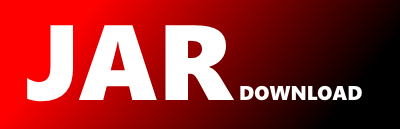
com.pulumi.aws.cognito.kotlin.inputs.RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cognito.kotlin.inputs
import com.pulumi.aws.cognito.inputs.RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property blockEmail Email template used when a detected risk event is blocked. See notify email type below.
* @property from The email address that is sending the email. The address must be either individually verified with Amazon Simple Email Service, or from a domain that has been verified with Amazon SES.
* @property mfaEmail The multi-factor authentication (MFA) email template used when MFA is challenged as part of a detected risk. See notify email type below.
* @property noActionEmail The email template used when a detected risk event is allowed. See notify email type below.
* @property replyTo The destination to which the receiver of an email should reply to.
* @property sourceArn The Amazon Resource Name (ARN) of the identity that is associated with the sending authorization policy. This identity permits Amazon Cognito to send for the email address specified in the From parameter.
*/
public data class RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationArgs(
public val blockEmail: Output? =
null,
public val from: Output? = null,
public val mfaEmail: Output? =
null,
public val noActionEmail: Output? =
null,
public val replyTo: Output? = null,
public val sourceArn: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.cognito.inputs.RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationArgs =
com.pulumi.aws.cognito.inputs.RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationArgs.builder()
.blockEmail(blockEmail?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.from(from?.applyValue({ args0 -> args0 }))
.mfaEmail(mfaEmail?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.noActionEmail(noActionEmail?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.replyTo(replyTo?.applyValue({ args0 -> args0 }))
.sourceArn(sourceArn.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationArgs].
*/
@PulumiTagMarker
public class RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationArgsBuilder
internal constructor() {
private var blockEmail:
Output? =
null
private var from: Output? = null
private var mfaEmail:
Output? =
null
private var noActionEmail:
Output? =
null
private var replyTo: Output? = null
private var sourceArn: Output? = null
/**
* @param value Email template used when a detected risk event is blocked. See notify email type below.
*/
@JvmName("mpwvsmioludyaypr")
public suspend fun blockEmail(`value`: Output) {
this.blockEmail = value
}
/**
* @param value The email address that is sending the email. The address must be either individually verified with Amazon Simple Email Service, or from a domain that has been verified with Amazon SES.
*/
@JvmName("tdagkjhrlfaflxgq")
public suspend fun from(`value`: Output) {
this.from = value
}
/**
* @param value The multi-factor authentication (MFA) email template used when MFA is challenged as part of a detected risk. See notify email type below.
*/
@JvmName("geuwpurdprljbfyn")
public suspend fun mfaEmail(`value`: Output) {
this.mfaEmail = value
}
/**
* @param value The email template used when a detected risk event is allowed. See notify email type below.
*/
@JvmName("imyjafxuscfstaij")
public suspend fun noActionEmail(`value`: Output) {
this.noActionEmail = value
}
/**
* @param value The destination to which the receiver of an email should reply to.
*/
@JvmName("fmiuprtawcpjvlfe")
public suspend fun replyTo(`value`: Output) {
this.replyTo = value
}
/**
* @param value The Amazon Resource Name (ARN) of the identity that is associated with the sending authorization policy. This identity permits Amazon Cognito to send for the email address specified in the From parameter.
*/
@JvmName("iwcnqnfmykbabctj")
public suspend fun sourceArn(`value`: Output) {
this.sourceArn = value
}
/**
* @param value Email template used when a detected risk event is blocked. See notify email type below.
*/
@JvmName("igogosgvyugmtyjv")
public suspend fun blockEmail(`value`: RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationBlockEmailArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.blockEmail = mapped
}
/**
* @param argument Email template used when a detected risk event is blocked. See notify email type below.
*/
@JvmName("qbddymccslhqgndc")
public suspend fun blockEmail(argument: suspend RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationBlockEmailArgsBuilder.() -> Unit) {
val toBeMapped =
RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationBlockEmailArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.blockEmail = mapped
}
/**
* @param value The email address that is sending the email. The address must be either individually verified with Amazon Simple Email Service, or from a domain that has been verified with Amazon SES.
*/
@JvmName("gkvcqdamvlnkxtxq")
public suspend fun from(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.from = mapped
}
/**
* @param value The multi-factor authentication (MFA) email template used when MFA is challenged as part of a detected risk. See notify email type below.
*/
@JvmName("kbqpdycjqgyhhxrx")
public suspend fun mfaEmail(`value`: RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationMfaEmailArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mfaEmail = mapped
}
/**
* @param argument The multi-factor authentication (MFA) email template used when MFA is challenged as part of a detected risk. See notify email type below.
*/
@JvmName("jrverbdagrlfusmp")
public suspend fun mfaEmail(argument: suspend RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationMfaEmailArgsBuilder.() -> Unit) {
val toBeMapped =
RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationMfaEmailArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.mfaEmail = mapped
}
/**
* @param value The email template used when a detected risk event is allowed. See notify email type below.
*/
@JvmName("sferxwkvootqxnen")
public suspend fun noActionEmail(`value`: RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationNoActionEmailArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.noActionEmail = mapped
}
/**
* @param argument The email template used when a detected risk event is allowed. See notify email type below.
*/
@JvmName("qeswoxpnqpkwiaqg")
public suspend fun noActionEmail(argument: suspend RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationNoActionEmailArgsBuilder.() -> Unit) {
val toBeMapped =
RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationNoActionEmailArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.noActionEmail = mapped
}
/**
* @param value The destination to which the receiver of an email should reply to.
*/
@JvmName("stradxhxrflvkiem")
public suspend fun replyTo(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.replyTo = mapped
}
/**
* @param value The Amazon Resource Name (ARN) of the identity that is associated with the sending authorization policy. This identity permits Amazon Cognito to send for the email address specified in the From parameter.
*/
@JvmName("vtyfykjtthqxamwu")
public suspend fun sourceArn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sourceArn = mapped
}
internal fun build(): RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationArgs =
RiskConfigurationAccountTakeoverRiskConfigurationNotifyConfigurationArgs(
blockEmail = blockEmail,
from = from,
mfaEmail = mfaEmail,
noActionEmail = noActionEmail,
replyTo = replyTo,
sourceArn = sourceArn ?: throw PulumiNullFieldException("sourceArn"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy