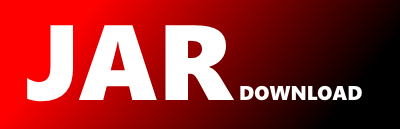
com.pulumi.aws.dynamodb.kotlin.outputs.GetTableResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.dynamodb.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getTable.
* @property arn
* @property attributes
* @property billingMode
* @property deletionProtectionEnabled
* @property globalSecondaryIndexes
* @property hashKey
* @property id The provider-assigned unique ID for this managed resource.
* @property localSecondaryIndexes
* @property name
* @property pointInTimeRecovery
* @property rangeKey
* @property readCapacity
* @property replicas
* @property serverSideEncryption
* @property streamArn
* @property streamEnabled
* @property streamLabel
* @property streamViewType
* @property tableClass
* @property tags
* @property ttl
* @property writeCapacity
*/
public data class GetTableResult(
public val arn: String,
public val attributes: List,
public val billingMode: String,
public val deletionProtectionEnabled: Boolean,
public val globalSecondaryIndexes: List,
public val hashKey: String,
public val id: String,
public val localSecondaryIndexes: List,
public val name: String,
public val pointInTimeRecovery: GetTablePointInTimeRecovery,
public val rangeKey: String,
public val readCapacity: Int,
public val replicas: List,
public val serverSideEncryption: GetTableServerSideEncryption,
public val streamArn: String,
public val streamEnabled: Boolean,
public val streamLabel: String,
public val streamViewType: String,
public val tableClass: String,
public val tags: Map,
public val ttl: GetTableTtl,
public val writeCapacity: Int,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.dynamodb.outputs.GetTableResult): GetTableResult =
GetTableResult(
arn = javaType.arn(),
attributes = javaType.attributes().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.dynamodb.kotlin.outputs.GetTableAttribute.Companion.toKotlin(args0)
})
}),
billingMode = javaType.billingMode(),
deletionProtectionEnabled = javaType.deletionProtectionEnabled(),
globalSecondaryIndexes = javaType.globalSecondaryIndexes().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.dynamodb.kotlin.outputs.GetTableGlobalSecondaryIndex.Companion.toKotlin(args0)
})
}),
hashKey = javaType.hashKey(),
id = javaType.id(),
localSecondaryIndexes = javaType.localSecondaryIndexes().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.dynamodb.kotlin.outputs.GetTableLocalSecondaryIndex.Companion.toKotlin(args0)
})
}),
name = javaType.name(),
pointInTimeRecovery = javaType.pointInTimeRecovery().let({ args0 ->
com.pulumi.aws.dynamodb.kotlin.outputs.GetTablePointInTimeRecovery.Companion.toKotlin(args0)
}),
rangeKey = javaType.rangeKey(),
readCapacity = javaType.readCapacity(),
replicas = javaType.replicas().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.dynamodb.kotlin.outputs.GetTableReplica.Companion.toKotlin(args0)
})
}),
serverSideEncryption = javaType.serverSideEncryption().let({ args0 ->
com.pulumi.aws.dynamodb.kotlin.outputs.GetTableServerSideEncryption.Companion.toKotlin(args0)
}),
streamArn = javaType.streamArn(),
streamEnabled = javaType.streamEnabled(),
streamLabel = javaType.streamLabel(),
streamViewType = javaType.streamViewType(),
tableClass = javaType.tableClass(),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
ttl = javaType.ttl().let({ args0 ->
com.pulumi.aws.dynamodb.kotlin.outputs.GetTableTtl.Companion.toKotlin(args0)
}),
writeCapacity = javaType.writeCapacity(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy