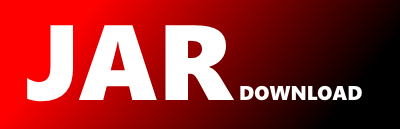
com.pulumi.aws.codepipeline.kotlin.inputs.PipelineTriggerGitConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.codepipeline.kotlin.inputs
import com.pulumi.aws.codepipeline.inputs.PipelineTriggerGitConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property pullRequests The field where the repository event that will start the pipeline is specified as pull requests. A `pull_request` block is documented below.
* @property pushes The field where the repository event that will start the pipeline, such as pushing Git tags, is specified with details. A `push` block is documented below.
* @property sourceActionName The name of the pipeline source action where the trigger configuration, such as Git tags, is specified. The trigger configuration will start the pipeline upon the specified change only.
*/
public data class PipelineTriggerGitConfigurationArgs(
public val pullRequests: Output>? = null,
public val pushes: Output>? = null,
public val sourceActionName: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.codepipeline.inputs.PipelineTriggerGitConfigurationArgs =
com.pulumi.aws.codepipeline.inputs.PipelineTriggerGitConfigurationArgs.builder()
.pullRequests(
pullRequests?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.pushes(pushes?.applyValue({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.sourceActionName(sourceActionName.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PipelineTriggerGitConfigurationArgs].
*/
@PulumiTagMarker
public class PipelineTriggerGitConfigurationArgsBuilder internal constructor() {
private var pullRequests: Output>? = null
private var pushes: Output>? = null
private var sourceActionName: Output? = null
/**
* @param value The field where the repository event that will start the pipeline is specified as pull requests. A `pull_request` block is documented below.
*/
@JvmName("hvxywnloifmkonyv")
public suspend fun pullRequests(`value`: Output>) {
this.pullRequests = value
}
@JvmName("eunbcgsivpajlfpa")
public suspend fun pullRequests(vararg values: Output) {
this.pullRequests = Output.all(values.asList())
}
/**
* @param values The field where the repository event that will start the pipeline is specified as pull requests. A `pull_request` block is documented below.
*/
@JvmName("wkpkfeyvrlteaaqs")
public suspend fun pullRequests(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy