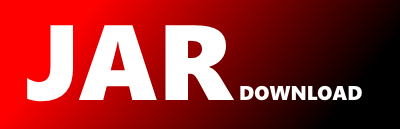
com.pulumi.aws.directconnect.kotlin.MacsecKeyAssociationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.directconnect.kotlin
import com.pulumi.aws.directconnect.MacsecKeyAssociationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Provides a MAC Security (MACSec) secret key resource for use with Direct Connect. See [MACsec prerequisites](https://docs.aws.amazon.com/directconnect/latest/UserGuide/direct-connect-mac-sec-getting-started.html#mac-sec-prerequisites) for information about MAC Security (MACsec) prerequisites.
* Creating this resource will also create a resource of type `aws.secretsmanager.Secret` which is managed by Direct Connect. While you can import this resource into your state, because this secret is managed by Direct Connect, you will not be able to make any modifications to it. See [How AWS Direct Connect uses AWS Secrets Manager](https://docs.aws.amazon.com/secretsmanager/latest/userguide/integrating_how-services-use-secrets_directconnect.html) for details.
* > **Note:** All arguments including `ckn` and `cak` will be stored in the raw state as plain-text.
* > **Note:** The `secret_arn` argument can only be used to reference a previously created MACSec key. You cannot associate a Secrets Manager secret created outside of the `aws.directconnect.MacsecKeyAssociation` resource.
* ## Example Usage
* ### Create MACSec key with CKN and CAK
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = aws.directconnect.getConnection({
* name: "tf-dx-connection",
* });
* const test = new aws.directconnect.MacsecKeyAssociation("test", {
* connectionId: example.then(example => example.id),
* ckn: "0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcdef",
* cak: "abcdef0123456789abcdef0123456789abcdef0123456789abcdef0123456789",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.directconnect.get_connection(name="tf-dx-connection")
* test = aws.directconnect.MacsecKeyAssociation("test",
* connection_id=example.id,
* ckn="0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcdef",
* cak="abcdef0123456789abcdef0123456789abcdef0123456789abcdef0123456789")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = Aws.DirectConnect.GetConnection.Invoke(new()
* {
* Name = "tf-dx-connection",
* });
* var test = new Aws.DirectConnect.MacsecKeyAssociation("test", new()
* {
* ConnectionId = example.Apply(getConnectionResult => getConnectionResult.Id),
* Ckn = "0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcdef",
* Cak = "abcdef0123456789abcdef0123456789abcdef0123456789abcdef0123456789",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/directconnect"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := directconnect.LookupConnection(ctx, &directconnect.LookupConnectionArgs{
* Name: "tf-dx-connection",
* }, nil)
* if err != nil {
* return err
* }
* _, err = directconnect.NewMacsecKeyAssociation(ctx, "test", &directconnect.MacsecKeyAssociationArgs{
* ConnectionId: pulumi.String(example.Id),
* Ckn: pulumi.String("0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcdef"),
* Cak: pulumi.String("abcdef0123456789abcdef0123456789abcdef0123456789abcdef0123456789"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.directconnect.DirectconnectFunctions;
* import com.pulumi.aws.directconnect.inputs.GetConnectionArgs;
* import com.pulumi.aws.directconnect.MacsecKeyAssociation;
* import com.pulumi.aws.directconnect.MacsecKeyAssociationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = DirectconnectFunctions.getConnection(GetConnectionArgs.builder()
* .name("tf-dx-connection")
* .build());
* var test = new MacsecKeyAssociation("test", MacsecKeyAssociationArgs.builder()
* .connectionId(example.applyValue(getConnectionResult -> getConnectionResult.id()))
* .ckn("0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcdef")
* .cak("abcdef0123456789abcdef0123456789abcdef0123456789abcdef0123456789")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: aws:directconnect:MacsecKeyAssociation
* properties:
* connectionId: ${example.id}
* ckn: 0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcdef
* cak: abcdef0123456789abcdef0123456789abcdef0123456789abcdef0123456789
* variables:
* example:
* fn::invoke:
* Function: aws:directconnect:getConnection
* Arguments:
* name: tf-dx-connection
* ```
*
* ### Create MACSec key with existing Secrets Manager secret
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = aws.directconnect.getConnection({
* name: "tf-dx-connection",
* });
* const exampleGetSecret = aws.secretsmanager.getSecret({
* name: "directconnect!prod/us-east-1/directconnect/0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcdef",
* });
* const test = new aws.directconnect.MacsecKeyAssociation("test", {
* connectionId: example.then(example => example.id),
* secretArn: exampleGetSecret.then(exampleGetSecret => exampleGetSecret.arn),
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.directconnect.get_connection(name="tf-dx-connection")
* example_get_secret = aws.secretsmanager.get_secret(name="directconnect!prod/us-east-1/directconnect/0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcdef")
* test = aws.directconnect.MacsecKeyAssociation("test",
* connection_id=example.id,
* secret_arn=example_get_secret.arn)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = Aws.DirectConnect.GetConnection.Invoke(new()
* {
* Name = "tf-dx-connection",
* });
* var exampleGetSecret = Aws.SecretsManager.GetSecret.Invoke(new()
* {
* Name = "directconnect!prod/us-east-1/directconnect/0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcdef",
* });
* var test = new Aws.DirectConnect.MacsecKeyAssociation("test", new()
* {
* ConnectionId = example.Apply(getConnectionResult => getConnectionResult.Id),
* SecretArn = exampleGetSecret.Apply(getSecretResult => getSecretResult.Arn),
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/directconnect"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/secretsmanager"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := directconnect.LookupConnection(ctx, &directconnect.LookupConnectionArgs{
* Name: "tf-dx-connection",
* }, nil)
* if err != nil {
* return err
* }
* exampleGetSecret, err := secretsmanager.LookupSecret(ctx, &secretsmanager.LookupSecretArgs{
* Name: pulumi.StringRef("directconnect!prod/us-east-1/directconnect/0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcdef"),
* }, nil)
* if err != nil {
* return err
* }
* _, err = directconnect.NewMacsecKeyAssociation(ctx, "test", &directconnect.MacsecKeyAssociationArgs{
* ConnectionId: pulumi.String(example.Id),
* SecretArn: pulumi.String(exampleGetSecret.Arn),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.directconnect.DirectconnectFunctions;
* import com.pulumi.aws.directconnect.inputs.GetConnectionArgs;
* import com.pulumi.aws.secretsmanager.SecretsmanagerFunctions;
* import com.pulumi.aws.secretsmanager.inputs.GetSecretArgs;
* import com.pulumi.aws.directconnect.MacsecKeyAssociation;
* import com.pulumi.aws.directconnect.MacsecKeyAssociationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = DirectconnectFunctions.getConnection(GetConnectionArgs.builder()
* .name("tf-dx-connection")
* .build());
* final var exampleGetSecret = SecretsmanagerFunctions.getSecret(GetSecretArgs.builder()
* .name("directconnect!prod/us-east-1/directconnect/0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcdef")
* .build());
* var test = new MacsecKeyAssociation("test", MacsecKeyAssociationArgs.builder()
* .connectionId(example.applyValue(getConnectionResult -> getConnectionResult.id()))
* .secretArn(exampleGetSecret.applyValue(getSecretResult -> getSecretResult.arn()))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: aws:directconnect:MacsecKeyAssociation
* properties:
* connectionId: ${example.id}
* secretArn: ${exampleGetSecret.arn}
* variables:
* example:
* fn::invoke:
* Function: aws:directconnect:getConnection
* Arguments:
* name: tf-dx-connection
* exampleGetSecret:
* fn::invoke:
* Function: aws:secretsmanager:getSecret
* Arguments:
* name: directconnect!prod/us-east-1/directconnect/0123456789abcdef0123456789abcdef0123456789abcdef0123456789abcdef
* ```
*
* @property cak The MAC Security (MACsec) CAK to associate with the dedicated connection. The valid values are 64 hexadecimal characters (0-9, A-E). Required if using `ckn`.
* @property ckn The MAC Security (MACsec) CKN to associate with the dedicated connection. The valid values are 64 hexadecimal characters (0-9, A-E). Required if using `cak`.
* @property connectionId The ID of the dedicated Direct Connect connection. The connection must be a dedicated connection in the `AVAILABLE` state.
* @property secretArn The Amazon Resource Name (ARN) of the MAC Security (MACsec) secret key to associate with the dedicated connection.
* > **Note:** `ckn` and `cak` are mutually exclusive with `secret_arn` - these arguments cannot be used together. If you use `ckn` and `cak`, you should not use `secret_arn`. If you use the `secret_arn` argument to reference an existing MAC Security (MACSec) secret key, you should not use `ckn` or `cak`.
*/
public data class MacsecKeyAssociationArgs(
public val cak: Output? = null,
public val ckn: Output? = null,
public val connectionId: Output? = null,
public val secretArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.directconnect.MacsecKeyAssociationArgs =
com.pulumi.aws.directconnect.MacsecKeyAssociationArgs.builder()
.cak(cak?.applyValue({ args0 -> args0 }))
.ckn(ckn?.applyValue({ args0 -> args0 }))
.connectionId(connectionId?.applyValue({ args0 -> args0 }))
.secretArn(secretArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [MacsecKeyAssociationArgs].
*/
@PulumiTagMarker
public class MacsecKeyAssociationArgsBuilder internal constructor() {
private var cak: Output? = null
private var ckn: Output? = null
private var connectionId: Output? = null
private var secretArn: Output? = null
/**
* @param value The MAC Security (MACsec) CAK to associate with the dedicated connection. The valid values are 64 hexadecimal characters (0-9, A-E). Required if using `ckn`.
*/
@JvmName("nafqnsfkgfsgsutq")
public suspend fun cak(`value`: Output) {
this.cak = value
}
/**
* @param value The MAC Security (MACsec) CKN to associate with the dedicated connection. The valid values are 64 hexadecimal characters (0-9, A-E). Required if using `cak`.
*/
@JvmName("oikeqmgtucitmfbj")
public suspend fun ckn(`value`: Output) {
this.ckn = value
}
/**
* @param value The ID of the dedicated Direct Connect connection. The connection must be a dedicated connection in the `AVAILABLE` state.
*/
@JvmName("toefqmdouesuokqy")
public suspend fun connectionId(`value`: Output) {
this.connectionId = value
}
/**
* @param value The Amazon Resource Name (ARN) of the MAC Security (MACsec) secret key to associate with the dedicated connection.
* > **Note:** `ckn` and `cak` are mutually exclusive with `secret_arn` - these arguments cannot be used together. If you use `ckn` and `cak`, you should not use `secret_arn`. If you use the `secret_arn` argument to reference an existing MAC Security (MACSec) secret key, you should not use `ckn` or `cak`.
*/
@JvmName("budltrwobmsxspwa")
public suspend fun secretArn(`value`: Output) {
this.secretArn = value
}
/**
* @param value The MAC Security (MACsec) CAK to associate with the dedicated connection. The valid values are 64 hexadecimal characters (0-9, A-E). Required if using `ckn`.
*/
@JvmName("lgulgcxfgphvolcq")
public suspend fun cak(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cak = mapped
}
/**
* @param value The MAC Security (MACsec) CKN to associate with the dedicated connection. The valid values are 64 hexadecimal characters (0-9, A-E). Required if using `cak`.
*/
@JvmName("qbvynhkfshnlkdlw")
public suspend fun ckn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ckn = mapped
}
/**
* @param value The ID of the dedicated Direct Connect connection. The connection must be a dedicated connection in the `AVAILABLE` state.
*/
@JvmName("temyocsowyasiktn")
public suspend fun connectionId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectionId = mapped
}
/**
* @param value The Amazon Resource Name (ARN) of the MAC Security (MACsec) secret key to associate with the dedicated connection.
* > **Note:** `ckn` and `cak` are mutually exclusive with `secret_arn` - these arguments cannot be used together. If you use `ckn` and `cak`, you should not use `secret_arn`. If you use the `secret_arn` argument to reference an existing MAC Security (MACSec) secret key, you should not use `ckn` or `cak`.
*/
@JvmName("jcddctcogyiipgux")
public suspend fun secretArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secretArn = mapped
}
internal fun build(): MacsecKeyAssociationArgs = MacsecKeyAssociationArgs(
cak = cak,
ckn = ckn,
connectionId = connectionId,
secretArn = secretArn,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy