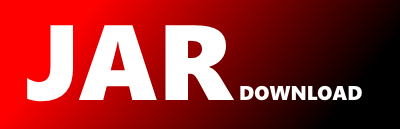
com.pulumi.aws.comprehend.kotlin.EntityRecognizer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.comprehend.kotlin
import com.pulumi.aws.comprehend.kotlin.outputs.EntityRecognizerInputDataConfig
import com.pulumi.aws.comprehend.kotlin.outputs.EntityRecognizerVpcConfig
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import com.pulumi.aws.comprehend.kotlin.outputs.EntityRecognizerInputDataConfig.Companion.toKotlin as entityRecognizerInputDataConfigToKotlin
import com.pulumi.aws.comprehend.kotlin.outputs.EntityRecognizerVpcConfig.Companion.toKotlin as entityRecognizerVpcConfigToKotlin
/**
* Builder for [EntityRecognizer].
*/
@PulumiTagMarker
public class EntityRecognizerResourceBuilder internal constructor() {
public var name: String? = null
public var args: EntityRecognizerArgs = EntityRecognizerArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend EntityRecognizerArgsBuilder.() -> Unit) {
val builder = EntityRecognizerArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): EntityRecognizer {
val builtJavaResource = com.pulumi.aws.comprehend.EntityRecognizer(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return EntityRecognizer(builtJavaResource)
}
}
/**
* Resource for managing an AWS Comprehend Entity Recognizer.
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const documents = new aws.s3.BucketObjectv2("documents", {});
* const entities = new aws.s3.BucketObjectv2("entities", {});
* const example = new aws.comprehend.EntityRecognizer("example", {
* name: "example",
* dataAccessRoleArn: exampleAwsIamRole.arn,
* languageCode: "en",
* inputDataConfig: {
* entityTypes: [
* {
* type: "ENTITY_1",
* },
* {
* type: "ENTITY_2",
* },
* ],
* documents: {
* s3Uri: pulumi.interpolate`s3://${documentsAwsS3Bucket.bucket}/${documents.id}`,
* },
* entityList: {
* s3Uri: pulumi.interpolate`s3://${entitiesAwsS3Bucket.bucket}/${entities.id}`,
* },
* },
* }, {
* dependsOn: [exampleAwsIamRolePolicy],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* documents = aws.s3.BucketObjectv2("documents")
* entities = aws.s3.BucketObjectv2("entities")
* example = aws.comprehend.EntityRecognizer("example",
* name="example",
* data_access_role_arn=example_aws_iam_role["arn"],
* language_code="en",
* input_data_config={
* "entity_types": [
* {
* "type": "ENTITY_1",
* },
* {
* "type": "ENTITY_2",
* },
* ],
* "documents": {
* "s3_uri": documents.id.apply(lambda id: f"s3://{documents_aws_s3_bucket['bucket']}/{id}"),
* },
* "entity_list": {
* "s3_uri": entities.id.apply(lambda id: f"s3://{entities_aws_s3_bucket['bucket']}/{id}"),
* },
* },
* opts = pulumi.ResourceOptions(depends_on=[example_aws_iam_role_policy]))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var documents = new Aws.S3.BucketObjectv2("documents");
* var entities = new Aws.S3.BucketObjectv2("entities");
* var example = new Aws.Comprehend.EntityRecognizer("example", new()
* {
* Name = "example",
* DataAccessRoleArn = exampleAwsIamRole.Arn,
* LanguageCode = "en",
* InputDataConfig = new Aws.Comprehend.Inputs.EntityRecognizerInputDataConfigArgs
* {
* EntityTypes = new[]
* {
* new Aws.Comprehend.Inputs.EntityRecognizerInputDataConfigEntityTypeArgs
* {
* Type = "ENTITY_1",
* },
* new Aws.Comprehend.Inputs.EntityRecognizerInputDataConfigEntityTypeArgs
* {
* Type = "ENTITY_2",
* },
* },
* Documents = new Aws.Comprehend.Inputs.EntityRecognizerInputDataConfigDocumentsArgs
* {
* S3Uri = documents.Id.Apply(id => $"s3://{documentsAwsS3Bucket.Bucket}/{id}"),
* },
* EntityList = new Aws.Comprehend.Inputs.EntityRecognizerInputDataConfigEntityListArgs
* {
* S3Uri = entities.Id.Apply(id => $"s3://{entitiesAwsS3Bucket.Bucket}/{id}"),
* },
* },
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* exampleAwsIamRolePolicy,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/comprehend"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* documents, err := s3.NewBucketObjectv2(ctx, "documents", nil)
* if err != nil {
* return err
* }
* entities, err := s3.NewBucketObjectv2(ctx, "entities", nil)
* if err != nil {
* return err
* }
* _, err = comprehend.NewEntityRecognizer(ctx, "example", &comprehend.EntityRecognizerArgs{
* Name: pulumi.String("example"),
* DataAccessRoleArn: pulumi.Any(exampleAwsIamRole.Arn),
* LanguageCode: pulumi.String("en"),
* InputDataConfig: &comprehend.EntityRecognizerInputDataConfigArgs{
* EntityTypes: comprehend.EntityRecognizerInputDataConfigEntityTypeArray{
* &comprehend.EntityRecognizerInputDataConfigEntityTypeArgs{
* Type: pulumi.String("ENTITY_1"),
* },
* &comprehend.EntityRecognizerInputDataConfigEntityTypeArgs{
* Type: pulumi.String("ENTITY_2"),
* },
* },
* Documents: &comprehend.EntityRecognizerInputDataConfigDocumentsArgs{
* S3Uri: documents.ID().ApplyT(func(id string) (string, error) {
* return fmt.Sprintf("s3://%v/%v", documentsAwsS3Bucket.Bucket, id), nil
* }).(pulumi.StringOutput),
* },
* EntityList: &comprehend.EntityRecognizerInputDataConfigEntityListArgs{
* S3Uri: entities.ID().ApplyT(func(id string) (string, error) {
* return fmt.Sprintf("s3://%v/%v", entitiesAwsS3Bucket.Bucket, id), nil
* }).(pulumi.StringOutput),
* },
* },
* }, pulumi.DependsOn([]pulumi.Resource{
* exampleAwsIamRolePolicy,
* }))
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketObjectv2;
* import com.pulumi.aws.comprehend.EntityRecognizer;
* import com.pulumi.aws.comprehend.EntityRecognizerArgs;
* import com.pulumi.aws.comprehend.inputs.EntityRecognizerInputDataConfigArgs;
* import com.pulumi.aws.comprehend.inputs.EntityRecognizerInputDataConfigDocumentsArgs;
* import com.pulumi.aws.comprehend.inputs.EntityRecognizerInputDataConfigEntityListArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var documents = new BucketObjectv2("documents");
* var entities = new BucketObjectv2("entities");
* var example = new EntityRecognizer("example", EntityRecognizerArgs.builder()
* .name("example")
* .dataAccessRoleArn(exampleAwsIamRole.arn())
* .languageCode("en")
* .inputDataConfig(EntityRecognizerInputDataConfigArgs.builder()
* .entityTypes(
* EntityRecognizerInputDataConfigEntityTypeArgs.builder()
* .type("ENTITY_1")
* .build(),
* EntityRecognizerInputDataConfigEntityTypeArgs.builder()
* .type("ENTITY_2")
* .build())
* .documents(EntityRecognizerInputDataConfigDocumentsArgs.builder()
* .s3Uri(documents.id().applyValue(id -> String.format("s3://%s/%s", documentsAwsS3Bucket.bucket(),id)))
* .build())
* .entityList(EntityRecognizerInputDataConfigEntityListArgs.builder()
* .s3Uri(entities.id().applyValue(id -> String.format("s3://%s/%s", entitiesAwsS3Bucket.bucket(),id)))
* .build())
* .build())
* .build(), CustomResourceOptions.builder()
* .dependsOn(exampleAwsIamRolePolicy)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:comprehend:EntityRecognizer
* properties:
* name: example
* dataAccessRoleArn: ${exampleAwsIamRole.arn}
* languageCode: en
* inputDataConfig:
* entityTypes:
* - type: ENTITY_1
* - type: ENTITY_2
* documents:
* s3Uri: s3://${documentsAwsS3Bucket.bucket}/${documents.id}
* entityList:
* s3Uri: s3://${entitiesAwsS3Bucket.bucket}/${entities.id}
* options:
* dependson:
* - ${exampleAwsIamRolePolicy}
* documents:
* type: aws:s3:BucketObjectv2
* entities:
* type: aws:s3:BucketObjectv2
* ```
*
* ## Import
* Using `pulumi import`, import Comprehend Entity Recognizer using the ARN. For example:
* ```sh
* $ pulumi import aws:comprehend/entityRecognizer:EntityRecognizer example arn:aws:comprehend:us-west-2:123456789012:entity-recognizer/example
* ```
*/
public class EntityRecognizer internal constructor(
override val javaResource: com.pulumi.aws.comprehend.EntityRecognizer,
) : KotlinCustomResource(javaResource, EntityRecognizerMapper) {
/**
* ARN of the Entity Recognizer version.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* The ARN for an IAM Role which allows Comprehend to read the training and testing data.
*/
public val dataAccessRoleArn: Output
get() = javaResource.dataAccessRoleArn().applyValue({ args0 -> args0 })
/**
* Configuration for the training and testing data.
* See the `input_data_config` Configuration Block section below.
*/
public val inputDataConfig: Output
get() = javaResource.inputDataConfig().applyValue({ args0 ->
args0.let({ args0 ->
entityRecognizerInputDataConfigToKotlin(args0)
})
})
/**
* Two-letter language code for the language.
* One of `en`, `es`, `fr`, `it`, `de`, or `pt`.
*/
public val languageCode: Output
get() = javaResource.languageCode().applyValue({ args0 -> args0 })
/**
* The ID or ARN of a KMS Key used to encrypt trained Entity Recognizers.
*/
public val modelKmsKeyId: Output?
get() = javaResource.modelKmsKeyId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Name for the Entity Recognizer.
* Has a maximum length of 63 characters.
* Can contain upper- and lower-case letters, numbers, and hypen (`-`).
* The following arguments are optional:
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* A map of tags to assign to the resource. If configured with a provider `default_tags` Configuration Block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy