebuild.FleetArgs;
* import com.pulumi.aws.codebuild.inputs.FleetScalingConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new Fleet("test", FleetArgs.builder()
* .baseCapacity(2)
* .computeType("BUILD_GENERAL1_SMALL")
* .environmentType("LINUX_CONTAINER")
* .name("full-example-codebuild-fleet")
* .overflowBehavior("QUEUE")
* .scalingConfiguration(FleetScalingConfigurationArgs.builder()
* .maxCapacity(5)
* .scalingType("TARGET_TRACKING_SCALING")
* .targetTrackingScalingConfigs(FleetScalingConfigurationTargetTrackingScalingConfigArgs.builder()
* .metricType("FLEET_UTILIZATION_RATE")
* .targetValue(97.5)
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: aws:codebuild:Fleet
* properties:
* baseCapacity: 2
* computeType: BUILD_GENERAL1_SMALL
* environmentType: LINUX_CONTAINER
* name: full-example-codebuild-fleet
* overflowBehavior: QUEUE
* scalingConfiguration:
* maxCapacity: 5
* scalingType: TARGET_TRACKING_SCALING
* targetTrackingScalingConfigs:
* - metricType: FLEET_UTILIZATION_RATE
* targetValue: 97.5
* ```
*
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.codebuild.Fleet("example", {name: "example-codebuild-fleet"});
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.codebuild.Fleet("example", name="example-codebuild-fleet")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.CodeBuild.Fleet("example", new()
* {
* Name = "example-codebuild-fleet",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/codebuild"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := codebuild.NewFleet(ctx, "example", &codebuild.FleetArgs{
* Name: pulumi.String("example-codebuild-fleet"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.codebuild.Fleet;
* import com.pulumi.aws.codebuild.FleetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Fleet("example", FleetArgs.builder()
* .name("example-codebuild-fleet")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:codebuild:Fleet
* properties:
* name: example-codebuild-fleet
* ```
*
* ## Import
* Using `pulumi import`, import CodeBuild Fleet using the `name`. For example:
* ```sh
* $ pulumi import aws:codebuild/fleet:Fleet name fleet-name
* ```
*/
public class Fleet internal constructor(
override val javaResource: com.pulumi.aws.codebuild.Fleet,
) : KotlinCustomResource(javaResource, FleetMapper) {
/**
* ARN of the Fleet.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Number of machines allocated to the fleet.
*/
public val baseCapacity: Output
get() = javaResource.baseCapacity().applyValue({ args0 -> args0 })
/**
* Compute resources the compute fleet uses. See [compute types](https://docs.aws.amazon.com/codebuild/latest/userguide/build-env-ref-compute-types.html#environment.types) for more information and valid values.
*/
public val computeType: Output
get() = javaResource.computeType().applyValue({ args0 -> args0 })
/**
* Creation time of the fleet.
*/
public val created: Output
get() = javaResource.created().applyValue({ args0 -> args0 })
/**
* Environment type of the compute fleet. See [environment types](https://docs.aws.amazon.com/codebuild/latest/userguide/build-env-ref-compute-types.html#environment.types) for more information and valid values.
* The following arguments are optional:
*/
public val environmentType: Output
get() = javaResource.environmentType().applyValue({ args0 -> args0 })
/**
* The service role associated with the compute fleet.
*/
public val fleetServiceRole: Output?
get() = javaResource.fleetServiceRole().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The Amazon Machine Image (AMI) of the compute fleet.
*/
public val imageId: Output?
get() = javaResource.imageId().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Last modification time of the fleet.
*/
public val lastModified: Output
get() = javaResource.lastModified().applyValue({ args0 -> args0 })
/**
* Fleet name.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Overflow behavior for compute fleet. Valid values: `ON_DEMAND`, `QUEUE`.
*/
public val overflowBehavior: Output
get() = javaResource.overflowBehavior().applyValue({ args0 -> args0 })
/**
* Configuration block. Detailed below. This option is only valid when your overflow behavior is `QUEUE`.
*/
public val scalingConfiguration: Output?
get() = javaResource.scalingConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> fleetScalingConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* Nested attribute containing information about the current status of the fleet.
*/
public val statuses: Output>
get() = javaResource.statuses().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
fleetStatusToKotlin(args0)
})
})
})
/**
* Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy