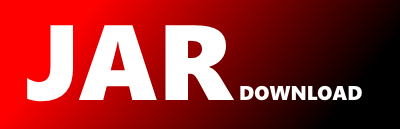
com.pulumi.aws.ec2.kotlin.FlowLogArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ec2.kotlin
import com.pulumi.aws.ec2.FlowLogArgs.builder
import com.pulumi.aws.ec2.kotlin.inputs.FlowLogDestinationOptionsArgs
import com.pulumi.aws.ec2.kotlin.inputs.FlowLogDestinationOptionsArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Deprecated
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Provides a VPC/Subnet/ENI/Transit Gateway/Transit Gateway Attachment Flow Log to capture IP traffic for a specific network
* interface, subnet, or VPC. Logs are sent to a CloudWatch Log Group, a S3 Bucket, or Amazon Kinesis Data Firehose
* ## Example Usage
* ### CloudWatch Logging
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const exampleLogGroup = new aws.cloudwatch.LogGroup("example", {name: "example"});
* const assumeRole = aws.iam.getPolicyDocument({
* statements: [{
* effect: "Allow",
* principals: [{
* type: "Service",
* identifiers: ["vpc-flow-logs.amazonaws.com"],
* }],
* actions: ["sts:AssumeRole"],
* }],
* });
* const exampleRole = new aws.iam.Role("example", {
* name: "example",
* assumeRolePolicy: assumeRole.then(assumeRole => assumeRole.json),
* });
* const exampleFlowLog = new aws.ec2.FlowLog("example", {
* iamRoleArn: exampleRole.arn,
* logDestination: exampleLogGroup.arn,
* trafficType: "ALL",
* vpcId: exampleAwsVpc.id,
* });
* const example = aws.iam.getPolicyDocument({
* statements: [{
* effect: "Allow",
* actions: [
* "logs:CreateLogGroup",
* "logs:CreateLogStream",
* "logs:PutLogEvents",
* "logs:DescribeLogGroups",
* "logs:DescribeLogStreams",
* ],
* resources: ["*"],
* }],
* });
* const exampleRolePolicy = new aws.iam.RolePolicy("example", {
* name: "example",
* role: exampleRole.id,
* policy: example.then(example => example.json),
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example_log_group = aws.cloudwatch.LogGroup("example", name="example")
* assume_role = aws.iam.get_policy_document(statements=[{
* "effect": "Allow",
* "principals": [{
* "type": "Service",
* "identifiers": ["vpc-flow-logs.amazonaws.com"],
* }],
* "actions": ["sts:AssumeRole"],
* }])
* example_role = aws.iam.Role("example",
* name="example",
* assume_role_policy=assume_role.json)
* example_flow_log = aws.ec2.FlowLog("example",
* iam_role_arn=example_role.arn,
* log_destination=example_log_group.arn,
* traffic_type="ALL",
* vpc_id=example_aws_vpc["id"])
* example = aws.iam.get_policy_document(statements=[{
* "effect": "Allow",
* "actions": [
* "logs:CreateLogGroup",
* "logs:CreateLogStream",
* "logs:PutLogEvents",
* "logs:DescribeLogGroups",
* "logs:DescribeLogStreams",
* ],
* "resources": ["*"],
* }])
* example_role_policy = aws.iam.RolePolicy("example",
* name="example",
* role=example_role.id,
* policy=example.json)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var exampleLogGroup = new Aws.CloudWatch.LogGroup("example", new()
* {
* Name = "example",
* });
* var assumeRole = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Effect = "Allow",
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "Service",
* Identifiers = new[]
* {
* "vpc-flow-logs.amazonaws.com",
* },
* },
* },
* Actions = new[]
* {
* "sts:AssumeRole",
* },
* },
* },
* });
* var exampleRole = new Aws.Iam.Role("example", new()
* {
* Name = "example",
* AssumeRolePolicy = assumeRole.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* var exampleFlowLog = new Aws.Ec2.FlowLog("example", new()
* {
* IamRoleArn = exampleRole.Arn,
* LogDestination = exampleLogGroup.Arn,
* TrafficType = "ALL",
* VpcId = exampleAwsVpc.Id,
* });
* var example = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Effect = "Allow",
* Actions = new[]
* {
* "logs:CreateLogGroup",
* "logs:CreateLogStream",
* "logs:PutLogEvents",
* "logs:DescribeLogGroups",
* "logs:DescribeLogStreams",
* },
* Resources = new[]
* {
* "*",
* },
* },
* },
* });
* var exampleRolePolicy = new Aws.Iam.RolePolicy("example", new()
* {
* Name = "example",
* Role = exampleRole.Id,
* Policy = example.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/cloudwatch"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ec2"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* exampleLogGroup, err := cloudwatch.NewLogGroup(ctx, "example", &cloudwatch.LogGroupArgs{
* Name: pulumi.String("example"),
* })
* if err != nil {
* return err
* }
* assumeRole, err := iam.GetPolicyDocument(ctx, &iam.GetPolicyDocumentArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Effect: pulumi.StringRef("Allow"),
* Principals: []iam.GetPolicyDocumentStatementPrincipal{
* {
* Type: "Service",
* Identifiers: []string{
* "vpc-flow-logs.amazonaws.com",
* },
* },
* },
* Actions: []string{
* "sts:AssumeRole",
* },
* },
* },
* }, nil)
* if err != nil {
* return err
* }
* exampleRole, err := iam.NewRole(ctx, "example", &iam.RoleArgs{
* Name: pulumi.String("example"),
* AssumeRolePolicy: pulumi.String(assumeRole.Json),
* })
* if err != nil {
* return err
* }
* _, err = ec2.NewFlowLog(ctx, "example", &ec2.FlowLogArgs{
* IamRoleArn: exampleRole.Arn,
* LogDestination: exampleLogGroup.Arn,
* TrafficType: pulumi.String("ALL"),
* VpcId: pulumi.Any(exampleAwsVpc.Id),
* })
* if err != nil {
* return err
* }
* example, err := iam.GetPolicyDocument(ctx, &iam.GetPolicyDocumentArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Effect: pulumi.StringRef("Allow"),
* Actions: []string{
* "logs:CreateLogGroup",
* "logs:CreateLogStream",
* "logs:PutLogEvents",
* "logs:DescribeLogGroups",
* "logs:DescribeLogStreams",
* },
* Resources: []string{
* "*",
* },
* },
* },
* }, nil)
* if err != nil {
* return err
* }
* _, err = iam.NewRolePolicy(ctx, "example", &iam.RolePolicyArgs{
* Name: pulumi.String("example"),
* Role: exampleRole.ID(),
* Policy: pulumi.String(example.Json),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.cloudwatch.LogGroup;
* import com.pulumi.aws.cloudwatch.LogGroupArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.ec2.FlowLog;
* import com.pulumi.aws.ec2.FlowLogArgs;
* import com.pulumi.aws.iam.RolePolicy;
* import com.pulumi.aws.iam.RolePolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var exampleLogGroup = new LogGroup("exampleLogGroup", LogGroupArgs.builder()
* .name("example")
* .build());
* final var assumeRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("vpc-flow-logs.amazonaws.com")
* .build())
* .actions("sts:AssumeRole")
* .build())
* .build());
* var exampleRole = new Role("exampleRole", RoleArgs.builder()
* .name("example")
* .assumeRolePolicy(assumeRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* var exampleFlowLog = new FlowLog("exampleFlowLog", FlowLogArgs.builder()
* .iamRoleArn(exampleRole.arn())
* .logDestination(exampleLogGroup.arn())
* .trafficType("ALL")
* .vpcId(exampleAwsVpc.id())
* .build());
* final var example = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions(
* "logs:CreateLogGroup",
* "logs:CreateLogStream",
* "logs:PutLogEvents",
* "logs:DescribeLogGroups",
* "logs:DescribeLogStreams")
* .resources("*")
* .build())
* .build());
* var exampleRolePolicy = new RolePolicy("exampleRolePolicy", RolePolicyArgs.builder()
* .name("example")
* .role(exampleRole.id())
* .policy(example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleFlowLog:
* type: aws:ec2:FlowLog
* name: example
* properties:
* iamRoleArn: ${exampleRole.arn}
* logDestination: ${exampleLogGroup.arn}
* trafficType: ALL
* vpcId: ${exampleAwsVpc.id}
* exampleLogGroup:
* type: aws:cloudwatch:LogGroup
* name: example
* properties:
* name: example
* exampleRole:
* type: aws:iam:Role
* name: example
* properties:
* name: example
* assumeRolePolicy: ${assumeRole.json}
* exampleRolePolicy:
* type: aws:iam:RolePolicy
* name: example
* properties:
* name: example
* role: ${exampleRole.id}
* policy: ${example.json}
* variables:
* assumeRole:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - effect: Allow
* principals:
* - type: Service
* identifiers:
* - vpc-flow-logs.amazonaws.com
* actions:
* - sts:AssumeRole
* example:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - effect: Allow
* actions:
* - logs:CreateLogGroup
* - logs:CreateLogStream
* - logs:PutLogEvents
* - logs:DescribeLogGroups
* - logs:DescribeLogStreams
* resources:
* - '*'
* ```
*
* ### Amazon Kinesis Data Firehose logging
*
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.kinesis.FirehoseDeliveryStream;
* import com.pulumi.aws.kinesis.FirehoseDeliveryStreamArgs;
* import com.pulumi.aws.kinesis.inputs.FirehoseDeliveryStreamExtendedS3ConfigurationArgs;
* import com.pulumi.aws.ec2.FlowLog;
* import com.pulumi.aws.ec2.FlowLogArgs;
* import com.pulumi.aws.s3.BucketAclV2;
* import com.pulumi.aws.s3.BucketAclV2Args;
* import com.pulumi.aws.iam.RolePolicy;
* import com.pulumi.aws.iam.RolePolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var exampleBucketV2 = new BucketV2("exampleBucketV2", BucketV2Args.builder()
* .bucket("example")
* .build());
* final var assumeRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("firehose.amazonaws.com")
* .build())
* .actions("sts:AssumeRole")
* .build())
* .build());
* var exampleRole = new Role("exampleRole", RoleArgs.builder()
* .name("firehose_test_role")
* .assumeRolePolicy(assumeRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* var exampleFirehoseDeliveryStream = new FirehoseDeliveryStream("exampleFirehoseDeliveryStream", FirehoseDeliveryStreamArgs.builder()
* .name("kinesis_firehose_test")
* .destination("extended_s3")
* .extendedS3Configuration(FirehoseDeliveryStreamExtendedS3ConfigurationArgs.builder()
* .roleArn(exampleRole.arn())
* .bucketArn(exampleBucketV2.arn())
* .build())
* .tags(Map.of("LogDeliveryEnabled", "true"))
* .build());
* var exampleFlowLog = new FlowLog("exampleFlowLog", FlowLogArgs.builder()
* .logDestination(exampleFirehoseDeliveryStream.arn())
* .logDestinationType("kinesis-data-firehose")
* .trafficType("ALL")
* .vpcId(exampleAwsVpc.id())
* .build());
* var exampleBucketAclV2 = new BucketAclV2("exampleBucketAclV2", BucketAclV2Args.builder()
* .bucket(exampleBucketV2.id())
* .acl("private")
* .build());
* final var example = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .effect("Allow")
* .actions(
* "logs:CreateLogDelivery",
* "logs:DeleteLogDelivery",
* "logs:ListLogDeliveries",
* "logs:GetLogDelivery",
* "firehose:TagDeliveryStream")
* .resources("*")
* .build());
* var exampleRolePolicy = new RolePolicy("exampleRolePolicy", RolePolicyArgs.builder()
* .name("test")
* .role(exampleRole.id())
* .policy(example.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* exampleFlowLog:
* type: aws:ec2:FlowLog
* name: example
* properties:
* logDestination: ${exampleFirehoseDeliveryStream.arn}
* logDestinationType: kinesis-data-firehose
* trafficType: ALL
* vpcId: ${exampleAwsVpc.id}
* exampleFirehoseDeliveryStream:
* type: aws:kinesis:FirehoseDeliveryStream
* name: example
* properties:
* name: kinesis_firehose_test
* destination: extended_s3
* extendedS3Configuration:
* roleArn: ${exampleRole.arn}
* bucketArn: ${exampleBucketV2.arn}
* tags:
* LogDeliveryEnabled: 'true'
* exampleBucketV2:
* type: aws:s3:BucketV2
* name: example
* properties:
* bucket: example
* exampleBucketAclV2:
* type: aws:s3:BucketAclV2
* name: example
* properties:
* bucket: ${exampleBucketV2.id}
* acl: private
* exampleRole:
* type: aws:iam:Role
* name: example
* properties:
* name: firehose_test_role
* assumeRolePolicy: ${assumeRole.json}
* exampleRolePolicy:
* type: aws:iam:RolePolicy
* name: example
* properties:
* name: test
* role: ${exampleRole.id}
* policy: ${example.json}
* variables:
* assumeRole:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - effect: Allow
* principals:
* - type: Service
* identifiers:
* - firehose.amazonaws.com
* actions:
* - sts:AssumeRole
* example:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* effect: Allow
* actions:
* - logs:CreateLogDelivery
* - logs:DeleteLogDelivery
* - logs:ListLogDeliveries
* - logs:GetLogDelivery
* - firehose:TagDeliveryStream
* resources:
* - '*'
* ```
*
* ### S3 Logging
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const exampleBucketV2 = new aws.s3.BucketV2("example", {bucket: "example"});
* const example = new aws.ec2.FlowLog("example", {
* logDestination: exampleBucketV2.arn,
* logDestinationType: "s3",
* trafficType: "ALL",
* vpcId: exampleAwsVpc.id,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example_bucket_v2 = aws.s3.BucketV2("example", bucket="example")
* example = aws.ec2.FlowLog("example",
* log_destination=example_bucket_v2.arn,
* log_destination_type="s3",
* traffic_type="ALL",
* vpc_id=example_aws_vpc["id"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var exampleBucketV2 = new Aws.S3.BucketV2("example", new()
* {
* Bucket = "example",
* });
* var example = new Aws.Ec2.FlowLog("example", new()
* {
* LogDestination = exampleBucketV2.Arn,
* LogDestinationType = "s3",
* TrafficType = "ALL",
* VpcId = exampleAwsVpc.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ec2"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* exampleBucketV2, err := s3.NewBucketV2(ctx, "example", &s3.BucketV2Args{
* Bucket: pulumi.String("example"),
* })
* if err != nil {
* return err
* }
* _, err = ec2.NewFlowLog(ctx, "example", &ec2.FlowLogArgs{
* LogDestination: exampleBucketV2.Arn,
* LogDestinationType: pulumi.String("s3"),
* TrafficType: pulumi.String("ALL"),
* VpcId: pulumi.Any(exampleAwsVpc.Id),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.ec2.FlowLog;
* import com.pulumi.aws.ec2.FlowLogArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var exampleBucketV2 = new BucketV2("exampleBucketV2", BucketV2Args.builder()
* .bucket("example")
* .build());
* var example = new FlowLog("example", FlowLogArgs.builder()
* .logDestination(exampleBucketV2.arn())
* .logDestinationType("s3")
* .trafficType("ALL")
* .vpcId(exampleAwsVpc.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:ec2:FlowLog
* properties:
* logDestination: ${exampleBucketV2.arn}
* logDestinationType: s3
* trafficType: ALL
* vpcId: ${exampleAwsVpc.id}
* exampleBucketV2:
* type: aws:s3:BucketV2
* name: example
* properties:
* bucket: example
* ```
*
* ### S3 Logging in Apache Parquet format with per-hour partitions
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const exampleBucketV2 = new aws.s3.BucketV2("example", {bucket: "example"});
* const example = new aws.ec2.FlowLog("example", {
* logDestination: exampleBucketV2.arn,
* logDestinationType: "s3",
* trafficType: "ALL",
* vpcId: exampleAwsVpc.id,
* destinationOptions: {
* fileFormat: "parquet",
* perHourPartition: true,
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example_bucket_v2 = aws.s3.BucketV2("example", bucket="example")
* example = aws.ec2.FlowLog("example",
* log_destination=example_bucket_v2.arn,
* log_destination_type="s3",
* traffic_type="ALL",
* vpc_id=example_aws_vpc["id"],
* destination_options={
* "file_format": "parquet",
* "per_hour_partition": True,
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var exampleBucketV2 = new Aws.S3.BucketV2("example", new()
* {
* Bucket = "example",
* });
* var example = new Aws.Ec2.FlowLog("example", new()
* {
* LogDestination = exampleBucketV2.Arn,
* LogDestinationType = "s3",
* TrafficType = "ALL",
* VpcId = exampleAwsVpc.Id,
* DestinationOptions = new Aws.Ec2.Inputs.FlowLogDestinationOptionsArgs
* {
* FileFormat = "parquet",
* PerHourPartition = true,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ec2"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/s3"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* exampleBucketV2, err := s3.NewBucketV2(ctx, "example", &s3.BucketV2Args{
* Bucket: pulumi.String("example"),
* })
* if err != nil {
* return err
* }
* _, err = ec2.NewFlowLog(ctx, "example", &ec2.FlowLogArgs{
* LogDestination: exampleBucketV2.Arn,
* LogDestinationType: pulumi.String("s3"),
* TrafficType: pulumi.String("ALL"),
* VpcId: pulumi.Any(exampleAwsVpc.Id),
* DestinationOptions: &ec2.FlowLogDestinationOptionsArgs{
* FileFormat: pulumi.String("parquet"),
* PerHourPartition: pulumi.Bool(true),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.ec2.FlowLog;
* import com.pulumi.aws.ec2.FlowLogArgs;
* import com.pulumi.aws.ec2.inputs.FlowLogDestinationOptionsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var exampleBucketV2 = new BucketV2("exampleBucketV2", BucketV2Args.builder()
* .bucket("example")
* .build());
* var example = new FlowLog("example", FlowLogArgs.builder()
* .logDestination(exampleBucketV2.arn())
* .logDestinationType("s3")
* .trafficType("ALL")
* .vpcId(exampleAwsVpc.id())
* .destinationOptions(FlowLogDestinationOptionsArgs.builder()
* .fileFormat("parquet")
* .perHourPartition(true)
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:ec2:FlowLog
* properties:
* logDestination: ${exampleBucketV2.arn}
* logDestinationType: s3
* trafficType: ALL
* vpcId: ${exampleAwsVpc.id}
* destinationOptions:
* fileFormat: parquet
* perHourPartition: true
* exampleBucketV2:
* type: aws:s3:BucketV2
* name: example
* properties:
* bucket: example
* ```
*
* ## Import
* Using `pulumi import`, import Flow Logs using the `id`. For example:
* ```sh
* $ pulumi import aws:ec2/flowLog:FlowLog test_flow_log fl-1a2b3c4d
* ```
* @property deliverCrossAccountRole ARN of the IAM role that allows Amazon EC2 to publish flow logs across accounts.
* @property destinationOptions Describes the destination options for a flow log. More details below.
* @property eniId Elastic Network Interface ID to attach to
* @property iamRoleArn The ARN for the IAM role that's used to post flow logs to a CloudWatch Logs log group
* @property logDestination The ARN of the logging destination. Either `log_destination` or `log_group_name` must be set.
* @property logDestinationType The type of the logging destination. Valid values: `cloud-watch-logs`, `s3`, `kinesis-data-firehose`. Default: `cloud-watch-logs`.
* @property logFormat The fields to include in the flow log record. Accepted format example: `"$${interface-id} $${srcaddr} $${dstaddr} $${srcport} $${dstport}"`.
* @property logGroupName **Deprecated:** Use `log_destination` instead. The name of the CloudWatch log group. Either `log_group_name` or `log_destination` must be set.
* @property maxAggregationInterval The maximum interval of time
* during which a flow of packets is captured and aggregated into a flow
* log record. Valid Values: `60` seconds (1 minute) or `600` seconds (10
* minutes). Default: `600`. When `transit_gateway_id` or `transit_gateway_attachment_id` is specified, `max_aggregation_interval` *must* be 60 seconds (1 minute).
* @property subnetId Subnet ID to attach to
* @property tags Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
* @property trafficType The type of traffic to capture. Valid values: `ACCEPT`,`REJECT`, `ALL`.
* @property transitGatewayAttachmentId Transit Gateway Attachment ID to attach to
* @property transitGatewayId Transit Gateway ID to attach to
* @property vpcId VPC ID to attach to
*/
public data class FlowLogArgs(
public val deliverCrossAccountRole: Output? = null,
public val destinationOptions: Output? = null,
public val eniId: Output? = null,
public val iamRoleArn: Output? = null,
public val logDestination: Output? = null,
public val logDestinationType: Output? = null,
public val logFormat: Output? = null,
@Deprecated(
message = """
use 'log_destination' argument instead
""",
)
public val logGroupName: Output? = null,
public val maxAggregationInterval: Output? = null,
public val subnetId: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy