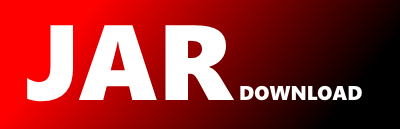
com.pulumi.aws.codebuild.kotlin.inputs.ProjectSecondarySourceArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.codebuild.kotlin.inputs
import com.pulumi.aws.codebuild.inputs.ProjectSecondarySourceArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property buildStatusConfig Configuration block that contains information that defines how the build project reports the build status to the source provider. This option is only used when the source provider is GitHub, GitHub Enterprise, GitLab, GitLab Self Managed, or Bitbucket. `build_status_config` blocks are documented below.
* @property buildspec The build spec declaration to use for this build project's related builds. This must be set when `type` is `NO_SOURCE`. It can either be a path to a file residing in the repository to be built or a local file path leveraging the `file()` built-in.
* @property gitCloneDepth Truncate git history to this many commits. Use `0` for a `Full` checkout which you need to run commands like `git branch --show-current`. See [AWS CodePipeline User Guide: Tutorial: Use full clone with a GitHub pipeline source](https://docs.aws.amazon.com/codepipeline/latest/userguide/tutorials-github-gitclone.html) for details.
* @property gitSubmodulesConfig Configuration block. Detailed below.
* @property insecureSsl Ignore SSL warnings when connecting to source control.
* @property location Location of the source code from git or s3.
* @property reportBuildStatus Whether to report the status of a build's start and finish to your source provider. This option is valid only when your source provider is GitHub, GitHub Enterprise, GitLab, GitLab Self Managed, or Bitbucket.
* @property sourceIdentifier An identifier for this project source. The identifier can only contain alphanumeric characters and underscores, and must be less than 128 characters in length.
* @property type Type of repository that contains the source code to be built. Valid values: `BITBUCKET`, `CODECOMMIT`, `CODEPIPELINE`, `GITHUB`, `GITHUB_ENTERPRISE`, `GITLAB`, `GITLAB_SELF_MANAGED`, `NO_SOURCE`, `S3`.
*/
public data class ProjectSecondarySourceArgs(
public val buildStatusConfig: Output? = null,
public val buildspec: Output? = null,
public val gitCloneDepth: Output? = null,
public val gitSubmodulesConfig: Output? = null,
public val insecureSsl: Output? = null,
public val location: Output? = null,
public val reportBuildStatus: Output? = null,
public val sourceIdentifier: Output,
public val type: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.codebuild.inputs.ProjectSecondarySourceArgs =
com.pulumi.aws.codebuild.inputs.ProjectSecondarySourceArgs.builder()
.buildStatusConfig(buildStatusConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.buildspec(buildspec?.applyValue({ args0 -> args0 }))
.gitCloneDepth(gitCloneDepth?.applyValue({ args0 -> args0 }))
.gitSubmodulesConfig(
gitSubmodulesConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.insecureSsl(insecureSsl?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.reportBuildStatus(reportBuildStatus?.applyValue({ args0 -> args0 }))
.sourceIdentifier(sourceIdentifier.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ProjectSecondarySourceArgs].
*/
@PulumiTagMarker
public class ProjectSecondarySourceArgsBuilder internal constructor() {
private var buildStatusConfig: Output? = null
private var buildspec: Output? = null
private var gitCloneDepth: Output? = null
private var gitSubmodulesConfig: Output? = null
private var insecureSsl: Output? = null
private var location: Output? = null
private var reportBuildStatus: Output? = null
private var sourceIdentifier: Output? = null
private var type: Output? = null
/**
* @param value Configuration block that contains information that defines how the build project reports the build status to the source provider. This option is only used when the source provider is GitHub, GitHub Enterprise, GitLab, GitLab Self Managed, or Bitbucket. `build_status_config` blocks are documented below.
*/
@JvmName("gfgjuyqsudufxrld")
public suspend fun buildStatusConfig(`value`: Output) {
this.buildStatusConfig = value
}
/**
* @param value The build spec declaration to use for this build project's related builds. This must be set when `type` is `NO_SOURCE`. It can either be a path to a file residing in the repository to be built or a local file path leveraging the `file()` built-in.
*/
@JvmName("xiovrglanktxfgvv")
public suspend fun buildspec(`value`: Output) {
this.buildspec = value
}
/**
* @param value Truncate git history to this many commits. Use `0` for a `Full` checkout which you need to run commands like `git branch --show-current`. See [AWS CodePipeline User Guide: Tutorial: Use full clone with a GitHub pipeline source](https://docs.aws.amazon.com/codepipeline/latest/userguide/tutorials-github-gitclone.html) for details.
*/
@JvmName("hqgdvvkhxrxrpwvf")
public suspend fun gitCloneDepth(`value`: Output) {
this.gitCloneDepth = value
}
/**
* @param value Configuration block. Detailed below.
*/
@JvmName("ttbmjohvkaoqoebk")
public suspend fun gitSubmodulesConfig(`value`: Output) {
this.gitSubmodulesConfig = value
}
/**
* @param value Ignore SSL warnings when connecting to source control.
*/
@JvmName("osryshyeimsvhnjb")
public suspend fun insecureSsl(`value`: Output) {
this.insecureSsl = value
}
/**
* @param value Location of the source code from git or s3.
*/
@JvmName("uhnpljjviwnoqqsu")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value Whether to report the status of a build's start and finish to your source provider. This option is valid only when your source provider is GitHub, GitHub Enterprise, GitLab, GitLab Self Managed, or Bitbucket.
*/
@JvmName("ackjlvuqfwfcsmmg")
public suspend fun reportBuildStatus(`value`: Output) {
this.reportBuildStatus = value
}
/**
* @param value An identifier for this project source. The identifier can only contain alphanumeric characters and underscores, and must be less than 128 characters in length.
*/
@JvmName("nfeiytodjrpqntgx")
public suspend fun sourceIdentifier(`value`: Output) {
this.sourceIdentifier = value
}
/**
* @param value Type of repository that contains the source code to be built. Valid values: `BITBUCKET`, `CODECOMMIT`, `CODEPIPELINE`, `GITHUB`, `GITHUB_ENTERPRISE`, `GITLAB`, `GITLAB_SELF_MANAGED`, `NO_SOURCE`, `S3`.
*/
@JvmName("scaanihqynnbfgbf")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value Configuration block that contains information that defines how the build project reports the build status to the source provider. This option is only used when the source provider is GitHub, GitHub Enterprise, GitLab, GitLab Self Managed, or Bitbucket. `build_status_config` blocks are documented below.
*/
@JvmName("dhcbncdnsigktemx")
public suspend fun buildStatusConfig(`value`: ProjectSecondarySourceBuildStatusConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.buildStatusConfig = mapped
}
/**
* @param argument Configuration block that contains information that defines how the build project reports the build status to the source provider. This option is only used when the source provider is GitHub, GitHub Enterprise, GitLab, GitLab Self Managed, or Bitbucket. `build_status_config` blocks are documented below.
*/
@JvmName("wsggwkxycnldhsno")
public suspend fun buildStatusConfig(argument: suspend ProjectSecondarySourceBuildStatusConfigArgsBuilder.() -> Unit) {
val toBeMapped = ProjectSecondarySourceBuildStatusConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.buildStatusConfig = mapped
}
/**
* @param value The build spec declaration to use for this build project's related builds. This must be set when `type` is `NO_SOURCE`. It can either be a path to a file residing in the repository to be built or a local file path leveraging the `file()` built-in.
*/
@JvmName("wapqgeacjtybdaum")
public suspend fun buildspec(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.buildspec = mapped
}
/**
* @param value Truncate git history to this many commits. Use `0` for a `Full` checkout which you need to run commands like `git branch --show-current`. See [AWS CodePipeline User Guide: Tutorial: Use full clone with a GitHub pipeline source](https://docs.aws.amazon.com/codepipeline/latest/userguide/tutorials-github-gitclone.html) for details.
*/
@JvmName("qrjmulqdqnumpbys")
public suspend fun gitCloneDepth(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gitCloneDepth = mapped
}
/**
* @param value Configuration block. Detailed below.
*/
@JvmName("dwctnupekkbkpqgg")
public suspend fun gitSubmodulesConfig(`value`: ProjectSecondarySourceGitSubmodulesConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gitSubmodulesConfig = mapped
}
/**
* @param argument Configuration block. Detailed below.
*/
@JvmName("ihrmtkpuucrihssx")
public suspend fun gitSubmodulesConfig(argument: suspend ProjectSecondarySourceGitSubmodulesConfigArgsBuilder.() -> Unit) {
val toBeMapped = ProjectSecondarySourceGitSubmodulesConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.gitSubmodulesConfig = mapped
}
/**
* @param value Ignore SSL warnings when connecting to source control.
*/
@JvmName("ptnxiaoklmcqwgyp")
public suspend fun insecureSsl(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.insecureSsl = mapped
}
/**
* @param value Location of the source code from git or s3.
*/
@JvmName("aylcuthsthdbxxcy")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value Whether to report the status of a build's start and finish to your source provider. This option is valid only when your source provider is GitHub, GitHub Enterprise, GitLab, GitLab Self Managed, or Bitbucket.
*/
@JvmName("xvotflwshdtwbqyx")
public suspend fun reportBuildStatus(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.reportBuildStatus = mapped
}
/**
* @param value An identifier for this project source. The identifier can only contain alphanumeric characters and underscores, and must be less than 128 characters in length.
*/
@JvmName("fnsnwmddcgcebgwg")
public suspend fun sourceIdentifier(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sourceIdentifier = mapped
}
/**
* @param value Type of repository that contains the source code to be built. Valid values: `BITBUCKET`, `CODECOMMIT`, `CODEPIPELINE`, `GITHUB`, `GITHUB_ENTERPRISE`, `GITLAB`, `GITLAB_SELF_MANAGED`, `NO_SOURCE`, `S3`.
*/
@JvmName("udduaogwccrpgpmk")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): ProjectSecondarySourceArgs = ProjectSecondarySourceArgs(
buildStatusConfig = buildStatusConfig,
buildspec = buildspec,
gitCloneDepth = gitCloneDepth,
gitSubmodulesConfig = gitSubmodulesConfig,
insecureSsl = insecureSsl,
location = location,
reportBuildStatus = reportBuildStatus,
sourceIdentifier = sourceIdentifier ?: throw PulumiNullFieldException("sourceIdentifier"),
type = type ?: throw PulumiNullFieldException("type"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy