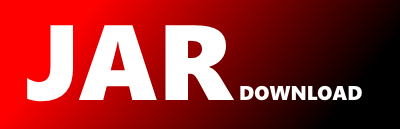
com.pulumi.aws.dms.kotlin.inputs.EndpointS3SettingsArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.dms.kotlin.inputs
import com.pulumi.aws.dms.inputs.EndpointS3SettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property addColumnName Whether to add column name information to the .csv output file. Default is `false`.
* @property bucketFolder S3 object prefix.
* @property bucketName S3 bucket name.
* @property cannedAclForObjects Predefined (canned) access control list for objects created in an S3 bucket. Valid values include `none`, `private`, `public-read`, `public-read-write`, `authenticated-read`, `aws-exec-read`, `bucket-owner-read`, and `bucket-owner-full-control`. Default is `none`.
* @property cdcInsertsAndUpdates Whether to write insert and update operations to .csv or .parquet output files. Default is `false`.
* @property cdcInsertsOnly Whether to write insert operations to .csv or .parquet output files. Default is `false`.
* @property cdcMaxBatchInterval Maximum length of the interval, defined in seconds, after which to output a file to Amazon S3. Default is `60`.
* @property cdcMinFileSize Minimum file size condition as defined in kilobytes to output a file to Amazon S3. Default is `32000`. **NOTE:** Previously, this setting was measured in megabytes but now represents kilobytes. Update configurations accordingly.
* @property cdcPath Folder path of CDC files. For an S3 source, this setting is required if a task captures change data; otherwise, it's optional. If `cdc_path` is set, AWS DMS reads CDC files from this path and replicates the data changes to the target endpoint. Supported in AWS DMS versions 3.4.2 and later.
* @property compressionType Set to compress target files. Default is `NONE`. Valid values are `GZIP` and `NONE`.
* @property csvDelimiter Delimiter used to separate columns in the source files. Default is `,`.
* @property csvNoSupValue String to use for all columns not included in the supplemental log.
* @property csvNullValue String to as null when writing to the target.
* @property csvRowDelimiter Delimiter used to separate rows in the source files. Default is `\n`.
* @property dataFormat Output format for the files that AWS DMS uses to create S3 objects. Valid values are `csv` and `parquet`. Default is `csv`.
* @property dataPageSize Size of one data page in bytes. Default is `1048576` (1 MiB).
* @property datePartitionDelimiter Date separating delimiter to use during folder partitioning. Valid values are `SLASH`, `UNDERSCORE`, `DASH`, and `NONE`. Default is `SLASH`.
* @property datePartitionEnabled Partition S3 bucket folders based on transaction commit dates. Default is `false`.
* @property datePartitionSequence Date format to use during folder partitioning. Use this parameter when `date_partition_enabled` is set to true. Valid values are `YYYYMMDD`, `YYYYMMDDHH`, `YYYYMM`, `MMYYYYDD`, and `DDMMYYYY`. Default is `YYYYMMDD`.
* @property dictPageSizeLimit Maximum size in bytes of an encoded dictionary page of a column. Default is `1048576` (1 MiB).
* @property enableStatistics Whether to enable statistics for Parquet pages and row groups. Default is `true`.
* @property encodingType Type of encoding to use. Value values are `rle_dictionary`, `plain`, and `plain_dictionary`. Default is `rle_dictionary`.
* @property encryptionMode Server-side encryption mode that you want to encrypt your .csv or .parquet object files copied to S3. Valid values are `SSE_S3` and `SSE_KMS`. Default is `SSE_S3`.
* @property externalTableDefinition JSON document that describes how AWS DMS should interpret the data.
* @property glueCatalogGeneration Whether to integrate AWS Glue Data Catalog with an Amazon S3 target. See [Using AWS Glue Data Catalog with an Amazon S3 target for AWS DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.S3.html#CHAP_Target.S3.GlueCatalog) for more information. Default is `false`.
* @property ignoreHeaderRows When this value is set to `1`, DMS ignores the first row header in a .csv file. Default is `0`.
* @property includeOpForFullLoad Whether to enable a full load to write INSERT operations to the .csv output files only to indicate how the rows were added to the source database. Default is `false`.
* @property maxFileSize Maximum size (in KB) of any .csv file to be created while migrating to an S3 target during full load. Valid values are from `1` to `1048576`. Default is `1048576` (1 GB).
* @property parquetTimestampInMillisecond Specifies the precision of any TIMESTAMP column values written to an S3 object file in .parquet format. Default is `false`.
* @property parquetVersion Version of the .parquet file format. Default is `parquet-1-0`. Valid values are `parquet-1-0` and `parquet-2-0`.
* @property preserveTransactions Whether DMS saves the transaction order for a CDC load on the S3 target specified by `cdc_path`. Default is `false`.
* @property rfc4180 For an S3 source, whether each leading double quotation mark has to be followed by an ending double quotation mark. Default is `true`.
* @property rowGroupLength Number of rows in a row group. Default is `10000`.
* @property serverSideEncryptionKmsKeyId ARN or Id of KMS Key to use when `encryption_mode` is `SSE_KMS`.
* @property serviceAccessRoleArn ARN of the IAM Role with permissions to read from or write to the S3 Bucket.
* @property timestampColumnName Column to add with timestamp information to the endpoint data for an Amazon S3 target.
* @property useCsvNoSupValue Whether to use `csv_no_sup_value` for columns not included in the supplemental log.
* @property useTaskStartTimeForFullLoadTimestamp When set to true, uses the task start time as the timestamp column value instead of the time data is written to target. For full load, when set to true, each row of the timestamp column contains the task start time. For CDC loads, each row of the timestamp column contains the transaction commit time. When set to false, the full load timestamp in the timestamp column increments with the time data arrives at the target. Default is `false`.
*/
public data class EndpointS3SettingsArgs(
public val addColumnName: Output? = null,
public val bucketFolder: Output? = null,
public val bucketName: Output? = null,
public val cannedAclForObjects: Output? = null,
public val cdcInsertsAndUpdates: Output? = null,
public val cdcInsertsOnly: Output? = null,
public val cdcMaxBatchInterval: Output? = null,
public val cdcMinFileSize: Output? = null,
public val cdcPath: Output? = null,
public val compressionType: Output? = null,
public val csvDelimiter: Output? = null,
public val csvNoSupValue: Output? = null,
public val csvNullValue: Output? = null,
public val csvRowDelimiter: Output? = null,
public val dataFormat: Output? = null,
public val dataPageSize: Output? = null,
public val datePartitionDelimiter: Output? = null,
public val datePartitionEnabled: Output? = null,
public val datePartitionSequence: Output? = null,
public val dictPageSizeLimit: Output? = null,
public val enableStatistics: Output? = null,
public val encodingType: Output? = null,
public val encryptionMode: Output? = null,
public val externalTableDefinition: Output? = null,
public val glueCatalogGeneration: Output? = null,
public val ignoreHeaderRows: Output? = null,
public val includeOpForFullLoad: Output? = null,
public val maxFileSize: Output? = null,
public val parquetTimestampInMillisecond: Output? = null,
public val parquetVersion: Output? = null,
public val preserveTransactions: Output? = null,
public val rfc4180: Output? = null,
public val rowGroupLength: Output? = null,
public val serverSideEncryptionKmsKeyId: Output? = null,
public val serviceAccessRoleArn: Output? = null,
public val timestampColumnName: Output? = null,
public val useCsvNoSupValue: Output? = null,
public val useTaskStartTimeForFullLoadTimestamp: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.dms.inputs.EndpointS3SettingsArgs =
com.pulumi.aws.dms.inputs.EndpointS3SettingsArgs.builder()
.addColumnName(addColumnName?.applyValue({ args0 -> args0 }))
.bucketFolder(bucketFolder?.applyValue({ args0 -> args0 }))
.bucketName(bucketName?.applyValue({ args0 -> args0 }))
.cannedAclForObjects(cannedAclForObjects?.applyValue({ args0 -> args0 }))
.cdcInsertsAndUpdates(cdcInsertsAndUpdates?.applyValue({ args0 -> args0 }))
.cdcInsertsOnly(cdcInsertsOnly?.applyValue({ args0 -> args0 }))
.cdcMaxBatchInterval(cdcMaxBatchInterval?.applyValue({ args0 -> args0 }))
.cdcMinFileSize(cdcMinFileSize?.applyValue({ args0 -> args0 }))
.cdcPath(cdcPath?.applyValue({ args0 -> args0 }))
.compressionType(compressionType?.applyValue({ args0 -> args0 }))
.csvDelimiter(csvDelimiter?.applyValue({ args0 -> args0 }))
.csvNoSupValue(csvNoSupValue?.applyValue({ args0 -> args0 }))
.csvNullValue(csvNullValue?.applyValue({ args0 -> args0 }))
.csvRowDelimiter(csvRowDelimiter?.applyValue({ args0 -> args0 }))
.dataFormat(dataFormat?.applyValue({ args0 -> args0 }))
.dataPageSize(dataPageSize?.applyValue({ args0 -> args0 }))
.datePartitionDelimiter(datePartitionDelimiter?.applyValue({ args0 -> args0 }))
.datePartitionEnabled(datePartitionEnabled?.applyValue({ args0 -> args0 }))
.datePartitionSequence(datePartitionSequence?.applyValue({ args0 -> args0 }))
.dictPageSizeLimit(dictPageSizeLimit?.applyValue({ args0 -> args0 }))
.enableStatistics(enableStatistics?.applyValue({ args0 -> args0 }))
.encodingType(encodingType?.applyValue({ args0 -> args0 }))
.encryptionMode(encryptionMode?.applyValue({ args0 -> args0 }))
.externalTableDefinition(externalTableDefinition?.applyValue({ args0 -> args0 }))
.glueCatalogGeneration(glueCatalogGeneration?.applyValue({ args0 -> args0 }))
.ignoreHeaderRows(ignoreHeaderRows?.applyValue({ args0 -> args0 }))
.includeOpForFullLoad(includeOpForFullLoad?.applyValue({ args0 -> args0 }))
.maxFileSize(maxFileSize?.applyValue({ args0 -> args0 }))
.parquetTimestampInMillisecond(parquetTimestampInMillisecond?.applyValue({ args0 -> args0 }))
.parquetVersion(parquetVersion?.applyValue({ args0 -> args0 }))
.preserveTransactions(preserveTransactions?.applyValue({ args0 -> args0 }))
.rfc4180(rfc4180?.applyValue({ args0 -> args0 }))
.rowGroupLength(rowGroupLength?.applyValue({ args0 -> args0 }))
.serverSideEncryptionKmsKeyId(serverSideEncryptionKmsKeyId?.applyValue({ args0 -> args0 }))
.serviceAccessRoleArn(serviceAccessRoleArn?.applyValue({ args0 -> args0 }))
.timestampColumnName(timestampColumnName?.applyValue({ args0 -> args0 }))
.useCsvNoSupValue(useCsvNoSupValue?.applyValue({ args0 -> args0 }))
.useTaskStartTimeForFullLoadTimestamp(
useTaskStartTimeForFullLoadTimestamp?.applyValue({ args0 ->
args0
}),
).build()
}
/**
* Builder for [EndpointS3SettingsArgs].
*/
@PulumiTagMarker
public class EndpointS3SettingsArgsBuilder internal constructor() {
private var addColumnName: Output? = null
private var bucketFolder: Output? = null
private var bucketName: Output? = null
private var cannedAclForObjects: Output? = null
private var cdcInsertsAndUpdates: Output? = null
private var cdcInsertsOnly: Output? = null
private var cdcMaxBatchInterval: Output? = null
private var cdcMinFileSize: Output? = null
private var cdcPath: Output? = null
private var compressionType: Output? = null
private var csvDelimiter: Output? = null
private var csvNoSupValue: Output? = null
private var csvNullValue: Output? = null
private var csvRowDelimiter: Output? = null
private var dataFormat: Output? = null
private var dataPageSize: Output? = null
private var datePartitionDelimiter: Output? = null
private var datePartitionEnabled: Output? = null
private var datePartitionSequence: Output? = null
private var dictPageSizeLimit: Output? = null
private var enableStatistics: Output? = null
private var encodingType: Output? = null
private var encryptionMode: Output? = null
private var externalTableDefinition: Output? = null
private var glueCatalogGeneration: Output? = null
private var ignoreHeaderRows: Output? = null
private var includeOpForFullLoad: Output? = null
private var maxFileSize: Output? = null
private var parquetTimestampInMillisecond: Output? = null
private var parquetVersion: Output? = null
private var preserveTransactions: Output? = null
private var rfc4180: Output? = null
private var rowGroupLength: Output? = null
private var serverSideEncryptionKmsKeyId: Output? = null
private var serviceAccessRoleArn: Output? = null
private var timestampColumnName: Output? = null
private var useCsvNoSupValue: Output? = null
private var useTaskStartTimeForFullLoadTimestamp: Output? = null
/**
* @param value Whether to add column name information to the .csv output file. Default is `false`.
*/
@JvmName("aimpmspeeajvnfba")
public suspend fun addColumnName(`value`: Output) {
this.addColumnName = value
}
/**
* @param value S3 object prefix.
*/
@JvmName("qvjgthyrocygapba")
public suspend fun bucketFolder(`value`: Output) {
this.bucketFolder = value
}
/**
* @param value S3 bucket name.
*/
@JvmName("tpgpfqsnlefxcluv")
public suspend fun bucketName(`value`: Output) {
this.bucketName = value
}
/**
* @param value Predefined (canned) access control list for objects created in an S3 bucket. Valid values include `none`, `private`, `public-read`, `public-read-write`, `authenticated-read`, `aws-exec-read`, `bucket-owner-read`, and `bucket-owner-full-control`. Default is `none`.
*/
@JvmName("xvtxggqtdesfunao")
public suspend fun cannedAclForObjects(`value`: Output) {
this.cannedAclForObjects = value
}
/**
* @param value Whether to write insert and update operations to .csv or .parquet output files. Default is `false`.
*/
@JvmName("vipnjthtlscqeoyp")
public suspend fun cdcInsertsAndUpdates(`value`: Output) {
this.cdcInsertsAndUpdates = value
}
/**
* @param value Whether to write insert operations to .csv or .parquet output files. Default is `false`.
*/
@JvmName("xwrkrllmcrijlibp")
public suspend fun cdcInsertsOnly(`value`: Output) {
this.cdcInsertsOnly = value
}
/**
* @param value Maximum length of the interval, defined in seconds, after which to output a file to Amazon S3. Default is `60`.
*/
@JvmName("pgysnaurfmucpqlx")
public suspend fun cdcMaxBatchInterval(`value`: Output) {
this.cdcMaxBatchInterval = value
}
/**
* @param value Minimum file size condition as defined in kilobytes to output a file to Amazon S3. Default is `32000`. **NOTE:** Previously, this setting was measured in megabytes but now represents kilobytes. Update configurations accordingly.
*/
@JvmName("qlkycoltvubqtmkj")
public suspend fun cdcMinFileSize(`value`: Output) {
this.cdcMinFileSize = value
}
/**
* @param value Folder path of CDC files. For an S3 source, this setting is required if a task captures change data; otherwise, it's optional. If `cdc_path` is set, AWS DMS reads CDC files from this path and replicates the data changes to the target endpoint. Supported in AWS DMS versions 3.4.2 and later.
*/
@JvmName("kgbrvyskcuywrany")
public suspend fun cdcPath(`value`: Output) {
this.cdcPath = value
}
/**
* @param value Set to compress target files. Default is `NONE`. Valid values are `GZIP` and `NONE`.
*/
@JvmName("fshbxgenjfxywdie")
public suspend fun compressionType(`value`: Output) {
this.compressionType = value
}
/**
* @param value Delimiter used to separate columns in the source files. Default is `,`.
*/
@JvmName("dpbdxjlnwlpueykb")
public suspend fun csvDelimiter(`value`: Output) {
this.csvDelimiter = value
}
/**
* @param value String to use for all columns not included in the supplemental log.
*/
@JvmName("nlcvxccfuayxwjgp")
public suspend fun csvNoSupValue(`value`: Output) {
this.csvNoSupValue = value
}
/**
* @param value String to as null when writing to the target.
*/
@JvmName("ubethibgkbksycvy")
public suspend fun csvNullValue(`value`: Output) {
this.csvNullValue = value
}
/**
* @param value Delimiter used to separate rows in the source files. Default is `\n`.
*/
@JvmName("vtwsnhhqojeorayr")
public suspend fun csvRowDelimiter(`value`: Output) {
this.csvRowDelimiter = value
}
/**
* @param value Output format for the files that AWS DMS uses to create S3 objects. Valid values are `csv` and `parquet`. Default is `csv`.
*/
@JvmName("wrhsycuekmxclmvk")
public suspend fun dataFormat(`value`: Output) {
this.dataFormat = value
}
/**
* @param value Size of one data page in bytes. Default is `1048576` (1 MiB).
*/
@JvmName("xdcdgyvuoblwwbqd")
public suspend fun dataPageSize(`value`: Output) {
this.dataPageSize = value
}
/**
* @param value Date separating delimiter to use during folder partitioning. Valid values are `SLASH`, `UNDERSCORE`, `DASH`, and `NONE`. Default is `SLASH`.
*/
@JvmName("pjgticxvhuwjmwoe")
public suspend fun datePartitionDelimiter(`value`: Output) {
this.datePartitionDelimiter = value
}
/**
* @param value Partition S3 bucket folders based on transaction commit dates. Default is `false`.
*/
@JvmName("vfpbjwdoqrkkhvbd")
public suspend fun datePartitionEnabled(`value`: Output) {
this.datePartitionEnabled = value
}
/**
* @param value Date format to use during folder partitioning. Use this parameter when `date_partition_enabled` is set to true. Valid values are `YYYYMMDD`, `YYYYMMDDHH`, `YYYYMM`, `MMYYYYDD`, and `DDMMYYYY`. Default is `YYYYMMDD`.
*/
@JvmName("wyqcfsuslrwsxdut")
public suspend fun datePartitionSequence(`value`: Output) {
this.datePartitionSequence = value
}
/**
* @param value Maximum size in bytes of an encoded dictionary page of a column. Default is `1048576` (1 MiB).
*/
@JvmName("yhmrpxpbmncdjjei")
public suspend fun dictPageSizeLimit(`value`: Output) {
this.dictPageSizeLimit = value
}
/**
* @param value Whether to enable statistics for Parquet pages and row groups. Default is `true`.
*/
@JvmName("bjkiidmmmhmunbhc")
public suspend fun enableStatistics(`value`: Output) {
this.enableStatistics = value
}
/**
* @param value Type of encoding to use. Value values are `rle_dictionary`, `plain`, and `plain_dictionary`. Default is `rle_dictionary`.
*/
@JvmName("afilhjrggcuiuogm")
public suspend fun encodingType(`value`: Output) {
this.encodingType = value
}
/**
* @param value Server-side encryption mode that you want to encrypt your .csv or .parquet object files copied to S3. Valid values are `SSE_S3` and `SSE_KMS`. Default is `SSE_S3`.
*/
@JvmName("tmytnafsgrxljrjw")
public suspend fun encryptionMode(`value`: Output) {
this.encryptionMode = value
}
/**
* @param value JSON document that describes how AWS DMS should interpret the data.
*/
@JvmName("ajokmnsatwuiqdtk")
public suspend fun externalTableDefinition(`value`: Output) {
this.externalTableDefinition = value
}
/**
* @param value Whether to integrate AWS Glue Data Catalog with an Amazon S3 target. See [Using AWS Glue Data Catalog with an Amazon S3 target for AWS DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.S3.html#CHAP_Target.S3.GlueCatalog) for more information. Default is `false`.
*/
@JvmName("pbwapixcvpulbenx")
public suspend fun glueCatalogGeneration(`value`: Output) {
this.glueCatalogGeneration = value
}
/**
* @param value When this value is set to `1`, DMS ignores the first row header in a .csv file. Default is `0`.
*/
@JvmName("ocufflucgnnbhnpj")
public suspend fun ignoreHeaderRows(`value`: Output) {
this.ignoreHeaderRows = value
}
/**
* @param value Whether to enable a full load to write INSERT operations to the .csv output files only to indicate how the rows were added to the source database. Default is `false`.
*/
@JvmName("csbecbuxutlnkjym")
public suspend fun includeOpForFullLoad(`value`: Output) {
this.includeOpForFullLoad = value
}
/**
* @param value Maximum size (in KB) of any .csv file to be created while migrating to an S3 target during full load. Valid values are from `1` to `1048576`. Default is `1048576` (1 GB).
*/
@JvmName("lwtigdtuubwtguvi")
public suspend fun maxFileSize(`value`: Output) {
this.maxFileSize = value
}
/**
* @param value Specifies the precision of any TIMESTAMP column values written to an S3 object file in .parquet format. Default is `false`.
*/
@JvmName("bcckveunhpkblyvh")
public suspend fun parquetTimestampInMillisecond(`value`: Output) {
this.parquetTimestampInMillisecond = value
}
/**
* @param value Version of the .parquet file format. Default is `parquet-1-0`. Valid values are `parquet-1-0` and `parquet-2-0`.
*/
@JvmName("yfifvlnsanqgkpmq")
public suspend fun parquetVersion(`value`: Output) {
this.parquetVersion = value
}
/**
* @param value Whether DMS saves the transaction order for a CDC load on the S3 target specified by `cdc_path`. Default is `false`.
*/
@JvmName("qddtbeemtmtriisd")
public suspend fun preserveTransactions(`value`: Output) {
this.preserveTransactions = value
}
/**
* @param value For an S3 source, whether each leading double quotation mark has to be followed by an ending double quotation mark. Default is `true`.
*/
@JvmName("gxtydoqwpygewcgd")
public suspend fun rfc4180(`value`: Output) {
this.rfc4180 = value
}
/**
* @param value Number of rows in a row group. Default is `10000`.
*/
@JvmName("agfcxtypchhhptva")
public suspend fun rowGroupLength(`value`: Output) {
this.rowGroupLength = value
}
/**
* @param value ARN or Id of KMS Key to use when `encryption_mode` is `SSE_KMS`.
*/
@JvmName("njeuywjtfvigfefg")
public suspend fun serverSideEncryptionKmsKeyId(`value`: Output) {
this.serverSideEncryptionKmsKeyId = value
}
/**
* @param value ARN of the IAM Role with permissions to read from or write to the S3 Bucket.
*/
@JvmName("bsddhjhcifurotxk")
public suspend fun serviceAccessRoleArn(`value`: Output) {
this.serviceAccessRoleArn = value
}
/**
* @param value Column to add with timestamp information to the endpoint data for an Amazon S3 target.
*/
@JvmName("tsraorrdjpfwyhoo")
public suspend fun timestampColumnName(`value`: Output) {
this.timestampColumnName = value
}
/**
* @param value Whether to use `csv_no_sup_value` for columns not included in the supplemental log.
*/
@JvmName("angbctnubtvooluh")
public suspend fun useCsvNoSupValue(`value`: Output) {
this.useCsvNoSupValue = value
}
/**
* @param value When set to true, uses the task start time as the timestamp column value instead of the time data is written to target. For full load, when set to true, each row of the timestamp column contains the task start time. For CDC loads, each row of the timestamp column contains the transaction commit time. When set to false, the full load timestamp in the timestamp column increments with the time data arrives at the target. Default is `false`.
*/
@JvmName("qhbtodkvpjfgnjuf")
public suspend fun useTaskStartTimeForFullLoadTimestamp(`value`: Output) {
this.useTaskStartTimeForFullLoadTimestamp = value
}
/**
* @param value Whether to add column name information to the .csv output file. Default is `false`.
*/
@JvmName("kwjwuiniwyqracqa")
public suspend fun addColumnName(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.addColumnName = mapped
}
/**
* @param value S3 object prefix.
*/
@JvmName("dcvpwbffmsnhedqi")
public suspend fun bucketFolder(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bucketFolder = mapped
}
/**
* @param value S3 bucket name.
*/
@JvmName("qqoubxvuaxikmwmv")
public suspend fun bucketName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bucketName = mapped
}
/**
* @param value Predefined (canned) access control list for objects created in an S3 bucket. Valid values include `none`, `private`, `public-read`, `public-read-write`, `authenticated-read`, `aws-exec-read`, `bucket-owner-read`, and `bucket-owner-full-control`. Default is `none`.
*/
@JvmName("nrvjxxrrqvwaaxpr")
public suspend fun cannedAclForObjects(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cannedAclForObjects = mapped
}
/**
* @param value Whether to write insert and update operations to .csv or .parquet output files. Default is `false`.
*/
@JvmName("fakuydxkitlhimdv")
public suspend fun cdcInsertsAndUpdates(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cdcInsertsAndUpdates = mapped
}
/**
* @param value Whether to write insert operations to .csv or .parquet output files. Default is `false`.
*/
@JvmName("lurthroaivuxprao")
public suspend fun cdcInsertsOnly(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cdcInsertsOnly = mapped
}
/**
* @param value Maximum length of the interval, defined in seconds, after which to output a file to Amazon S3. Default is `60`.
*/
@JvmName("imfsojfmwsksqeaq")
public suspend fun cdcMaxBatchInterval(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cdcMaxBatchInterval = mapped
}
/**
* @param value Minimum file size condition as defined in kilobytes to output a file to Amazon S3. Default is `32000`. **NOTE:** Previously, this setting was measured in megabytes but now represents kilobytes. Update configurations accordingly.
*/
@JvmName("vuhnpktvveumjkox")
public suspend fun cdcMinFileSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cdcMinFileSize = mapped
}
/**
* @param value Folder path of CDC files. For an S3 source, this setting is required if a task captures change data; otherwise, it's optional. If `cdc_path` is set, AWS DMS reads CDC files from this path and replicates the data changes to the target endpoint. Supported in AWS DMS versions 3.4.2 and later.
*/
@JvmName("bvefeebiqpgkemfv")
public suspend fun cdcPath(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cdcPath = mapped
}
/**
* @param value Set to compress target files. Default is `NONE`. Valid values are `GZIP` and `NONE`.
*/
@JvmName("eswkmtluhjyuarsu")
public suspend fun compressionType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.compressionType = mapped
}
/**
* @param value Delimiter used to separate columns in the source files. Default is `,`.
*/
@JvmName("uwuhhoakxihauxck")
public suspend fun csvDelimiter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.csvDelimiter = mapped
}
/**
* @param value String to use for all columns not included in the supplemental log.
*/
@JvmName("mjncwhhpbgqgnrxf")
public suspend fun csvNoSupValue(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.csvNoSupValue = mapped
}
/**
* @param value String to as null when writing to the target.
*/
@JvmName("fdjiasqfgssawpbp")
public suspend fun csvNullValue(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.csvNullValue = mapped
}
/**
* @param value Delimiter used to separate rows in the source files. Default is `\n`.
*/
@JvmName("xiyrrifrgqnhbysi")
public suspend fun csvRowDelimiter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.csvRowDelimiter = mapped
}
/**
* @param value Output format for the files that AWS DMS uses to create S3 objects. Valid values are `csv` and `parquet`. Default is `csv`.
*/
@JvmName("twnwryyctgtkifeo")
public suspend fun dataFormat(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataFormat = mapped
}
/**
* @param value Size of one data page in bytes. Default is `1048576` (1 MiB).
*/
@JvmName("anrnnqklkjaigadt")
public suspend fun dataPageSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataPageSize = mapped
}
/**
* @param value Date separating delimiter to use during folder partitioning. Valid values are `SLASH`, `UNDERSCORE`, `DASH`, and `NONE`. Default is `SLASH`.
*/
@JvmName("hqhnfrlfsvvbmyjv")
public suspend fun datePartitionDelimiter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.datePartitionDelimiter = mapped
}
/**
* @param value Partition S3 bucket folders based on transaction commit dates. Default is `false`.
*/
@JvmName("wlvxgwgdodhffxjl")
public suspend fun datePartitionEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.datePartitionEnabled = mapped
}
/**
* @param value Date format to use during folder partitioning. Use this parameter when `date_partition_enabled` is set to true. Valid values are `YYYYMMDD`, `YYYYMMDDHH`, `YYYYMM`, `MMYYYYDD`, and `DDMMYYYY`. Default is `YYYYMMDD`.
*/
@JvmName("xsiredalmbgpjkos")
public suspend fun datePartitionSequence(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.datePartitionSequence = mapped
}
/**
* @param value Maximum size in bytes of an encoded dictionary page of a column. Default is `1048576` (1 MiB).
*/
@JvmName("bslxqforigohhedc")
public suspend fun dictPageSizeLimit(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dictPageSizeLimit = mapped
}
/**
* @param value Whether to enable statistics for Parquet pages and row groups. Default is `true`.
*/
@JvmName("unjwjhdasiqhrhso")
public suspend fun enableStatistics(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableStatistics = mapped
}
/**
* @param value Type of encoding to use. Value values are `rle_dictionary`, `plain`, and `plain_dictionary`. Default is `rle_dictionary`.
*/
@JvmName("pxbpxridryhipldi")
public suspend fun encodingType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encodingType = mapped
}
/**
* @param value Server-side encryption mode that you want to encrypt your .csv or .parquet object files copied to S3. Valid values are `SSE_S3` and `SSE_KMS`. Default is `SSE_S3`.
*/
@JvmName("dohcwmwutwogynkd")
public suspend fun encryptionMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryptionMode = mapped
}
/**
* @param value JSON document that describes how AWS DMS should interpret the data.
*/
@JvmName("xkylgkkhvsyuhpxc")
public suspend fun externalTableDefinition(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.externalTableDefinition = mapped
}
/**
* @param value Whether to integrate AWS Glue Data Catalog with an Amazon S3 target. See [Using AWS Glue Data Catalog with an Amazon S3 target for AWS DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.S3.html#CHAP_Target.S3.GlueCatalog) for more information. Default is `false`.
*/
@JvmName("aplhlfpheycwnmwx")
public suspend fun glueCatalogGeneration(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.glueCatalogGeneration = mapped
}
/**
* @param value When this value is set to `1`, DMS ignores the first row header in a .csv file. Default is `0`.
*/
@JvmName("iwpxobhqullcsuao")
public suspend fun ignoreHeaderRows(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ignoreHeaderRows = mapped
}
/**
* @param value Whether to enable a full load to write INSERT operations to the .csv output files only to indicate how the rows were added to the source database. Default is `false`.
*/
@JvmName("gdmofbawabirjmrp")
public suspend fun includeOpForFullLoad(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.includeOpForFullLoad = mapped
}
/**
* @param value Maximum size (in KB) of any .csv file to be created while migrating to an S3 target during full load. Valid values are from `1` to `1048576`. Default is `1048576` (1 GB).
*/
@JvmName("meqbnydafkrmdmmo")
public suspend fun maxFileSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxFileSize = mapped
}
/**
* @param value Specifies the precision of any TIMESTAMP column values written to an S3 object file in .parquet format. Default is `false`.
*/
@JvmName("giusjopvpwyxfaqv")
public suspend fun parquetTimestampInMillisecond(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.parquetTimestampInMillisecond = mapped
}
/**
* @param value Version of the .parquet file format. Default is `parquet-1-0`. Valid values are `parquet-1-0` and `parquet-2-0`.
*/
@JvmName("jlcrscnawlttuvnw")
public suspend fun parquetVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.parquetVersion = mapped
}
/**
* @param value Whether DMS saves the transaction order for a CDC load on the S3 target specified by `cdc_path`. Default is `false`.
*/
@JvmName("coipfwjxlxuvivxx")
public suspend fun preserveTransactions(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preserveTransactions = mapped
}
/**
* @param value For an S3 source, whether each leading double quotation mark has to be followed by an ending double quotation mark. Default is `true`.
*/
@JvmName("ewaookyvspujaktg")
public suspend fun rfc4180(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rfc4180 = mapped
}
/**
* @param value Number of rows in a row group. Default is `10000`.
*/
@JvmName("fgsdtpypcpigbikp")
public suspend fun rowGroupLength(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rowGroupLength = mapped
}
/**
* @param value ARN or Id of KMS Key to use when `encryption_mode` is `SSE_KMS`.
*/
@JvmName("otvvfrierccaanaa")
public suspend fun serverSideEncryptionKmsKeyId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serverSideEncryptionKmsKeyId = mapped
}
/**
* @param value ARN of the IAM Role with permissions to read from or write to the S3 Bucket.
*/
@JvmName("waqyakfcigqrfkkw")
public suspend fun serviceAccessRoleArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceAccessRoleArn = mapped
}
/**
* @param value Column to add with timestamp information to the endpoint data for an Amazon S3 target.
*/
@JvmName("qdexbvxcscltyigj")
public suspend fun timestampColumnName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timestampColumnName = mapped
}
/**
* @param value Whether to use `csv_no_sup_value` for columns not included in the supplemental log.
*/
@JvmName("aklkpxxdvsmlgqmq")
public suspend fun useCsvNoSupValue(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useCsvNoSupValue = mapped
}
/**
* @param value When set to true, uses the task start time as the timestamp column value instead of the time data is written to target. For full load, when set to true, each row of the timestamp column contains the task start time. For CDC loads, each row of the timestamp column contains the transaction commit time. When set to false, the full load timestamp in the timestamp column increments with the time data arrives at the target. Default is `false`.
*/
@JvmName("qrdtwfsqwuatnyil")
public suspend fun useTaskStartTimeForFullLoadTimestamp(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useTaskStartTimeForFullLoadTimestamp = mapped
}
internal fun build(): EndpointS3SettingsArgs = EndpointS3SettingsArgs(
addColumnName = addColumnName,
bucketFolder = bucketFolder,
bucketName = bucketName,
cannedAclForObjects = cannedAclForObjects,
cdcInsertsAndUpdates = cdcInsertsAndUpdates,
cdcInsertsOnly = cdcInsertsOnly,
cdcMaxBatchInterval = cdcMaxBatchInterval,
cdcMinFileSize = cdcMinFileSize,
cdcPath = cdcPath,
compressionType = compressionType,
csvDelimiter = csvDelimiter,
csvNoSupValue = csvNoSupValue,
csvNullValue = csvNullValue,
csvRowDelimiter = csvRowDelimiter,
dataFormat = dataFormat,
dataPageSize = dataPageSize,
datePartitionDelimiter = datePartitionDelimiter,
datePartitionEnabled = datePartitionEnabled,
datePartitionSequence = datePartitionSequence,
dictPageSizeLimit = dictPageSizeLimit,
enableStatistics = enableStatistics,
encodingType = encodingType,
encryptionMode = encryptionMode,
externalTableDefinition = externalTableDefinition,
glueCatalogGeneration = glueCatalogGeneration,
ignoreHeaderRows = ignoreHeaderRows,
includeOpForFullLoad = includeOpForFullLoad,
maxFileSize = maxFileSize,
parquetTimestampInMillisecond = parquetTimestampInMillisecond,
parquetVersion = parquetVersion,
preserveTransactions = preserveTransactions,
rfc4180 = rfc4180,
rowGroupLength = rowGroupLength,
serverSideEncryptionKmsKeyId = serverSideEncryptionKmsKeyId,
serviceAccessRoleArn = serviceAccessRoleArn,
timestampColumnName = timestampColumnName,
useCsvNoSupValue = useCsvNoSupValue,
useTaskStartTimeForFullLoadTimestamp = useTaskStartTimeForFullLoadTimestamp,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy