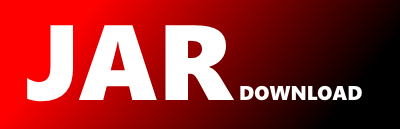
com.pulumi.aws.devopsguru.kotlin.ServiceIntegrationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.devopsguru.kotlin
import com.pulumi.aws.devopsguru.ServiceIntegrationArgs.builder
import com.pulumi.aws.devopsguru.kotlin.inputs.ServiceIntegrationKmsServerSideEncryptionArgs
import com.pulumi.aws.devopsguru.kotlin.inputs.ServiceIntegrationKmsServerSideEncryptionArgsBuilder
import com.pulumi.aws.devopsguru.kotlin.inputs.ServiceIntegrationLogsAnomalyDetectionArgs
import com.pulumi.aws.devopsguru.kotlin.inputs.ServiceIntegrationLogsAnomalyDetectionArgsBuilder
import com.pulumi.aws.devopsguru.kotlin.inputs.ServiceIntegrationOpsCenterArgs
import com.pulumi.aws.devopsguru.kotlin.inputs.ServiceIntegrationOpsCenterArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.devopsguru.ServiceIntegration("example", {
* kmsServerSideEncryption: {
* optInStatus: "ENABLED",
* type: "AWS_OWNED_KMS_KEY",
* },
* logsAnomalyDetection: {
* optInStatus: "ENABLED",
* },
* opsCenter: {
* optInStatus: "ENABLED",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.devopsguru.ServiceIntegration("example",
* kms_server_side_encryption={
* "opt_in_status": "ENABLED",
* "type": "AWS_OWNED_KMS_KEY",
* },
* logs_anomaly_detection={
* "opt_in_status": "ENABLED",
* },
* ops_center={
* "opt_in_status": "ENABLED",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.DevOpsGuru.ServiceIntegration("example", new()
* {
* KmsServerSideEncryption = new Aws.DevOpsGuru.Inputs.ServiceIntegrationKmsServerSideEncryptionArgs
* {
* OptInStatus = "ENABLED",
* Type = "AWS_OWNED_KMS_KEY",
* },
* LogsAnomalyDetection = new Aws.DevOpsGuru.Inputs.ServiceIntegrationLogsAnomalyDetectionArgs
* {
* OptInStatus = "ENABLED",
* },
* OpsCenter = new Aws.DevOpsGuru.Inputs.ServiceIntegrationOpsCenterArgs
* {
* OptInStatus = "ENABLED",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/devopsguru"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := devopsguru.NewServiceIntegration(ctx, "example", &devopsguru.ServiceIntegrationArgs{
* KmsServerSideEncryption: &devopsguru.ServiceIntegrationKmsServerSideEncryptionArgs{
* OptInStatus: pulumi.String("ENABLED"),
* Type: pulumi.String("AWS_OWNED_KMS_KEY"),
* },
* LogsAnomalyDetection: &devopsguru.ServiceIntegrationLogsAnomalyDetectionArgs{
* OptInStatus: pulumi.String("ENABLED"),
* },
* OpsCenter: &devopsguru.ServiceIntegrationOpsCenterArgs{
* OptInStatus: pulumi.String("ENABLED"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.devopsguru.ServiceIntegration;
* import com.pulumi.aws.devopsguru.ServiceIntegrationArgs;
* import com.pulumi.aws.devopsguru.inputs.ServiceIntegrationKmsServerSideEncryptionArgs;
* import com.pulumi.aws.devopsguru.inputs.ServiceIntegrationLogsAnomalyDetectionArgs;
* import com.pulumi.aws.devopsguru.inputs.ServiceIntegrationOpsCenterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ServiceIntegration("example", ServiceIntegrationArgs.builder()
* .kmsServerSideEncryption(ServiceIntegrationKmsServerSideEncryptionArgs.builder()
* .optInStatus("ENABLED")
* .type("AWS_OWNED_KMS_KEY")
* .build())
* .logsAnomalyDetection(ServiceIntegrationLogsAnomalyDetectionArgs.builder()
* .optInStatus("ENABLED")
* .build())
* .opsCenter(ServiceIntegrationOpsCenterArgs.builder()
* .optInStatus("ENABLED")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:devopsguru:ServiceIntegration
* properties:
* kmsServerSideEncryption:
* optInStatus: ENABLED
* type: AWS_OWNED_KMS_KEY
* logsAnomalyDetection:
* optInStatus: ENABLED
* opsCenter:
* optInStatus: ENABLED
* ```
*
* ### Customer Managed KMS Key
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.kms.Key("example", {});
* const exampleServiceIntegration = new aws.devopsguru.ServiceIntegration("example", {
* kmsServerSideEncryption: {
* kmsKeyId: test.arn,
* optInStatus: "ENABLED",
* type: "CUSTOMER_MANAGED_KEY",
* },
* logsAnomalyDetection: {
* optInStatus: "DISABLED",
* },
* opsCenter: {
* optInStatus: "DISABLED",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.kms.Key("example")
* example_service_integration = aws.devopsguru.ServiceIntegration("example",
* kms_server_side_encryption={
* "kms_key_id": test["arn"],
* "opt_in_status": "ENABLED",
* "type": "CUSTOMER_MANAGED_KEY",
* },
* logs_anomaly_detection={
* "opt_in_status": "DISABLED",
* },
* ops_center={
* "opt_in_status": "DISABLED",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Kms.Key("example");
* var exampleServiceIntegration = new Aws.DevOpsGuru.ServiceIntegration("example", new()
* {
* KmsServerSideEncryption = new Aws.DevOpsGuru.Inputs.ServiceIntegrationKmsServerSideEncryptionArgs
* {
* KmsKeyId = test.Arn,
* OptInStatus = "ENABLED",
* Type = "CUSTOMER_MANAGED_KEY",
* },
* LogsAnomalyDetection = new Aws.DevOpsGuru.Inputs.ServiceIntegrationLogsAnomalyDetectionArgs
* {
* OptInStatus = "DISABLED",
* },
* OpsCenter = new Aws.DevOpsGuru.Inputs.ServiceIntegrationOpsCenterArgs
* {
* OptInStatus = "DISABLED",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/devopsguru"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/kms"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := kms.NewKey(ctx, "example", nil)
* if err != nil {
* return err
* }
* _, err = devopsguru.NewServiceIntegration(ctx, "example", &devopsguru.ServiceIntegrationArgs{
* KmsServerSideEncryption: &devopsguru.ServiceIntegrationKmsServerSideEncryptionArgs{
* KmsKeyId: pulumi.Any(test.Arn),
* OptInStatus: pulumi.String("ENABLED"),
* Type: pulumi.String("CUSTOMER_MANAGED_KEY"),
* },
* LogsAnomalyDetection: &devopsguru.ServiceIntegrationLogsAnomalyDetectionArgs{
* OptInStatus: pulumi.String("DISABLED"),
* },
* OpsCenter: &devopsguru.ServiceIntegrationOpsCenterArgs{
* OptInStatus: pulumi.String("DISABLED"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kms.Key;
* import com.pulumi.aws.devopsguru.ServiceIntegration;
* import com.pulumi.aws.devopsguru.ServiceIntegrationArgs;
* import com.pulumi.aws.devopsguru.inputs.ServiceIntegrationKmsServerSideEncryptionArgs;
* import com.pulumi.aws.devopsguru.inputs.ServiceIntegrationLogsAnomalyDetectionArgs;
* import com.pulumi.aws.devopsguru.inputs.ServiceIntegrationOpsCenterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Key("example");
* var exampleServiceIntegration = new ServiceIntegration("exampleServiceIntegration", ServiceIntegrationArgs.builder()
* .kmsServerSideEncryption(ServiceIntegrationKmsServerSideEncryptionArgs.builder()
* .kmsKeyId(test.arn())
* .optInStatus("ENABLED")
* .type("CUSTOMER_MANAGED_KEY")
* .build())
* .logsAnomalyDetection(ServiceIntegrationLogsAnomalyDetectionArgs.builder()
* .optInStatus("DISABLED")
* .build())
* .opsCenter(ServiceIntegrationOpsCenterArgs.builder()
* .optInStatus("DISABLED")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:kms:Key
* exampleServiceIntegration:
* type: aws:devopsguru:ServiceIntegration
* name: example
* properties:
* kmsServerSideEncryption:
* kmsKeyId: ${test.arn}
* optInStatus: ENABLED
* type: CUSTOMER_MANAGED_KEY
* logsAnomalyDetection:
* optInStatus: DISABLED
* opsCenter:
* optInStatus: DISABLED
* ```
*
* ## Import
* Using `pulumi import`, import DevOps Guru Service Integration using the `id`. For example:
* ```sh
* $ pulumi import aws:devopsguru/serviceIntegration:ServiceIntegration example us-east-1
* ```
* @property kmsServerSideEncryption Information about whether DevOps Guru is configured to encrypt server-side data using KMS. See `kms_server_side_encryption` below.
* @property logsAnomalyDetection Information about whether DevOps Guru is configured to perform log anomaly detection on Amazon CloudWatch log groups. See `logs_anomaly_detection` below.
* @property opsCenter Information about whether DevOps Guru is configured to create an OpsItem in AWS Systems Manager OpsCenter for each created insight. See `ops_center` below.
*/
public data class ServiceIntegrationArgs(
public val kmsServerSideEncryption: Output? = null,
public val logsAnomalyDetection: Output? = null,
public val opsCenter: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.devopsguru.ServiceIntegrationArgs =
com.pulumi.aws.devopsguru.ServiceIntegrationArgs.builder()
.kmsServerSideEncryption(
kmsServerSideEncryption?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.logsAnomalyDetection(
logsAnomalyDetection?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.opsCenter(opsCenter?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ServiceIntegrationArgs].
*/
@PulumiTagMarker
public class ServiceIntegrationArgsBuilder internal constructor() {
private var kmsServerSideEncryption: Output? = null
private var logsAnomalyDetection: Output? = null
private var opsCenter: Output? = null
/**
* @param value Information about whether DevOps Guru is configured to encrypt server-side data using KMS. See `kms_server_side_encryption` below.
*/
@JvmName("rxbgeeikunekgayn")
public suspend fun kmsServerSideEncryption(`value`: Output) {
this.kmsServerSideEncryption = value
}
/**
* @param value Information about whether DevOps Guru is configured to perform log anomaly detection on Amazon CloudWatch log groups. See `logs_anomaly_detection` below.
*/
@JvmName("ergwkfmcmfnkixiu")
public suspend fun logsAnomalyDetection(`value`: Output) {
this.logsAnomalyDetection = value
}
/**
* @param value Information about whether DevOps Guru is configured to create an OpsItem in AWS Systems Manager OpsCenter for each created insight. See `ops_center` below.
*/
@JvmName("smiyoavenirljecx")
public suspend fun opsCenter(`value`: Output) {
this.opsCenter = value
}
/**
* @param value Information about whether DevOps Guru is configured to encrypt server-side data using KMS. See `kms_server_side_encryption` below.
*/
@JvmName("ijfkfqtiiksxndfk")
public suspend fun kmsServerSideEncryption(`value`: ServiceIntegrationKmsServerSideEncryptionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kmsServerSideEncryption = mapped
}
/**
* @param argument Information about whether DevOps Guru is configured to encrypt server-side data using KMS. See `kms_server_side_encryption` below.
*/
@JvmName("twdawtrdsahrnrxh")
public suspend fun kmsServerSideEncryption(argument: suspend ServiceIntegrationKmsServerSideEncryptionArgsBuilder.() -> Unit) {
val toBeMapped = ServiceIntegrationKmsServerSideEncryptionArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.kmsServerSideEncryption = mapped
}
/**
* @param value Information about whether DevOps Guru is configured to perform log anomaly detection on Amazon CloudWatch log groups. See `logs_anomaly_detection` below.
*/
@JvmName("viwudxmxclsfnvvl")
public suspend fun logsAnomalyDetection(`value`: ServiceIntegrationLogsAnomalyDetectionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.logsAnomalyDetection = mapped
}
/**
* @param argument Information about whether DevOps Guru is configured to perform log anomaly detection on Amazon CloudWatch log groups. See `logs_anomaly_detection` below.
*/
@JvmName("kodjawkddggbojxs")
public suspend fun logsAnomalyDetection(argument: suspend ServiceIntegrationLogsAnomalyDetectionArgsBuilder.() -> Unit) {
val toBeMapped = ServiceIntegrationLogsAnomalyDetectionArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.logsAnomalyDetection = mapped
}
/**
* @param value Information about whether DevOps Guru is configured to create an OpsItem in AWS Systems Manager OpsCenter for each created insight. See `ops_center` below.
*/
@JvmName("epfjmrvnpscugkaj")
public suspend fun opsCenter(`value`: ServiceIntegrationOpsCenterArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.opsCenter = mapped
}
/**
* @param argument Information about whether DevOps Guru is configured to create an OpsItem in AWS Systems Manager OpsCenter for each created insight. See `ops_center` below.
*/
@JvmName("wpwtdkavkvvysgen")
public suspend fun opsCenter(argument: suspend ServiceIntegrationOpsCenterArgsBuilder.() -> Unit) {
val toBeMapped = ServiceIntegrationOpsCenterArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.opsCenter = mapped
}
internal fun build(): ServiceIntegrationArgs = ServiceIntegrationArgs(
kmsServerSideEncryption = kmsServerSideEncryption,
logsAnomalyDetection = logsAnomalyDetection,
opsCenter = opsCenter,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy