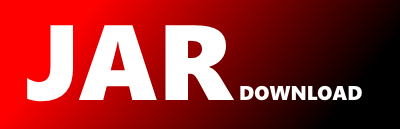
com.pulumi.aws.cognito.kotlin.inputs.UserPoolEmailConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cognito.kotlin.inputs
import com.pulumi.aws.cognito.inputs.UserPoolEmailConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property configurationSet Email configuration set name from SES.
* @property emailSendingAccount Email delivery method to use. `COGNITO_DEFAULT` for the default email functionality built into Cognito or `DEVELOPER` to use your Amazon SES configuration. Required to be `DEVELOPER` if `from_email_address` is set.
* @property fromEmailAddress Sender’s email address or sender’s display name with their email address (e.g., `[email protected]`, `John Smith ` or `\"John Smith Ph.D.\" `). Escaped double quotes are required around display names that contain certain characters as specified in [RFC 5322](https://tools.ietf.org/html/rfc5322).
* @property replyToEmailAddress REPLY-TO email address.
* @property sourceArn ARN of the SES verified email identity to use. Required if `email_sending_account` is set to `DEVELOPER`.
*/
public data class UserPoolEmailConfigurationArgs(
public val configurationSet: Output? = null,
public val emailSendingAccount: Output? = null,
public val fromEmailAddress: Output? = null,
public val replyToEmailAddress: Output? = null,
public val sourceArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.cognito.inputs.UserPoolEmailConfigurationArgs =
com.pulumi.aws.cognito.inputs.UserPoolEmailConfigurationArgs.builder()
.configurationSet(configurationSet?.applyValue({ args0 -> args0 }))
.emailSendingAccount(emailSendingAccount?.applyValue({ args0 -> args0 }))
.fromEmailAddress(fromEmailAddress?.applyValue({ args0 -> args0 }))
.replyToEmailAddress(replyToEmailAddress?.applyValue({ args0 -> args0 }))
.sourceArn(sourceArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [UserPoolEmailConfigurationArgs].
*/
@PulumiTagMarker
public class UserPoolEmailConfigurationArgsBuilder internal constructor() {
private var configurationSet: Output? = null
private var emailSendingAccount: Output? = null
private var fromEmailAddress: Output? = null
private var replyToEmailAddress: Output? = null
private var sourceArn: Output? = null
/**
* @param value Email configuration set name from SES.
*/
@JvmName("sldulgimqkxdkupn")
public suspend fun configurationSet(`value`: Output) {
this.configurationSet = value
}
/**
* @param value Email delivery method to use. `COGNITO_DEFAULT` for the default email functionality built into Cognito or `DEVELOPER` to use your Amazon SES configuration. Required to be `DEVELOPER` if `from_email_address` is set.
*/
@JvmName("afgulgdjkhmhilxe")
public suspend fun emailSendingAccount(`value`: Output) {
this.emailSendingAccount = value
}
/**
* @param value Sender’s email address or sender’s display name with their email address (e.g., `[email protected]`, `John Smith ` or `\"John Smith Ph.D.\" `). Escaped double quotes are required around display names that contain certain characters as specified in [RFC 5322](https://tools.ietf.org/html/rfc5322).
*/
@JvmName("hdcemluusgipkkid")
public suspend fun fromEmailAddress(`value`: Output) {
this.fromEmailAddress = value
}
/**
* @param value REPLY-TO email address.
*/
@JvmName("ihsedmusqexhoyda")
public suspend fun replyToEmailAddress(`value`: Output) {
this.replyToEmailAddress = value
}
/**
* @param value ARN of the SES verified email identity to use. Required if `email_sending_account` is set to `DEVELOPER`.
*/
@JvmName("xrpfadsajtcjbgdk")
public suspend fun sourceArn(`value`: Output) {
this.sourceArn = value
}
/**
* @param value Email configuration set name from SES.
*/
@JvmName("krhkgcxadkuveilx")
public suspend fun configurationSet(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.configurationSet = mapped
}
/**
* @param value Email delivery method to use. `COGNITO_DEFAULT` for the default email functionality built into Cognito or `DEVELOPER` to use your Amazon SES configuration. Required to be `DEVELOPER` if `from_email_address` is set.
*/
@JvmName("ljerxearruswdqco")
public suspend fun emailSendingAccount(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.emailSendingAccount = mapped
}
/**
* @param value Sender’s email address or sender’s display name with their email address (e.g., `[email protected]`, `John Smith ` or `\"John Smith Ph.D.\" `). Escaped double quotes are required around display names that contain certain characters as specified in [RFC 5322](https://tools.ietf.org/html/rfc5322).
*/
@JvmName("epmgpgnvsvweafqc")
public suspend fun fromEmailAddress(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fromEmailAddress = mapped
}
/**
* @param value REPLY-TO email address.
*/
@JvmName("lmalrukjbtppsoju")
public suspend fun replyToEmailAddress(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.replyToEmailAddress = mapped
}
/**
* @param value ARN of the SES verified email identity to use. Required if `email_sending_account` is set to `DEVELOPER`.
*/
@JvmName("jfpjirnfuiqdwwwp")
public suspend fun sourceArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceArn = mapped
}
internal fun build(): UserPoolEmailConfigurationArgs = UserPoolEmailConfigurationArgs(
configurationSet = configurationSet,
emailSendingAccount = emailSendingAccount,
fromEmailAddress = fromEmailAddress,
replyToEmailAddress = replyToEmailAddress,
sourceArn = sourceArn,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy