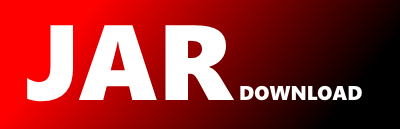
com.pulumi.aws.customerprofiles.kotlin.inputs.ProfileShippingAddressArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.customerprofiles.kotlin.inputs
import com.pulumi.aws.customerprofiles.inputs.ProfileShippingAddressArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property address1 The first line of a customer address.
* @property address2 The second line of a customer address.
* @property address3 The third line of a customer address.
* @property address4 The fourth line of a customer address.
* @property city The city in which a customer lives.
* @property country The country in which a customer lives.
* @property county The county in which a customer lives.
* @property postalCode The postal code of a customer address.
* @property province The province in which a customer lives.
* @property state The state in which a customer lives.
*/
public data class ProfileShippingAddressArgs(
public val address1: Output? = null,
public val address2: Output? = null,
public val address3: Output? = null,
public val address4: Output? = null,
public val city: Output? = null,
public val country: Output? = null,
public val county: Output? = null,
public val postalCode: Output? = null,
public val province: Output? = null,
public val state: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.customerprofiles.inputs.ProfileShippingAddressArgs =
com.pulumi.aws.customerprofiles.inputs.ProfileShippingAddressArgs.builder()
.address1(address1?.applyValue({ args0 -> args0 }))
.address2(address2?.applyValue({ args0 -> args0 }))
.address3(address3?.applyValue({ args0 -> args0 }))
.address4(address4?.applyValue({ args0 -> args0 }))
.city(city?.applyValue({ args0 -> args0 }))
.country(country?.applyValue({ args0 -> args0 }))
.county(county?.applyValue({ args0 -> args0 }))
.postalCode(postalCode?.applyValue({ args0 -> args0 }))
.province(province?.applyValue({ args0 -> args0 }))
.state(state?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ProfileShippingAddressArgs].
*/
@PulumiTagMarker
public class ProfileShippingAddressArgsBuilder internal constructor() {
private var address1: Output? = null
private var address2: Output? = null
private var address3: Output? = null
private var address4: Output? = null
private var city: Output? = null
private var country: Output? = null
private var county: Output? = null
private var postalCode: Output? = null
private var province: Output? = null
private var state: Output? = null
/**
* @param value The first line of a customer address.
*/
@JvmName("hlhcmfgjrjrhogmq")
public suspend fun address1(`value`: Output) {
this.address1 = value
}
/**
* @param value The second line of a customer address.
*/
@JvmName("vocptqeqgudpykde")
public suspend fun address2(`value`: Output) {
this.address2 = value
}
/**
* @param value The third line of a customer address.
*/
@JvmName("ahlfhjdpybaftrio")
public suspend fun address3(`value`: Output) {
this.address3 = value
}
/**
* @param value The fourth line of a customer address.
*/
@JvmName("qcyhmsnjwavvaatv")
public suspend fun address4(`value`: Output) {
this.address4 = value
}
/**
* @param value The city in which a customer lives.
*/
@JvmName("ksoprdiiibenccft")
public suspend fun city(`value`: Output) {
this.city = value
}
/**
* @param value The country in which a customer lives.
*/
@JvmName("kigkpvlvivhnccbs")
public suspend fun country(`value`: Output) {
this.country = value
}
/**
* @param value The county in which a customer lives.
*/
@JvmName("ocpygllmxxpqgttj")
public suspend fun county(`value`: Output) {
this.county = value
}
/**
* @param value The postal code of a customer address.
*/
@JvmName("xwxhvygowrksexgm")
public suspend fun postalCode(`value`: Output) {
this.postalCode = value
}
/**
* @param value The province in which a customer lives.
*/
@JvmName("qgobnrqkinycbwmm")
public suspend fun province(`value`: Output) {
this.province = value
}
/**
* @param value The state in which a customer lives.
*/
@JvmName("tsvwfdvlbkvkvkdo")
public suspend fun state(`value`: Output) {
this.state = value
}
/**
* @param value The first line of a customer address.
*/
@JvmName("mffedkkdjbcfhgbe")
public suspend fun address1(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.address1 = mapped
}
/**
* @param value The second line of a customer address.
*/
@JvmName("vgvbehlpynuxpeql")
public suspend fun address2(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.address2 = mapped
}
/**
* @param value The third line of a customer address.
*/
@JvmName("nrpalrosqbhdvuni")
public suspend fun address3(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.address3 = mapped
}
/**
* @param value The fourth line of a customer address.
*/
@JvmName("jjeqrmhepnheqfps")
public suspend fun address4(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.address4 = mapped
}
/**
* @param value The city in which a customer lives.
*/
@JvmName("oaduaspslypwviiu")
public suspend fun city(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.city = mapped
}
/**
* @param value The country in which a customer lives.
*/
@JvmName("qoylrwjdtxdnjywg")
public suspend fun country(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.country = mapped
}
/**
* @param value The county in which a customer lives.
*/
@JvmName("mwcqpgtrdqqofyav")
public suspend fun county(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.county = mapped
}
/**
* @param value The postal code of a customer address.
*/
@JvmName("nakefmmwumghcwrg")
public suspend fun postalCode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.postalCode = mapped
}
/**
* @param value The province in which a customer lives.
*/
@JvmName("iosoyklnionhbmke")
public suspend fun province(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.province = mapped
}
/**
* @param value The state in which a customer lives.
*/
@JvmName("kiwuaildksxbxsfi")
public suspend fun state(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.state = mapped
}
internal fun build(): ProfileShippingAddressArgs = ProfileShippingAddressArgs(
address1 = address1,
address2 = address2,
address3 = address3,
address4 = address4,
city = city,
country = country,
county = county,
postalCode = postalCode,
province = province,
state = state,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy