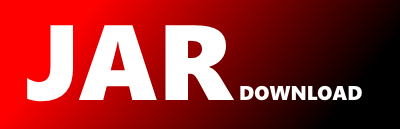
com.pulumi.aws.cognito.kotlin.ManagedUserPoolClientArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cognito.kotlin
import com.pulumi.aws.cognito.ManagedUserPoolClientArgs.builder
import com.pulumi.aws.cognito.kotlin.inputs.ManagedUserPoolClientAnalyticsConfigurationArgs
import com.pulumi.aws.cognito.kotlin.inputs.ManagedUserPoolClientAnalyticsConfigurationArgsBuilder
import com.pulumi.aws.cognito.kotlin.inputs.ManagedUserPoolClientTokenValidityUnitsArgs
import com.pulumi.aws.cognito.kotlin.inputs.ManagedUserPoolClientTokenValidityUnitsArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Use the `aws.cognito.UserPoolClient` resource to manage a Cognito User Pool Client.
* **This resource is advanced** and has special caveats to consider before use. Please read this document completely before using the resource.
* Use the `aws.cognito.ManagedUserPoolClient` resource to manage a Cognito User Pool Client that is automatically created by an AWS service. For instance, when [configuring an OpenSearch Domain to use Cognito authentication](https://docs.aws.amazon.com/opensearch-service/latest/developerguide/cognito-auth.html), the OpenSearch service creates the User Pool Client during setup and removes it when it is no longer required. As a result, the `aws.cognito.ManagedUserPoolClient` resource does not create or delete this resource, but instead assumes management of it.
* Use the `aws.cognito.UserPoolClient` resource to manage Cognito User Pool Clients for normal use cases.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const exampleUserPool = new aws.cognito.UserPool("example", {name: "example"});
* const exampleIdentityPool = new aws.cognito.IdentityPool("example", {identityPoolName: "example"});
* const current = aws.getPartition({});
* const example = current.then(current => aws.iam.getPolicyDocument({
* statements: [{
* sid: "",
* actions: ["sts:AssumeRole"],
* effect: "Allow",
* principals: [{
* type: "Service",
* identifiers: [`es.${current.dnsSuffix}`],
* }],
* }],
* }));
* const exampleRole = new aws.iam.Role("example", {
* name: "example-role",
* path: "/service-role/",
* assumeRolePolicy: example.then(example => example.json),
* });
* const exampleRolePolicyAttachment = new aws.iam.RolePolicyAttachment("example", {
* role: exampleRole.name,
* policyArn: current.then(current => `arn:${current.partition}:iam::aws:policy/AmazonESCognitoAccess`),
* });
* const exampleDomain = new aws.opensearch.Domain("example", {
* domainName: "example",
* cognitoOptions: {
* enabled: true,
* userPoolId: exampleUserPool.id,
* identityPoolId: exampleIdentityPool.id,
* roleArn: exampleRole.arn,
* },
* ebsOptions: {
* ebsEnabled: true,
* volumeSize: 10,
* },
* }, {
* dependsOn: [
* exampleAwsCognitoUserPoolDomain,
* exampleRolePolicyAttachment,
* ],
* });
* const exampleManagedUserPoolClient = new aws.cognito.ManagedUserPoolClient("example", {
* namePrefix: "AmazonOpenSearchService-example",
* userPoolId: exampleUserPool.id,
* }, {
* dependsOn: [exampleDomain],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example_user_pool = aws.cognito.UserPool("example", name="example")
* example_identity_pool = aws.cognito.IdentityPool("example", identity_pool_name="example")
* current = aws.get_partition()
* example = aws.iam.get_policy_document(statements=[{
* "sid": "",
* "actions": ["sts:AssumeRole"],
* "effect": "Allow",
* "principals": [{
* "type": "Service",
* "identifiers": [f"es.{current.dns_suffix}"],
* }],
* }])
* example_role = aws.iam.Role("example",
* name="example-role",
* path="/service-role/",
* assume_role_policy=example.json)
* example_role_policy_attachment = aws.iam.RolePolicyAttachment("example",
* role=example_role.name,
* policy_arn=f"arn:{current.partition}:iam::aws:policy/AmazonESCognitoAccess")
* example_domain = aws.opensearch.Domain("example",
* domain_name="example",
* cognito_options={
* "enabled": True,
* "user_pool_id": example_user_pool.id,
* "identity_pool_id": example_identity_pool.id,
* "role_arn": example_role.arn,
* },
* ebs_options={
* "ebs_enabled": True,
* "volume_size": 10,
* },
* opts = pulumi.ResourceOptions(depends_on=[
* example_aws_cognito_user_pool_domain,
* example_role_policy_attachment,
* ]))
* example_managed_user_pool_client = aws.cognito.ManagedUserPoolClient("example",
* name_prefix="AmazonOpenSearchService-example",
* user_pool_id=example_user_pool.id,
* opts = pulumi.ResourceOptions(depends_on=[example_domain]))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var exampleUserPool = new Aws.Cognito.UserPool("example", new()
* {
* Name = "example",
* });
* var exampleIdentityPool = new Aws.Cognito.IdentityPool("example", new()
* {
* IdentityPoolName = "example",
* });
* var current = Aws.GetPartition.Invoke();
* var example = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Sid = "",
* Actions = new[]
* {
* "sts:AssumeRole",
* },
* Effect = "Allow",
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "Service",
* Identifiers = new[]
* {
* $"es.{current.Apply(getPartitionResult => getPartitionResult.DnsSuffix)}",
* },
* },
* },
* },
* },
* });
* var exampleRole = new Aws.Iam.Role("example", new()
* {
* Name = "example-role",
* Path = "/service-role/",
* AssumeRolePolicy = example.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* var exampleRolePolicyAttachment = new Aws.Iam.RolePolicyAttachment("example", new()
* {
* Role = exampleRole.Name,
* PolicyArn = $"arn:{current.Apply(getPartitionResult => getPartitionResult.Partition)}:iam::aws:policy/AmazonESCognitoAccess",
* });
* var exampleDomain = new Aws.OpenSearch.Domain("example", new()
* {
* DomainName = "example",
* CognitoOptions = new Aws.OpenSearch.Inputs.DomainCognitoOptionsArgs
* {
* Enabled = true,
* UserPoolId = exampleUserPool.Id,
* IdentityPoolId = exampleIdentityPool.Id,
* RoleArn = exampleRole.Arn,
* },
* EbsOptions = new Aws.OpenSearch.Inputs.DomainEbsOptionsArgs
* {
* EbsEnabled = true,
* VolumeSize = 10,
* },
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* exampleAwsCognitoUserPoolDomain,
* exampleRolePolicyAttachment,
* },
* });
* var exampleManagedUserPoolClient = new Aws.Cognito.ManagedUserPoolClient("example", new()
* {
* NamePrefix = "AmazonOpenSearchService-example",
* UserPoolId = exampleUserPool.Id,
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* exampleDomain,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cognito"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/opensearch"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* exampleUserPool, err := cognito.NewUserPool(ctx, "example", &cognito.UserPoolArgs{
* Name: pulumi.String("example"),
* })
* if err != nil {
* return err
* }
* exampleIdentityPool, err := cognito.NewIdentityPool(ctx, "example", &cognito.IdentityPoolArgs{
* IdentityPoolName: pulumi.String("example"),
* })
* if err != nil {
* return err
* }
* current, err := aws.GetPartition(ctx, &aws.GetPartitionArgs{}, nil)
* if err != nil {
* return err
* }
* example, err := iam.GetPolicyDocument(ctx, &iam.GetPolicyDocumentArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Sid: pulumi.StringRef(""),
* Actions: []string{
* "sts:AssumeRole",
* },
* Effect: pulumi.StringRef("Allow"),
* Principals: []iam.GetPolicyDocumentStatementPrincipal{
* {
* Type: "Service",
* Identifiers: []string{
* fmt.Sprintf("es.%v", current.DnsSuffix),
* },
* },
* },
* },
* },
* }, nil)
* if err != nil {
* return err
* }
* exampleRole, err := iam.NewRole(ctx, "example", &iam.RoleArgs{
* Name: pulumi.String("example-role"),
* Path: pulumi.String("/service-role/"),
* AssumeRolePolicy: pulumi.String(example.Json),
* })
* if err != nil {
* return err
* }
* exampleRolePolicyAttachment, err := iam.NewRolePolicyAttachment(ctx, "example", &iam.RolePolicyAttachmentArgs{
* Role: exampleRole.Name,
* PolicyArn: pulumi.Sprintf("arn:%v:iam::aws:policy/AmazonESCognitoAccess", current.Partition),
* })
* if err != nil {
* return err
* }
* exampleDomain, err := opensearch.NewDomain(ctx, "example", &opensearch.DomainArgs{
* DomainName: pulumi.String("example"),
* CognitoOptions: &opensearch.DomainCognitoOptionsArgs{
* Enabled: pulumi.Bool(true),
* UserPoolId: exampleUserPool.ID(),
* IdentityPoolId: exampleIdentityPool.ID(),
* RoleArn: exampleRole.Arn,
* },
* EbsOptions: &opensearch.DomainEbsOptionsArgs{
* EbsEnabled: pulumi.Bool(true),
* VolumeSize: pulumi.Int(10),
* },
* }, pulumi.DependsOn([]pulumi.Resource{
* exampleAwsCognitoUserPoolDomain,
* exampleRolePolicyAttachment,
* }))
* if err != nil {
* return err
* }
* _, err = cognito.NewManagedUserPoolClient(ctx, "example", &cognito.ManagedUserPoolClientArgs{
* NamePrefix: pulumi.String("AmazonOpenSearchService-example"),
* UserPoolId: exampleUserPool.ID(),
* }, pulumi.DependsOn([]pulumi.Resource{
* exampleDomain,
* }))
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cognito.UserPool;
* import com.pulumi.aws.cognito.UserPoolArgs;
* import com.pulumi.aws.cognito.IdentityPool;
* import com.pulumi.aws.cognito.IdentityPoolArgs;
* import com.pulumi.aws.AwsFunctions;
* import com.pulumi.aws.inputs.GetPartitionArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.iam.RolePolicyAttachment;
* import com.pulumi.aws.iam.RolePolicyAttachmentArgs;
* import com.pulumi.aws.opensearch.Domain;
* import com.pulumi.aws.opensearch.DomainArgs;
* import com.pulumi.aws.opensearch.inputs.DomainCognitoOptionsArgs;
* import com.pulumi.aws.opensearch.inputs.DomainEbsOptionsArgs;
* import com.pulumi.aws.cognito.ManagedUserPoolClient;
* import com.pulumi.aws.cognito.ManagedUserPoolClientArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var exampleUserPool = new UserPool("exampleUserPool", UserPoolArgs.builder()
* .name("example")
* .build());
* var exampleIdentityPool = new IdentityPool("exampleIdentityPool", IdentityPoolArgs.builder()
* .identityPoolName("example")
* .build());
* final var current = AwsFunctions.getPartition();
* final var example = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .sid("")
* .actions("sts:AssumeRole")
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers(String.format("es.%s", current.applyValue(getPartitionResult -> getPartitionResult.dnsSuffix())))
* .build())
* .build())
* .build());
* var exampleRole = new Role("exampleRole", RoleArgs.builder()
* .name("example-role")
* .path("/service-role/")
* .assumeRolePolicy(example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* var exampleRolePolicyAttachment = new RolePolicyAttachment("exampleRolePolicyAttachment", RolePolicyAttachmentArgs.builder()
* .role(exampleRole.name())
* .policyArn(String.format("arn:%s:iam::aws:policy/AmazonESCognitoAccess", current.applyValue(getPartitionResult -> getPartitionResult.partition())))
* .build());
* var exampleDomain = new Domain("exampleDomain", DomainArgs.builder()
* .domainName("example")
* .cognitoOptions(DomainCognitoOptionsArgs.builder()
* .enabled(true)
* .userPoolId(exampleUserPool.id())
* .identityPoolId(exampleIdentityPool.id())
* .roleArn(exampleRole.arn())
* .build())
* .ebsOptions(DomainEbsOptionsArgs.builder()
* .ebsEnabled(true)
* .volumeSize(10)
* .build())
* .build(), CustomResourceOptions.builder()
* .dependsOn(
* exampleAwsCognitoUserPoolDomain,
* exampleRolePolicyAttachment)
* .build());
* var exampleManagedUserPoolClient = new ManagedUserPoolClient("exampleManagedUserPoolClient", ManagedUserPoolClientArgs.builder()
* .namePrefix("AmazonOpenSearchService-example")
* .userPoolId(exampleUserPool.id())
* .build(), CustomResourceOptions.builder()
* .dependsOn(exampleDomain)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleManagedUserPoolClient:
* type: aws:cognito:ManagedUserPoolClient
* name: example
* properties:
* namePrefix: AmazonOpenSearchService-example
* userPoolId: ${exampleUserPool.id}
* options:
* dependson:
* - ${exampleDomain}
* exampleUserPool:
* type: aws:cognito:UserPool
* name: example
* properties:
* name: example
* exampleIdentityPool:
* type: aws:cognito:IdentityPool
* name: example
* properties:
* identityPoolName: example
* exampleDomain:
* type: aws:opensearch:Domain
* name: example
* properties:
* domainName: example
* cognitoOptions:
* enabled: true
* userPoolId: ${exampleUserPool.id}
* identityPoolId: ${exampleIdentityPool.id}
* roleArn: ${exampleRole.arn}
* ebsOptions:
* ebsEnabled: true
* volumeSize: 10
* options:
* dependson:
* - ${exampleAwsCognitoUserPoolDomain}
* - ${exampleRolePolicyAttachment}
* exampleRole:
* type: aws:iam:Role
* name: example
* properties:
* name: example-role
* path: /service-role/
* assumeRolePolicy: ${example.json}
* exampleRolePolicyAttachment:
* type: aws:iam:RolePolicyAttachment
* name: example
* properties:
* role: ${exampleRole.name}
* policyArn: arn:${current.partition}:iam::aws:policy/AmazonESCognitoAccess
* variables:
* example:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - sid:
* actions:
* - sts:AssumeRole
* effect: Allow
* principals:
* - type: Service
* identifiers:
* - es.${current.dnsSuffix}
* current:
* fn::invoke:
* Function: aws:getPartition
* Arguments: {}
* ```
*
* ## Import
* Using `pulumi import`, import Cognito User Pool Clients using the `id` of the Cognito User Pool and the `id` of the Cognito User Pool Client. For example:
* ```sh
* $ pulumi import aws:cognito/managedUserPoolClient:ManagedUserPoolClient client us-west-2_abc123/3ho4ek12345678909nh3fmhpko
* ```
* @property accessTokenValidity Time limit, between 5 minutes and 1 day, after which the access token is no longer valid and cannot be used. By default, the unit is hours. The unit can be overridden by a value in `token_validity_units.access_token`.
* @property allowedOauthFlows List of allowed OAuth flows, including `code`, `implicit`, and `client_credentials`. `allowed_oauth_flows_user_pool_client` must be set to `true` before you can configure this option.
* @property allowedOauthFlowsUserPoolClient Whether the client is allowed to use OAuth 2.0 features. `allowed_oauth_flows_user_pool_client` must be set to `true` before you can configure the following arguments: `callback_urls`, `logout_urls`, `allowed_oauth_scopes` and `allowed_oauth_flows`.
* @property allowedOauthScopes List of allowed OAuth scopes, including `phone`, `email`, `openid`, `profile`, and `aws.cognito.signin.user.admin`. `allowed_oauth_flows_user_pool_client` must be set to `true` before you can configure this option.
* @property analyticsConfiguration Configuration block for Amazon Pinpoint analytics that collects metrics for this user pool. See details below.
* @property authSessionValidity Duration, in minutes, of the session token created by Amazon Cognito for each API request in an authentication flow. The session token must be responded to by the native user of the user pool before it expires. Valid values for `auth_session_validity` are between `3` and `15`, with a default value of `3`.
* @property callbackUrls List of allowed callback URLs for the identity providers. `allowed_oauth_flows_user_pool_client` must be set to `true` before you can configure this option.
* @property defaultRedirectUri Default redirect URI and must be included in the list of callback URLs.
* @property enablePropagateAdditionalUserContextData Enables the propagation of additional user context data.
* @property enableTokenRevocation Enables or disables token revocation.
* @property explicitAuthFlows List of authentication flows. The available options include ADMIN_NO_SRP_AUTH, CUSTOM_AUTH_FLOW_ONLY, USER_PASSWORD_AUTH, ALLOW_ADMIN_USER_PASSWORD_AUTH, ALLOW_CUSTOM_AUTH, ALLOW_USER_PASSWORD_AUTH, ALLOW_USER_SRP_AUTH, and ALLOW_REFRESH_TOKEN_AUTH.
* @property idTokenValidity Time limit, between 5 minutes and 1 day, after which the ID token is no longer valid and cannot be used. By default, the unit is hours. The unit can be overridden by a value in `token_validity_units.id_token`.
* @property logoutUrls List of allowed logout URLs for the identity providers. `allowed_oauth_flows_user_pool_client` must be set to `true` before you can configure this option.
* @property namePattern Regular expression that matches the name of the desired User Pool Client. It must only match one User Pool Client.
* @property namePrefix String that matches the beginning of the name of the desired User Pool Client. It must match only one User Pool Client.
* The following arguments are optional:
* @property preventUserExistenceErrors Setting determines the errors and responses returned by Cognito APIs when a user does not exist in the user pool during authentication, account confirmation, and password recovery.
* @property readAttributes List of user pool attributes that the application client can read from.
* @property refreshTokenValidity Time limit, between 60 minutes and 10 years, after which the refresh token is no longer valid and cannot be used. By default, the unit is days. The unit can be overridden by a value in `token_validity_units.refresh_token`.
* @property supportedIdentityProviders List of provider names for the identity providers that are supported on this client. It uses the `provider_name` attribute of the `aws.cognito.IdentityProvider` resource(s), or the equivalent string(s).
* @property tokenValidityUnits Configuration block for representing the validity times in units. See details below. Detailed below.
* @property userPoolId User pool that the client belongs to.
* @property writeAttributes List of user pool attributes that the application client can write to.
*/
public data class ManagedUserPoolClientArgs(
public val accessTokenValidity: Output? = null,
public val allowedOauthFlows: Output>? = null,
public val allowedOauthFlowsUserPoolClient: Output? = null,
public val allowedOauthScopes: Output>? = null,
public val analyticsConfiguration: Output? =
null,
public val authSessionValidity: Output? = null,
public val callbackUrls: Output>? = null,
public val defaultRedirectUri: Output? = null,
public val enablePropagateAdditionalUserContextData: Output? = null,
public val enableTokenRevocation: Output? = null,
public val explicitAuthFlows: Output>? = null,
public val idTokenValidity: Output? = null,
public val logoutUrls: Output>? = null,
public val namePattern: Output? = null,
public val namePrefix: Output? = null,
public val preventUserExistenceErrors: Output? = null,
public val readAttributes: Output>? = null,
public val refreshTokenValidity: Output? = null,
public val supportedIdentityProviders: Output>? = null,
public val tokenValidityUnits: Output? = null,
public val userPoolId: Output? = null,
public val writeAttributes: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.cognito.ManagedUserPoolClientArgs =
com.pulumi.aws.cognito.ManagedUserPoolClientArgs.builder()
.accessTokenValidity(accessTokenValidity?.applyValue({ args0 -> args0 }))
.allowedOauthFlows(allowedOauthFlows?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.allowedOauthFlowsUserPoolClient(allowedOauthFlowsUserPoolClient?.applyValue({ args0 -> args0 }))
.allowedOauthScopes(allowedOauthScopes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.analyticsConfiguration(
analyticsConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.authSessionValidity(authSessionValidity?.applyValue({ args0 -> args0 }))
.callbackUrls(callbackUrls?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.defaultRedirectUri(defaultRedirectUri?.applyValue({ args0 -> args0 }))
.enablePropagateAdditionalUserContextData(
enablePropagateAdditionalUserContextData?.applyValue({ args0 ->
args0
}),
)
.enableTokenRevocation(enableTokenRevocation?.applyValue({ args0 -> args0 }))
.explicitAuthFlows(explicitAuthFlows?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.idTokenValidity(idTokenValidity?.applyValue({ args0 -> args0 }))
.logoutUrls(logoutUrls?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.namePattern(namePattern?.applyValue({ args0 -> args0 }))
.namePrefix(namePrefix?.applyValue({ args0 -> args0 }))
.preventUserExistenceErrors(preventUserExistenceErrors?.applyValue({ args0 -> args0 }))
.readAttributes(readAttributes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.refreshTokenValidity(refreshTokenValidity?.applyValue({ args0 -> args0 }))
.supportedIdentityProviders(
supportedIdentityProviders?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
)
.tokenValidityUnits(
tokenValidityUnits?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.userPoolId(userPoolId?.applyValue({ args0 -> args0 }))
.writeAttributes(writeAttributes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [ManagedUserPoolClientArgs].
*/
@PulumiTagMarker
public class ManagedUserPoolClientArgsBuilder internal constructor() {
private var accessTokenValidity: Output? = null
private var allowedOauthFlows: Output>? = null
private var allowedOauthFlowsUserPoolClient: Output? = null
private var allowedOauthScopes: Output>? = null
private var analyticsConfiguration: Output? =
null
private var authSessionValidity: Output? = null
private var callbackUrls: Output>? = null
private var defaultRedirectUri: Output? = null
private var enablePropagateAdditionalUserContextData: Output? = null
private var enableTokenRevocation: Output? = null
private var explicitAuthFlows: Output>? = null
private var idTokenValidity: Output? = null
private var logoutUrls: Output>? = null
private var namePattern: Output? = null
private var namePrefix: Output? = null
private var preventUserExistenceErrors: Output? = null
private var readAttributes: Output>? = null
private var refreshTokenValidity: Output? = null
private var supportedIdentityProviders: Output>? = null
private var tokenValidityUnits: Output? = null
private var userPoolId: Output? = null
private var writeAttributes: Output>? = null
/**
* @param value Time limit, between 5 minutes and 1 day, after which the access token is no longer valid and cannot be used. By default, the unit is hours. The unit can be overridden by a value in `token_validity_units.access_token`.
*/
@JvmName("nrybqgjbsurjwxho")
public suspend fun accessTokenValidity(`value`: Output) {
this.accessTokenValidity = value
}
/**
* @param value List of allowed OAuth flows, including `code`, `implicit`, and `client_credentials`. `allowed_oauth_flows_user_pool_client` must be set to `true` before you can configure this option.
*/
@JvmName("vifmtgjaalxgwmcd")
public suspend fun allowedOauthFlows(`value`: Output>) {
this.allowedOauthFlows = value
}
@JvmName("lhupjfuosgkljcrj")
public suspend fun allowedOauthFlows(vararg values: Output) {
this.allowedOauthFlows = Output.all(values.asList())
}
/**
* @param values List of allowed OAuth flows, including `code`, `implicit`, and `client_credentials`. `allowed_oauth_flows_user_pool_client` must be set to `true` before you can configure this option.
*/
@JvmName("gkltqqgaphhqmndn")
public suspend fun allowedOauthFlows(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy