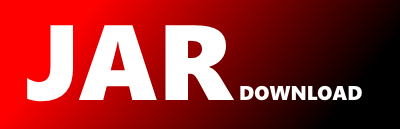
com.pulumi.aws.codedeploy.kotlin.inputs.DeploymentGroupLoadBalancerInfoArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.codedeploy.kotlin.inputs
import com.pulumi.aws.codedeploy.inputs.DeploymentGroupLoadBalancerInfoArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property elbInfos The Classic Elastic Load Balancer to use in a deployment. Conflicts with `target_group_info` and `target_group_pair_info`.
* @property targetGroupInfos The (Application/Network Load Balancer) target group to use in a deployment. Conflicts with `elb_info` and `target_group_pair_info`.
* @property targetGroupPairInfo The (Application/Network Load Balancer) target group pair to use in a deployment. Conflicts with `elb_info` and `target_group_info`.
*/
public data class DeploymentGroupLoadBalancerInfoArgs(
public val elbInfos: Output>? = null,
public val targetGroupInfos: Output>? =
null,
public val targetGroupPairInfo: Output? =
null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.codedeploy.inputs.DeploymentGroupLoadBalancerInfoArgs =
com.pulumi.aws.codedeploy.inputs.DeploymentGroupLoadBalancerInfoArgs.builder()
.elbInfos(
elbInfos?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.targetGroupInfos(
targetGroupInfos?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.targetGroupPairInfo(
targetGroupPairInfo?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [DeploymentGroupLoadBalancerInfoArgs].
*/
@PulumiTagMarker
public class DeploymentGroupLoadBalancerInfoArgsBuilder internal constructor() {
private var elbInfos: Output>? = null
private var targetGroupInfos: Output>? =
null
private var targetGroupPairInfo: Output? =
null
/**
* @param value The Classic Elastic Load Balancer to use in a deployment. Conflicts with `target_group_info` and `target_group_pair_info`.
*/
@JvmName("ttvvwseedablahwl")
public suspend fun elbInfos(`value`: Output>) {
this.elbInfos = value
}
@JvmName("hjwpdmjsraekqpep")
public suspend fun elbInfos(vararg values: Output) {
this.elbInfos = Output.all(values.asList())
}
/**
* @param values The Classic Elastic Load Balancer to use in a deployment. Conflicts with `target_group_info` and `target_group_pair_info`.
*/
@JvmName("aupkdgfebfttdjig")
public suspend fun elbInfos(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy