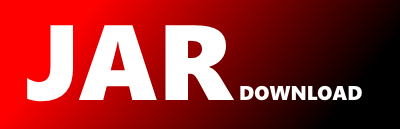
com.pulumi.aws.controltower.kotlin.ControlTowerControlArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.controltower.kotlin
import com.pulumi.aws.controltower.ControlTowerControlArgs.builder
import com.pulumi.aws.controltower.kotlin.inputs.ControlTowerControlParameterArgs
import com.pulumi.aws.controltower.kotlin.inputs.ControlTowerControlParameterArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Allows the application of pre-defined controls to organizational units. For more information on usage, please see the
* [AWS Control Tower User Guide](https://docs.aws.amazon.com/controltower/latest/userguide/enable-guardrails.html).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const current = aws.getRegion({});
* const example = aws.organizations.getOrganization({});
* const exampleGetOrganizationalUnits = example.then(example => aws.organizations.getOrganizationalUnits({
* parentId: example.roots?.[0]?.id,
* }));
* const exampleControlTowerControl = new aws.controltower.ControlTowerControl("example", {
* controlIdentifier: current.then(current => `arn:aws:controltower:${current.name}::control/AWS-GR_EC2_VOLUME_INUSE_CHECK`),
* targetIdentifier: exampleGetOrganizationalUnits.then(exampleGetOrganizationalUnits => .filter(x => x.name == "Infrastructure").map(x => (x.arn))[0]),
* parameters: [{
* key: "AllowedRegions",
* value: JSON.stringify(["us-east-1"]),
* }],
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_aws as aws
* current = aws.get_region()
* example = aws.organizations.get_organization()
* example_get_organizational_units = aws.organizations.get_organizational_units(parent_id=example.roots[0].id)
* example_control_tower_control = aws.controltower.ControlTowerControl("example",
* control_identifier=f"arn:aws:controltower:{current.name}::control/AWS-GR_EC2_VOLUME_INUSE_CHECK",
* target_identifier=[x.arn for x in example_get_organizational_units.children if x.name == "Infrastructure"][0],
* parameters=[{
* "key": "AllowedRegions",
* "value": json.dumps(["us-east-1"]),
* }])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var current = Aws.GetRegion.Invoke();
* var example = Aws.Organizations.GetOrganization.Invoke();
* var exampleGetOrganizationalUnits = Aws.Organizations.GetOrganizationalUnits.Invoke(new()
* {
* ParentId = example.Apply(getOrganizationResult => getOrganizationResult.Roots[0]?.Id),
* });
* var exampleControlTowerControl = new Aws.ControlTower.ControlTowerControl("example", new()
* {
* ControlIdentifier = $"arn:aws:controltower:{current.Apply(getRegionResult => getRegionResult.Name)}::control/AWS-GR_EC2_VOLUME_INUSE_CHECK",
* TargetIdentifier = .Where(x => x.Name == "Infrastructure").Select(x =>
* {
* return x.Arn;
* }).ToList()[0],
* Parameters = new[]
* {
* new Aws.ControlTower.Inputs.ControlTowerControlParameterArgs
* {
* Key = "AllowedRegions",
* Value = JsonSerializer.Serialize(new[]
* {
* "us-east-1",
* }),
* },
* },
* });
* });
* ```
*
* ## Import
* Using `pulumi import`, import Control Tower Controls using their `organizational_unit_arn/control_identifier`. For example:
* ```sh
* $ pulumi import aws:controltower/controlTowerControl:ControlTowerControl example arn:aws:organizations::123456789101:ou/o-qqaejywet/ou-qg5o-ufbhdtv3,arn:aws:controltower:us-east-1::control/WTDSMKDKDNLE
* ```
* @property controlIdentifier The ARN of the control. Only Strongly recommended and Elective controls are permitted, with the exception of the Region deny guardrail.
* @property parameters Parameter values which are specified to configure the control when you enable it. See Parameters for more details.
* @property targetIdentifier The ARN of the organizational unit.
* The following arguments are optional:
*/
public data class ControlTowerControlArgs(
public val controlIdentifier: Output? = null,
public val parameters: Output>? = null,
public val targetIdentifier: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.controltower.ControlTowerControlArgs =
com.pulumi.aws.controltower.ControlTowerControlArgs.builder()
.controlIdentifier(controlIdentifier?.applyValue({ args0 -> args0 }))
.parameters(
parameters?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.targetIdentifier(targetIdentifier?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ControlTowerControlArgs].
*/
@PulumiTagMarker
public class ControlTowerControlArgsBuilder internal constructor() {
private var controlIdentifier: Output? = null
private var parameters: Output>? = null
private var targetIdentifier: Output? = null
/**
* @param value The ARN of the control. Only Strongly recommended and Elective controls are permitted, with the exception of the Region deny guardrail.
*/
@JvmName("avlhoceathsrhuck")
public suspend fun controlIdentifier(`value`: Output) {
this.controlIdentifier = value
}
/**
* @param value Parameter values which are specified to configure the control when you enable it. See Parameters for more details.
*/
@JvmName("xyntcghkdthmujli")
public suspend fun parameters(`value`: Output>) {
this.parameters = value
}
@JvmName("komtmmbwggkxtdgk")
public suspend fun parameters(vararg values: Output) {
this.parameters = Output.all(values.asList())
}
/**
* @param values Parameter values which are specified to configure the control when you enable it. See Parameters for more details.
*/
@JvmName("aryfnorwlkfxiffd")
public suspend fun parameters(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy