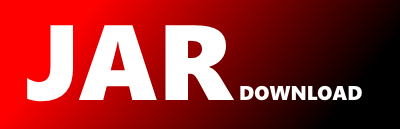
com.pulumi.aws.codebuild.kotlin.inputs.ProjectLogsConfigS3LogsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.codebuild.kotlin.inputs
import com.pulumi.aws.codebuild.inputs.ProjectLogsConfigS3LogsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property bucketOwnerAccess Specifies the bucket owner's access for objects that another account uploads to their Amazon S3 bucket. By default, only the account that uploads the objects to the bucket has access to these objects. This property allows you to give the bucket owner access to these objects. Valid values are `NONE`, `READ_ONLY`, and `FULL`. your CodeBuild service role must have the `s3:PutBucketAcl` permission. This permission allows CodeBuild to modify the access control list for the bucket.
* @property encryptionDisabled Whether to disable encrypting S3 logs. Defaults to `false`.
* @property location Name of the S3 bucket and the path prefix for S3 logs. Must be set if status is `ENABLED`, otherwise it must be empty.
* @property status Current status of logs in S3 for a build project. Valid values: `ENABLED`, `DISABLED`. Defaults to `DISABLED`.
*/
public data class ProjectLogsConfigS3LogsArgs(
public val bucketOwnerAccess: Output? = null,
public val encryptionDisabled: Output? = null,
public val location: Output? = null,
public val status: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.codebuild.inputs.ProjectLogsConfigS3LogsArgs =
com.pulumi.aws.codebuild.inputs.ProjectLogsConfigS3LogsArgs.builder()
.bucketOwnerAccess(bucketOwnerAccess?.applyValue({ args0 -> args0 }))
.encryptionDisabled(encryptionDisabled?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.status(status?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ProjectLogsConfigS3LogsArgs].
*/
@PulumiTagMarker
public class ProjectLogsConfigS3LogsArgsBuilder internal constructor() {
private var bucketOwnerAccess: Output? = null
private var encryptionDisabled: Output? = null
private var location: Output? = null
private var status: Output? = null
/**
* @param value Specifies the bucket owner's access for objects that another account uploads to their Amazon S3 bucket. By default, only the account that uploads the objects to the bucket has access to these objects. This property allows you to give the bucket owner access to these objects. Valid values are `NONE`, `READ_ONLY`, and `FULL`. your CodeBuild service role must have the `s3:PutBucketAcl` permission. This permission allows CodeBuild to modify the access control list for the bucket.
*/
@JvmName("tfjxdaicxuunuecs")
public suspend fun bucketOwnerAccess(`value`: Output) {
this.bucketOwnerAccess = value
}
/**
* @param value Whether to disable encrypting S3 logs. Defaults to `false`.
*/
@JvmName("kibpusjgojnevgtw")
public suspend fun encryptionDisabled(`value`: Output) {
this.encryptionDisabled = value
}
/**
* @param value Name of the S3 bucket and the path prefix for S3 logs. Must be set if status is `ENABLED`, otherwise it must be empty.
*/
@JvmName("uvrxjdutrstqrepj")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value Current status of logs in S3 for a build project. Valid values: `ENABLED`, `DISABLED`. Defaults to `DISABLED`.
*/
@JvmName("tlnwrcycueqcbcvo")
public suspend fun status(`value`: Output) {
this.status = value
}
/**
* @param value Specifies the bucket owner's access for objects that another account uploads to their Amazon S3 bucket. By default, only the account that uploads the objects to the bucket has access to these objects. This property allows you to give the bucket owner access to these objects. Valid values are `NONE`, `READ_ONLY`, and `FULL`. your CodeBuild service role must have the `s3:PutBucketAcl` permission. This permission allows CodeBuild to modify the access control list for the bucket.
*/
@JvmName("abqtbokkywhijbam")
public suspend fun bucketOwnerAccess(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bucketOwnerAccess = mapped
}
/**
* @param value Whether to disable encrypting S3 logs. Defaults to `false`.
*/
@JvmName("lyirafxxtqhvwevx")
public suspend fun encryptionDisabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryptionDisabled = mapped
}
/**
* @param value Name of the S3 bucket and the path prefix for S3 logs. Must be set if status is `ENABLED`, otherwise it must be empty.
*/
@JvmName("xfxfpxeqhqtcjvsi")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value Current status of logs in S3 for a build project. Valid values: `ENABLED`, `DISABLED`. Defaults to `DISABLED`.
*/
@JvmName("dunhlfvkvkmakptg")
public suspend fun status(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.status = mapped
}
internal fun build(): ProjectLogsConfigS3LogsArgs = ProjectLogsConfigS3LogsArgs(
bucketOwnerAccess = bucketOwnerAccess,
encryptionDisabled = encryptionDisabled,
location = location,
status = status,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy