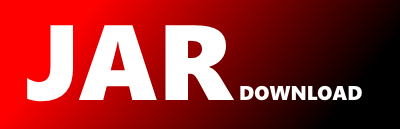
com.pulumi.aws.cognito.kotlin.inputs.UserPoolVerificationMessageTemplateArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.cognito.kotlin.inputs
import com.pulumi.aws.cognito.inputs.UserPoolVerificationMessageTemplateArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property defaultEmailOption Default email option. Must be either `CONFIRM_WITH_CODE` or `CONFIRM_WITH_LINK`. Defaults to `CONFIRM_WITH_CODE`.
* @property emailMessage Email message template. Must contain the `{####}` placeholder. Conflicts with `email_verification_message` argument.
* @property emailMessageByLink Email message template for sending a confirmation link to the user, it must contain the `{##Click Here##}` placeholder.
* @property emailSubject Subject line for the email message template. Conflicts with `email_verification_subject` argument.
* @property emailSubjectByLink Subject line for the email message template for sending a confirmation link to the user.
* @property smsMessage SMS message template. Must contain the `{####}` placeholder. Conflicts with `sms_verification_message` argument.
*/
public data class UserPoolVerificationMessageTemplateArgs(
public val defaultEmailOption: Output? = null,
public val emailMessage: Output? = null,
public val emailMessageByLink: Output? = null,
public val emailSubject: Output? = null,
public val emailSubjectByLink: Output? = null,
public val smsMessage: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.cognito.inputs.UserPoolVerificationMessageTemplateArgs =
com.pulumi.aws.cognito.inputs.UserPoolVerificationMessageTemplateArgs.builder()
.defaultEmailOption(defaultEmailOption?.applyValue({ args0 -> args0 }))
.emailMessage(emailMessage?.applyValue({ args0 -> args0 }))
.emailMessageByLink(emailMessageByLink?.applyValue({ args0 -> args0 }))
.emailSubject(emailSubject?.applyValue({ args0 -> args0 }))
.emailSubjectByLink(emailSubjectByLink?.applyValue({ args0 -> args0 }))
.smsMessage(smsMessage?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [UserPoolVerificationMessageTemplateArgs].
*/
@PulumiTagMarker
public class UserPoolVerificationMessageTemplateArgsBuilder internal constructor() {
private var defaultEmailOption: Output? = null
private var emailMessage: Output? = null
private var emailMessageByLink: Output? = null
private var emailSubject: Output? = null
private var emailSubjectByLink: Output? = null
private var smsMessage: Output? = null
/**
* @param value Default email option. Must be either `CONFIRM_WITH_CODE` or `CONFIRM_WITH_LINK`. Defaults to `CONFIRM_WITH_CODE`.
*/
@JvmName("wvtlcajhpcqnmjhm")
public suspend fun defaultEmailOption(`value`: Output) {
this.defaultEmailOption = value
}
/**
* @param value Email message template. Must contain the `{####}` placeholder. Conflicts with `email_verification_message` argument.
*/
@JvmName("jokvgkiurdtlcoxp")
public suspend fun emailMessage(`value`: Output) {
this.emailMessage = value
}
/**
* @param value Email message template for sending a confirmation link to the user, it must contain the `{##Click Here##}` placeholder.
*/
@JvmName("fvpbwstsepbpwuau")
public suspend fun emailMessageByLink(`value`: Output) {
this.emailMessageByLink = value
}
/**
* @param value Subject line for the email message template. Conflicts with `email_verification_subject` argument.
*/
@JvmName("umrdpbirjicoryyq")
public suspend fun emailSubject(`value`: Output) {
this.emailSubject = value
}
/**
* @param value Subject line for the email message template for sending a confirmation link to the user.
*/
@JvmName("ryxcspnhsjyhavow")
public suspend fun emailSubjectByLink(`value`: Output) {
this.emailSubjectByLink = value
}
/**
* @param value SMS message template. Must contain the `{####}` placeholder. Conflicts with `sms_verification_message` argument.
*/
@JvmName("pxqcqhaaswgbdtem")
public suspend fun smsMessage(`value`: Output) {
this.smsMessage = value
}
/**
* @param value Default email option. Must be either `CONFIRM_WITH_CODE` or `CONFIRM_WITH_LINK`. Defaults to `CONFIRM_WITH_CODE`.
*/
@JvmName("twjcsvvbdbxlsstw")
public suspend fun defaultEmailOption(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultEmailOption = mapped
}
/**
* @param value Email message template. Must contain the `{####}` placeholder. Conflicts with `email_verification_message` argument.
*/
@JvmName("lmcwmvhfesbboqsu")
public suspend fun emailMessage(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.emailMessage = mapped
}
/**
* @param value Email message template for sending a confirmation link to the user, it must contain the `{##Click Here##}` placeholder.
*/
@JvmName("eqrptxpsfogmsdoe")
public suspend fun emailMessageByLink(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.emailMessageByLink = mapped
}
/**
* @param value Subject line for the email message template. Conflicts with `email_verification_subject` argument.
*/
@JvmName("hdkfpuhledrhnkab")
public suspend fun emailSubject(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.emailSubject = mapped
}
/**
* @param value Subject line for the email message template for sending a confirmation link to the user.
*/
@JvmName("qojtpnuuwogkkyyn")
public suspend fun emailSubjectByLink(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.emailSubjectByLink = mapped
}
/**
* @param value SMS message template. Must contain the `{####}` placeholder. Conflicts with `sms_verification_message` argument.
*/
@JvmName("ogakpwifxfauasff")
public suspend fun smsMessage(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.smsMessage = mapped
}
internal fun build(): UserPoolVerificationMessageTemplateArgs =
UserPoolVerificationMessageTemplateArgs(
defaultEmailOption = defaultEmailOption,
emailMessage = emailMessage,
emailMessageByLink = emailMessageByLink,
emailSubject = emailSubject,
emailSubjectByLink = emailSubjectByLink,
smsMessage = smsMessage,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy