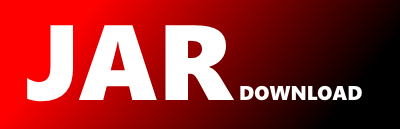
com.pulumi.aws.connect.kotlin.inputs.UserHierarchyStructureHierarchyStructureArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.connect.kotlin.inputs
import com.pulumi.aws.connect.inputs.UserHierarchyStructureHierarchyStructureArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property levelFive A block that defines the details of level five. The level block is documented below.
* Each level block supports the following arguments:
* @property levelFour A block that defines the details of level four. The level block is documented below.
* @property levelOne A block that defines the details of level one. The level block is documented below.
* @property levelThree A block that defines the details of level three. The level block is documented below.
* @property levelTwo A block that defines the details of level two. The level block is documented below.
*/
public data class UserHierarchyStructureHierarchyStructureArgs(
public val levelFive: Output? = null,
public val levelFour: Output? = null,
public val levelOne: Output? = null,
public val levelThree: Output? = null,
public val levelTwo: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.connect.inputs.UserHierarchyStructureHierarchyStructureArgs = com.pulumi.aws.connect.inputs.UserHierarchyStructureHierarchyStructureArgs.builder()
.levelFive(levelFive?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.levelFour(levelFour?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.levelOne(levelOne?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.levelThree(levelThree?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.levelTwo(levelTwo?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [UserHierarchyStructureHierarchyStructureArgs].
*/
@PulumiTagMarker
public class UserHierarchyStructureHierarchyStructureArgsBuilder internal constructor() {
private var levelFive: Output? = null
private var levelFour: Output? = null
private var levelOne: Output? = null
private var levelThree: Output? = null
private var levelTwo: Output? = null
/**
* @param value A block that defines the details of level five. The level block is documented below.
* Each level block supports the following arguments:
*/
@JvmName("mdjujxadoyhxyoyl")
public suspend fun levelFive(`value`: Output) {
this.levelFive = value
}
/**
* @param value A block that defines the details of level four. The level block is documented below.
*/
@JvmName("cgofiesgxvauocsc")
public suspend fun levelFour(`value`: Output) {
this.levelFour = value
}
/**
* @param value A block that defines the details of level one. The level block is documented below.
*/
@JvmName("dcgtwmepdaolksfl")
public suspend fun levelOne(`value`: Output) {
this.levelOne = value
}
/**
* @param value A block that defines the details of level three. The level block is documented below.
*/
@JvmName("evjviscsurwbciho")
public suspend fun levelThree(`value`: Output) {
this.levelThree = value
}
/**
* @param value A block that defines the details of level two. The level block is documented below.
*/
@JvmName("ropwlmxkjdwjvlqm")
public suspend fun levelTwo(`value`: Output) {
this.levelTwo = value
}
/**
* @param value A block that defines the details of level five. The level block is documented below.
* Each level block supports the following arguments:
*/
@JvmName("kehuluxecvljdmgr")
public suspend fun levelFive(`value`: UserHierarchyStructureHierarchyStructureLevelFiveArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.levelFive = mapped
}
/**
* @param argument A block that defines the details of level five. The level block is documented below.
* Each level block supports the following arguments:
*/
@JvmName("nhyveonqwsyfsyvu")
public suspend fun levelFive(argument: suspend UserHierarchyStructureHierarchyStructureLevelFiveArgsBuilder.() -> Unit) {
val toBeMapped = UserHierarchyStructureHierarchyStructureLevelFiveArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.levelFive = mapped
}
/**
* @param value A block that defines the details of level four. The level block is documented below.
*/
@JvmName("iacvibfdfchtbvsn")
public suspend fun levelFour(`value`: UserHierarchyStructureHierarchyStructureLevelFourArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.levelFour = mapped
}
/**
* @param argument A block that defines the details of level four. The level block is documented below.
*/
@JvmName("mjlevhhdqetpvegg")
public suspend fun levelFour(argument: suspend UserHierarchyStructureHierarchyStructureLevelFourArgsBuilder.() -> Unit) {
val toBeMapped = UserHierarchyStructureHierarchyStructureLevelFourArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.levelFour = mapped
}
/**
* @param value A block that defines the details of level one. The level block is documented below.
*/
@JvmName("dtrprfdukkhxqtte")
public suspend fun levelOne(`value`: UserHierarchyStructureHierarchyStructureLevelOneArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.levelOne = mapped
}
/**
* @param argument A block that defines the details of level one. The level block is documented below.
*/
@JvmName("bfkeyhwkbsyvweda")
public suspend fun levelOne(argument: suspend UserHierarchyStructureHierarchyStructureLevelOneArgsBuilder.() -> Unit) {
val toBeMapped = UserHierarchyStructureHierarchyStructureLevelOneArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.levelOne = mapped
}
/**
* @param value A block that defines the details of level three. The level block is documented below.
*/
@JvmName("iyjmducskoomricy")
public suspend fun levelThree(`value`: UserHierarchyStructureHierarchyStructureLevelThreeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.levelThree = mapped
}
/**
* @param argument A block that defines the details of level three. The level block is documented below.
*/
@JvmName("jokvsvbynvygwbgn")
public suspend fun levelThree(argument: suspend UserHierarchyStructureHierarchyStructureLevelThreeArgsBuilder.() -> Unit) {
val toBeMapped = UserHierarchyStructureHierarchyStructureLevelThreeArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.levelThree = mapped
}
/**
* @param value A block that defines the details of level two. The level block is documented below.
*/
@JvmName("tennsvthsqkphups")
public suspend fun levelTwo(`value`: UserHierarchyStructureHierarchyStructureLevelTwoArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.levelTwo = mapped
}
/**
* @param argument A block that defines the details of level two. The level block is documented below.
*/
@JvmName("fhykfshwgdqgtdra")
public suspend fun levelTwo(argument: suspend UserHierarchyStructureHierarchyStructureLevelTwoArgsBuilder.() -> Unit) {
val toBeMapped = UserHierarchyStructureHierarchyStructureLevelTwoArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.levelTwo = mapped
}
internal fun build(): UserHierarchyStructureHierarchyStructureArgs =
UserHierarchyStructureHierarchyStructureArgs(
levelFive = levelFive,
levelFour = levelFour,
levelOne = levelOne,
levelThree = levelThree,
levelTwo = levelTwo,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy