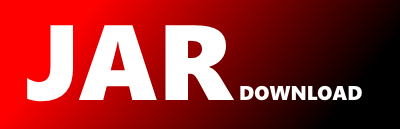
com.pulumi.aws.dms.kotlin.inputs.EndpointKinesisSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.dms.kotlin.inputs
import com.pulumi.aws.dms.inputs.EndpointKinesisSettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property includeControlDetails Shows detailed control information for table definition, column definition, and table and column changes in the Kinesis message output. Default is `false`.
* @property includeNullAndEmpty Include NULL and empty columns in the target. Default is `false`.
* @property includePartitionValue Shows the partition value within the Kinesis message output, unless the partition type is schema-table-type. Default is `false`.
* @property includeTableAlterOperations Includes any data definition language (DDL) operations that change the table in the control data. Default is `false`.
* @property includeTransactionDetails Provides detailed transaction information from the source database. Default is `false`.
* @property messageFormat Output format for the records created. Default is `json`. Valid values are `json` and `json-unformatted` (a single line with no tab).
* @property partitionIncludeSchemaTable Prefixes schema and table names to partition values, when the partition type is primary-key-type. Default is `false`.
* @property serviceAccessRoleArn ARN of the IAM Role with permissions to write to the Kinesis data stream.
* @property streamArn ARN of the Kinesis data stream.
*/
public data class EndpointKinesisSettingsArgs(
public val includeControlDetails: Output? = null,
public val includeNullAndEmpty: Output? = null,
public val includePartitionValue: Output? = null,
public val includeTableAlterOperations: Output? = null,
public val includeTransactionDetails: Output? = null,
public val messageFormat: Output? = null,
public val partitionIncludeSchemaTable: Output? = null,
public val serviceAccessRoleArn: Output? = null,
public val streamArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.dms.inputs.EndpointKinesisSettingsArgs =
com.pulumi.aws.dms.inputs.EndpointKinesisSettingsArgs.builder()
.includeControlDetails(includeControlDetails?.applyValue({ args0 -> args0 }))
.includeNullAndEmpty(includeNullAndEmpty?.applyValue({ args0 -> args0 }))
.includePartitionValue(includePartitionValue?.applyValue({ args0 -> args0 }))
.includeTableAlterOperations(includeTableAlterOperations?.applyValue({ args0 -> args0 }))
.includeTransactionDetails(includeTransactionDetails?.applyValue({ args0 -> args0 }))
.messageFormat(messageFormat?.applyValue({ args0 -> args0 }))
.partitionIncludeSchemaTable(partitionIncludeSchemaTable?.applyValue({ args0 -> args0 }))
.serviceAccessRoleArn(serviceAccessRoleArn?.applyValue({ args0 -> args0 }))
.streamArn(streamArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EndpointKinesisSettingsArgs].
*/
@PulumiTagMarker
public class EndpointKinesisSettingsArgsBuilder internal constructor() {
private var includeControlDetails: Output? = null
private var includeNullAndEmpty: Output? = null
private var includePartitionValue: Output? = null
private var includeTableAlterOperations: Output? = null
private var includeTransactionDetails: Output? = null
private var messageFormat: Output? = null
private var partitionIncludeSchemaTable: Output? = null
private var serviceAccessRoleArn: Output? = null
private var streamArn: Output? = null
/**
* @param value Shows detailed control information for table definition, column definition, and table and column changes in the Kinesis message output. Default is `false`.
*/
@JvmName("kdyborusjtlnaijx")
public suspend fun includeControlDetails(`value`: Output) {
this.includeControlDetails = value
}
/**
* @param value Include NULL and empty columns in the target. Default is `false`.
*/
@JvmName("bgbejtjcjsxauipl")
public suspend fun includeNullAndEmpty(`value`: Output) {
this.includeNullAndEmpty = value
}
/**
* @param value Shows the partition value within the Kinesis message output, unless the partition type is schema-table-type. Default is `false`.
*/
@JvmName("pwbcktoimwvpuquk")
public suspend fun includePartitionValue(`value`: Output) {
this.includePartitionValue = value
}
/**
* @param value Includes any data definition language (DDL) operations that change the table in the control data. Default is `false`.
*/
@JvmName("ceahxmlbaeyxfsln")
public suspend fun includeTableAlterOperations(`value`: Output) {
this.includeTableAlterOperations = value
}
/**
* @param value Provides detailed transaction information from the source database. Default is `false`.
*/
@JvmName("afrpajhmikxsfkch")
public suspend fun includeTransactionDetails(`value`: Output) {
this.includeTransactionDetails = value
}
/**
* @param value Output format for the records created. Default is `json`. Valid values are `json` and `json-unformatted` (a single line with no tab).
*/
@JvmName("nuvpsiqhbeavkakt")
public suspend fun messageFormat(`value`: Output) {
this.messageFormat = value
}
/**
* @param value Prefixes schema and table names to partition values, when the partition type is primary-key-type. Default is `false`.
*/
@JvmName("gkgmyqgkqfwxtrmj")
public suspend fun partitionIncludeSchemaTable(`value`: Output) {
this.partitionIncludeSchemaTable = value
}
/**
* @param value ARN of the IAM Role with permissions to write to the Kinesis data stream.
*/
@JvmName("wpuoberytqipyhgk")
public suspend fun serviceAccessRoleArn(`value`: Output) {
this.serviceAccessRoleArn = value
}
/**
* @param value ARN of the Kinesis data stream.
*/
@JvmName("airkubopjpljtsjq")
public suspend fun streamArn(`value`: Output) {
this.streamArn = value
}
/**
* @param value Shows detailed control information for table definition, column definition, and table and column changes in the Kinesis message output. Default is `false`.
*/
@JvmName("qqnoyxsdkuuqxibb")
public suspend fun includeControlDetails(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.includeControlDetails = mapped
}
/**
* @param value Include NULL and empty columns in the target. Default is `false`.
*/
@JvmName("fecweogiwmejkean")
public suspend fun includeNullAndEmpty(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.includeNullAndEmpty = mapped
}
/**
* @param value Shows the partition value within the Kinesis message output, unless the partition type is schema-table-type. Default is `false`.
*/
@JvmName("rvbtayxoanlbhbbs")
public suspend fun includePartitionValue(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.includePartitionValue = mapped
}
/**
* @param value Includes any data definition language (DDL) operations that change the table in the control data. Default is `false`.
*/
@JvmName("nnlwbhalsyvrkrbh")
public suspend fun includeTableAlterOperations(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.includeTableAlterOperations = mapped
}
/**
* @param value Provides detailed transaction information from the source database. Default is `false`.
*/
@JvmName("pnviqykdovljnldo")
public suspend fun includeTransactionDetails(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.includeTransactionDetails = mapped
}
/**
* @param value Output format for the records created. Default is `json`. Valid values are `json` and `json-unformatted` (a single line with no tab).
*/
@JvmName("pdofgxftkgyibdeh")
public suspend fun messageFormat(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.messageFormat = mapped
}
/**
* @param value Prefixes schema and table names to partition values, when the partition type is primary-key-type. Default is `false`.
*/
@JvmName("nimethinqvblcayh")
public suspend fun partitionIncludeSchemaTable(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.partitionIncludeSchemaTable = mapped
}
/**
* @param value ARN of the IAM Role with permissions to write to the Kinesis data stream.
*/
@JvmName("mllxdbxulkwoildm")
public suspend fun serviceAccessRoleArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceAccessRoleArn = mapped
}
/**
* @param value ARN of the Kinesis data stream.
*/
@JvmName("vyolwosgdojqlmms")
public suspend fun streamArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.streamArn = mapped
}
internal fun build(): EndpointKinesisSettingsArgs = EndpointKinesisSettingsArgs(
includeControlDetails = includeControlDetails,
includeNullAndEmpty = includeNullAndEmpty,
includePartitionValue = includePartitionValue,
includeTableAlterOperations = includeTableAlterOperations,
includeTransactionDetails = includeTransactionDetails,
messageFormat = messageFormat,
partitionIncludeSchemaTable = partitionIncludeSchemaTable,
serviceAccessRoleArn = serviceAccessRoleArn,
streamArn = streamArn,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy