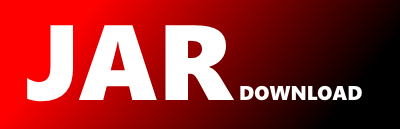
com.pulumi.aws.dlm.kotlin.inputs.LifecyclePolicyPolicyDetailsScheduleCreateRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.dlm.kotlin.inputs
import com.pulumi.aws.dlm.inputs.LifecyclePolicyPolicyDetailsScheduleCreateRuleArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property cronExpression The schedule, as a Cron expression. The schedule interval must be between 1 hour and 1 year. Conflicts with `interval`, `interval_unit`, and `times`.
* @property interval
* @property intervalUnit
* @property location Specifies the destination for snapshots created by the policy. To create snapshots in the same Region as the source resource, specify `CLOUD`. To create snapshots on the same Outpost as the source resource, specify `OUTPOST_LOCAL`. If you omit this parameter, `CLOUD` is used by default. If the policy targets resources in an AWS Region, then you must create snapshots in the same Region as the source resource. If the policy targets resources on an Outpost, then you can create snapshots on the same Outpost as the source resource, or in the Region of that Outpost. Valid values are `CLOUD` and `OUTPOST_LOCAL`.
* @property times A list of times in 24 hour clock format that sets when the lifecycle policy should be evaluated. Max of 1. Conflicts with `cron_expression`. Must be set if `interval` is set.
*/
public data class LifecyclePolicyPolicyDetailsScheduleCreateRuleArgs(
public val cronExpression: Output? = null,
public val interval: Output? = null,
public val intervalUnit: Output? = null,
public val location: Output? = null,
public val times: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.dlm.inputs.LifecyclePolicyPolicyDetailsScheduleCreateRuleArgs =
com.pulumi.aws.dlm.inputs.LifecyclePolicyPolicyDetailsScheduleCreateRuleArgs.builder()
.cronExpression(cronExpression?.applyValue({ args0 -> args0 }))
.interval(interval?.applyValue({ args0 -> args0 }))
.intervalUnit(intervalUnit?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.times(times?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LifecyclePolicyPolicyDetailsScheduleCreateRuleArgs].
*/
@PulumiTagMarker
public class LifecyclePolicyPolicyDetailsScheduleCreateRuleArgsBuilder internal constructor() {
private var cronExpression: Output? = null
private var interval: Output? = null
private var intervalUnit: Output? = null
private var location: Output? = null
private var times: Output? = null
/**
* @param value The schedule, as a Cron expression. The schedule interval must be between 1 hour and 1 year. Conflicts with `interval`, `interval_unit`, and `times`.
*/
@JvmName("rgqslmvkhfncdiit")
public suspend fun cronExpression(`value`: Output) {
this.cronExpression = value
}
/**
* @param value
*/
@JvmName("mlbjkwxchflvdpmv")
public suspend fun interval(`value`: Output) {
this.interval = value
}
/**
* @param value
*/
@JvmName("tqfpfkwouhfaiyyu")
public suspend fun intervalUnit(`value`: Output) {
this.intervalUnit = value
}
/**
* @param value Specifies the destination for snapshots created by the policy. To create snapshots in the same Region as the source resource, specify `CLOUD`. To create snapshots on the same Outpost as the source resource, specify `OUTPOST_LOCAL`. If you omit this parameter, `CLOUD` is used by default. If the policy targets resources in an AWS Region, then you must create snapshots in the same Region as the source resource. If the policy targets resources on an Outpost, then you can create snapshots on the same Outpost as the source resource, or in the Region of that Outpost. Valid values are `CLOUD` and `OUTPOST_LOCAL`.
*/
@JvmName("xjqbtjhdcssrmbdr")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value A list of times in 24 hour clock format that sets when the lifecycle policy should be evaluated. Max of 1. Conflicts with `cron_expression`. Must be set if `interval` is set.
*/
@JvmName("prakqulafhvhordg")
public suspend fun times(`value`: Output) {
this.times = value
}
/**
* @param value The schedule, as a Cron expression. The schedule interval must be between 1 hour and 1 year. Conflicts with `interval`, `interval_unit`, and `times`.
*/
@JvmName("gckfpdlttqcgqkcu")
public suspend fun cronExpression(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cronExpression = mapped
}
/**
* @param value
*/
@JvmName("vfekrcrxygchausc")
public suspend fun interval(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.interval = mapped
}
/**
* @param value
*/
@JvmName("rxtvusxjsmkvkwka")
public suspend fun intervalUnit(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.intervalUnit = mapped
}
/**
* @param value Specifies the destination for snapshots created by the policy. To create snapshots in the same Region as the source resource, specify `CLOUD`. To create snapshots on the same Outpost as the source resource, specify `OUTPOST_LOCAL`. If you omit this parameter, `CLOUD` is used by default. If the policy targets resources in an AWS Region, then you must create snapshots in the same Region as the source resource. If the policy targets resources on an Outpost, then you can create snapshots on the same Outpost as the source resource, or in the Region of that Outpost. Valid values are `CLOUD` and `OUTPOST_LOCAL`.
*/
@JvmName("ickcybupxoavydoj")
public suspend fun location(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.location = mapped
}
/**
* @param value A list of times in 24 hour clock format that sets when the lifecycle policy should be evaluated. Max of 1. Conflicts with `cron_expression`. Must be set if `interval` is set.
*/
@JvmName("sifscsfgjlourvym")
public suspend fun times(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.times = mapped
}
internal fun build(): LifecyclePolicyPolicyDetailsScheduleCreateRuleArgs =
LifecyclePolicyPolicyDetailsScheduleCreateRuleArgs(
cronExpression = cronExpression,
interval = interval,
intervalUnit = intervalUnit,
location = location,
times = times,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy