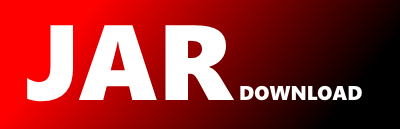
com.pulumi.aws.dms.kotlin.ReplicationConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.dms.kotlin
import com.pulumi.aws.dms.ReplicationConfigArgs.builder
import com.pulumi.aws.dms.kotlin.inputs.ReplicationConfigComputeConfigArgs
import com.pulumi.aws.dms.kotlin.inputs.ReplicationConfigComputeConfigArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Provides a DMS Serverless replication config resource.
* > **NOTE:** Changing most arguments will stop the replication if it is running. You can set `start_replication` to resume the replication afterwards.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const name = new aws.dms.ReplicationConfig("name", {
* replicationConfigIdentifier: "test-dms-serverless-replication-tf",
* resourceIdentifier: "test-dms-serverless-replication-tf",
* replicationType: "cdc",
* sourceEndpointArn: source.endpointArn,
* targetEndpointArn: target.endpointArn,
* tableMappings: ` {
* "rules":[{"rule-type":"selection","rule-id":"1","rule-name":"1","rule-action":"include","object-locator":{"schema-name":"%%","table-name":"%%"}}]
* }
* `,
* startReplication: true,
* computeConfig: {
* replicationSubnetGroupId: _default.replicationSubnetGroupId,
* maxCapacityUnits: 64,
* minCapacityUnits: 2,
* preferredMaintenanceWindow: "sun:23:45-mon:00:30",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* name = aws.dms.ReplicationConfig("name",
* replication_config_identifier="test-dms-serverless-replication-tf",
* resource_identifier="test-dms-serverless-replication-tf",
* replication_type="cdc",
* source_endpoint_arn=source["endpointArn"],
* target_endpoint_arn=target["endpointArn"],
* table_mappings=""" {
* "rules":[{"rule-type":"selection","rule-id":"1","rule-name":"1","rule-action":"include","object-locator":{"schema-name":"%%","table-name":"%%"}}]
* }
* """,
* start_replication=True,
* compute_config={
* "replication_subnet_group_id": default["replicationSubnetGroupId"],
* "max_capacity_units": 64,
* "min_capacity_units": 2,
* "preferred_maintenance_window": "sun:23:45-mon:00:30",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var name = new Aws.Dms.ReplicationConfig("name", new()
* {
* ReplicationConfigIdentifier = "test-dms-serverless-replication-tf",
* ResourceIdentifier = "test-dms-serverless-replication-tf",
* ReplicationType = "cdc",
* SourceEndpointArn = source.EndpointArn,
* TargetEndpointArn = target.EndpointArn,
* TableMappings = @" {
* ""rules"":[{""rule-type"":""selection"",""rule-id"":""1"",""rule-name"":""1"",""rule-action"":""include"",""object-locator"":{""schema-name"":""%%"",""table-name"":""%%""}}]
* }
* ",
* StartReplication = true,
* ComputeConfig = new Aws.Dms.Inputs.ReplicationConfigComputeConfigArgs
* {
* ReplicationSubnetGroupId = @default.ReplicationSubnetGroupId,
* MaxCapacityUnits = 64,
* MinCapacityUnits = 2,
* PreferredMaintenanceWindow = "sun:23:45-mon:00:30",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/dms"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := dms.NewReplicationConfig(ctx, "name", &dms.ReplicationConfigArgs{
* ReplicationConfigIdentifier: pulumi.String("test-dms-serverless-replication-tf"),
* ResourceIdentifier: pulumi.String("test-dms-serverless-replication-tf"),
* ReplicationType: pulumi.String("cdc"),
* SourceEndpointArn: pulumi.Any(source.EndpointArn),
* TargetEndpointArn: pulumi.Any(target.EndpointArn),
* TableMappings: pulumi.String(" {\n \"rules\":[{\"rule-type\":\"selection\",\"rule-id\":\"1\",\"rule-name\":\"1\",\"rule-action\":\"include\",\"object-locator\":{\"schema-name\":\"%%\",\"table-name\":\"%%\"}}]\n }\n"),
* StartReplication: pulumi.Bool(true),
* ComputeConfig: &dms.ReplicationConfigComputeConfigArgs{
* ReplicationSubnetGroupId: pulumi.Any(_default.ReplicationSubnetGroupId),
* MaxCapacityUnits: pulumi.Int(64),
* MinCapacityUnits: pulumi.Int(2),
* PreferredMaintenanceWindow: pulumi.String("sun:23:45-mon:00:30"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.dms.ReplicationConfig;
* import com.pulumi.aws.dms.ReplicationConfigArgs;
* import com.pulumi.aws.dms.inputs.ReplicationConfigComputeConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var name = new ReplicationConfig("name", ReplicationConfigArgs.builder()
* .replicationConfigIdentifier("test-dms-serverless-replication-tf")
* .resourceIdentifier("test-dms-serverless-replication-tf")
* .replicationType("cdc")
* .sourceEndpointArn(source.endpointArn())
* .targetEndpointArn(target.endpointArn())
* .tableMappings("""
* {
* "rules":[{"rule-type":"selection","rule-id":"1","rule-name":"1","rule-action":"include","object-locator":{"schema-name":"%%","table-name":"%%"}}]
* }
* """)
* .startReplication(true)
* .computeConfig(ReplicationConfigComputeConfigArgs.builder()
* .replicationSubnetGroupId(default_.replicationSubnetGroupId())
* .maxCapacityUnits("64")
* .minCapacityUnits("2")
* .preferredMaintenanceWindow("sun:23:45-mon:00:30")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* name:
* type: aws:dms:ReplicationConfig
* properties:
* replicationConfigIdentifier: test-dms-serverless-replication-tf
* resourceIdentifier: test-dms-serverless-replication-tf
* replicationType: cdc
* sourceEndpointArn: ${source.endpointArn}
* targetEndpointArn: ${target.endpointArn}
* tableMappings: |2
* {
* "rules":[{"rule-type":"selection","rule-id":"1","rule-name":"1","rule-action":"include","object-locator":{"schema-name":"%%","table-name":"%%"}}]
* }
* startReplication: true
* computeConfig:
* replicationSubnetGroupId: ${default.replicationSubnetGroupId}
* maxCapacityUnits: '64'
* minCapacityUnits: '2'
* preferredMaintenanceWindow: sun:23:45-mon:00:30
* ```
*
* ## Import
* Using `pulumi import`, import a replication config using the `arn`. For example:
* ```sh
* $ pulumi import aws:dms/replicationConfig:ReplicationConfig example arn:aws:dms:us-east-1:123456789012:replication-config:UX6OL6MHMMJKFFOXE3H7LLJCMEKBDUG4ZV7DRSI
* ```
* @property computeConfig Configuration block for provisioning an DMS Serverless replication.
* @property replicationConfigIdentifier Unique identifier that you want to use to create the config.
* @property replicationSettings An escaped JSON string that are used to provision this replication configuration. For example, [Change processing tuning settings](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Tasks.CustomizingTasks.TaskSettings.ChangeProcessingTuning.html)
* @property replicationType The migration type. Can be one of `full-load | cdc | full-load-and-cdc`.
* @property resourceIdentifier Unique value or name that you set for a given resource that can be used to construct an Amazon Resource Name (ARN) for that resource. For more information, see [Fine-grained access control using resource names and tags](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Security.html#CHAP_Security.FineGrainedAccess)
* @property sourceEndpointArn The Amazon Resource Name (ARN) string that uniquely identifies the source endpoint.
* @property startReplication Whether to run or stop the serverless replication, default is false.
* @property supplementalSettings JSON settings for specifying supplemental data. For more information see [Specifying supplemental data for task settings](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Tasks.TaskData.html)
* @property tableMappings An escaped JSON string that contains the table mappings. For information on table mapping see [Using Table Mapping with an AWS Database Migration Service Task to Select and Filter Data](http://docs.aws.amazon.com/dms/latest/userguide/CHAP_Tasks.CustomizingTasks.TableMapping.html)
* @property tags A map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
* @property targetEndpointArn The Amazon Resource Name (ARN) string that uniquely identifies the target endpoint.
*/
public data class ReplicationConfigArgs(
public val computeConfig: Output? = null,
public val replicationConfigIdentifier: Output? = null,
public val replicationSettings: Output? = null,
public val replicationType: Output? = null,
public val resourceIdentifier: Output? = null,
public val sourceEndpointArn: Output? = null,
public val startReplication: Output? = null,
public val supplementalSettings: Output? = null,
public val tableMappings: Output? = null,
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy