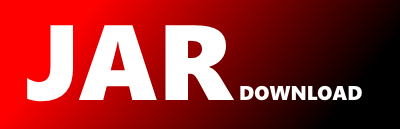
com.pulumi.aws.dms.kotlin.inputs.ReplicationConfigComputeConfigArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.dms.kotlin.inputs
import com.pulumi.aws.dms.inputs.ReplicationConfigComputeConfigArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property availabilityZone The Availability Zone where the DMS Serverless replication using this configuration will run. The default value is a random.
* @property dnsNameServers A list of custom DNS name servers supported for the DMS Serverless replication to access your source or target database.
* @property kmsKeyId An Key Management Service (KMS) key Amazon Resource Name (ARN) that is used to encrypt the data during DMS Serverless replication. If you don't specify a value for the KmsKeyId parameter, DMS uses your default encryption key.
* @property maxCapacityUnits Specifies the maximum value of the DMS capacity units (DCUs) for which a given DMS Serverless replication can be provisioned. A single DCU is 2GB of RAM, with 2 DCUs as the minimum value allowed. The list of valid DCU values includes 2, 4, 8, 16, 32, 64, 128, 192, 256, and 384.
* @property minCapacityUnits Specifies the minimum value of the DMS capacity units (DCUs) for which a given DMS Serverless replication can be provisioned. The list of valid DCU values includes 2, 4, 8, 16, 32, 64, 128, 192, 256, and 384. If this value isn't set DMS scans the current activity of available source tables to identify an optimum setting for this parameter.
* @property multiAz Specifies if the replication instance is a multi-az deployment. You cannot set the `availability_zone` parameter if the `multi_az` parameter is set to `true`.
* @property preferredMaintenanceWindow The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
* - Default: A 30-minute window selected at random from an 8-hour block of time per region, occurring on a random day of the week.
* - Format: `ddd:hh24:mi-ddd:hh24:mi`
* - Valid Days: `mon, tue, wed, thu, fri, sat, sun`
* - Constraints: Minimum 30-minute window.
* @property replicationSubnetGroupId Specifies a subnet group identifier to associate with the DMS Serverless replication.
* @property vpcSecurityGroupIds Specifies the virtual private cloud (VPC) security group to use with the DMS Serverless replication. The VPC security group must work with the VPC containing the replication.
*/
public data class ReplicationConfigComputeConfigArgs(
public val availabilityZone: Output? = null,
public val dnsNameServers: Output? = null,
public val kmsKeyId: Output? = null,
public val maxCapacityUnits: Output? = null,
public val minCapacityUnits: Output? = null,
public val multiAz: Output? = null,
public val preferredMaintenanceWindow: Output? = null,
public val replicationSubnetGroupId: Output,
public val vpcSecurityGroupIds: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.dms.inputs.ReplicationConfigComputeConfigArgs =
com.pulumi.aws.dms.inputs.ReplicationConfigComputeConfigArgs.builder()
.availabilityZone(availabilityZone?.applyValue({ args0 -> args0 }))
.dnsNameServers(dnsNameServers?.applyValue({ args0 -> args0 }))
.kmsKeyId(kmsKeyId?.applyValue({ args0 -> args0 }))
.maxCapacityUnits(maxCapacityUnits?.applyValue({ args0 -> args0 }))
.minCapacityUnits(minCapacityUnits?.applyValue({ args0 -> args0 }))
.multiAz(multiAz?.applyValue({ args0 -> args0 }))
.preferredMaintenanceWindow(preferredMaintenanceWindow?.applyValue({ args0 -> args0 }))
.replicationSubnetGroupId(replicationSubnetGroupId.applyValue({ args0 -> args0 }))
.vpcSecurityGroupIds(
vpcSecurityGroupIds?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
).build()
}
/**
* Builder for [ReplicationConfigComputeConfigArgs].
*/
@PulumiTagMarker
public class ReplicationConfigComputeConfigArgsBuilder internal constructor() {
private var availabilityZone: Output? = null
private var dnsNameServers: Output? = null
private var kmsKeyId: Output? = null
private var maxCapacityUnits: Output? = null
private var minCapacityUnits: Output? = null
private var multiAz: Output? = null
private var preferredMaintenanceWindow: Output? = null
private var replicationSubnetGroupId: Output? = null
private var vpcSecurityGroupIds: Output>? = null
/**
* @param value The Availability Zone where the DMS Serverless replication using this configuration will run. The default value is a random.
*/
@JvmName("tjwxbmyuqpvhliec")
public suspend fun availabilityZone(`value`: Output) {
this.availabilityZone = value
}
/**
* @param value A list of custom DNS name servers supported for the DMS Serverless replication to access your source or target database.
*/
@JvmName("wqyfxmfsayuxixsi")
public suspend fun dnsNameServers(`value`: Output) {
this.dnsNameServers = value
}
/**
* @param value An Key Management Service (KMS) key Amazon Resource Name (ARN) that is used to encrypt the data during DMS Serverless replication. If you don't specify a value for the KmsKeyId parameter, DMS uses your default encryption key.
*/
@JvmName("dehebtyeawxhmkii")
public suspend fun kmsKeyId(`value`: Output) {
this.kmsKeyId = value
}
/**
* @param value Specifies the maximum value of the DMS capacity units (DCUs) for which a given DMS Serverless replication can be provisioned. A single DCU is 2GB of RAM, with 2 DCUs as the minimum value allowed. The list of valid DCU values includes 2, 4, 8, 16, 32, 64, 128, 192, 256, and 384.
*/
@JvmName("curubffmexmhtppt")
public suspend fun maxCapacityUnits(`value`: Output) {
this.maxCapacityUnits = value
}
/**
* @param value Specifies the minimum value of the DMS capacity units (DCUs) for which a given DMS Serverless replication can be provisioned. The list of valid DCU values includes 2, 4, 8, 16, 32, 64, 128, 192, 256, and 384. If this value isn't set DMS scans the current activity of available source tables to identify an optimum setting for this parameter.
*/
@JvmName("bjfxngixmuyqiqrw")
public suspend fun minCapacityUnits(`value`: Output) {
this.minCapacityUnits = value
}
/**
* @param value Specifies if the replication instance is a multi-az deployment. You cannot set the `availability_zone` parameter if the `multi_az` parameter is set to `true`.
*/
@JvmName("pgcokusfohfqrtjp")
public suspend fun multiAz(`value`: Output) {
this.multiAz = value
}
/**
* @param value The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
* - Default: A 30-minute window selected at random from an 8-hour block of time per region, occurring on a random day of the week.
* - Format: `ddd:hh24:mi-ddd:hh24:mi`
* - Valid Days: `mon, tue, wed, thu, fri, sat, sun`
* - Constraints: Minimum 30-minute window.
*/
@JvmName("hokykexxeltcrodt")
public suspend fun preferredMaintenanceWindow(`value`: Output) {
this.preferredMaintenanceWindow = value
}
/**
* @param value Specifies a subnet group identifier to associate with the DMS Serverless replication.
*/
@JvmName("ffaduqpsatakrwfq")
public suspend fun replicationSubnetGroupId(`value`: Output) {
this.replicationSubnetGroupId = value
}
/**
* @param value Specifies the virtual private cloud (VPC) security group to use with the DMS Serverless replication. The VPC security group must work with the VPC containing the replication.
*/
@JvmName("ppjyebniirshguop")
public suspend fun vpcSecurityGroupIds(`value`: Output>) {
this.vpcSecurityGroupIds = value
}
@JvmName("ovfrxpaxoeivlxlw")
public suspend fun vpcSecurityGroupIds(vararg values: Output) {
this.vpcSecurityGroupIds = Output.all(values.asList())
}
/**
* @param values Specifies the virtual private cloud (VPC) security group to use with the DMS Serverless replication. The VPC security group must work with the VPC containing the replication.
*/
@JvmName("ldvtilgejcmwmggp")
public suspend fun vpcSecurityGroupIds(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy