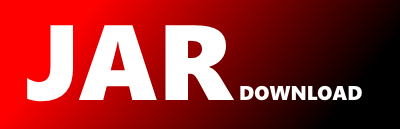
com.pulumi.aws.cognito.kotlin.User.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cognito.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
/**
* Builder for [User].
*/
@PulumiTagMarker
public class UserResourceBuilder internal constructor() {
public var name: String? = null
public var args: UserArgs = UserArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend UserArgsBuilder.() -> Unit) {
val builder = UserArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): User {
val builtJavaResource = com.pulumi.aws.cognito.User(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return User(builtJavaResource)
}
}
/**
* Provides a Cognito User Resource.
* ## Example Usage
* ### Basic configuration
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.cognito.UserPool("example", {name: "MyExamplePool"});
* const exampleUser = new aws.cognito.User("example", {
* userPoolId: example.id,
* username: "example",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.cognito.UserPool("example", name="MyExamplePool")
* example_user = aws.cognito.User("example",
* user_pool_id=example.id,
* username="example")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Cognito.UserPool("example", new()
* {
* Name = "MyExamplePool",
* });
* var exampleUser = new Aws.Cognito.User("example", new()
* {
* UserPoolId = example.Id,
* Username = "example",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cognito"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := cognito.NewUserPool(ctx, "example", &cognito.UserPoolArgs{
* Name: pulumi.String("MyExamplePool"),
* })
* if err != nil {
* return err
* }
* _, err = cognito.NewUser(ctx, "example", &cognito.UserArgs{
* UserPoolId: example.ID(),
* Username: pulumi.String("example"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cognito.UserPool;
* import com.pulumi.aws.cognito.UserPoolArgs;
* import com.pulumi.aws.cognito.User;
* import com.pulumi.aws.cognito.UserArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new UserPool("example", UserPoolArgs.builder()
* .name("MyExamplePool")
* .build());
* var exampleUser = new User("exampleUser", UserArgs.builder()
* .userPoolId(example.id())
* .username("example")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:cognito:UserPool
* properties:
* name: MyExamplePool
* exampleUser:
* type: aws:cognito:User
* name: example
* properties:
* userPoolId: ${example.id}
* username: example
* ```
*
* ### Setting user attributes
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.cognito.UserPool("example", {
* name: "mypool",
* schemas: [
* {
* name: "example",
* attributeDataType: "Boolean",
* mutable: false,
* required: false,
* developerOnlyAttribute: false,
* },
* {
* name: "foo",
* attributeDataType: "String",
* mutable: false,
* required: false,
* developerOnlyAttribute: false,
* stringAttributeConstraints: {},
* },
* ],
* });
* const exampleUser = new aws.cognito.User("example", {
* userPoolId: example.id,
* username: "example",
* attributes: {
* example: "true",
* foo: "bar",
* email: "[email protected]",
* email_verified: "true",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.cognito.UserPool("example",
* name="mypool",
* schemas=[
* {
* "name": "example",
* "attribute_data_type": "Boolean",
* "mutable": False,
* "required": False,
* "developer_only_attribute": False,
* },
* {
* "name": "foo",
* "attribute_data_type": "String",
* "mutable": False,
* "required": False,
* "developer_only_attribute": False,
* "string_attribute_constraints": {},
* },
* ])
* example_user = aws.cognito.User("example",
* user_pool_id=example.id,
* username="example",
* attributes={
* "example": "true",
* "foo": "bar",
* "email": "[email protected]",
* "email_verified": "true",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Cognito.UserPool("example", new()
* {
* Name = "mypool",
* Schemas = new[]
* {
* new Aws.Cognito.Inputs.UserPoolSchemaArgs
* {
* Name = "example",
* AttributeDataType = "Boolean",
* Mutable = false,
* Required = false,
* DeveloperOnlyAttribute = false,
* },
* new Aws.Cognito.Inputs.UserPoolSchemaArgs
* {
* Name = "foo",
* AttributeDataType = "String",
* Mutable = false,
* Required = false,
* DeveloperOnlyAttribute = false,
* StringAttributeConstraints = null,
* },
* },
* });
* var exampleUser = new Aws.Cognito.User("example", new()
* {
* UserPoolId = example.Id,
* Username = "example",
* Attributes =
* {
* { "example", "true" },
* { "foo", "bar" },
* { "email", "[email protected]" },
* { "email_verified", "true" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cognito"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := cognito.NewUserPool(ctx, "example", &cognito.UserPoolArgs{
* Name: pulumi.String("mypool"),
* Schemas: cognito.UserPoolSchemaArray{
* &cognito.UserPoolSchemaArgs{
* Name: pulumi.String("example"),
* AttributeDataType: pulumi.String("Boolean"),
* Mutable: pulumi.Bool(false),
* Required: pulumi.Bool(false),
* DeveloperOnlyAttribute: pulumi.Bool(false),
* },
* &cognito.UserPoolSchemaArgs{
* Name: pulumi.String("foo"),
* AttributeDataType: pulumi.String("String"),
* Mutable: pulumi.Bool(false),
* Required: pulumi.Bool(false),
* DeveloperOnlyAttribute: pulumi.Bool(false),
* StringAttributeConstraints: &cognito.UserPoolSchemaStringAttributeConstraintsArgs{},
* },
* },
* })
* if err != nil {
* return err
* }
* _, err = cognito.NewUser(ctx, "example", &cognito.UserArgs{
* UserPoolId: example.ID(),
* Username: pulumi.String("example"),
* Attributes: pulumi.StringMap{
* "example": pulumi.String("true"),
* "foo": pulumi.String("bar"),
* "email": pulumi.String("[email protected]"),
* "email_verified": pulumi.String("true"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cognito.UserPool;
* import com.pulumi.aws.cognito.UserPoolArgs;
* import com.pulumi.aws.cognito.inputs.UserPoolSchemaArgs;
* import com.pulumi.aws.cognito.inputs.UserPoolSchemaStringAttributeConstraintsArgs;
* import com.pulumi.aws.cognito.User;
* import com.pulumi.aws.cognito.UserArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new UserPool("example", UserPoolArgs.builder()
* .name("mypool")
* .schemas(
* UserPoolSchemaArgs.builder()
* .name("example")
* .attributeDataType("Boolean")
* .mutable(false)
* .required(false)
* .developerOnlyAttribute(false)
* .build(),
* UserPoolSchemaArgs.builder()
* .name("foo")
* .attributeDataType("String")
* .mutable(false)
* .required(false)
* .developerOnlyAttribute(false)
* .stringAttributeConstraints()
* .build())
* .build());
* var exampleUser = new User("exampleUser", UserArgs.builder()
* .userPoolId(example.id())
* .username("example")
* .attributes(Map.ofEntries(
* Map.entry("example", true),
* Map.entry("foo", "bar"),
* Map.entry("email", "[email protected]"),
* Map.entry("email_verified", true)
* ))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:cognito:UserPool
* properties:
* name: mypool
* schemas:
* - name: example
* attributeDataType: Boolean
* mutable: false
* required: false
* developerOnlyAttribute: false
* - name: foo
* attributeDataType: String
* mutable: false
* required: false
* developerOnlyAttribute: false
* stringAttributeConstraints: {}
* exampleUser:
* type: aws:cognito:User
* name: example
* properties:
* userPoolId: ${example.id}
* username: example
* attributes:
* example: true
* foo: bar
* email: [email protected]
* email_verified: true
* ```
*
* ## Import
* Using `pulumi import`, import Cognito User using the `user_pool_id`/`name` attributes concatenated. For example:
* ```sh
* $ pulumi import aws:cognito/user:User user us-east-1_vG78M4goG/user
* ```
*/
public class User internal constructor(
override val javaResource: com.pulumi.aws.cognito.User,
) : KotlinCustomResource(javaResource, UserMapper) {
/**
* A map that contains user attributes and attribute values to be set for the user.
*/
public val attributes: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy