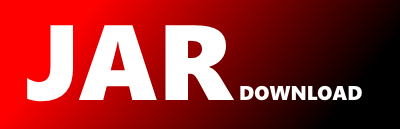
com.pulumi.aws.cognito.kotlin.UserPoolClientArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cognito.kotlin
import com.pulumi.aws.cognito.UserPoolClientArgs.builder
import com.pulumi.aws.cognito.kotlin.inputs.UserPoolClientAnalyticsConfigurationArgs
import com.pulumi.aws.cognito.kotlin.inputs.UserPoolClientAnalyticsConfigurationArgsBuilder
import com.pulumi.aws.cognito.kotlin.inputs.UserPoolClientTokenValidityUnitsArgs
import com.pulumi.aws.cognito.kotlin.inputs.UserPoolClientTokenValidityUnitsArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Provides a Cognito User Pool Client resource.
* To manage a User Pool Client created by another service, such as when [configuring an OpenSearch Domain to use Cognito authentication](https://docs.aws.amazon.com/opensearch-service/latest/developerguide/cognito-auth.html),
* use the `aws.cognito.ManagedUserPoolClient` resource instead.
* ## Example Usage
* ### Create a basic user pool client
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const pool = new aws.cognito.UserPool("pool", {name: "pool"});
* const client = new aws.cognito.UserPoolClient("client", {
* name: "client",
* userPoolId: pool.id,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* pool = aws.cognito.UserPool("pool", name="pool")
* client = aws.cognito.UserPoolClient("client",
* name="client",
* user_pool_id=pool.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var pool = new Aws.Cognito.UserPool("pool", new()
* {
* Name = "pool",
* });
* var client = new Aws.Cognito.UserPoolClient("client", new()
* {
* Name = "client",
* UserPoolId = pool.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cognito"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* pool, err := cognito.NewUserPool(ctx, "pool", &cognito.UserPoolArgs{
* Name: pulumi.String("pool"),
* })
* if err != nil {
* return err
* }
* _, err = cognito.NewUserPoolClient(ctx, "client", &cognito.UserPoolClientArgs{
* Name: pulumi.String("client"),
* UserPoolId: pool.ID(),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cognito.UserPool;
* import com.pulumi.aws.cognito.UserPoolArgs;
* import com.pulumi.aws.cognito.UserPoolClient;
* import com.pulumi.aws.cognito.UserPoolClientArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pool = new UserPool("pool", UserPoolArgs.builder()
* .name("pool")
* .build());
* var client = new UserPoolClient("client", UserPoolClientArgs.builder()
* .name("client")
* .userPoolId(pool.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* client:
* type: aws:cognito:UserPoolClient
* properties:
* name: client
* userPoolId: ${pool.id}
* pool:
* type: aws:cognito:UserPool
* properties:
* name: pool
* ```
*
* ### Create a user pool client with no SRP authentication
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const pool = new aws.cognito.UserPool("pool", {name: "pool"});
* const client = new aws.cognito.UserPoolClient("client", {
* name: "client",
* userPoolId: pool.id,
* generateSecret: true,
* explicitAuthFlows: ["ADMIN_NO_SRP_AUTH"],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* pool = aws.cognito.UserPool("pool", name="pool")
* client = aws.cognito.UserPoolClient("client",
* name="client",
* user_pool_id=pool.id,
* generate_secret=True,
* explicit_auth_flows=["ADMIN_NO_SRP_AUTH"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var pool = new Aws.Cognito.UserPool("pool", new()
* {
* Name = "pool",
* });
* var client = new Aws.Cognito.UserPoolClient("client", new()
* {
* Name = "client",
* UserPoolId = pool.Id,
* GenerateSecret = true,
* ExplicitAuthFlows = new[]
* {
* "ADMIN_NO_SRP_AUTH",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cognito"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* pool, err := cognito.NewUserPool(ctx, "pool", &cognito.UserPoolArgs{
* Name: pulumi.String("pool"),
* })
* if err != nil {
* return err
* }
* _, err = cognito.NewUserPoolClient(ctx, "client", &cognito.UserPoolClientArgs{
* Name: pulumi.String("client"),
* UserPoolId: pool.ID(),
* GenerateSecret: pulumi.Bool(true),
* ExplicitAuthFlows: pulumi.StringArray{
* pulumi.String("ADMIN_NO_SRP_AUTH"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cognito.UserPool;
* import com.pulumi.aws.cognito.UserPoolArgs;
* import com.pulumi.aws.cognito.UserPoolClient;
* import com.pulumi.aws.cognito.UserPoolClientArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pool = new UserPool("pool", UserPoolArgs.builder()
* .name("pool")
* .build());
* var client = new UserPoolClient("client", UserPoolClientArgs.builder()
* .name("client")
* .userPoolId(pool.id())
* .generateSecret(true)
* .explicitAuthFlows("ADMIN_NO_SRP_AUTH")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* client:
* type: aws:cognito:UserPoolClient
* properties:
* name: client
* userPoolId: ${pool.id}
* generateSecret: true
* explicitAuthFlows:
* - ADMIN_NO_SRP_AUTH
* pool:
* type: aws:cognito:UserPool
* properties:
* name: pool
* ```
*
* ### Create a user pool client with pinpoint analytics
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const testUserPool = new aws.cognito.UserPool("test", {name: "pool"});
* const testApp = new aws.pinpoint.App("test", {name: "pinpoint"});
* const assumeRole = aws.iam.getPolicyDocument({
* statements: [{
* effect: "Allow",
* principals: [{
* type: "Service",
* identifiers: ["cognito-idp.amazonaws.com"],
* }],
* actions: ["sts:AssumeRole"],
* }],
* });
* const testRole = new aws.iam.Role("test", {
* name: "role",
* assumeRolePolicy: assumeRole.then(assumeRole => assumeRole.json),
* });
* const testUserPoolClient = new aws.cognito.UserPoolClient("test", {
* name: "pool_client",
* userPoolId: testUserPool.id,
* analyticsConfiguration: {
* applicationId: testApp.applicationId,
* externalId: "some_id",
* roleArn: testRole.arn,
* userDataShared: true,
* },
* });
* const current = aws.getCallerIdentity({});
* const test = aws.iam.getPolicyDocumentOutput({
* statements: [{
* effect: "Allow",
* actions: [
* "mobiletargeting:UpdateEndpoint",
* "mobiletargeting:PutEvents",
* ],
* resources: [pulumi.all([current, testApp.applicationId]).apply(([current, applicationId]) => `arn:aws:mobiletargeting:*:${current.accountId}:apps/${applicationId}*`)],
* }],
* });
* const testRolePolicy = new aws.iam.RolePolicy("test", {
* name: "role_policy",
* role: testRole.id,
* policy: test.apply(test => test.json),
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* test_user_pool = aws.cognito.UserPool("test", name="pool")
* test_app = aws.pinpoint.App("test", name="pinpoint")
* assume_role = aws.iam.get_policy_document(statements=[{
* "effect": "Allow",
* "principals": [{
* "type": "Service",
* "identifiers": ["cognito-idp.amazonaws.com"],
* }],
* "actions": ["sts:AssumeRole"],
* }])
* test_role = aws.iam.Role("test",
* name="role",
* assume_role_policy=assume_role.json)
* test_user_pool_client = aws.cognito.UserPoolClient("test",
* name="pool_client",
* user_pool_id=test_user_pool.id,
* analytics_configuration={
* "application_id": test_app.application_id,
* "external_id": "some_id",
* "role_arn": test_role.arn,
* "user_data_shared": True,
* })
* current = aws.get_caller_identity()
* test = aws.iam.get_policy_document_output(statements=[{
* "effect": "Allow",
* "actions": [
* "mobiletargeting:UpdateEndpoint",
* "mobiletargeting:PutEvents",
* ],
* "resources": [test_app.application_id.apply(lambda application_id: f"arn:aws:mobiletargeting:*:{current.account_id}:apps/{application_id}*")],
* }])
* test_role_policy = aws.iam.RolePolicy("test",
* name="role_policy",
* role=test_role.id,
* policy=test.json)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var testUserPool = new Aws.Cognito.UserPool("test", new()
* {
* Name = "pool",
* });
* var testApp = new Aws.Pinpoint.App("test", new()
* {
* Name = "pinpoint",
* });
* var assumeRole = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Effect = "Allow",
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "Service",
* Identifiers = new[]
* {
* "cognito-idp.amazonaws.com",
* },
* },
* },
* Actions = new[]
* {
* "sts:AssumeRole",
* },
* },
* },
* });
* var testRole = new Aws.Iam.Role("test", new()
* {
* Name = "role",
* AssumeRolePolicy = assumeRole.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* var testUserPoolClient = new Aws.Cognito.UserPoolClient("test", new()
* {
* Name = "pool_client",
* UserPoolId = testUserPool.Id,
* AnalyticsConfiguration = new Aws.Cognito.Inputs.UserPoolClientAnalyticsConfigurationArgs
* {
* ApplicationId = testApp.ApplicationId,
* ExternalId = "some_id",
* RoleArn = testRole.Arn,
* UserDataShared = true,
* },
* });
* var current = Aws.GetCallerIdentity.Invoke();
* var test = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Effect = "Allow",
* Actions = new[]
* {
* "mobiletargeting:UpdateEndpoint",
* "mobiletargeting:PutEvents",
* },
* Resources = new[]
* {
* $"arn:aws:mobiletargeting:*:{current.Apply(getCallerIdentityResult => getCallerIdentityResult.AccountId)}:apps/{testApp.ApplicationId}*",
* },
* },
* },
* });
* var testRolePolicy = new Aws.Iam.RolePolicy("test", new()
* {
* Name = "role_policy",
* Role = testRole.Id,
* Policy = test.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cognito"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/pinpoint"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* testUserPool, err := cognito.NewUserPool(ctx, "test", &cognito.UserPoolArgs{
* Name: pulumi.String("pool"),
* })
* if err != nil {
* return err
* }
* testApp, err := pinpoint.NewApp(ctx, "test", &pinpoint.AppArgs{
* Name: pulumi.String("pinpoint"),
* })
* if err != nil {
* return err
* }
* assumeRole, err := iam.GetPolicyDocument(ctx, &iam.GetPolicyDocumentArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Effect: pulumi.StringRef("Allow"),
* Principals: []iam.GetPolicyDocumentStatementPrincipal{
* {
* Type: "Service",
* Identifiers: []string{
* "cognito-idp.amazonaws.com",
* },
* },
* },
* Actions: []string{
* "sts:AssumeRole",
* },
* },
* },
* }, nil)
* if err != nil {
* return err
* }
* testRole, err := iam.NewRole(ctx, "test", &iam.RoleArgs{
* Name: pulumi.String("role"),
* AssumeRolePolicy: pulumi.String(assumeRole.Json),
* })
* if err != nil {
* return err
* }
* _, err = cognito.NewUserPoolClient(ctx, "test", &cognito.UserPoolClientArgs{
* Name: pulumi.String("pool_client"),
* UserPoolId: testUserPool.ID(),
* AnalyticsConfiguration: &cognito.UserPoolClientAnalyticsConfigurationArgs{
* ApplicationId: testApp.ApplicationId,
* ExternalId: pulumi.String("some_id"),
* RoleArn: testRole.Arn,
* UserDataShared: pulumi.Bool(true),
* },
* })
* if err != nil {
* return err
* }
* current, err := aws.GetCallerIdentity(ctx, &aws.GetCallerIdentityArgs{}, nil)
* if err != nil {
* return err
* }
* test := iam.GetPolicyDocumentOutput(ctx, iam.GetPolicyDocumentOutputArgs{
* Statements: iam.GetPolicyDocumentStatementArray{
* &iam.GetPolicyDocumentStatementArgs{
* Effect: pulumi.String("Allow"),
* Actions: pulumi.StringArray{
* pulumi.String("mobiletargeting:UpdateEndpoint"),
* pulumi.String("mobiletargeting:PutEvents"),
* },
* Resources: pulumi.StringArray{
* testApp.ApplicationId.ApplyT(func(applicationId string) (string, error) {
* return fmt.Sprintf("arn:aws:mobiletargeting:*:%v:apps/%v*", current.AccountId, applicationId), nil
* }).(pulumi.StringOutput),
* },
* },
* },
* }, nil)
* _, err = iam.NewRolePolicy(ctx, "test", &iam.RolePolicyArgs{
* Name: pulumi.String("role_policy"),
* Role: testRole.ID(),
* Policy: pulumi.String(test.ApplyT(func(test iam.GetPolicyDocumentResult) (*string, error) {
* return &test.Json, nil
* }).(pulumi.StringPtrOutput)),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cognito.UserPool;
* import com.pulumi.aws.cognito.UserPoolArgs;
* import com.pulumi.aws.pinpoint.App;
* import com.pulumi.aws.pinpoint.AppArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.cognito.UserPoolClient;
* import com.pulumi.aws.cognito.UserPoolClientArgs;
* import com.pulumi.aws.cognito.inputs.UserPoolClientAnalyticsConfigurationArgs;
* import com.pulumi.aws.AwsFunctions;
* import com.pulumi.aws.inputs.GetCallerIdentityArgs;
* import com.pulumi.aws.iam.RolePolicy;
* import com.pulumi.aws.iam.RolePolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var testUserPool = new UserPool("testUserPool", UserPoolArgs.builder()
* .name("pool")
* .build());
* var testApp = new App("testApp", AppArgs.builder()
* .name("pinpoint")
* .build());
* final var assumeRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("cognito-idp.amazonaws.com")
* .build())
* .actions("sts:AssumeRole")
* .build())
* .build());
* var testRole = new Role("testRole", RoleArgs.builder()
* .name("role")
* .assumeRolePolicy(assumeRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* var testUserPoolClient = new UserPoolClient("testUserPoolClient", UserPoolClientArgs.builder()
* .name("pool_client")
* .userPoolId(testUserPool.id())
* .analyticsConfiguration(UserPoolClientAnalyticsConfigurationArgs.builder()
* .applicationId(testApp.applicationId())
* .externalId("some_id")
* .roleArn(testRole.arn())
* .userDataShared(true)
* .build())
* .build());
* final var current = AwsFunctions.getCallerIdentity();
* final var test = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions(
* "mobiletargeting:UpdateEndpoint",
* "mobiletargeting:PutEvents")
* .resources(testApp.applicationId().applyValue(applicationId -> String.format("arn:aws:mobiletargeting:*:%s:apps/%s*", current.applyValue(getCallerIdentityResult -> getCallerIdentityResult.accountId()),applicationId)))
* .build())
* .build());
* var testRolePolicy = new RolePolicy("testRolePolicy", RolePolicyArgs.builder()
* .name("role_policy")
* .role(testRole.id())
* .policy(test.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult).applyValue(test -> test.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json())))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* testUserPoolClient:
* type: aws:cognito:UserPoolClient
* name: test
* properties:
* name: pool_client
* userPoolId: ${testUserPool.id}
* analyticsConfiguration:
* applicationId: ${testApp.applicationId}
* externalId: some_id
* roleArn: ${testRole.arn}
* userDataShared: true
* testUserPool:
* type: aws:cognito:UserPool
* name: test
* properties:
* name: pool
* testApp:
* type: aws:pinpoint:App
* name: test
* properties:
* name: pinpoint
* testRole:
* type: aws:iam:Role
* name: test
* properties:
* name: role
* assumeRolePolicy: ${assumeRole.json}
* testRolePolicy:
* type: aws:iam:RolePolicy
* name: test
* properties:
* name: role_policy
* role: ${testRole.id}
* policy: ${test.json}
* variables:
* current:
* fn::invoke:
* Function: aws:getCallerIdentity
* Arguments: {}
* assumeRole:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - effect: Allow
* principals:
* - type: Service
* identifiers:
* - cognito-idp.amazonaws.com
* actions:
* - sts:AssumeRole
* test:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - effect: Allow
* actions:
* - mobiletargeting:UpdateEndpoint
* - mobiletargeting:PutEvents
* resources:
* - arn:aws:mobiletargeting:*:${current.accountId}:apps/${testApp.applicationId}*
* ```
*
* ### Create a user pool client with Cognito as the identity provider
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const pool = new aws.cognito.UserPool("pool", {name: "pool"});
* const userpoolClient = new aws.cognito.UserPoolClient("userpool_client", {
* name: "client",
* userPoolId: pool.id,
* callbackUrls: ["https://example.com"],
* allowedOauthFlowsUserPoolClient: true,
* allowedOauthFlows: [
* "code",
* "implicit",
* ],
* allowedOauthScopes: [
* "email",
* "openid",
* ],
* supportedIdentityProviders: ["COGNITO"],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* pool = aws.cognito.UserPool("pool", name="pool")
* userpool_client = aws.cognito.UserPoolClient("userpool_client",
* name="client",
* user_pool_id=pool.id,
* callback_urls=["https://example.com"],
* allowed_oauth_flows_user_pool_client=True,
* allowed_oauth_flows=[
* "code",
* "implicit",
* ],
* allowed_oauth_scopes=[
* "email",
* "openid",
* ],
* supported_identity_providers=["COGNITO"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var pool = new Aws.Cognito.UserPool("pool", new()
* {
* Name = "pool",
* });
* var userpoolClient = new Aws.Cognito.UserPoolClient("userpool_client", new()
* {
* Name = "client",
* UserPoolId = pool.Id,
* CallbackUrls = new[]
* {
* "https://example.com",
* },
* AllowedOauthFlowsUserPoolClient = true,
* AllowedOauthFlows = new[]
* {
* "code",
* "implicit",
* },
* AllowedOauthScopes = new[]
* {
* "email",
* "openid",
* },
* SupportedIdentityProviders = new[]
* {
* "COGNITO",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cognito"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* pool, err := cognito.NewUserPool(ctx, "pool", &cognito.UserPoolArgs{
* Name: pulumi.String("pool"),
* })
* if err != nil {
* return err
* }
* _, err = cognito.NewUserPoolClient(ctx, "userpool_client", &cognito.UserPoolClientArgs{
* Name: pulumi.String("client"),
* UserPoolId: pool.ID(),
* CallbackUrls: pulumi.StringArray{
* pulumi.String("https://example.com"),
* },
* AllowedOauthFlowsUserPoolClient: pulumi.Bool(true),
* AllowedOauthFlows: pulumi.StringArray{
* pulumi.String("code"),
* pulumi.String("implicit"),
* },
* AllowedOauthScopes: pulumi.StringArray{
* pulumi.String("email"),
* pulumi.String("openid"),
* },
* SupportedIdentityProviders: pulumi.StringArray{
* pulumi.String("COGNITO"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cognito.UserPool;
* import com.pulumi.aws.cognito.UserPoolArgs;
* import com.pulumi.aws.cognito.UserPoolClient;
* import com.pulumi.aws.cognito.UserPoolClientArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pool = new UserPool("pool", UserPoolArgs.builder()
* .name("pool")
* .build());
* var userpoolClient = new UserPoolClient("userpoolClient", UserPoolClientArgs.builder()
* .name("client")
* .userPoolId(pool.id())
* .callbackUrls("https://example.com")
* .allowedOauthFlowsUserPoolClient(true)
* .allowedOauthFlows(
* "code",
* "implicit")
* .allowedOauthScopes(
* "email",
* "openid")
* .supportedIdentityProviders("COGNITO")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* userpoolClient:
* type: aws:cognito:UserPoolClient
* name: userpool_client
* properties:
* name: client
* userPoolId: ${pool.id}
* callbackUrls:
* - https://example.com
* allowedOauthFlowsUserPoolClient: true
* allowedOauthFlows:
* - code
* - implicit
* allowedOauthScopes:
* - email
* - openid
* supportedIdentityProviders:
* - COGNITO
* pool:
* type: aws:cognito:UserPool
* properties:
* name: pool
* ```
*
* ## Import
* Using `pulumi import`, import Cognito User Pool Clients using the `id` of the Cognito User Pool, and the `id` of the Cognito User Pool Client. For example:
* ```sh
* $ pulumi import aws:cognito/userPoolClient:UserPoolClient client us-west-2_abc123/3ho4ek12345678909nh3fmhpko
* ```
* @property accessTokenValidity Time limit, between 5 minutes and 1 day, after which the access token is no longer valid and cannot be used. By default, the unit is hours. The unit can be overridden by a value in `token_validity_units.access_token`.
* @property allowedOauthFlows List of allowed OAuth flows, including `code`, `implicit`, and `client_credentials`. `allowed_oauth_flows_user_pool_client` must be set to `true` before you can configure this option.
* @property allowedOauthFlowsUserPoolClient Whether the client is allowed to use OAuth 2.0 features. `allowed_oauth_flows_user_pool_client` must be set to `true` before you can configure the following arguments: `callback_urls`, `logout_urls`, `allowed_oauth_scopes` and `allowed_oauth_flows`.
* @property allowedOauthScopes List of allowed OAuth scopes, including `phone`, `email`, `openid`, `profile`, and `aws.cognito.signin.user.admin`. `allowed_oauth_flows_user_pool_client` must be set to `true` before you can configure this option.
* @property analyticsConfiguration Configuration block for Amazon Pinpoint analytics that collects metrics for this user pool. See details below.
* @property authSessionValidity Duration, in minutes, of the session token created by Amazon Cognito for each API request in an authentication flow. The session token must be responded to by the native user of the user pool before it expires. Valid values for `auth_session_validity` are between `3` and `15`, with a default value of `3`.
* @property callbackUrls List of allowed callback URLs for the identity providers. `allowed_oauth_flows_user_pool_client` must be set to `true` before you can configure this option.
* @property defaultRedirectUri Default redirect URI and must be included in the list of callback URLs.
* @property enablePropagateAdditionalUserContextData Enables the propagation of additional user context data.
* @property enableTokenRevocation Enables or disables token revocation.
* @property explicitAuthFlows List of authentication flows. The available options include ADMIN_NO_SRP_AUTH, CUSTOM_AUTH_FLOW_ONLY, USER_PASSWORD_AUTH, ALLOW_ADMIN_USER_PASSWORD_AUTH, ALLOW_CUSTOM_AUTH, ALLOW_USER_PASSWORD_AUTH, ALLOW_USER_SRP_AUTH, and ALLOW_REFRESH_TOKEN_AUTH.
* @property generateSecret Boolean flag indicating whether an application secret should be generated.
* @property idTokenValidity Time limit, between 5 minutes and 1 day, after which the ID token is no longer valid and cannot be used. By default, the unit is hours. The unit can be overridden by a value in `token_validity_units.id_token`.
* @property logoutUrls List of allowed logout URLs for the identity providers. `allowed_oauth_flows_user_pool_client` must be set to `true` before you can configure this option.
* @property name Name of the application client.
* @property preventUserExistenceErrors Setting determines the errors and responses returned by Cognito APIs when a user does not exist in the user pool during authentication, account confirmation, and password recovery.
* @property readAttributes List of user pool attributes that the application client can read from.
* @property refreshTokenValidity Time limit, between 60 minutes and 10 years, after which the refresh token is no longer valid and cannot be used. By default, the unit is days. The unit can be overridden by a value in `token_validity_units.refresh_token`.
* @property supportedIdentityProviders List of provider names for the identity providers that are supported on this client. It uses the `provider_name` attribute of the `aws.cognito.IdentityProvider` resource(s), or the equivalent string(s).
* @property tokenValidityUnits Configuration block for representing the validity times in units. See details below. Detailed below.
* @property userPoolId User pool the client belongs to.
* The following arguments are optional:
* @property writeAttributes List of user pool attributes that the application client can write to.
*/
public data class UserPoolClientArgs(
public val accessTokenValidity: Output? = null,
public val allowedOauthFlows: Output>? = null,
public val allowedOauthFlowsUserPoolClient: Output? = null,
public val allowedOauthScopes: Output>? = null,
public val analyticsConfiguration: Output? = null,
public val authSessionValidity: Output? = null,
public val callbackUrls: Output>? = null,
public val defaultRedirectUri: Output? = null,
public val enablePropagateAdditionalUserContextData: Output? = null,
public val enableTokenRevocation: Output? = null,
public val explicitAuthFlows: Output>? = null,
public val generateSecret: Output? = null,
public val idTokenValidity: Output? = null,
public val logoutUrls: Output>? = null,
public val name: Output? = null,
public val preventUserExistenceErrors: Output? = null,
public val readAttributes: Output>? = null,
public val refreshTokenValidity: Output? = null,
public val supportedIdentityProviders: Output>? = null,
public val tokenValidityUnits: Output? = null,
public val userPoolId: Output? = null,
public val writeAttributes: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.cognito.UserPoolClientArgs =
com.pulumi.aws.cognito.UserPoolClientArgs.builder()
.accessTokenValidity(accessTokenValidity?.applyValue({ args0 -> args0 }))
.allowedOauthFlows(allowedOauthFlows?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.allowedOauthFlowsUserPoolClient(allowedOauthFlowsUserPoolClient?.applyValue({ args0 -> args0 }))
.allowedOauthScopes(allowedOauthScopes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.analyticsConfiguration(
analyticsConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.authSessionValidity(authSessionValidity?.applyValue({ args0 -> args0 }))
.callbackUrls(callbackUrls?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.defaultRedirectUri(defaultRedirectUri?.applyValue({ args0 -> args0 }))
.enablePropagateAdditionalUserContextData(
enablePropagateAdditionalUserContextData?.applyValue({ args0 ->
args0
}),
)
.enableTokenRevocation(enableTokenRevocation?.applyValue({ args0 -> args0 }))
.explicitAuthFlows(explicitAuthFlows?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.generateSecret(generateSecret?.applyValue({ args0 -> args0 }))
.idTokenValidity(idTokenValidity?.applyValue({ args0 -> args0 }))
.logoutUrls(logoutUrls?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.name(name?.applyValue({ args0 -> args0 }))
.preventUserExistenceErrors(preventUserExistenceErrors?.applyValue({ args0 -> args0 }))
.readAttributes(readAttributes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.refreshTokenValidity(refreshTokenValidity?.applyValue({ args0 -> args0 }))
.supportedIdentityProviders(
supportedIdentityProviders?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
)
.tokenValidityUnits(
tokenValidityUnits?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.userPoolId(userPoolId?.applyValue({ args0 -> args0 }))
.writeAttributes(writeAttributes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [UserPoolClientArgs].
*/
@PulumiTagMarker
public class UserPoolClientArgsBuilder internal constructor() {
private var accessTokenValidity: Output? = null
private var allowedOauthFlows: Output>? = null
private var allowedOauthFlowsUserPoolClient: Output? = null
private var allowedOauthScopes: Output>? = null
private var analyticsConfiguration: Output? = null
private var authSessionValidity: Output? = null
private var callbackUrls: Output>? = null
private var defaultRedirectUri: Output? = null
private var enablePropagateAdditionalUserContextData: Output? = null
private var enableTokenRevocation: Output? = null
private var explicitAuthFlows: Output>? = null
private var generateSecret: Output? = null
private var idTokenValidity: Output? = null
private var logoutUrls: Output>? = null
private var name: Output? = null
private var preventUserExistenceErrors: Output? = null
private var readAttributes: Output>? = null
private var refreshTokenValidity: Output? = null
private var supportedIdentityProviders: Output>? = null
private var tokenValidityUnits: Output? = null
private var userPoolId: Output? = null
private var writeAttributes: Output>? = null
/**
* @param value Time limit, between 5 minutes and 1 day, after which the access token is no longer valid and cannot be used. By default, the unit is hours. The unit can be overridden by a value in `token_validity_units.access_token`.
*/
@JvmName("alhepmedsvexwrwq")
public suspend fun accessTokenValidity(`value`: Output) {
this.accessTokenValidity = value
}
/**
* @param value List of allowed OAuth flows, including `code`, `implicit`, and `client_credentials`. `allowed_oauth_flows_user_pool_client` must be set to `true` before you can configure this option.
*/
@JvmName("cjgjpcpeqsbexbfl")
public suspend fun allowedOauthFlows(`value`: Output>) {
this.allowedOauthFlows = value
}
@JvmName("sdxdswaxctbxejos")
public suspend fun allowedOauthFlows(vararg values: Output) {
this.allowedOauthFlows = Output.all(values.asList())
}
/**
* @param values List of allowed OAuth flows, including `code`, `implicit`, and `client_credentials`. `allowed_oauth_flows_user_pool_client` must be set to `true` before you can configure this option.
*/
@JvmName("ktraqnhgnggbkqli")
public suspend fun allowedOauthFlows(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy