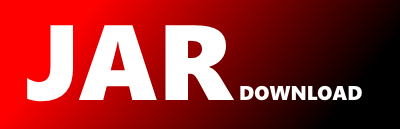
com.pulumi.aws.dms.kotlin.inputs.EndpointPostgresSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.dms.kotlin.inputs
import com.pulumi.aws.dms.inputs.EndpointPostgresSettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property afterConnectScript For use with change data capture (CDC) only, this attribute has AWS DMS bypass foreign keys and user triggers to reduce the time it takes to bulk load data.
* @property babelfishDatabaseName The Babelfish for Aurora PostgreSQL database name for the endpoint.
* @property captureDdls To capture DDL events, AWS DMS creates various artifacts in the PostgreSQL database when the task starts.
* @property databaseMode Specifies the default behavior of the replication's handling of PostgreSQL- compatible endpoints that require some additional configuration, such as Babelfish endpoints.
* @property ddlArtifactsSchema Sets the schema in which the operational DDL database artifacts are created. Default is `public`.
* @property executeTimeout Sets the client statement timeout for the PostgreSQL instance, in seconds. Default value is `60`.
* @property failTasksOnLobTruncation When set to `true`, this value causes a task to fail if the actual size of a LOB column is greater than the specified `LobMaxSize`. Default is `false`.
* @property heartbeatEnable The write-ahead log (WAL) heartbeat feature mimics a dummy transaction. By doing this, it prevents idle logical replication slots from holding onto old WAL logs, which can result in storage full situations on the source.
* @property heartbeatFrequency Sets the WAL heartbeat frequency (in minutes). Default value is `5`.
* @property heartbeatSchema Sets the schema in which the heartbeat artifacts are created. Default value is `public`.
* @property mapBooleanAsBoolean You can use PostgreSQL endpoint settings to map a boolean as a boolean from your PostgreSQL source to a Amazon Redshift target. Default value is `false`.
* @property mapJsonbAsClob Optional When true, DMS migrates JSONB values as CLOB.
* @property mapLongVarcharAs Optional When true, DMS migrates LONG values as VARCHAR.
* @property maxFileSize Specifies the maximum size (in KB) of any .csv file used to transfer data to PostgreSQL. Default is `32,768 KB`.
* @property pluginName Specifies the plugin to use to create a replication slot. Valid values: `pglogical`, `test_decoding`.
* @property slotName Sets the name of a previously created logical replication slot for a CDC load of the PostgreSQL source instance.
*/
public data class EndpointPostgresSettingsArgs(
public val afterConnectScript: Output? = null,
public val babelfishDatabaseName: Output? = null,
public val captureDdls: Output? = null,
public val databaseMode: Output? = null,
public val ddlArtifactsSchema: Output? = null,
public val executeTimeout: Output? = null,
public val failTasksOnLobTruncation: Output? = null,
public val heartbeatEnable: Output? = null,
public val heartbeatFrequency: Output? = null,
public val heartbeatSchema: Output? = null,
public val mapBooleanAsBoolean: Output? = null,
public val mapJsonbAsClob: Output? = null,
public val mapLongVarcharAs: Output? = null,
public val maxFileSize: Output? = null,
public val pluginName: Output? = null,
public val slotName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.dms.inputs.EndpointPostgresSettingsArgs =
com.pulumi.aws.dms.inputs.EndpointPostgresSettingsArgs.builder()
.afterConnectScript(afterConnectScript?.applyValue({ args0 -> args0 }))
.babelfishDatabaseName(babelfishDatabaseName?.applyValue({ args0 -> args0 }))
.captureDdls(captureDdls?.applyValue({ args0 -> args0 }))
.databaseMode(databaseMode?.applyValue({ args0 -> args0 }))
.ddlArtifactsSchema(ddlArtifactsSchema?.applyValue({ args0 -> args0 }))
.executeTimeout(executeTimeout?.applyValue({ args0 -> args0 }))
.failTasksOnLobTruncation(failTasksOnLobTruncation?.applyValue({ args0 -> args0 }))
.heartbeatEnable(heartbeatEnable?.applyValue({ args0 -> args0 }))
.heartbeatFrequency(heartbeatFrequency?.applyValue({ args0 -> args0 }))
.heartbeatSchema(heartbeatSchema?.applyValue({ args0 -> args0 }))
.mapBooleanAsBoolean(mapBooleanAsBoolean?.applyValue({ args0 -> args0 }))
.mapJsonbAsClob(mapJsonbAsClob?.applyValue({ args0 -> args0 }))
.mapLongVarcharAs(mapLongVarcharAs?.applyValue({ args0 -> args0 }))
.maxFileSize(maxFileSize?.applyValue({ args0 -> args0 }))
.pluginName(pluginName?.applyValue({ args0 -> args0 }))
.slotName(slotName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EndpointPostgresSettingsArgs].
*/
@PulumiTagMarker
public class EndpointPostgresSettingsArgsBuilder internal constructor() {
private var afterConnectScript: Output? = null
private var babelfishDatabaseName: Output? = null
private var captureDdls: Output? = null
private var databaseMode: Output? = null
private var ddlArtifactsSchema: Output? = null
private var executeTimeout: Output? = null
private var failTasksOnLobTruncation: Output? = null
private var heartbeatEnable: Output? = null
private var heartbeatFrequency: Output? = null
private var heartbeatSchema: Output? = null
private var mapBooleanAsBoolean: Output? = null
private var mapJsonbAsClob: Output? = null
private var mapLongVarcharAs: Output? = null
private var maxFileSize: Output? = null
private var pluginName: Output? = null
private var slotName: Output? = null
/**
* @param value For use with change data capture (CDC) only, this attribute has AWS DMS bypass foreign keys and user triggers to reduce the time it takes to bulk load data.
*/
@JvmName("vrhxokookkgatxtd")
public suspend fun afterConnectScript(`value`: Output) {
this.afterConnectScript = value
}
/**
* @param value The Babelfish for Aurora PostgreSQL database name for the endpoint.
*/
@JvmName("fjcgkbanlhkksngi")
public suspend fun babelfishDatabaseName(`value`: Output) {
this.babelfishDatabaseName = value
}
/**
* @param value To capture DDL events, AWS DMS creates various artifacts in the PostgreSQL database when the task starts.
*/
@JvmName("uabgsslynltbqdhs")
public suspend fun captureDdls(`value`: Output) {
this.captureDdls = value
}
/**
* @param value Specifies the default behavior of the replication's handling of PostgreSQL- compatible endpoints that require some additional configuration, such as Babelfish endpoints.
*/
@JvmName("lfkeksagtrnjbonx")
public suspend fun databaseMode(`value`: Output) {
this.databaseMode = value
}
/**
* @param value Sets the schema in which the operational DDL database artifacts are created. Default is `public`.
*/
@JvmName("auagqgmlpfnwqfoi")
public suspend fun ddlArtifactsSchema(`value`: Output) {
this.ddlArtifactsSchema = value
}
/**
* @param value Sets the client statement timeout for the PostgreSQL instance, in seconds. Default value is `60`.
*/
@JvmName("cjaooqlexxfsdpby")
public suspend fun executeTimeout(`value`: Output) {
this.executeTimeout = value
}
/**
* @param value When set to `true`, this value causes a task to fail if the actual size of a LOB column is greater than the specified `LobMaxSize`. Default is `false`.
*/
@JvmName("ijyjduaopfkjqgra")
public suspend fun failTasksOnLobTruncation(`value`: Output) {
this.failTasksOnLobTruncation = value
}
/**
* @param value The write-ahead log (WAL) heartbeat feature mimics a dummy transaction. By doing this, it prevents idle logical replication slots from holding onto old WAL logs, which can result in storage full situations on the source.
*/
@JvmName("ocayjnxhpjimoylx")
public suspend fun heartbeatEnable(`value`: Output) {
this.heartbeatEnable = value
}
/**
* @param value Sets the WAL heartbeat frequency (in minutes). Default value is `5`.
*/
@JvmName("odghpwlkbujsvxwe")
public suspend fun heartbeatFrequency(`value`: Output) {
this.heartbeatFrequency = value
}
/**
* @param value Sets the schema in which the heartbeat artifacts are created. Default value is `public`.
*/
@JvmName("scakftpkolhaicvt")
public suspend fun heartbeatSchema(`value`: Output) {
this.heartbeatSchema = value
}
/**
* @param value You can use PostgreSQL endpoint settings to map a boolean as a boolean from your PostgreSQL source to a Amazon Redshift target. Default value is `false`.
*/
@JvmName("rcbtttmmqjttdshx")
public suspend fun mapBooleanAsBoolean(`value`: Output) {
this.mapBooleanAsBoolean = value
}
/**
* @param value Optional When true, DMS migrates JSONB values as CLOB.
*/
@JvmName("qnxubtqtfigrdenc")
public suspend fun mapJsonbAsClob(`value`: Output) {
this.mapJsonbAsClob = value
}
/**
* @param value Optional When true, DMS migrates LONG values as VARCHAR.
*/
@JvmName("tcteusaykyljmptb")
public suspend fun mapLongVarcharAs(`value`: Output) {
this.mapLongVarcharAs = value
}
/**
* @param value Specifies the maximum size (in KB) of any .csv file used to transfer data to PostgreSQL. Default is `32,768 KB`.
*/
@JvmName("ntubapchfkrpwhen")
public suspend fun maxFileSize(`value`: Output) {
this.maxFileSize = value
}
/**
* @param value Specifies the plugin to use to create a replication slot. Valid values: `pglogical`, `test_decoding`.
*/
@JvmName("qbmdmjwmqjddntuj")
public suspend fun pluginName(`value`: Output) {
this.pluginName = value
}
/**
* @param value Sets the name of a previously created logical replication slot for a CDC load of the PostgreSQL source instance.
*/
@JvmName("rpnwxhfqnxilmcpd")
public suspend fun slotName(`value`: Output) {
this.slotName = value
}
/**
* @param value For use with change data capture (CDC) only, this attribute has AWS DMS bypass foreign keys and user triggers to reduce the time it takes to bulk load data.
*/
@JvmName("gukoypibaeymjnrl")
public suspend fun afterConnectScript(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.afterConnectScript = mapped
}
/**
* @param value The Babelfish for Aurora PostgreSQL database name for the endpoint.
*/
@JvmName("nmaxgkwpgyuvmlxi")
public suspend fun babelfishDatabaseName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.babelfishDatabaseName = mapped
}
/**
* @param value To capture DDL events, AWS DMS creates various artifacts in the PostgreSQL database when the task starts.
*/
@JvmName("lrodxoktfomtmwae")
public suspend fun captureDdls(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.captureDdls = mapped
}
/**
* @param value Specifies the default behavior of the replication's handling of PostgreSQL- compatible endpoints that require some additional configuration, such as Babelfish endpoints.
*/
@JvmName("wbmlvvscncxgjjah")
public suspend fun databaseMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.databaseMode = mapped
}
/**
* @param value Sets the schema in which the operational DDL database artifacts are created. Default is `public`.
*/
@JvmName("sdyrkabffunhrunm")
public suspend fun ddlArtifactsSchema(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ddlArtifactsSchema = mapped
}
/**
* @param value Sets the client statement timeout for the PostgreSQL instance, in seconds. Default value is `60`.
*/
@JvmName("xdnsokjttucbkfsu")
public suspend fun executeTimeout(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.executeTimeout = mapped
}
/**
* @param value When set to `true`, this value causes a task to fail if the actual size of a LOB column is greater than the specified `LobMaxSize`. Default is `false`.
*/
@JvmName("xsraxbabrwwjfcrj")
public suspend fun failTasksOnLobTruncation(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failTasksOnLobTruncation = mapped
}
/**
* @param value The write-ahead log (WAL) heartbeat feature mimics a dummy transaction. By doing this, it prevents idle logical replication slots from holding onto old WAL logs, which can result in storage full situations on the source.
*/
@JvmName("idoayjwbwjfslqrk")
public suspend fun heartbeatEnable(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.heartbeatEnable = mapped
}
/**
* @param value Sets the WAL heartbeat frequency (in minutes). Default value is `5`.
*/
@JvmName("dxperjllealibqsf")
public suspend fun heartbeatFrequency(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.heartbeatFrequency = mapped
}
/**
* @param value Sets the schema in which the heartbeat artifacts are created. Default value is `public`.
*/
@JvmName("ccqfppgpyujaisfi")
public suspend fun heartbeatSchema(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.heartbeatSchema = mapped
}
/**
* @param value You can use PostgreSQL endpoint settings to map a boolean as a boolean from your PostgreSQL source to a Amazon Redshift target. Default value is `false`.
*/
@JvmName("mmmteaxqthkktone")
public suspend fun mapBooleanAsBoolean(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mapBooleanAsBoolean = mapped
}
/**
* @param value Optional When true, DMS migrates JSONB values as CLOB.
*/
@JvmName("urlsdtlheqawiaqn")
public suspend fun mapJsonbAsClob(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mapJsonbAsClob = mapped
}
/**
* @param value Optional When true, DMS migrates LONG values as VARCHAR.
*/
@JvmName("jcnnlkykamrjblsa")
public suspend fun mapLongVarcharAs(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mapLongVarcharAs = mapped
}
/**
* @param value Specifies the maximum size (in KB) of any .csv file used to transfer data to PostgreSQL. Default is `32,768 KB`.
*/
@JvmName("xhplyaawpflbmgvm")
public suspend fun maxFileSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxFileSize = mapped
}
/**
* @param value Specifies the plugin to use to create a replication slot. Valid values: `pglogical`, `test_decoding`.
*/
@JvmName("xcwuxvuqwuakvqll")
public suspend fun pluginName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pluginName = mapped
}
/**
* @param value Sets the name of a previously created logical replication slot for a CDC load of the PostgreSQL source instance.
*/
@JvmName("sbfirdksbiokbisg")
public suspend fun slotName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.slotName = mapped
}
internal fun build(): EndpointPostgresSettingsArgs = EndpointPostgresSettingsArgs(
afterConnectScript = afterConnectScript,
babelfishDatabaseName = babelfishDatabaseName,
captureDdls = captureDdls,
databaseMode = databaseMode,
ddlArtifactsSchema = ddlArtifactsSchema,
executeTimeout = executeTimeout,
failTasksOnLobTruncation = failTasksOnLobTruncation,
heartbeatEnable = heartbeatEnable,
heartbeatFrequency = heartbeatFrequency,
heartbeatSchema = heartbeatSchema,
mapBooleanAsBoolean = mapBooleanAsBoolean,
mapJsonbAsClob = mapJsonbAsClob,
mapLongVarcharAs = mapLongVarcharAs,
maxFileSize = maxFileSize,
pluginName = pluginName,
slotName = slotName,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy