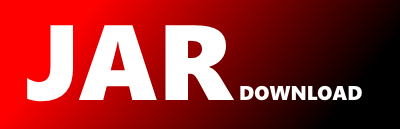
com.pulumi.aws.dms.kotlin.outputs.GetEndpointResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.dms.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getEndpoint.
* @property certificateArn
* @property databaseName
* @property elasticsearchSettings
* @property endpointArn
* @property endpointId
* @property endpointType
* @property engineName
* @property extraConnectionAttributes
* @property id The provider-assigned unique ID for this managed resource.
* @property kafkaSettings
* @property kinesisSettings
* @property kmsKeyArn
* @property mongodbSettings
* @property password
* @property port
* @property postgresSettings
* @property redisSettings
* @property redshiftSettings
* @property s3Settings
* @property secretsManagerAccessRoleArn
* @property secretsManagerArn
* @property serverName
* @property serviceAccessRole
* @property sslMode
* @property tags
* @property username
*/
public data class GetEndpointResult(
public val certificateArn: String,
public val databaseName: String,
public val elasticsearchSettings: List,
public val endpointArn: String,
public val endpointId: String,
public val endpointType: String,
public val engineName: String,
public val extraConnectionAttributes: String,
public val id: String,
public val kafkaSettings: List,
public val kinesisSettings: List,
public val kmsKeyArn: String,
public val mongodbSettings: List,
public val password: String,
public val port: Int,
public val postgresSettings: List,
public val redisSettings: List,
public val redshiftSettings: List,
public val s3Settings: List,
public val secretsManagerAccessRoleArn: String,
public val secretsManagerArn: String,
public val serverName: String,
public val serviceAccessRole: String,
public val sslMode: String,
public val tags: Map,
public val username: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.dms.outputs.GetEndpointResult): GetEndpointResult =
GetEndpointResult(
certificateArn = javaType.certificateArn(),
databaseName = javaType.databaseName(),
elasticsearchSettings = javaType.elasticsearchSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.dms.kotlin.outputs.GetEndpointElasticsearchSetting.Companion.toKotlin(args0)
})
}),
endpointArn = javaType.endpointArn(),
endpointId = javaType.endpointId(),
endpointType = javaType.endpointType(),
engineName = javaType.engineName(),
extraConnectionAttributes = javaType.extraConnectionAttributes(),
id = javaType.id(),
kafkaSettings = javaType.kafkaSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.dms.kotlin.outputs.GetEndpointKafkaSetting.Companion.toKotlin(args0)
})
}),
kinesisSettings = javaType.kinesisSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.dms.kotlin.outputs.GetEndpointKinesisSetting.Companion.toKotlin(args0)
})
}),
kmsKeyArn = javaType.kmsKeyArn(),
mongodbSettings = javaType.mongodbSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.dms.kotlin.outputs.GetEndpointMongodbSetting.Companion.toKotlin(args0)
})
}),
password = javaType.password(),
port = javaType.port(),
postgresSettings = javaType.postgresSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.dms.kotlin.outputs.GetEndpointPostgresSetting.Companion.toKotlin(args0)
})
}),
redisSettings = javaType.redisSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.dms.kotlin.outputs.GetEndpointRedisSetting.Companion.toKotlin(args0)
})
}),
redshiftSettings = javaType.redshiftSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.dms.kotlin.outputs.GetEndpointRedshiftSetting.Companion.toKotlin(args0)
})
}),
s3Settings = javaType.s3Settings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.dms.kotlin.outputs.GetEndpointS3Setting.Companion.toKotlin(args0)
})
}),
secretsManagerAccessRoleArn = javaType.secretsManagerAccessRoleArn(),
secretsManagerArn = javaType.secretsManagerArn(),
serverName = javaType.serverName(),
serviceAccessRole = javaType.serviceAccessRole(),
sslMode = javaType.sslMode(),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
username = javaType.username(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy