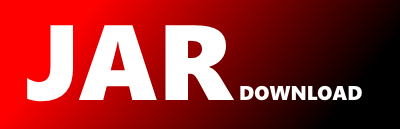
com.pulumi.aws.codepipeline.kotlin.inputs.PipelineTriggerGitConfigurationPullRequestArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.codepipeline.kotlin.inputs
import com.pulumi.aws.codepipeline.inputs.PipelineTriggerGitConfigurationPullRequestArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property branches The field that specifies to filter on branches for the pull request trigger configuration. A `branches` block is documented below.
* @property events A list that specifies which pull request events to filter on (opened, updated, closed) for the trigger configuration. Possible values are `OPEN`, `UPDATED ` and `CLOSED`.
* @property filePaths The field that specifies to filter on file paths for the pull request trigger configuration. A `file_paths` block is documented below.
*/
public data class PipelineTriggerGitConfigurationPullRequestArgs(
public val branches: Output? = null,
public val events: Output>? = null,
public val filePaths: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.codepipeline.inputs.PipelineTriggerGitConfigurationPullRequestArgs =
com.pulumi.aws.codepipeline.inputs.PipelineTriggerGitConfigurationPullRequestArgs.builder()
.branches(branches?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.events(events?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.filePaths(filePaths?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [PipelineTriggerGitConfigurationPullRequestArgs].
*/
@PulumiTagMarker
public class PipelineTriggerGitConfigurationPullRequestArgsBuilder internal constructor() {
private var branches: Output? = null
private var events: Output>? = null
private var filePaths: Output? = null
/**
* @param value The field that specifies to filter on branches for the pull request trigger configuration. A `branches` block is documented below.
*/
@JvmName("jkvhwfxalvpqhkok")
public suspend fun branches(`value`: Output) {
this.branches = value
}
/**
* @param value A list that specifies which pull request events to filter on (opened, updated, closed) for the trigger configuration. Possible values are `OPEN`, `UPDATED ` and `CLOSED`.
*/
@JvmName("rjtgitggmbqwvdfj")
public suspend fun events(`value`: Output>) {
this.events = value
}
@JvmName("opeoeyvcwpuvtmkj")
public suspend fun events(vararg values: Output) {
this.events = Output.all(values.asList())
}
/**
* @param values A list that specifies which pull request events to filter on (opened, updated, closed) for the trigger configuration. Possible values are `OPEN`, `UPDATED ` and `CLOSED`.
*/
@JvmName("cbwokohefpwygmbf")
public suspend fun events(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy