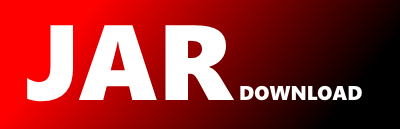
com.pulumi.aws.ec2.kotlin.LaunchConfiguration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.ec2.kotlin
import com.pulumi.aws.ec2.kotlin.outputs.LaunchConfigurationEbsBlockDevice
import com.pulumi.aws.ec2.kotlin.outputs.LaunchConfigurationEphemeralBlockDevice
import com.pulumi.aws.ec2.kotlin.outputs.LaunchConfigurationMetadataOptions
import com.pulumi.aws.ec2.kotlin.outputs.LaunchConfigurationRootBlockDevice
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.aws.ec2.kotlin.outputs.LaunchConfigurationEbsBlockDevice.Companion.toKotlin as launchConfigurationEbsBlockDeviceToKotlin
import com.pulumi.aws.ec2.kotlin.outputs.LaunchConfigurationEphemeralBlockDevice.Companion.toKotlin as launchConfigurationEphemeralBlockDeviceToKotlin
import com.pulumi.aws.ec2.kotlin.outputs.LaunchConfigurationMetadataOptions.Companion.toKotlin as launchConfigurationMetadataOptionsToKotlin
import com.pulumi.aws.ec2.kotlin.outputs.LaunchConfigurationRootBlockDevice.Companion.toKotlin as launchConfigurationRootBlockDeviceToKotlin
/**
* Builder for [LaunchConfiguration].
*/
@PulumiTagMarker
public class LaunchConfigurationResourceBuilder internal constructor() {
public var name: String? = null
public var args: LaunchConfigurationArgs = LaunchConfigurationArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend LaunchConfigurationArgsBuilder.() -> Unit) {
val builder = LaunchConfigurationArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): LaunchConfiguration {
val builtJavaResource = com.pulumi.aws.ec2.LaunchConfiguration(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return LaunchConfiguration(builtJavaResource)
}
}
/**
* Provides a resource to create a new launch configuration, used for autoscaling groups.
* !> **WARNING:** The use of launch configurations is discouraged in favor of launch templates. Read more in the [AWS EC2 Documentation](https://docs.aws.amazon.com/autoscaling/ec2/userguide/launch-configurations.html).
* > **Note** When using `aws.ec2.LaunchConfiguration` with `aws.autoscaling.Group`, it is recommended to use the `name_prefix` (Optional) instead of the `name` (Optional) attribute.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const ubuntu = aws.ec2.getAmi({
* mostRecent: true,
* filters: [
* {
* name: "name",
* values: ["ubuntu/images/hvm-ssd/ubuntu-trusty-14.04-amd64-server-*"],
* },
* {
* name: "virtualization-type",
* values: ["hvm"],
* },
* ],
* owners: ["099720109477"],
* });
* const asConf = new aws.ec2.LaunchConfiguration("as_conf", {
* name: "web_config",
* imageId: ubuntu.then(ubuntu => ubuntu.id),
* instanceType: "t2.micro",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* ubuntu = aws.ec2.get_ami(most_recent=True,
* filters=[
* {
* "name": "name",
* "values": ["ubuntu/images/hvm-ssd/ubuntu-trusty-14.04-amd64-server-*"],
* },
* {
* "name": "virtualization-type",
* "values": ["hvm"],
* },
* ],
* owners=["099720109477"])
* as_conf = aws.ec2.LaunchConfiguration("as_conf",
* name="web_config",
* image_id=ubuntu.id,
* instance_type="t2.micro")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var ubuntu = Aws.Ec2.GetAmi.Invoke(new()
* {
* MostRecent = true,
* Filters = new[]
* {
* new Aws.Ec2.Inputs.GetAmiFilterInputArgs
* {
* Name = "name",
* Values = new[]
* {
* "ubuntu/images/hvm-ssd/ubuntu-trusty-14.04-amd64-server-*",
* },
* },
* new Aws.Ec2.Inputs.GetAmiFilterInputArgs
* {
* Name = "virtualization-type",
* Values = new[]
* {
* "hvm",
* },
* },
* },
* Owners = new[]
* {
* "099720109477",
* },
* });
* var asConf = new Aws.Ec2.LaunchConfiguration("as_conf", new()
* {
* Name = "web_config",
* ImageId = ubuntu.Apply(getAmiResult => getAmiResult.Id),
* InstanceType = "t2.micro",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ec2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* ubuntu, err := ec2.LookupAmi(ctx, &ec2.LookupAmiArgs{
* MostRecent: pulumi.BoolRef(true),
* Filters: []ec2.GetAmiFilter{
* {
* Name: "name",
* Values: []string{
* "ubuntu/images/hvm-ssd/ubuntu-trusty-14.04-amd64-server-*",
* },
* },
* {
* Name: "virtualization-type",
* Values: []string{
* "hvm",
* },
* },
* },
* Owners: []string{
* "099720109477",
* },
* }, nil)
* if err != nil {
* return err
* }
* _, err = ec2.NewLaunchConfiguration(ctx, "as_conf", &ec2.LaunchConfigurationArgs{
* Name: pulumi.String("web_config"),
* ImageId: pulumi.String(ubuntu.Id),
* InstanceType: pulumi.String("t2.micro"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.Ec2Functions;
* import com.pulumi.aws.ec2.inputs.GetAmiArgs;
* import com.pulumi.aws.ec2.LaunchConfiguration;
* import com.pulumi.aws.ec2.LaunchConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var ubuntu = Ec2Functions.getAmi(GetAmiArgs.builder()
* .mostRecent(true)
* .filters(
* GetAmiFilterArgs.builder()
* .name("name")
* .values("ubuntu/images/hvm-ssd/ubuntu-trusty-14.04-amd64-server-*")
* .build(),
* GetAmiFilterArgs.builder()
* .name("virtualization-type")
* .values("hvm")
* .build())
* .owners("099720109477")
* .build());
* var asConf = new LaunchConfiguration("asConf", LaunchConfigurationArgs.builder()
* .name("web_config")
* .imageId(ubuntu.applyValue(getAmiResult -> getAmiResult.id()))
* .instanceType("t2.micro")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* asConf:
* type: aws:ec2:LaunchConfiguration
* name: as_conf
* properties:
* name: web_config
* imageId: ${ubuntu.id}
* instanceType: t2.micro
* variables:
* ubuntu:
* fn::invoke:
* Function: aws:ec2:getAmi
* Arguments:
* mostRecent: true
* filters:
* - name: name
* values:
* - ubuntu/images/hvm-ssd/ubuntu-trusty-14.04-amd64-server-*
* - name: virtualization-type
* values:
* - hvm
* owners:
* - '099720109477'
* ```
*
* ## Import
* Using `pulumi import`, import launch configurations using the `name`. For example:
* ```sh
* $ pulumi import aws:ec2/launchConfiguration:LaunchConfiguration as_conf pulumi-lg-123456
* ```
*/
public class LaunchConfiguration internal constructor(
override val javaResource: com.pulumi.aws.ec2.LaunchConfiguration,
) : KotlinCustomResource(javaResource, LaunchConfigurationMapper) {
/**
* The Amazon Resource Name of the launch configuration.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Associate a public ip address with an instance in a VPC.
*/
public val associatePublicIpAddress: Output?
get() = javaResource.associatePublicIpAddress().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Additional EBS block devices to attach to the instance. See Block Devices below for details.
*/
public val ebsBlockDevices: Output>
get() = javaResource.ebsBlockDevices().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> launchConfigurationEbsBlockDeviceToKotlin(args0) })
})
})
/**
* If true, the launched EC2 instance will be EBS-optimized.
*/
public val ebsOptimized: Output
get() = javaResource.ebsOptimized().applyValue({ args0 -> args0 })
/**
* Enables/disables detailed monitoring. This is enabled by default.
*/
public val enableMonitoring: Output?
get() = javaResource.enableMonitoring().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Customize Ephemeral (also known as "Instance Store") volumes on the instance. See Block Devices below for details.
*/
public val ephemeralBlockDevices: Output>?
get() = javaResource.ephemeralBlockDevices().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
launchConfigurationEphemeralBlockDeviceToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The name attribute of the IAM instance profile to associate with launched instances.
*/
public val iamInstanceProfile: Output?
get() = javaResource.iamInstanceProfile().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The EC2 image ID to launch.
*/
public val imageId: Output
get() = javaResource.imageId().applyValue({ args0 -> args0 })
/**
* The size of instance to launch.
* The following arguments are optional:
*/
public val instanceType: Output
get() = javaResource.instanceType().applyValue({ args0 -> args0 })
/**
* The key name that should be used for the instance.
*/
public val keyName: Output
get() = javaResource.keyName().applyValue({ args0 -> args0 })
/**
* The metadata options for the instance.
*/
public val metadataOptions: Output
get() = javaResource.metadataOptions().applyValue({ args0 ->
args0.let({ args0 ->
launchConfigurationMetadataOptionsToKotlin(args0)
})
})
/**
* The name of the launch configuration. If you leave this blank, this provider will auto-generate a unique name. Conflicts with `name_prefix`.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Creates a unique name beginning with the specified prefix. Conflicts with `name`.
*/
public val namePrefix: Output
get() = javaResource.namePrefix().applyValue({ args0 -> args0 })
/**
* The tenancy of the instance. Valid values are `default` or `dedicated`, see [AWS's Create Launch Configuration](http://docs.aws.amazon.com/AutoScaling/latest/APIReference/API_CreateLaunchConfiguration.html) for more details.
*/
public val placementTenancy: Output?
get() = javaResource.placementTenancy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Customize details about the root block device of the instance. See Block Devices below for details.
*/
public val rootBlockDevice: Output
get() = javaResource.rootBlockDevice().applyValue({ args0 ->
args0.let({ args0 ->
launchConfigurationRootBlockDeviceToKotlin(args0)
})
})
/**
* A list of associated security group IDS.
*/
public val securityGroups: Output>?
get() = javaResource.securityGroups().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The maximum price to use for reserving spot instances.
*/
public val spotPrice: Output?
get() = javaResource.spotPrice().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The user data to provide when launching the instance. Do not pass gzip-compressed data via this argument; see `user_data_base64` instead.
*/
public val userData: Output?
get() = javaResource.userData().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Can be used instead of `user_data` to pass base64-encoded binary data directly. Use this instead of `user_data` whenever the value is not a valid UTF-8 string. For example, gzip-encoded user data must be base64-encoded and passed via this argument to avoid corruption.
*/
public val userDataBase64: Output?
get() = javaResource.userDataBase64().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
}
public object LaunchConfigurationMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.aws.ec2.LaunchConfiguration::class == javaResource::class
override fun map(javaResource: Resource): LaunchConfiguration = LaunchConfiguration(
javaResource
as com.pulumi.aws.ec2.LaunchConfiguration,
)
}
/**
* @see [LaunchConfiguration].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [LaunchConfiguration].
*/
public suspend fun launchConfiguration(
name: String,
block: suspend LaunchConfigurationResourceBuilder.() -> Unit,
): LaunchConfiguration {
val builder = LaunchConfigurationResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [LaunchConfiguration].
* @param name The _unique_ name of the resulting resource.
*/
public fun launchConfiguration(name: String): LaunchConfiguration {
val builder = LaunchConfigurationResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy