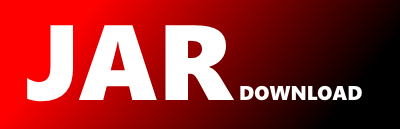
com.pulumi.aws.connect.kotlin.inputs.RoutingProfileQueueConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.connect.kotlin.inputs
import com.pulumi.aws.connect.inputs.RoutingProfileQueueConfigArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property channel Specifies the channels agents can handle in the Contact Control Panel (CCP) for this routing profile. Valid values are `VOICE`, `CHAT`, `TASK`.
* @property delay Specifies the delay, in seconds, that a contact should be in the queue before they are routed to an available agent
* @property priority Specifies the order in which contacts are to be handled for the queue.
* @property queueArn ARN for the queue.
* @property queueId Specifies the identifier for the queue.
* @property queueName Name for the queue.
*/
public data class RoutingProfileQueueConfigArgs(
public val channel: Output,
public val delay: Output,
public val priority: Output,
public val queueArn: Output? = null,
public val queueId: Output,
public val queueName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.connect.inputs.RoutingProfileQueueConfigArgs =
com.pulumi.aws.connect.inputs.RoutingProfileQueueConfigArgs.builder()
.channel(channel.applyValue({ args0 -> args0 }))
.delay(delay.applyValue({ args0 -> args0 }))
.priority(priority.applyValue({ args0 -> args0 }))
.queueArn(queueArn?.applyValue({ args0 -> args0 }))
.queueId(queueId.applyValue({ args0 -> args0 }))
.queueName(queueName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RoutingProfileQueueConfigArgs].
*/
@PulumiTagMarker
public class RoutingProfileQueueConfigArgsBuilder internal constructor() {
private var channel: Output? = null
private var delay: Output? = null
private var priority: Output? = null
private var queueArn: Output? = null
private var queueId: Output? = null
private var queueName: Output? = null
/**
* @param value Specifies the channels agents can handle in the Contact Control Panel (CCP) for this routing profile. Valid values are `VOICE`, `CHAT`, `TASK`.
*/
@JvmName("qseaiprxokopkrek")
public suspend fun channel(`value`: Output) {
this.channel = value
}
/**
* @param value Specifies the delay, in seconds, that a contact should be in the queue before they are routed to an available agent
*/
@JvmName("rgcvhduxqikisthu")
public suspend fun delay(`value`: Output) {
this.delay = value
}
/**
* @param value Specifies the order in which contacts are to be handled for the queue.
*/
@JvmName("effuumydohabuekk")
public suspend fun priority(`value`: Output) {
this.priority = value
}
/**
* @param value ARN for the queue.
*/
@JvmName("ovacopbfsykotgys")
public suspend fun queueArn(`value`: Output) {
this.queueArn = value
}
/**
* @param value Specifies the identifier for the queue.
*/
@JvmName("qdoowoynbfemdmmm")
public suspend fun queueId(`value`: Output) {
this.queueId = value
}
/**
* @param value Name for the queue.
*/
@JvmName("mxjihbxllbsxxpsu")
public suspend fun queueName(`value`: Output) {
this.queueName = value
}
/**
* @param value Specifies the channels agents can handle in the Contact Control Panel (CCP) for this routing profile. Valid values are `VOICE`, `CHAT`, `TASK`.
*/
@JvmName("yqeqgcrlodekvidb")
public suspend fun channel(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.channel = mapped
}
/**
* @param value Specifies the delay, in seconds, that a contact should be in the queue before they are routed to an available agent
*/
@JvmName("buudlcyjlfgqvwon")
public suspend fun delay(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.delay = mapped
}
/**
* @param value Specifies the order in which contacts are to be handled for the queue.
*/
@JvmName("pcpumgromknsoicl")
public suspend fun priority(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.priority = mapped
}
/**
* @param value ARN for the queue.
*/
@JvmName("dpnuourajyxencje")
public suspend fun queueArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.queueArn = mapped
}
/**
* @param value Specifies the identifier for the queue.
*/
@JvmName("ojbbkmwajjicomyc")
public suspend fun queueId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.queueId = mapped
}
/**
* @param value Name for the queue.
*/
@JvmName("yroitsliyuubfibs")
public suspend fun queueName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.queueName = mapped
}
internal fun build(): RoutingProfileQueueConfigArgs = RoutingProfileQueueConfigArgs(
channel = channel ?: throw PulumiNullFieldException("channel"),
delay = delay ?: throw PulumiNullFieldException("delay"),
priority = priority ?: throw PulumiNullFieldException("priority"),
queueArn = queueArn,
queueId = queueId ?: throw PulumiNullFieldException("queueId"),
queueName = queueName,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy