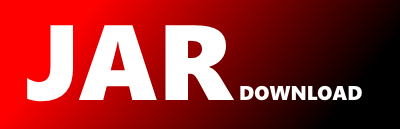
com.pulumi.aws.codecatalyst.kotlin.inputs.GetDevEnvironmentPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.codecatalyst.kotlin.inputs
import com.pulumi.aws.codecatalyst.inputs.GetDevEnvironmentPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getDevEnvironment.
* @property alias The user-specified alias for the Dev Environment.
* @property creatorId The system-generated unique ID of the user who created the Dev Environment.
* @property envId - (Required) The system-generated unique ID of the Dev Environment for which you want to view information. To retrieve a list of Dev Environment IDs, use [ListDevEnvironments](https://docs.aws.amazon.com/codecatalyst/latest/APIReference/API_ListDevEnvironments.html).
* @property projectName The name of the project in the space.
* @property repositories The source repository that contains the branch to clone into the Dev Environment.
* @property spaceName The name of the space.
* @property tags
*/
public data class GetDevEnvironmentPlainArgs(
public val alias: String? = null,
public val creatorId: String? = null,
public val envId: String,
public val projectName: String,
public val repositories: List? = null,
public val spaceName: String,
public val tags: Map? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.codecatalyst.inputs.GetDevEnvironmentPlainArgs =
com.pulumi.aws.codecatalyst.inputs.GetDevEnvironmentPlainArgs.builder()
.alias(alias?.let({ args0 -> args0 }))
.creatorId(creatorId?.let({ args0 -> args0 }))
.envId(envId.let({ args0 -> args0 }))
.projectName(projectName.let({ args0 -> args0 }))
.repositories(
repositories?.let({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.spaceName(spaceName.let({ args0 -> args0 }))
.tags(tags?.let({ args0 -> args0.map({ args0 -> args0.key.to(args0.value) }).toMap() })).build()
}
/**
* Builder for [GetDevEnvironmentPlainArgs].
*/
@PulumiTagMarker
public class GetDevEnvironmentPlainArgsBuilder internal constructor() {
private var alias: String? = null
private var creatorId: String? = null
private var envId: String? = null
private var projectName: String? = null
private var repositories: List? = null
private var spaceName: String? = null
private var tags: Map? = null
/**
* @param value The user-specified alias for the Dev Environment.
*/
@JvmName("xaprnydsbfqsnmde")
public suspend fun alias(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.alias = mapped
}
/**
* @param value The system-generated unique ID of the user who created the Dev Environment.
*/
@JvmName("dcoiiqjfdhfqptne")
public suspend fun creatorId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.creatorId = mapped
}
/**
* @param value - (Required) The system-generated unique ID of the Dev Environment for which you want to view information. To retrieve a list of Dev Environment IDs, use [ListDevEnvironments](https://docs.aws.amazon.com/codecatalyst/latest/APIReference/API_ListDevEnvironments.html).
*/
@JvmName("corerddwickquioi")
public suspend fun envId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.envId = mapped
}
/**
* @param value The name of the project in the space.
*/
@JvmName("tkyjffksbhbljhdm")
public suspend fun projectName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.projectName = mapped
}
/**
* @param value The source repository that contains the branch to clone into the Dev Environment.
*/
@JvmName("cidjgxhgmggijmbs")
public suspend fun repositories(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.repositories = mapped
}
/**
* @param argument The source repository that contains the branch to clone into the Dev Environment.
*/
@JvmName("yvyvqltwhibbmenj")
public suspend fun repositories(argument: List Unit>) {
val toBeMapped = argument.toList().map {
GetDevEnvironmentRepositoryBuilder().applySuspend {
it()
}.build()
}
val mapped = toBeMapped
this.repositories = mapped
}
/**
* @param argument The source repository that contains the branch to clone into the Dev Environment.
*/
@JvmName("uiceaokjunddjuwj")
public suspend fun repositories(vararg argument: suspend GetDevEnvironmentRepositoryBuilder.() -> Unit) {
val toBeMapped = argument.toList().map {
GetDevEnvironmentRepositoryBuilder().applySuspend {
it()
}.build()
}
val mapped = toBeMapped
this.repositories = mapped
}
/**
* @param argument The source repository that contains the branch to clone into the Dev Environment.
*/
@JvmName("gvhsmnkfklelaiap")
public suspend fun repositories(argument: suspend GetDevEnvironmentRepositoryBuilder.() -> Unit) {
val toBeMapped = listOf(
GetDevEnvironmentRepositoryBuilder().applySuspend {
argument()
}.build(),
)
val mapped = toBeMapped
this.repositories = mapped
}
/**
* @param values The source repository that contains the branch to clone into the Dev Environment.
*/
@JvmName("scuxmghigbyilavs")
public suspend fun repositories(vararg values: GetDevEnvironmentRepository) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.repositories = mapped
}
/**
* @param value The name of the space.
*/
@JvmName("mphgqyckaixsdqvb")
public suspend fun spaceName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.spaceName = mapped
}
/**
* @param value
*/
@JvmName("ephhwwjihyqujtho")
public suspend fun tags(`value`: Map?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tags = mapped
}
/**
* @param values
*/
@JvmName("tdutkfgcuubsvkym")
public fun tags(vararg values: Pair) {
val toBeMapped = values.toMap()
val mapped = toBeMapped.let({ args0 -> args0 })
this.tags = mapped
}
internal fun build(): GetDevEnvironmentPlainArgs = GetDevEnvironmentPlainArgs(
alias = alias,
creatorId = creatorId,
envId = envId ?: throw PulumiNullFieldException("envId"),
projectName = projectName ?: throw PulumiNullFieldException("projectName"),
repositories = repositories,
spaceName = spaceName ?: throw PulumiNullFieldException("spaceName"),
tags = tags,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy