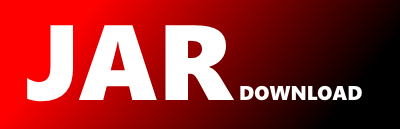
com.pulumi.aws.docdb.kotlin.Cluster.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.docdb.kotlin
import com.pulumi.aws.docdb.kotlin.outputs.ClusterRestoreToPointInTime
import com.pulumi.aws.docdb.kotlin.outputs.ClusterRestoreToPointInTime.Companion.toKotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
/**
* Builder for [Cluster].
*/
@PulumiTagMarker
public class ClusterResourceBuilder internal constructor() {
public var name: String? = null
public var args: ClusterArgs = ClusterArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ClusterArgsBuilder.() -> Unit) {
val builder = ClusterArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Cluster {
val builtJavaResource = com.pulumi.aws.docdb.Cluster(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Cluster(builtJavaResource)
}
}
/**
* Manages a DocumentDB Cluster.
* Changes to a DocumentDB Cluster can occur when you manually change a
* parameter, such as `port`, and are reflected in the next maintenance
* window. Because of this, this provider may report a difference in its planning
* phase because a modification has not yet taken place. You can use the
* `apply_immediately` flag to instruct the service to apply the change immediately
* (see documentation below).
* > **Note:** using `apply_immediately` can result in a brief downtime as the server reboots.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const docdb = new aws.docdb.Cluster("docdb", {
* clusterIdentifier: "my-docdb-cluster",
* engine: "docdb",
* masterUsername: "foo",
* masterPassword: "mustbeeightchars",
* backupRetentionPeriod: 5,
* preferredBackupWindow: "07:00-09:00",
* skipFinalSnapshot: true,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* docdb = aws.docdb.Cluster("docdb",
* cluster_identifier="my-docdb-cluster",
* engine="docdb",
* master_username="foo",
* master_password="mustbeeightchars",
* backup_retention_period=5,
* preferred_backup_window="07:00-09:00",
* skip_final_snapshot=True)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var docdb = new Aws.DocDB.Cluster("docdb", new()
* {
* ClusterIdentifier = "my-docdb-cluster",
* Engine = "docdb",
* MasterUsername = "foo",
* MasterPassword = "mustbeeightchars",
* BackupRetentionPeriod = 5,
* PreferredBackupWindow = "07:00-09:00",
* SkipFinalSnapshot = true,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/docdb"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := docdb.NewCluster(ctx, "docdb", &docdb.ClusterArgs{
* ClusterIdentifier: pulumi.String("my-docdb-cluster"),
* Engine: pulumi.String("docdb"),
* MasterUsername: pulumi.String("foo"),
* MasterPassword: pulumi.String("mustbeeightchars"),
* BackupRetentionPeriod: pulumi.Int(5),
* PreferredBackupWindow: pulumi.String("07:00-09:00"),
* SkipFinalSnapshot: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.docdb.Cluster;
* import com.pulumi.aws.docdb.ClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var docdb = new Cluster("docdb", ClusterArgs.builder()
* .clusterIdentifier("my-docdb-cluster")
* .engine("docdb")
* .masterUsername("foo")
* .masterPassword("mustbeeightchars")
* .backupRetentionPeriod(5)
* .preferredBackupWindow("07:00-09:00")
* .skipFinalSnapshot(true)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* docdb:
* type: aws:docdb:Cluster
* properties:
* clusterIdentifier: my-docdb-cluster
* engine: docdb
* masterUsername: foo
* masterPassword: mustbeeightchars
* backupRetentionPeriod: 5
* preferredBackupWindow: 07:00-09:00
* skipFinalSnapshot: true
* ```
*
* ## Import
* Using `pulumi import`, import DocumentDB Clusters using the `cluster_identifier`. For example:
* ```sh
* $ pulumi import aws:docdb/cluster:Cluster docdb_cluster docdb-prod-cluster
* ```
*/
public class Cluster internal constructor(
override val javaResource: com.pulumi.aws.docdb.Cluster,
) : KotlinCustomResource(javaResource, ClusterMapper) {
/**
* A value that indicates whether major version upgrades are allowed. Constraints: You must allow major version upgrades when specifying a value for the EngineVersion parameter that is a different major version than the DB cluster's current version.
*/
public val allowMajorVersionUpgrade: Output?
get() = javaResource.allowMajorVersionUpgrade().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies whether any cluster modifications
* are applied immediately, or during the next maintenance window. Default is
* `false`.
*/
public val applyImmediately: Output?
get() = javaResource.applyImmediately().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Amazon Resource Name (ARN) of cluster
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* A list of EC2 Availability Zones that
* instances in the DB cluster can be created in.
*/
public val availabilityZones: Output>
get() = javaResource.availabilityZones().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The days to retain backups for. Default `1`
*/
public val backupRetentionPeriod: Output?
get() = javaResource.backupRetentionPeriod().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The cluster identifier. If omitted, the provider will assign a random, unique identifier.
*/
public val clusterIdentifier: Output
get() = javaResource.clusterIdentifier().applyValue({ args0 -> args0 })
/**
* Creates a unique cluster identifier beginning with the specified prefix. Conflicts with `cluster_identifier`.
*/
public val clusterIdentifierPrefix: Output
get() = javaResource.clusterIdentifierPrefix().applyValue({ args0 -> args0 })
/**
* List of DocumentDB Instances that are a part of this cluster
*/
public val clusterMembers: Output>
get() = javaResource.clusterMembers().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* The DocumentDB Cluster Resource ID
*/
public val clusterResourceId: Output
get() = javaResource.clusterResourceId().applyValue({ args0 -> args0 })
/**
* A cluster parameter group to associate with the cluster.
*/
public val dbClusterParameterGroupName: Output
get() = javaResource.dbClusterParameterGroupName().applyValue({ args0 -> args0 })
/**
* A DB subnet group to associate with this DB instance.
*/
public val dbSubnetGroupName: Output
get() = javaResource.dbSubnetGroupName().applyValue({ args0 -> args0 })
/**
* A boolean value that indicates whether the DB cluster has deletion protection enabled. The database can't be deleted when deletion protection is enabled. Defaults to `false`.
*/
public val deletionProtection: Output?
get() = javaResource.deletionProtection().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* List of log types to export to cloudwatch. If omitted, no logs will be exported.
* The following log types are supported: `audit`, `profiler`.
*/
public val enabledCloudwatchLogsExports: Output>?
get() = javaResource.enabledCloudwatchLogsExports().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The DNS address of the DocumentDB instance
*/
public val endpoint: Output
get() = javaResource.endpoint().applyValue({ args0 -> args0 })
/**
* The name of the database engine to be used for this DB cluster. Defaults to `docdb`. Valid values: `docdb`.
*/
public val engine: Output?
get() = javaResource.engine().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The database engine version. Updating this argument results in an outage.
*/
public val engineVersion: Output
get() = javaResource.engineVersion().applyValue({ args0 -> args0 })
/**
* The name of your final DB snapshot
* when this DB cluster is deleted. If omitted, no final snapshot will be
* made.
*/
public val finalSnapshotIdentifier: Output?
get() = javaResource.finalSnapshotIdentifier().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The global cluster identifier specified on `aws.docdb.GlobalCluster`.
*/
public val globalClusterIdentifier: Output?
get() = javaResource.globalClusterIdentifier().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The Route53 Hosted Zone ID of the endpoint
*/
public val hostedZoneId: Output
get() = javaResource.hostedZoneId().applyValue({ args0 -> args0 })
/**
* The ARN for the KMS encryption key. When specifying `kms_key_id`, `storage_encrypted` needs to be set to true.
*/
public val kmsKeyId: Output
get() = javaResource.kmsKeyId().applyValue({ args0 -> args0 })
/**
* Password for the master DB user. Note that this may
* show up in logs, and it will be stored in the state file. Please refer to the DocumentDB Naming Constraints.
*/
public val masterPassword: Output?
get() = javaResource.masterPassword().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Username for the master DB user.
*/
public val masterUsername: Output
get() = javaResource.masterUsername().applyValue({ args0 -> args0 })
/**
* The port on which the DB accepts connections
*/
public val port: Output?
get() = javaResource.port().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The daily time range during which automated backups are created if automated backups are enabled using the BackupRetentionPeriod parameter.Time in UTC
* Default: A 30-minute window selected at random from an 8-hour block of time per regionE.g., 04:00-09:00
*/
public val preferredBackupWindow: Output
get() = javaResource.preferredBackupWindow().applyValue({ args0 -> args0 })
/**
* The weekly time range during which system maintenance can occur, in (UTC) e.g., wed:04:00-wed:04:30
*/
public val preferredMaintenanceWindow: Output
get() = javaResource.preferredMaintenanceWindow().applyValue({ args0 -> args0 })
/**
* A read-only endpoint for the DocumentDB cluster, automatically load-balanced across replicas
*/
public val readerEndpoint: Output
get() = javaResource.readerEndpoint().applyValue({ args0 -> args0 })
/**
* A configuration block for restoring a DB instance to an arbitrary point in time. Requires the `identifier` argument to be set with the name of the new DB instance to be created. See Restore To Point In Time below for details.
*/
public val restoreToPointInTime: Output?
get() = javaResource.restoreToPointInTime().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> toKotlin(args0) })
}).orElse(null)
})
/**
* Determines whether a final DB snapshot is created before the DB cluster is deleted. If true is specified, no DB snapshot is created. If false is specified, a DB snapshot is created before the DB cluster is deleted, using the value from `final_snapshot_identifier`. Default is `false`.
*/
public val skipFinalSnapshot: Output?
get() = javaResource.skipFinalSnapshot().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies whether or not to create this cluster from a snapshot. You can use either the name or ARN when specifying a DB cluster snapshot, or the ARN when specifying a DB snapshot. Automated snapshots **should not** be used for this attribute, unless from a different cluster. Automated snapshots are deleted as part of cluster destruction when the resource is replaced.
*/
public val snapshotIdentifier: Output?
get() = javaResource.snapshotIdentifier().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies whether the DB cluster is encrypted. The default is `false`.
*/
public val storageEncrypted: Output?
get() = javaResource.storageEncrypted().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The storage type to associate with the DB cluster. Valid values: `standard`, `iopt1`.
*/
public val storageType: Output?
get() = javaResource.storageType().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A map of tags to assign to the DB cluster. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy